Handling Temporary Tables in JPA 2.1: Common Pitfalls
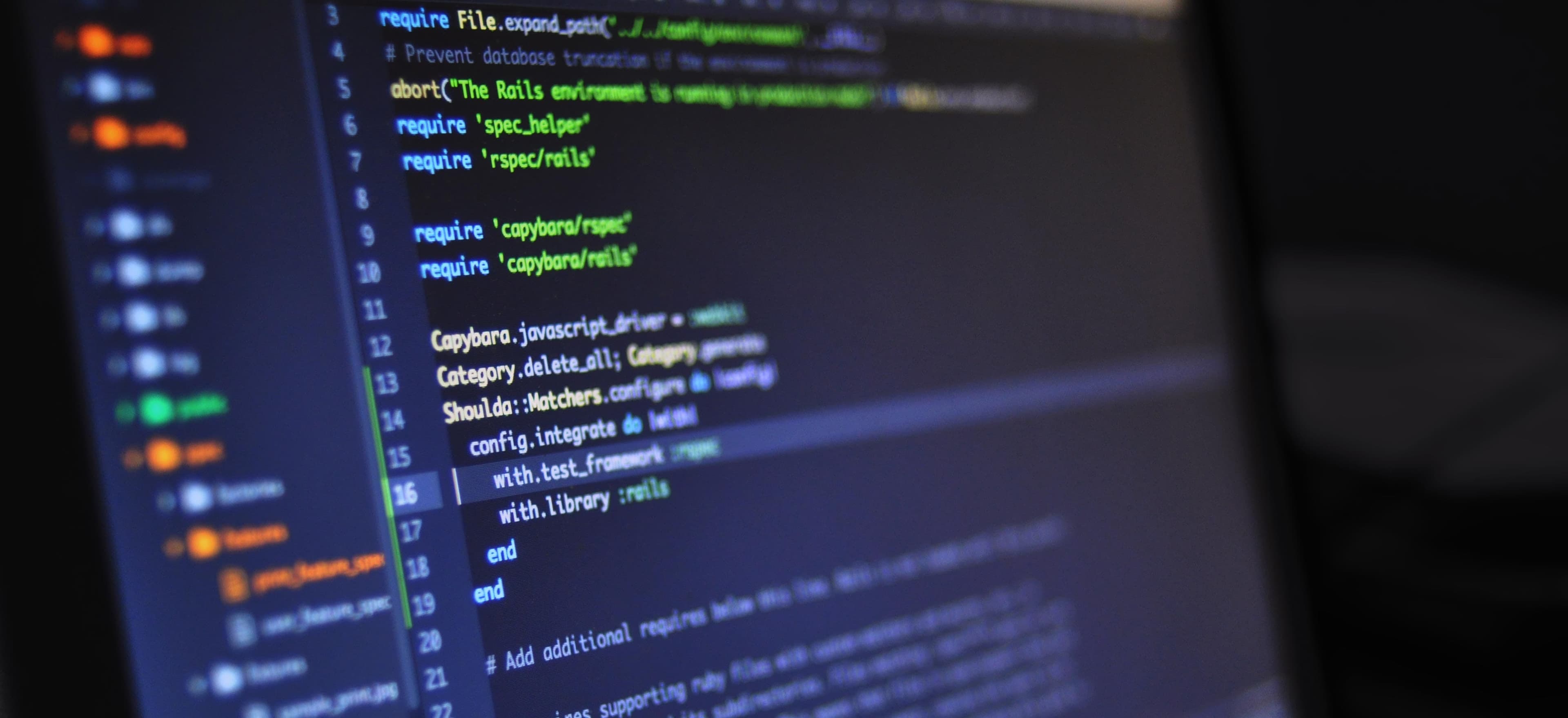
- Published on
Handling Temporary Tables in JPA 2.1: Common Pitfalls
Java Persistence API (JPA) has become a cornerstone of enterprise application development in the Java ecosystem. With its powerful features, JPA simplifies database interactions. However, when working with temporary tables, developers often encounter challenges. In this blog post, we will explore common pitfalls while handling temporary tables in JPA 2.1 and how to avoid them.
Understanding Temporary Tables
Temporary tables are a type of table that exists only for the duration of a database session or transaction. Unlike permanent tables, temporary tables are often used to store interim data during complex operations. They are particularly useful for performance optimization when handling large datasets.
