Mastering REST API: Top Interview Pitfalls to Avoid
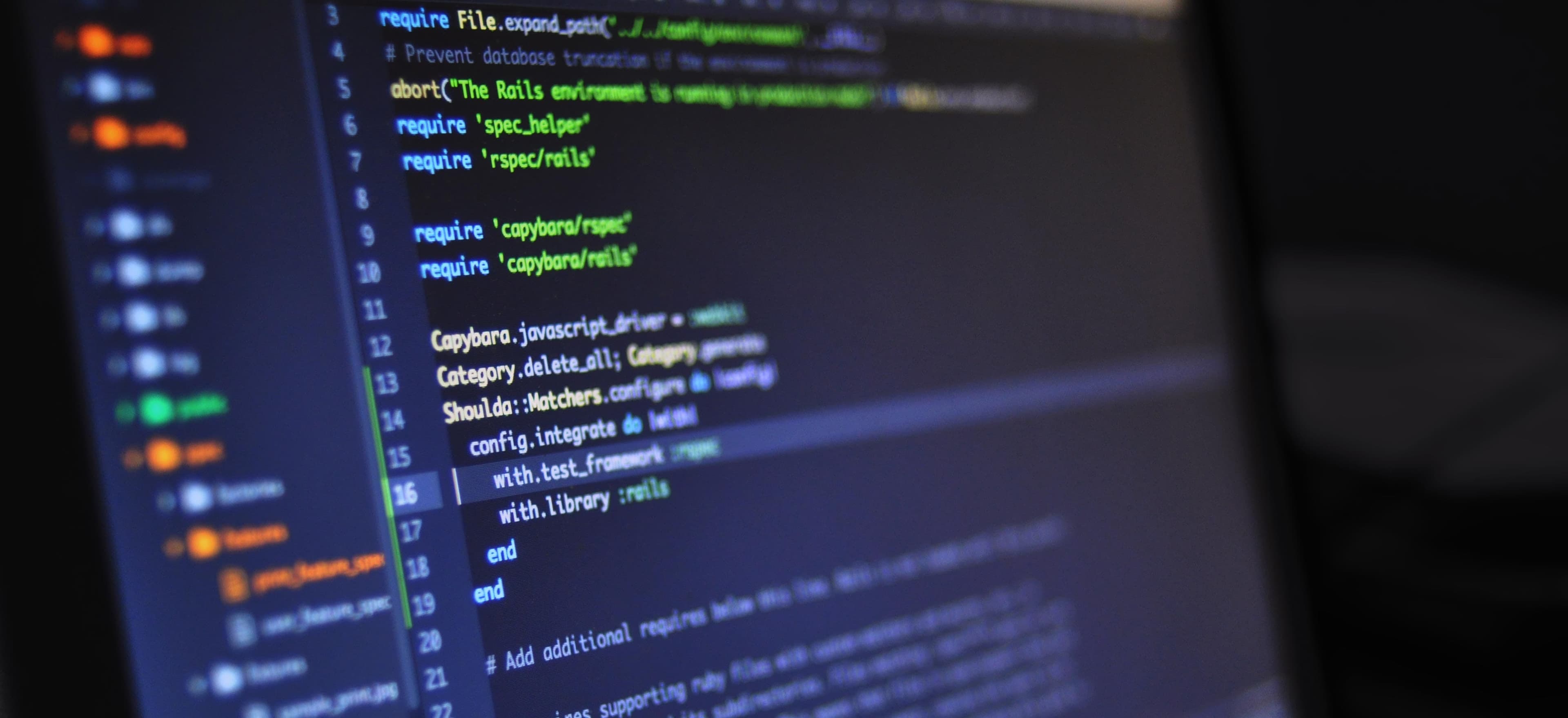
- Published on
Mastering REST API: Top Interview Pitfalls to Avoid
In the modern software development landscape, REST (Representational State Transfer) APIs are integral to creating scalable and maintainable web services. A solid understanding of REST APIs is crucial, especially if you're gearing up for a software development interview. This blog post will explore common pitfalls candidates face during REST API interviews and provide actionable insights to overcome these challenges.
What is a REST API?
Before diving into pitfalls, it's essential to understand what a REST API entails. REST is an architectural style that leverages standard HTTP methods for interfacing with web services. These methods include:
- GET: Retrieve data from the server
- POST: Send data to the server to create a resource
- PUT: Update an existing resource
- DELETE: Remove a resource
REST API Characteristics
A RESTful API adheres to several key principles:
- Statelessness: Each request from the client to the server must contain all the information needed to understand and process the request.
- Client-Server Architecture: The client and server are separate entities, allowing them to evolve independently.
