Mastering the Strategy Pattern in Java 8: Common Pitfalls
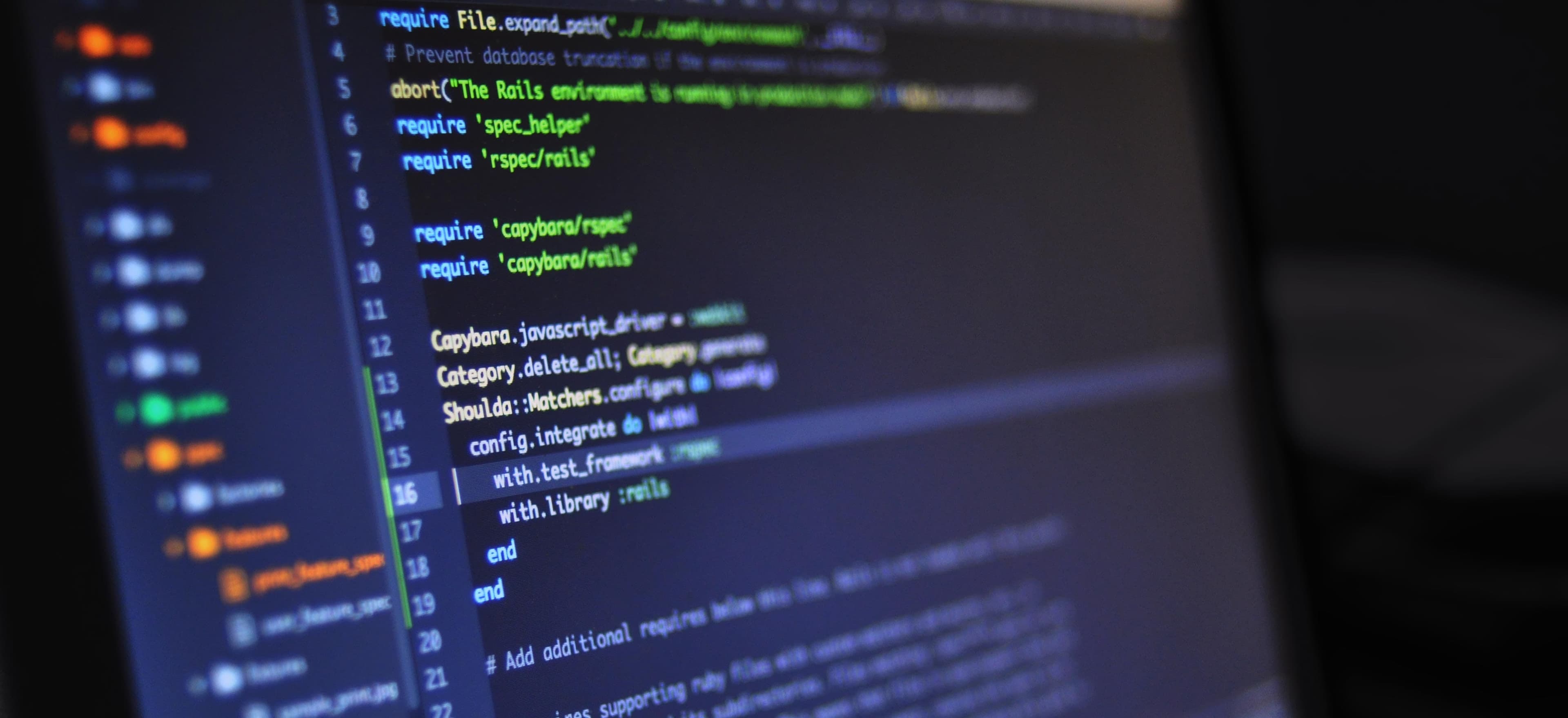
- Published on
Mastering the Strategy Pattern in Java 8: Common Pitfalls
When working with design patterns, the Strategy Pattern stands out for its ability to enable flexible and interchangeable algorithms. This capability is especially relevant in Java 8, which introduced lambdas and improved functional programming features. However, while it provides great opportunities, the pattern can be misapplied or misunderstood, leading to common pitfalls. In this post, we will delve into the Strategy Pattern, illustrate its implementation with code snippets, and highlight typical mistakes to avoid.
What is the Strategy Pattern?
The Strategy Pattern is a behavioral design pattern that enables selecting an algorithm's behavior at runtime. It defines a family of algorithms, encapsulates each one, and makes them interchangeable. As a result, the client code can choose which algorithm to use without depending on the specific details of the implementation.
Key Benefits
- Flexibility: Easily switch between different algorithms based on the context.
- Separation of Concerns: Encapsulates algorithms into separate classes, enhancing maintainability.
- Open/Closed Principle: You can add new strategies without modifying the existing codebase.
