Overcoming Java EE Deployment Challenges on Apache Mesos
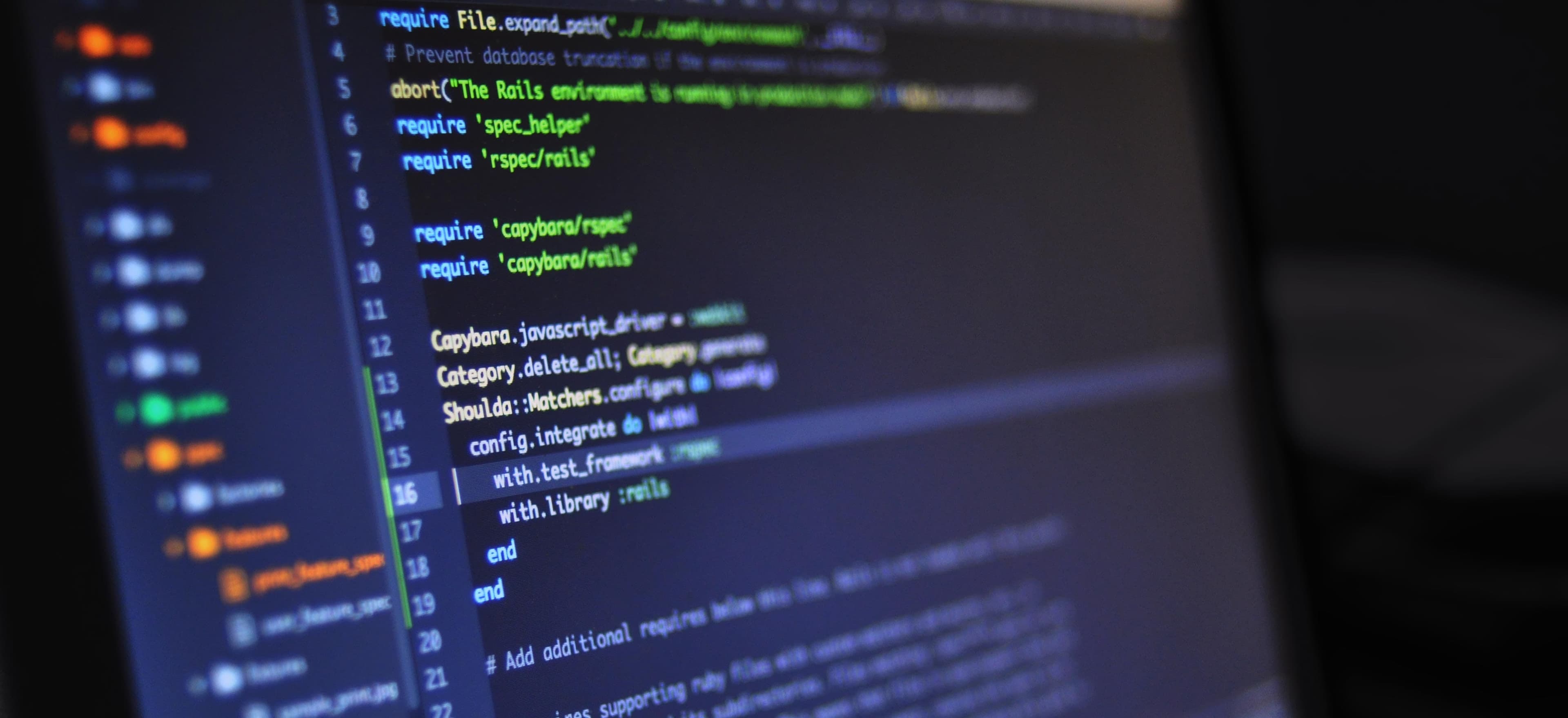
- Published on
Overcoming Java EE Deployment Challenges on Apache Mesos
The rise of microservices and cloud-native applications has transformed the landscape of software deployment. Java EE, a robust framework for building enterprise applications, faces unique challenges in this evolving environment. When combined with Apache Mesos—a powerful cluster manager for deploying and managing distributed applications—it presents its own deployment complications. In this blog post, we will explore these challenges and detail how to overcome them, enabling developers to effectively deploy Java EE applications on Apache Mesos.
Understanding Apache Mesos and Java EE
Before diving into the challenges, it is essential to clarify the roles of Apache Mesos and Java EE in the modern application landscape.
Apache Mesos is an open-source project that abstracts CPU, memory, storage, and other compute resources, enabling efficient resource sharing across distributed systems. In essence, it can run containers and frameworks like Kubernetes, Marathon, or Spark, making it a versatile tool for deploying applications on a large scale.
