Common JMS Configuration Issues in Apache TomEE
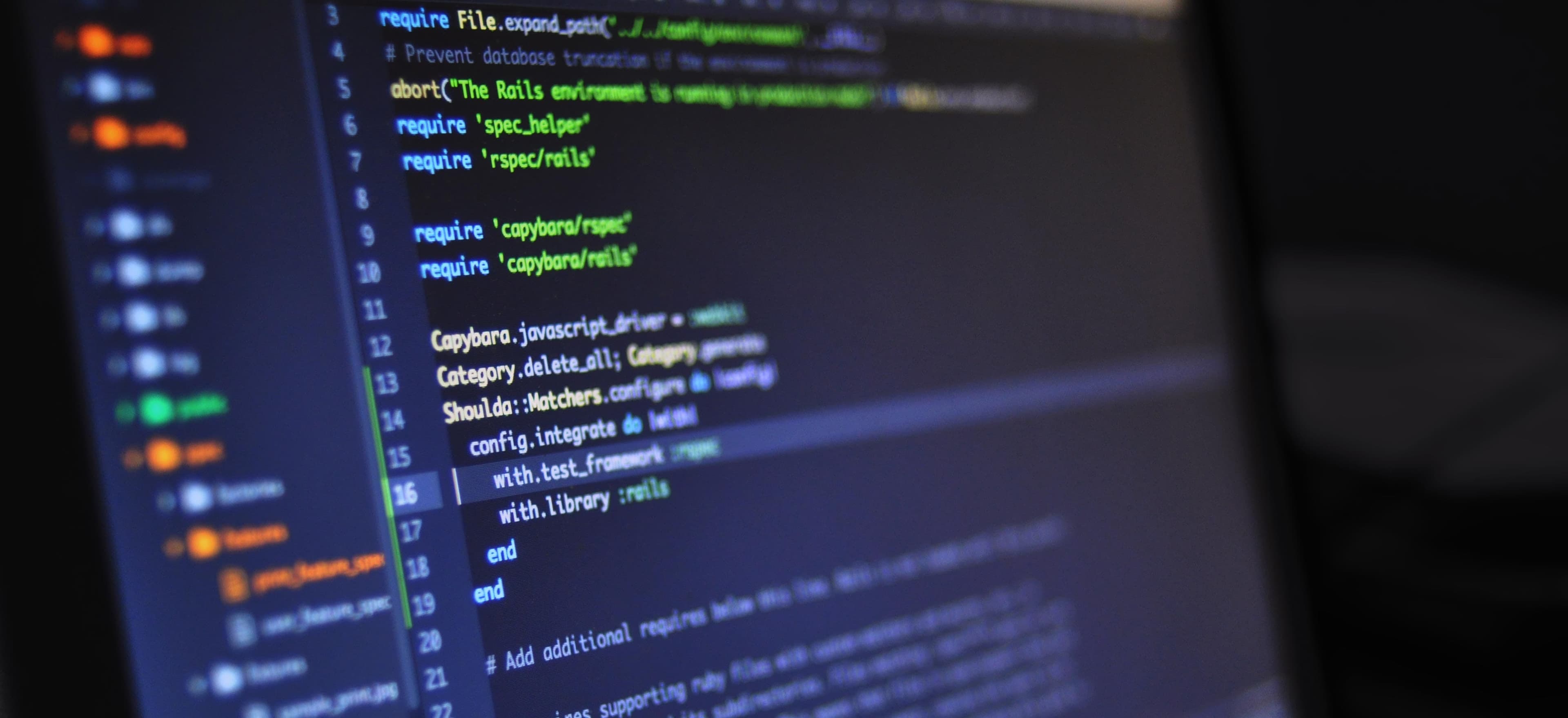
- Published on
Common JMS Configuration Issues in Apache TomEE
Apache TomEE is an open-source Java EE application server that extends Apache Tomcat while adding full support for Java EE features, including JMS (Java Message Service). While using JMS provides significant advantages for building robust, asynchronous applications, it can also bring about various configuration challenges. This blog post will explore some common JMS configuration issues in Apache TomEE and provide insights on how to resolve them.
Understanding JMS and Apache TomEE
Before diving into the configuration issues, let's ensure we have a solid grasp of what JMS is and how Apache TomEE integrates it. JMS is an API that allows application components to create, send, receive, and read messages. It is a key component for building scalable and reliable enterprise applications that require decoupled communication.
Apache TomEE not only supports JMS but also comes with several features such as container-managed transactions, resource management, and support for various messaging providers. Understanding these features will help you troubleshoot issues more effectively.