Implementing the Strategy Pattern
Let's illustrate the Strategy Pattern with a simple example: a payment system where users can choose different payment methods, such as credit card and PayPal.
Step 1: Define the Strategy Interface
Every strategy should implement the same interface. This allows clients to use different strategies interchangeably.
public interface PaymentStrategy {
void pay(int amount);
}
Step 2: Create Concrete Strategies
Now we can create concrete implementations of the PaymentStrategy
.
public class CreditCardPayment implements PaymentStrategy {
private String cardNumber;
public CreditCardPayment(String cardNumber) {
this.cardNumber = cardNumber;
}
@Override
public void pay(int amount) {
System.out.println("Paid " + amount + " using Credit Card: " + cardNumber);
}
}
public class PaypalPayment implements PaymentStrategy {
private String email;
public PaypalPayment(String email) {
this.email = email;
}
@Override
public void pay(int amount) {
System.out.println("Paid " + amount + " using PayPal: " + email);
}
}
Why Split Implementations?
By creating separate classes for each payment method, we achieve better modularity. If a new payment method is introduced (say Bitcoin), we can add it without modifying existing code.
Step 3: Create a Context Class
The Context class holds a reference to the PaymentStrategy
. This class will use the strategy to execute the payment.
public class ShoppingCart {
private PaymentStrategy paymentStrategy;
public void setPaymentStrategy(PaymentStrategy paymentStrategy) {
this.paymentStrategy = paymentStrategy;
}
public void checkout(int amount) {
paymentStrategy.pay(amount);
}
}
Example Usage
Let's see how the strategy works in action.
public class StrategyPatternDemo {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
cart.setPaymentStrategy(new CreditCardPayment("1234-5678-9101-1121"));
cart.checkout(500);
cart.setPaymentStrategy(new PaypalPayment("user@example.com"));
cart.checkout(300);
}
}
Understanding Java 8 Enhancements
Java 8 enables us to leverage lambdas, making our strategy implementation more concise. Let's see how we can refactor our PaymentStrategy
interface.
Functional Interface with Lambdas
The PaymentStrategy
can be defined as a functional interface, allowing for a cleaner implementation using a lambda expression.
@FunctionalInterface
public interface PaymentStrategy {
void pay(int amount);
}
Refactoring Strategies with Lambdas
Now instead of concrete classes, we can use lambda expressions:
PaymentStrategy creditCardPayment = amount ->
System.out.println("Paid " + amount + " using Credit Card");
PaymentStrategy paypalPayment = amount ->
System.out.println("Paid " + amount + " using PayPal");
Usage in Context
You can implement strategy selection just as before:
public class LambdaStrategyDemo {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
// Using lambda for CreditCard payment
cart.setPaymentStrategy(amount -> System.out.println("Paid " + amount + " using Credit Card"));
cart.checkout(500);
// Using lambda for PayPal payment
cart.setPaymentStrategy(amount -> System.out.println("Paid " + amount + " using PayPal"));
cart.checkout(300);
}
}
Common Pitfalls in the Strategy Pattern
The Strategy Pattern is powerful, but it is essential to implement it carefully. Here are some common pitfalls to avoid:
1. Overcomplicating Simple Scenarios
Pitfall: Implementing the Strategy Pattern when a simple if-else
or switch
statement would suffice.
Solution: Evaluate whether the complexity of the Strategy Pattern is warranted. If you only have two or three types of algorithms that are unlikely to change, simpler control structures might be sufficient.
2. Lack of Proper Context
Pitfall: Not defining the context where the strategies work can lead to confusion.
Solution: Always provide a clear context class that interacts with the strategies. Collating their behavior in a single client class helps maintain clarity.
3. Ignoring the Open/Closed Principle
Pitfall: Modifying existing strategies rather than adding new ones.
Solution: Follow the Open/Closed Principle by creating new strategy classes instead of altering existing ones. This prevents breaking changes in your codebase.
4. Using State in Strategies
Pitfall: Utilizing mutable state within strategy classes reduces the interchangeability of strategies.
Solution: Favor stateless strategies whenever possible. If you need to maintain state, consider passing the required data through parameters.
5. Neglecting Test Coverage
Pitfall: Not testing each strategy separately.
Solution: Write unit tests for each strategy implementation to ensure they work as expected. This strategy makes it easier to spot and fix bugs.
Lessons Learned
The Strategy Pattern is a valuable tool to streamline algorithm selection in your Java applications. When combined with Java 8's functional programming features, it becomes even more versatile. However, understanding and avoiding common pitfalls is crucial for effective implementation.
By keeping code modular, clear, and well-structured, you'll enhance maintainability and extensibility in your applications. For further reading on design patterns in Java, check out resources like Refactoring Guru or the book Design Patterns: Elements of Reusable Object-Oriented Software.
Master the Strategy Pattern, and unlock a myriad of possibilities for your Java applications!
Checkout our other articles