In JPA, we typically work with entity classes that map to database tables. However, temporary tables pose unique challenges due to their transient nature. A proper understanding of JPA annotations, transaction management, and session handling is essential for effective use.
Common Pitfalls in Handling Temporary Tables
1. Assumption of Entity Persistence
One major pitfall is the assumption that temporary tables function like regular entity tables. In JPA, entities must be persistent to be managed by the context. Temporary tables, however, are transient and thus may not behave as expected.
Example:
@Entity
@Table(name = "temp_table", schema = "temp_schema")
public class TempEntity {
@Id
@GeneratedValue
private Long id;
private String data;
}
In this scenario, simply mapping TempEntity
to temp_table
will not guarantee persistence due to the nature of temporary tables. Therefore, it's crucial to use transactions wisely and execute database operations that accommodate non-persistent structures.
2. Ignoring Transaction Boundaries
Temporary tables are often tied to specific transactions. Failing to properly manage transaction boundaries can lead to unexpected results, such as stale data or unintended data loss.
Solution:
Always ensure operations on temporary tables occur within defined transaction boundaries.
EntityTransaction transaction = entityManager.getTransaction();
try {
transaction.begin();
// Your logic for using the temporary table
transaction.commit();
} catch (RuntimeException e) {
if (transaction.isActive()) {
transaction.rollback();
}
throw e; // Rethrow or handle accordingly
}
This structure ensures that the context remains in sync with the temporary table's lifecycle.
3. Overlooking the Context Scope
Another frequent oversight is the assumption that the EntityManager
scoped to the transaction will maintain the state of the temporary table. Due to the transient nature of these tables, you need to ensure the EntityManager
is fully aware of their state and lifecycle.
Important Note:
Ensure you establish the entity manager in the context where the temporary table is utilized.
4. Lack of Proper Cleanup
Neglecting to drop temporary tables can lead to unnecessary resource consumption. Automatic cleanup of these tables may not always work as intended, and developers often overlook this detail.
Recommended Practice:
Manually handle cleanup by executing a statement to drop the temporary table at the end of the session.
public void processDataWithTempTable() {
EntityTransaction transaction = entityManager.getTransaction();
try {
transaction.begin();
// Create temporary table and process data
entityManager.createNativeQuery("CREATE TEMPORARY TABLE temp_table (...);").executeUpdate();
// Perform operations using the temporary table
transaction.commit();
} catch (RuntimeException e) {
if (transaction.isActive()) {
transaction.rollback();
}
throw e; // Rethrow or handle accordingly
} finally {
// Cleanup
entityManager.createNativeQuery("DROP TABLE temp_table;").executeUpdate();
}
}
5. Not Using Native Queries
When working with temporary tables, using the JPA Criteria API or JPQL may not yield expected results. For complex operations involving temporary tables, resorting to native SQL queries is often the better option.
Native Query Example:
List<Object[]> results = entityManager.createNativeQuery(
"SELECT * FROM temp_table WHERE condition = :param")
.setParameter("param", value)
.getResultList();
Using native queries allows for greater flexibility and precise control over your SQL operations, especially when working with temporary tables.
6. Unaware of Database Vendor Limitations
Different database vendors have varied support for temporary tables and their behaviors. It’s essential to familiarize yourself with the specific limitations and features of the database you are working with. For example:
- Oracle: Temporary tables can be session-specific or transaction-specific.
- MySQL: Temporary tables are session-specific but misuse of transactions can lead to locks.
Always refer to the specific database documentation to understand constraints.
Final Considerations
Handling temporary tables in JPA 2.1 requires a nuanced approach due to the unique nature of these objects. By understanding the common pitfalls outlined above, developers can create more effective and robust applications. Here’s a brief recap:
- Do not assume temporary tables behave like persistent tables.
- Always manage transaction boundaries.
- Be aware of the EntityManager context when using temporary tables.
- Implement cleanup mechanisms to avoid resource leaks.
- Utilize native queries for operations involving temporary tables.
- Be cognizant of the limitations of your underlying database.
By keeping these lessons in mind, you can navigate the complexities of temporary table management in JPA, ensuring your applications are both efficient and reliable. For more in-depth reading, refer to the following resources:
- JPA Specification
- Java Persistence with Hibernate
- Oracle Temporary Tables Documentation
Embrace the challenges of JPA and temporary tables, and write code that is not only performant but also maintainable and clear. Happy coding!