Common Pitfalls When Building RESTful Services with Spring Boot
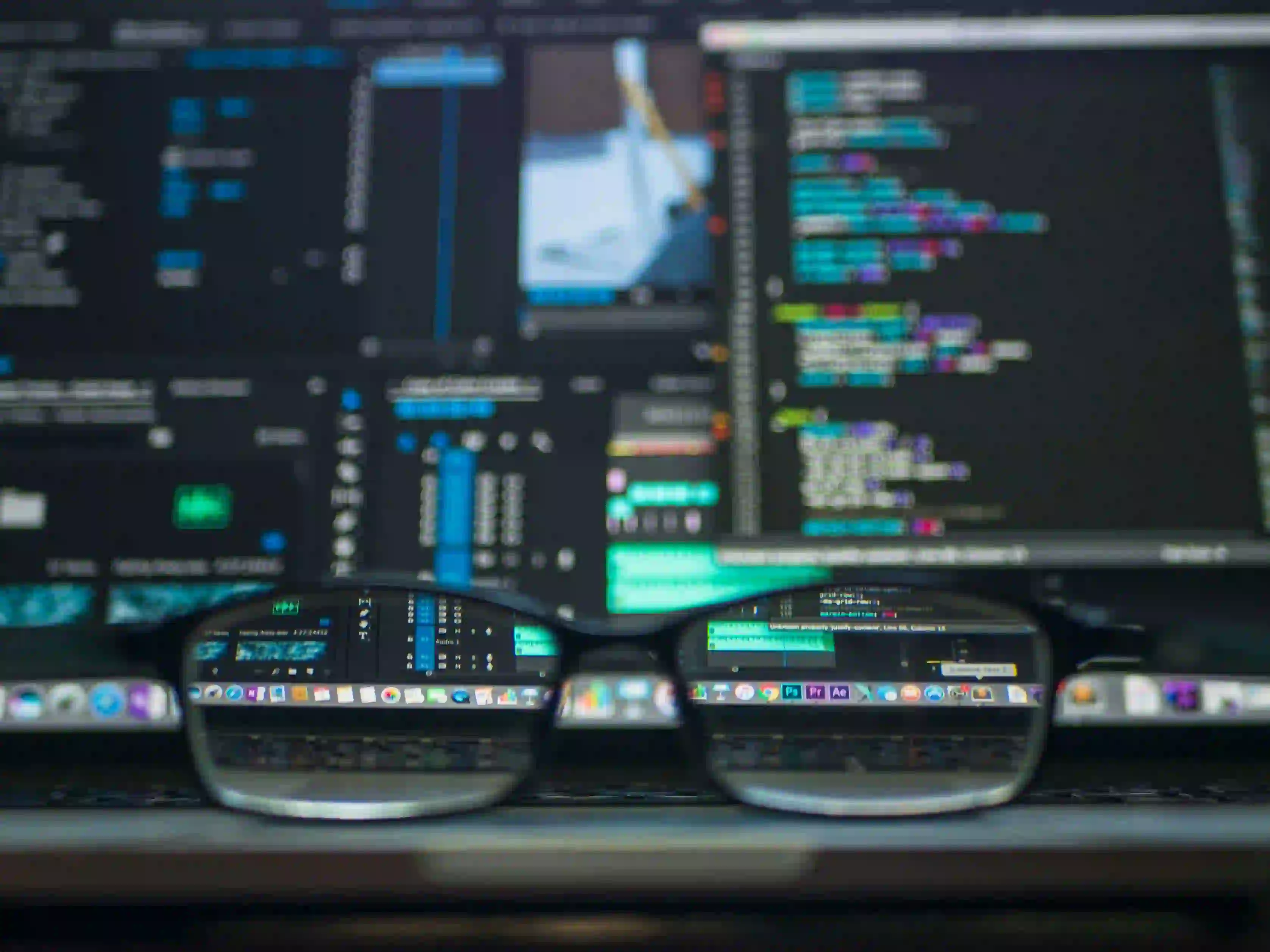
Common Pitfalls When Building RESTful Services with Spring Boot
Spring Boot has revolutionized the way we build RESTful services by simplifying architecture, configuration, and development. However, even with its advantages, developers often encounter challenges that can lead to inefficiencies or issues in their applications. This blog post discusses common pitfalls when building RESTful services using Spring Boot and offers insights on how to avoid them.
Table of Contents
- 1. Not Following REST Principles
- 2. Improper Error Handling
- 3. Ignoring HATEOAS
- 4. Overusing Annotations
- 5. Inefficient Database Access
- 6. Neglecting Security
- 7. Forgetting Documentation
- 8. Not Using Proper Caching
1. Not Following REST Principles
When building RESTful services, it's crucial to adhere to REST principles. This includes using proper resource-based URIs, HTTP methods, and status codes.