Common JMS Configuration Issues
1. Incorrect Resource Configuration
One of the most frequent problems developers encounter is the incorrect configuration of JMS resources in tomee.xml
. This configuration file is crucial for defining resources like connection factories and destinations.
Example Configuration
<Resource id="jms/MyConnectionFactory" type="javax.jms.ConnectionFactory">
ConnectionURL = tcp://localhost:61616
</Resource>
<Resource id="jms/MyQueue" type="javax.jms.Queue">
PhysicalName = myQueue
</Resource>
Why It Matters
If the resource IDs are not correctly referenced in your application, you might see javax.naming.NameNotFoundException
. Ensure all JNDI names accurately match those specified in tomee.xml
.
2. Resource Adapter Issues
Another common issue involves resource adapters not being correctly set up or defined. If you are using an external JMS provider like ActiveMQ, you need to ensure that the adequate resource adapter is correctly configured.
Example Configuration
<connector>
<name>jms</name>
<class>org.apache.activemq.ActiveMQConnectionFactory</class>
<properties>
<property name="brokerURL">tcp://localhost:61616</property>
</properties>
</connector>
Why It Matters
An improperly defined connector could result in failures when attempting to create connections. Make sure the classes and properties specified match the requirements of the external service you are using.
3. Connection Pool Problems
Connection pooling is vital in JMS to manage multiple simultaneous connections. Failing to configure connection pooling can lead to performance bottlenecks or even service disruptions.
Example Configuration
<Resource id="jms/MyConnectionFactory" type="org.apache.activemq.jms.pool.PooledConnectionFactory">
ConnectionURL = tcp://localhost:61616
MaxConnections = 10
MinConnections = 2
IdleTimeout = 30000
</Resource>
Why It Matters
A well-configured connection pool allows your application to scale while maintaining performance during peak times. Monitor actual connection usage and adjust the pool settings accordingly.
4. Transaction Management Issues
If you are using transactions, you need to ensure that your sessions and resource managers are correctly configured. Errors in transaction management could lead to message loss or duplicate processing.
Example of Transactional Code
@TransactionAttribute(TransactionAttributeType.REQUIRED)
public void sendMessage(String message) {
Connection connection = null;
Session session = null;
try {
connection = connectionFactory.createConnection();
session = connection.createSession(true, Session.SESSION_TRANSACTED);
Queue queue = session.createQueue("jms/MyQueue");
MessageProducer producer = session.createProducer(queue);
TextMessage textMessage = session.createTextMessage(message);
producer.send(textMessage);
session.commit(); // Ensures message is sent
} catch (JMSException e) {
// Handle the exception and possibly rollback
e.printStackTrace();
} finally {
// Close resources
if (session != null) {
session.close();
}
if (connection != null) {
connection.close();
}
}
}
Why It Matters
It's important to use session.commit()
correctly when operating in transactional mode. Using the wrong transaction attribute could lead to unexpected behaviors. Always ensure you rollback transactions in case of errors.
5. Classpath Conflicts
Classpath issues can arise when there are multiple versions of JMS libraries or when required libraries are missing. Ensure that your TomEE server has the necessary JMS libraries in its classpath.
How to Fix
- Validate your
pom.xml
if you are using Maven. Ensure dependencies do not have conflicts. - Use the
dependency:list
command to view included libraries.
Why It Matters
Classpath conflicts can cause ClassNotFoundException
or NoSuchMethodError
, leading to hard-to-diagnose issues.
Monitoring and Logging
Implementing a robust monitoring and logging strategy is essential for diagnosing JMS configuration issues. Apache TomEE supports JMX (Java Management Extensions), which provides insight into resource usage, including JMS connections and sessions.
Example JMX Configurations
Add JMX configurations in your tomee.xml
:
<jmx>
<property name="com.sun.management.jmxremote.port" value="9999"/>
<property name="com.sun.management.jmxremote.authenticate" value="false"/>
<property name="com.sun.management.jmxremote.ssl" value="false"/>
</jmx>
Why It Matters
By monitoring your application via JMX, you can quickly identify bottlenecks or erroneous behavior and adjust your JMS configuration accordingly.
The Last Word
In this blog post, we’ve explored some of the common JMS configuration issues encountered in Apache TomEE. From resource configuration to classpath conflicts, it's vital to ensure all aspects of your JMS setup are correct for optimum application performance.
References
- Apache TomEE Documentation
- Java Message Service (JMS) Specification
- ActiveMQ Documentation
Using the right configurations can make your application's messaging reliable and efficient. If you are facing any unique challenges, feel free to share them in the comments!
Checkout our other articles