Mastering Lucene Analysis: Overcoming Common Pitfalls
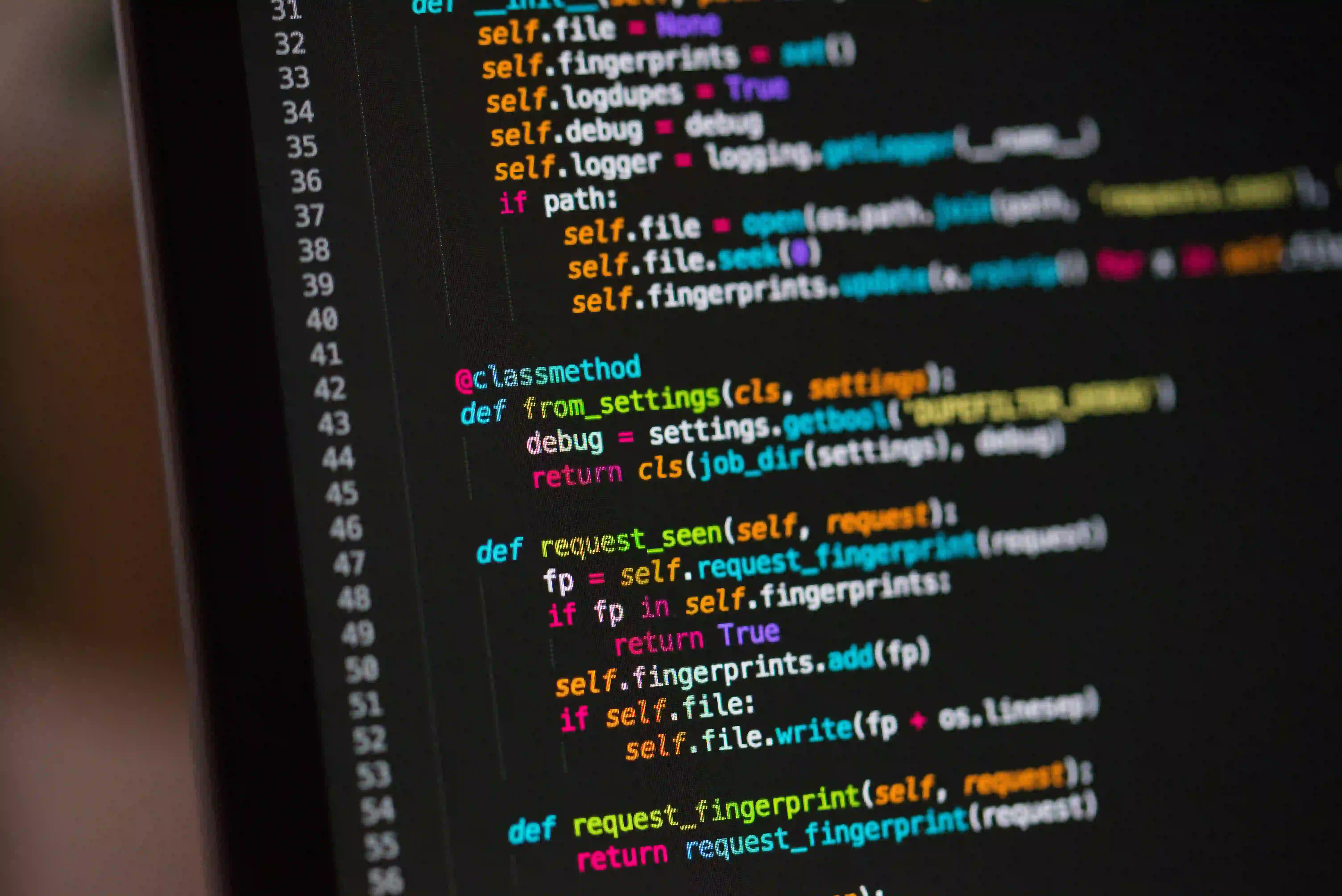
Mastering Lucene Analysis: Overcoming Common Pitfalls
When it comes to building search engines, Apache Lucene is a go-to choice for many developers. Its powerful text-search capabilities enable developers to index and search large volumes of text efficiently. However, Lucene analysis, which is the process of transforming text into a format suitable for searching, can be fraught with common pitfalls. In this blog post, we'll delve deep into Lucene analysis while discussing how to overcome these challenges effectively.
What is Lucene Analysis?
Lucene analysis is a critical component of the Lucene framework. It involves tokenizing text, removing stop words, normalizing terms, and applying various filters to ensure that search operations are fast and accurate. A proper understanding of Lucene analysis is vital for successful search implementations.
Key Components of Lucene Analysis
- Tokenizer: Breaks the input text into tokens (words).