Java EE, on the other hand, provides an API and runtime environment for developing and running large-scale applications. Its built-in features for transaction management, security, and concurrency make it an excellent choice for complex applications, but deploying it in a microservices world can introduce several hurdles.
Common Deployment Challenges
1. Configuration Management
One of the primary hurdles in deploying Java EE applications on Mesos is managing configurations across different environments. Java EE applications often have environment-specific settings. Misconfigurations can lead to runtime failures or sub-optimal performance.
Solution
Use Docker containers to encapsulate environment details. Containers can package the application with its configuration settings, ensuring consistency across development, testing, and production environments.
# Dockerfile for a Java EE Application
FROM openjdk:11-jre-slim
VOLUME /tmp
COPY target/myapp.war /usr/local/tomcat/webapps/
EXPOSE 8080
ENTRYPOINT ["java", "-jar", "/usr/local/tomcat/webapps/myapp.war"]
In this Dockerfile, a Java EE application is packaged into a slim JRE container. This ensures that every environment runs the same version of the application, reducing errors caused by configuration mismatches.
For more insights on Docker and Java EE, refer to Dockerize your Java EE applications.
2. Resource Allocation
Java EE applications can be resource-intensive. On Apache Mesos, resource allocation needs to be effective; otherwise, performance issues can arise when applications compete for limited resources. Configuring memory and CPU can feel overwhelming.
Solution
Utilize Mesos’ resource management capabilities to define and limit the resources allocated to each application. This can be done using command-line flags when starting your application.
mesos-agent --resources=gpus:1,cpu:2,memory:2048
This command allocates 2 CPUs and 2048 MB of memory to your Java EE application. Adjusting these parameters based on the application load helps prevent contention issues.
3. Service Discovery
In the traditional deployment of Java EE applications, service discovery is often handled by a central registry. However, in a microservices architecture on Apache Mesos, where services might scale up and down dynamically, effective service discovery becomes crucial to ensure reliability.
Solution
Implement a service discovery pattern using tools like Consul or Eureka. These allow for dynamic discovery of services and can help direct requests to the correct service instance.
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
@EnableEurekaClient
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
In this example, the Spring Boot application is powered by the Eureka client, allowing it to automatically register itself upon starting, facilitating dynamic service discovery within Mesos.
For further reading on service discovery methods, check out Service Discovery in Spring Cloud.
Automating Deployments with CI/CD
Having tackled configuration, resource allocation, and service discovery challenges, let's shift our focus towards automating deployments. CI/CD (Continuous Integration/Continuous Deployment) is essential for quick and reliable releases.
Implementing CI/CD with Jenkins
Integrating Jenkins with your Java EE application can streamline the deployment process onto Apache Mesos.
- Jenkins Pipeline: Define a pipeline script for building and deploying your application.
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
sh 'mvn clean package'
}
}
}
stage('Docker Build') {
steps {
script {
sh 'docker build -t myapp .'
}
}
}
stage('Deploy to Mesos') {
steps {
script {
sh 'mesos-deploy myapp'
}
}
}
}
}
In this Jenkins pipeline, we encapsulate the build and deploy process into stages. This approach allows for automatic testing and verification before deployment, reducing the chances of introducing bugs into the production environment.
Monitoring and Logging
Once your Java EE applications are up and running on Apache Mesos, the next challenge is monitoring and logging. Since applications can have multiple instances distributed across a cluster, centralizing logs and metrics becomes vital for troubleshooting.
- Centralized Logging with ELK Stack: Consider using the ELK (Elasticsearch, Logstash, Kibana) stack to collect and visualize logs.
# logstash.conf
input {
file {
path => "/var/log/myapp/*.log"
start_position => "beginning"
}
}
filter {
grok {
match => { "message" => "%{COMBINEDAPACHELOG}" }
}
}
output {
elasticsearch {
hosts => ["elasticsearch:9200"]
index => "myapp-logs-%{+YYYY.MM.dd}"
}
}
In this configuration for Logstash, we configure it to read logs from the Java EE application, parse them, and send them to Elasticsearch.
In Conclusion, Here is What Matters
Deploying Java EE applications on Apache Mesos is undoubtedly challenging, yet manageable with the right strategies. From managing configurations and resources to implementing service discovery and CI/CD pipelines, each aspect contributes to a smoother deployment process.
Understanding these challenges and how to overcome them empowers developers to leverage the full potential of Java EE and Mesos effectively. Embrace these solutions, and you will step into a more agile and resilient software development lifecycle.
If you're interested in expanding your Java EE knowledge further, consider exploring official documentation and resources. The journey of mastering Java EE in distributed environments is ongoing—keep experimenting and learning!
By diving into the complexities of deploying Java EE applications on Apache Mesos, you will not only refine your technical skills but also enhance your understanding of modern development practices. Happy coding!