Decoding JUnit Test Results: A Programmatic Approach
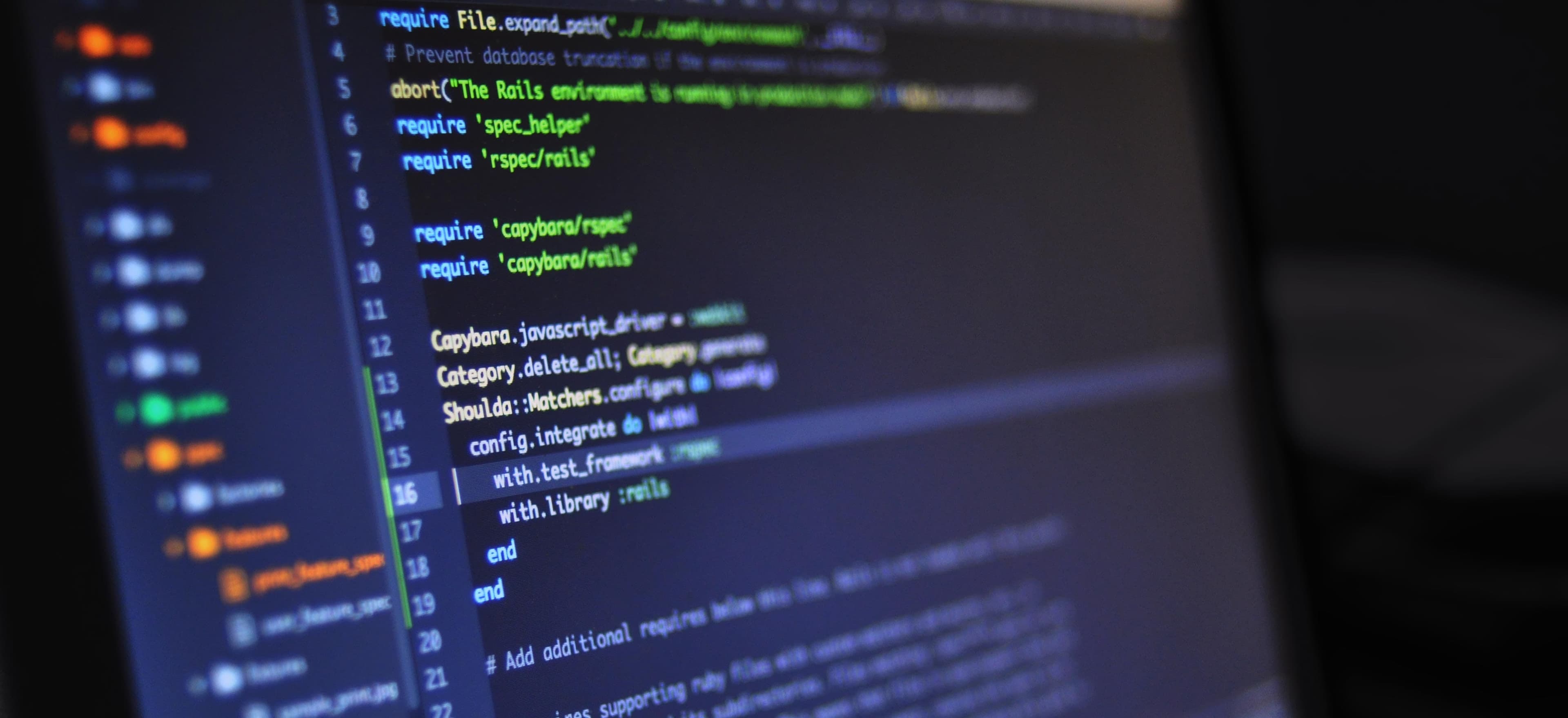
- Published on
Decoding JUnit Test Results: A Programmatic Approach
In the world of Java development, ensuring that your code behaves as expected is paramount. An essential part of this process is unit testing, which is often facilitated by powerful frameworks like JUnit. But what happens after you run your tests? How do you interpret and utilize the results? In this blog, we will delve into decoding JUnit test results programmatically, enabling you to enhance your testing strategy and overall code quality.
Understanding JUnit Test Results
JUnit provides various output formats for test results, which can be easily integrated into broader analysis workflows. The standard output contains information about which tests passed, failed, or were ignored. Moreover, JUnit can generate XML reports that further detail the execution of your test cases.
Sample Output from JUnit
When you run your tests, you might see output similar to this:
Tests run: 5, Failures: 1, Errors: 0, Skipped: 0, Time elapsed: 0.789 sec
- Tests run: Total number of tests executed.
- Failures: Number of tests that did not pass.