- Token Filters: Processes the tokens to produce a cleaner set. This may include lowercasing, stemming, and removing unwanted terms.
- Analyzer: Combines tokenizer and filters into a single unit to process input text.
Setting Up Your Lucene Analyzer
Before addressing the common pitfalls, it's crucial to understand how to set up a Lucene analyzer. Here's a simple example of creating a custom analyzer using Lucene’s built-in capabilities.
import org.apache.lucene.analysis.Analyzer;
import org.apache.lucene.analysis.TokenStream;
import org.apache.lucene.analysis.standard.StandardAnalyzer;
import org.apache.lucene.analysis.tokenattributes.CharTermAttribute;
import java.io.IOException;
import java.io.StringReader;
public class SimpleAnalyzerExample {
public static void main(String[] args) {
String text = "Lucene is a powerful Java library for text search.";
Analyzer analyzer = new StandardAnalyzer();
try (TokenStream tokenStream = analyzer.tokenStream("fieldName", new StringReader(text))) {
CharTermAttribute charTermAttribute = tokenStream.addAttribute(CharTermAttribute.class);
tokenStream.reset();
while (tokenStream.incrementToken()) {
System.out.println(charTermAttribute.toString());
}
tokenStream.end();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary
In this example, we created a StandardAnalyzer
, which is great for most use cases. The TokenStream
fetches the tokens (words), and CharTermAttribute
helps see the actual token values. Using built-in analyzers like StandardAnalyzer
saves you from reinventing the wheel, allowing you to focus on more complex features of your search engine.
Common Pitfalls in Lucene Analysis
1. Inadequate Tokenization
Improper tokenization often leads to poor search results. For instance, ignoring punctuation or splitting words that should be kept together—like "New York"—can hinder search accuracy.
Solution: Always evaluate the tokenizer settings you are using. Extend or customize when necessary.
import org.apache.lucene.analysis.Tokenizer;
import org.apache.lucene.analysis.tokenattributes.CharTermAttribute;
public class CustomTokenizer extends Tokenizer {
private final CharTermAttribute charTermAttr = addAttribute(CharTermAttribute.class);
// Your custom implementation for tokenization
}
2. Ignoring Stop Words
Stop words (common words like "and," "the," "is") can clutter your index and degrade performance. While some analysts argue for their removal, models depending on precise search queries (e.g., legal documents) may require them.
Solution: Utilize built-in stop filter classes or create a custom list tailored to your domain.
import org.apache.lucene.analysis.core.StopFilter;
import org.apache.lucene.analysis.standard.StandardTokenizer;
TokenFilter filter = new StopFilter(TokenStream input, StopAnalyzer.ENGLISH_STOP_WORDS_SET);
3. Not Using Stemming
Stemming allows you to treat different forms of a word as equivalent. For example, "running," "ran," and "run" should all match.
Solution: Include a stemming filter in your analysis pipeline.
import org.apache.lucene.analysis.en.EnglishMinimalStemFilter;
TokenStream tokenStream = new EnglishMinimalStemFilter(inputTokenStream);
4. Lack of Case Normalization
Search engines should be case-insensitive to match user expectations. Failing to normalize cases can create scenarios where "Java" and "java" are treated differently.
Solution: Integrate a lowercase filter into your analyzer.
import org.apache.lucene.analysis.core.LowerCaseFilter;
TokenStream lowerCaseTokenStream = new LowerCaseFilter(inputTokenStream);
5. Failing to Test Analyzer Effectiveness
Developers often overlook the importance of testing the analyzer to confirm it works as expected.
Solution: Implement a testing strategy that evaluates how well your analyzer handles various text inputs.
@Test
public void testAnalyzer() {
Analyzer analyzer = new CustomAnalyzer();
// Run tests with different inputs to evaluate performance
}
6. Static Analyzer Configuration
Some developers opt for a static configuration that may not be optimal for all data types.
Solution: Dynamically configure your analyzer based on content type or context. Using multiple analyzers can significantly improve search relevance.
if (documentType.equals("text")) {
analyzer = new StandardAnalyzer();
} else if (documentType.equals("numeric")) {
analyzer = new NumericAnalyzer();
}
My Closing Thoughts on the Matter
Mastering Lucene analysis requires an insightful approach to its various components. By addressing common pitfalls—such as inadequate tokenization, ignoring stop words, and failing to implement case normalization—you can enhance the efficiency and effectiveness of your search application.
Lucene provides powerful tools to ensure success, but it is the nuances of how these tools are used that determine the overall effectiveness of your search feature.
For further reading, check out the official Apache Lucene documentation and explore additional resources like the Search Engine Optimization (SEO) Guide.
Remember, a well-crafted search experience can set your application apart. Keep experimenting, testing, and iterating until you achieve the desired results. Happy coding!