Why it matters:
Improper RESTful design can confuse users and lead to poor API usability.
Example:
Suppose you decide to update a user with a POST request instead of a PUT request.
@PostMapping("/users/{id}")
public ResponseEntity<User> updateUser(@PathVariable Long id, @RequestBody User user) {
// logic to update a user
}
This should instead utilize PUT for updates:
@PutMapping("/users/{id}")
public ResponseEntity<User> updateUser(@PathVariable Long id, @RequestBody User user) {
// logic to update user
}
Following the correct HTTP methods improves clarity and adherence to RESTful API standards.
2. Improper Error Handling
Error handling is often an overlooked aspect in RESTful services. Returning the appropriate status codes can provide a better experience for API consumers.
Why it matters:
Consumers of your API require clear information about success or failure.
Example:
Instead of returning a generic error message for all exceptions, use custom exceptions and response bodies.
@ExceptionHandler(UserNotFoundException.class)
public ResponseEntity<ErrorResponse> handleUserNotFound(UserNotFoundException ex) {
ErrorResponse errorResponse = new ErrorResponse("USER_NOT_FOUND", ex.getMessage());
return ResponseEntity.status(HttpStatus.NOT_FOUND).body(errorResponse);
}
This custom error response allows clients to understand specific issues more clearly.
3. Ignoring HATEOAS
HATEOAS (Hypermedia as the Engine of Application State) is a constraint of the REST application architecture. It promotes discovering actions dynamically through links.
Why it matters:
It enhances API usability by allowing users to navigate through the API without needing to know the full endpoint structure.
Example:
To implement HATEOAS in Spring, you can utilize EntityModel
from Spring HATEOAS.
@GetMapping("/users/{id}")
public EntityModel<User> getUser(@PathVariable Long id) {
User user = userService.findById(id);
EntityModel<User> resource = EntityModel.of(user);
WebMvcLinkBuilder linkToUsers = linkTo(methodOn(this.getClass()).getAllUsers());
resource.add(linkToUsers.withRel("all-users"));
return resource;
}
With this implementation, you’re allowing clients to discover related links, improving user experience.
4. Overusing Annotations
Spring offers numerous annotations, but over-reliance can lead to complexities and reduce readability.
Why it matters:
Too many annotations can create a tangled codebase that is hard to maintain.
Example:
Instead of using multiple annotations, consider concise configurations.
@RestController
@RequestMapping("/api/v1/users")
public class UserController {
// logic here
}
Keeping it simple ensures others can read and maintain the code effectively.
5. Inefficient Database Access
Connecting directly to the database layer in every service can lead to performance bottlenecks.
Why it matters:
Database efficiency is central to application performance. Long-running queries can slow down your API significantly.
Example:
Implement pagination or jOOQ for batch queries.
@GetMapping("/users")
public Page<User> getUsers(Pageable pageable) {
return userService.findAllUsers(pageable);
}
This approach minimizes server load and improves response times.
6. Neglecting Security
Security should be a primary focus when building RESTful services, yet it's often sidelined.
Why it matters:
Neglecting security can lead to severe vulnerabilities and exploitation.
Example:
Use Spring Security to restrict unauthorized access.
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/api/v1/users").authenticated()
.and()
.httpBasic();
}
}
By implementing basic authentication, you add an important layer of protection to your API.
7. Forgetting Documentation
Failing to document your API can lead to confusion and miscommunication among its users.
Why it matters:
Good documentation enhances the usability of your API and promotes better adoption.
Example:
Consider using Swagger to auto-generate API documentation.
@EnableSwagger2
@Configuration
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("com.example.api"))
.paths(PathSelectors.any())
.build();
}
}
Accessible documentation allows clients to learn how to use your API effectively.
8. Not Using Proper Caching
Caching is essential to improve performance, yet it’s commonly omitted in the architectural decisions.
Why it matters:
Caching can drastically reduce the load on your system and accelerate response times.
Example:
Applying caching annotations is straightforward.
@Cacheable("users")
@GetMapping("/users/{id}")
public User getUser(@PathVariable Long id) {
return userService.findById(id);
}
Caching retrieves users from memory when available, reducing database calls.
The Bottom Line
Building RESTful services using Spring Boot is both rewarding and challenging. By being aware of these common pitfalls and actively choosing to mitigate them, you can create robust, efficient, and maintainable services that provide great user experiences.
For more details on Spring Boot and RESTful services, you can refer to the official documentation Spring Boot Reference Documentation.
By combining your knowledge of Spring Boot with best practices, you'll position yourself to build APIs that are reliable and efficient. Remember, continuous learning and adapting to best practices is key to successful software development. Happy coding!