Overcoming Common Pitfalls in Declarative UI Design with Vaadin
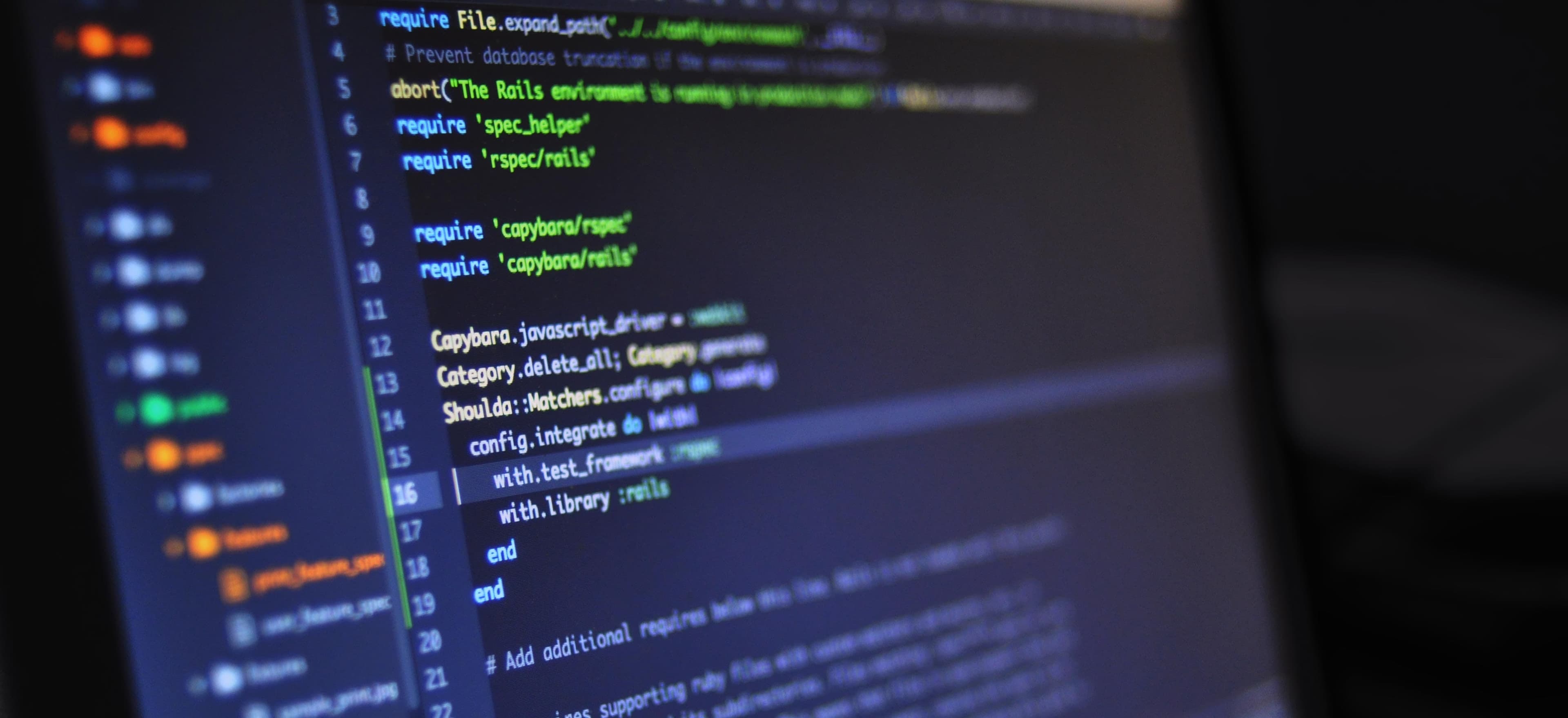
- Published on
Overcoming Common Pitfalls in Declarative UI Design with Vaadin
Declarative UI design has become a cornerstone of modern web applications. With the rise of frameworks like Vaadin, developers are empowered to build rich-user interfaces with ease. However, even with the advantages of a declarative approach, there are several common pitfalls that developers might encounter.
In this article, we will explore these pitfalls and offer practical solutions to help you navigate the landscape of declarative UI design in Vaadin.
What is Declarative UI Design?
Declarative UI design allows developers to define user interfaces using XML or other markup languages. This contrasts with imperative programming, where each UI component is instantiated and manipulated through code. A clear advantage of declarative design is that it promotes separation of concerns, making development more intuitive and maintainable.