- Errors: Tests that encountered an error during execution.
- Skipped: Tests that were ignored due to conditions.
- Time elapsed: Total time taken to execute tests.
Understanding these metrics can help you evaluate the reliability of your codebase.
Generating XML Reports
JUnit can generate XML reports using its built-in tools. These XML files encapsulate detailed information regarding every test run, making them perfect for programmatic analysis. The format is widely adopted and can be integrated into continuous integration pipelines, enabling automated quality control.
Enabling XML Output
Here's how you can configure JUnit to produce XML reports:
import org.junit.runner.JUnitCore;
import org.junit.runner.Result;
import org.junit.runner.notification.Failure;
public class TestRunner {
public static void main(String[] args) {
Result result = JUnitCore.runClasses(YourTestClass.class);
if (result.getFailureCount() > 0) {
System.out.println("Tests failed:");
for (Failure failure : result.getFailures()) {
System.out.println(failure.toString());
}
} else {
System.out.println("All tests passed.");
}
// Here, you can add logic to generate XML reports as needed.
}
}
This code snippet serves as a gateway for executing JUnit tests programmatically while capturing test results.
Parsing XML Reports Programmatically
Once you have generated the XML output, you can parse it using Java's built-in libraries (like javax.xml.parsers
) or a third-party library like Jdom or Dom4j.
Sample XML Parsing
Here’s a simple example using Java's DOM parser to read a JUnit XML report:
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
import org.w3c.dom.NodeList;
import org.w3c.dom.Element;
import java.io.File;
public class JUnitResultsParser {
public static void main(String[] args) {
try {
File inputFile = new File("path/to/junit-results.xml");
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder dBuilder = dbFactory.newDocumentBuilder();
Document doc = dBuilder.parse(inputFile);
doc.getDocumentElement().normalize();
NodeList testSuites = doc.getElementsByTagName("testsuite");
for (int i = 0; i < testSuites.getLength(); i++) {
Element suite = (Element) testSuites.item(i);
System.out.println("Suite Name: " + suite.getAttribute("name"));
System.out.println("Tests Count: " + suite.getAttribute("tests"));
System.out.println("Failures Count: " + suite.getAttribute("failures"));
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Explanation of Code
This snippet reads a JUnit XML report, extracting basic statistics on the test suite, including the number of tests and failures. Here's what each part does:
- DocumentBuilderFactory and DocumentBuilder initialize the XML parsing.
- getElementsByTagName retrieves all test suite entries.
- For each suite, relevant attributes (like name and counts) are printed out.
Why Parse XML?
Parsing XML allows developers to leverage test results beyond just visual inspection. By analyzing the data programmatically, you can:
- Automate reporting for continuous integration setups.
- Track metrics over time to assess code quality.
- Provide dashboards or notifications based on the test results.
Leveraging Third-Party Libraries
While Java's standard library for XML parsing is powerful, third-party libraries can often simplify tasks significantly. Libraries such as Jackson and Gson can help serialize XML to JSON format, making it easier to manipulate in modern applications.
An Example Using Jackson
If you're using Jackson to parse your XML, your code might look like this:
import com.fasterxml.jackson.dataformat.xml.XmlMapper;
import java.io.File;
import java.io.IOException;
public class JUnitResultsJackson {
public static void main(String[] args) {
XmlMapper xmlMapper = new XmlMapper();
try {
TestSuite testSuite = xmlMapper.readValue(new File("path/to/junit-results.xml"), TestSuite.class);
System.out.println("Suite Name: " + testSuite.getName());
System.out.println("Tests Count: " + testSuite.getTests());
System.out.println("Failures Count: " + testSuite.getFailures());
} catch (IOException e) {
e.printStackTrace();
}
}
}
Defining the TestSuite Class
You would also need to define the TestSuite
class to match the XML structure:
import com.fasterxml.jackson.annotation.JsonProperty;
public class TestSuite {
@JsonProperty("name")
private String name;
@JsonProperty("tests")
private int tests;
@JsonProperty("failures")
private int failures;
// Getters and setters
}
In Conclusion, Here is What Matters
Decoding JUnit test results programmatically unlocks a new realm of possibilities for Java developers. From generating automated reports to establishing quality metrics over time, understanding your test outcomes is crucial for maintaining a high-quality codebase.
By utilizing XML reports and parsing them efficiently, you can greatly enhance your testing strategies. For more intricate details on JUnit, you can check the official JUnit documentation or explore integration with tools like Maven for a complete CI/CD workflow.
Adapting these insights into your Java projects will not only improve your testing procedures but also cultivate a culture of quality in your coding practices. Happy coding!
Checkout our other articles