- Cacheable: Responses from the server should be cacheable to improve performance.
- Uniform Interface: A consistent interface simplifies the architecture and decouples the client from the server.
Common Interview Pitfalls
Now that we've established a foundation, let's explore the top pitfalls to avoid during your REST API discussions in interviews.
1. Misunderstanding HTTP Methods
Candidates often confuse the purpose of various HTTP methods. For instance, the distinction between PUT and PATCH can be unclear.
Why This Matters: Using the wrong method can lead to unintended consequences. For instance, using PUT may overwrite existing data, whereas PATCH is used for partial updates.
Code Snippet Example
// Using PUT to update an entire resource
@RequestMapping(value = "/user/{id}", method = RequestMethod.PUT)
public ResponseEntity<User> updateUser(@PathVariable Long id, @RequestBody User userDetails) {
User user = userService.findById(id);
user.setName(userDetails.getName());
user.setEmail(userDetails.getEmail());
return ResponseEntity.ok(userService.save(user));
}
// Using PATCH to update a part of a resource
@RequestMapping(value = "/user/{id}", method = RequestMethod.PATCH)
public ResponseEntity<User> partialUpdateUser(@PathVariable Long id, @RequestBody Map<String, Object> updates) {
User user = userService.findById(id);
if (updates.containsKey("name")) {
user.setName((String) updates.get("name"));
}
return ResponseEntity.ok(userService.save(user));
}
2. Ignoring Status Codes
Failing to use the correct HTTP status codes in responses can confuse clients and hinder proper application behavior. Common status codes include:
- 200 OK: Successful GET request
- 201 Created: Successful resource creation
- 400 Bad Request: Client-side error
- 404 Not Found: Resource not available
- 500 Internal Server Error: Server-side error
Why This Matters: Correct status code usage provides the necessary context for clients to react appropriately to the response.
3. Not Understanding Resource Naming Conventions
REST APIs require thoughtful resource naming. Interviewees often make the mistake of poorly naming endpoints.
Why This Matters: An endpoint's name should clearly convey its purpose. For example, /getAllUsers
is less ideal than /users
, which adheres to REST practices of using nouns and pluralization.
Code Snippet Example
// Good Endpoint Naming
@RequestMapping(value = "/users", method = RequestMethod.GET)
public List<User> getAllUsers() {
return userService.findAll();
}
// Poor Endpoint Naming
@RequestMapping(value = "/getAllUsers", method = RequestMethod.GET)
public List<User> getAllUsersIrregular() {
return userService.findAll();
}
4. Lack of Versioning
Skipping API versioning can lead to breaking changes in future releases. Versioning is critical in maintaining backward compatibility.
Why This Matters: Without versioning, clients relying on the API might break with new changes, leading to significant operational issues. A typical approach would be to prefix the API endpoint with a version number.
Code Snippet Example
@RequestMapping(value = "/api/v1/users", method = RequestMethod.GET)
public List<User> getAllUsersV1() {
return userService.findAll();
}
@RequestMapping(value = "/api/v2/users", method = RequestMethod.GET)
public List<User> getAllUsersV2() {
return userService.findAllWithMoreDetails();
}
5. Neglecting Documentation
Failing to provide proper API documentation, such as using Swagger or OpenAPI, can be detrimental. Candidates often overlook the importance of clear documentation.
Why This Matters: Good documentation serves as a guide for developers consuming your API, enabling faster adoption and reducing miscommunication.
6. Overcomplicating Responses
Many candidates tend to return overly complex objects or include too much data in their API responses. Keeping responses concise is vital.
Why This Matters: Simplicity in API responses enhances performance and helps clients use your API more effectively.
Code Snippet Example
// Returning a simple response object
public class UserResponse {
private Long id;
private String name;
// Getters and Setters
}
@RequestMapping(value = "/user/{id}", method = RequestMethod.GET)
public ResponseEntity<UserResponse> getUser(@PathVariable Long id) {
User user = userService.findById(id);
UserResponse response = new UserResponse();
response.setId(user.getId());
response.setName(user.getName());
return ResponseEntity.ok(response);
}
Final Thoughts
Preparing for a REST API-related interview entails understanding both the technical aspects and best practices. Avoiding common pitfalls, such as misunderstanding HTTP methods, neglecting status codes, poor naming conventions, and lacking documentation, can significantly enhance your performance.
Familiarize yourself with these principles and ensure you can confidently articulate your knowledge during your interviews. With dedication and practice, you'll be well on your way to mastering REST APIs!
For further reading on REST APIs, check out RESTful API Design or explore Spring Boot documentation for practical implementations in Java. Happy coding!
Checkout our other articles