Vaadin leverages this approach, providing a powerful framework to create complex web applications with less boilerplate code. However, there are challenges that accompany this simplified structure:
- Understanding the lifecycle of components.
- The risk of inefficient rendering.
- Overly complex layouts.
- Mismanagement of state.
We’ll delve into each of these pitfalls and outline strategies to overcome them.
1. Understanding the Lifecycle of Components
The Pitfall
One of the most common challenges in declarative UI design with Vaadin comes from misunderstanding the lifecycle of UI components. Each component goes through distinct phases, including creation, rendering, and destruction. Failure to grasp this lifecycle can lead to performance issues or unresponsive applications.
The Solution
Be proactive in learning how components are initialized and when their events are triggered. For example, leverage the @PostConstruct
annotation for initializing resources.
@Route("my-view")
public class MyView extends VerticalLayout {
@PostConstruct
public void init() {
add(new Button("Click Me", event -> {
Notification.show("Button clicked!");
}));
}
}
Why This Matters: Utilizing
@PostConstruct
ensures your component is set up with all necessary dependencies. Ignoring lifecycle events might introduce bugs that are hard to debug later.
For more details on Vaadin's component lifecycle, check out the official documentation.
2. The Risk of Inefficient Rendering
The Pitfall
While Vaadin is built to manage the rendering of UI components efficiently, developers sometimes inadvertently add components in a way that leads to unnecessary re-renders or layout recalculations. This can slow down the application, especially with complex views.
The Solution
Optimize rendering by using a combination of elements like Grid
or List
rather than generating components individually. Here's how you could efficiently render a list of items:
Grid<Person> grid = new Grid<>(Person.class);
grid.setItems(personService.findAll());
grid.setColumns("firstName", "lastName");
Why This Matters: The
Grid
component takes care of rendering efficiently, manages state, and reuses UI components when necessary, reducing overhead.
In cases where custom drawing is needed, make sure to implement the Renderer
interface to minimize unnecessary redraws while keeping your UI interactive.
3. Overly Complex Layouts
The Pitfall
Vaadin allows you to build complex UIs. However, this freedom can lead to convoluted layouts that are difficult to maintain. Nesting multiple layouts decisively can make your UI hard to navigate and modify.
The Solution
Keep your layout simple and modular. Use composite components wherever possible. Here is a simple example of constructing a modular layout:
public class UserCard extends VerticalLayout {
public UserCard(Person person) {
add(new Label(person.getFirstName() + " " + person.getLastName()));
add(new Button("View Details", e -> {
// Navigate to the details view
}));
}
}
// Usage in your main view
public class MainView extends VerticalLayout {
public MainView() {
for (Person person : personService.findAll()) {
add(new UserCard(person));
}
}
}
Why This Matters: By breaking components into smaller sections, you enhance maintainability and readability. This practice also promotes reusability across different views in your application.
4. Mismanagement of State
The Pitfall
In Vaadin applications, maintaining the state of components and views is crucial. Poor state management can lead to inconsistent UI behavior and confusing user interactions.
The Solution
Adopt a clear strategy for state management. Utilize Vaadin's built-in services for managing data. Here’s how you can manage user session data effectively:
@Route("profile")
public class ProfileView extends VerticalLayout {
public ProfileView() {
TextField nameField = new TextField("Name");
Button saveButton = new Button("Save", event -> {
VaadinSession.getCurrent().setAttribute("userName", nameField.getValue());
});
add(nameField, saveButton);
}
@BeforeEnter
public void beforeEnter() {
String userName = (String) VaadinSession.getCurrent().getAttribute("userName");
if (userName != null) {
nameField.setValue(userName);
}
}
}
Why This Matters: Using Vaadin's session management features allows for a smoother user experience by preserving the data across different views.
To Wrap Things Up
Declarative UI design in Vaadin offers immense potential for building scalable, modern web applications. However, a firm understanding of the lifecycle, rendering optimization, simplifying layouts, and state management is critical. By being aware of these pitfalls and implementing best practices, you can create more efficient and user-friendly applications.
Armed with these insights, venture forth into your Vaadin projects and embrace the elegance of declarative UI design!
For further reading on Vaadin's UI design principles, check out Vaadin's Best Practices.
Happy coding!
Checkout our other articles