Common Pitfalls in Logging Requests to Elasticsearch
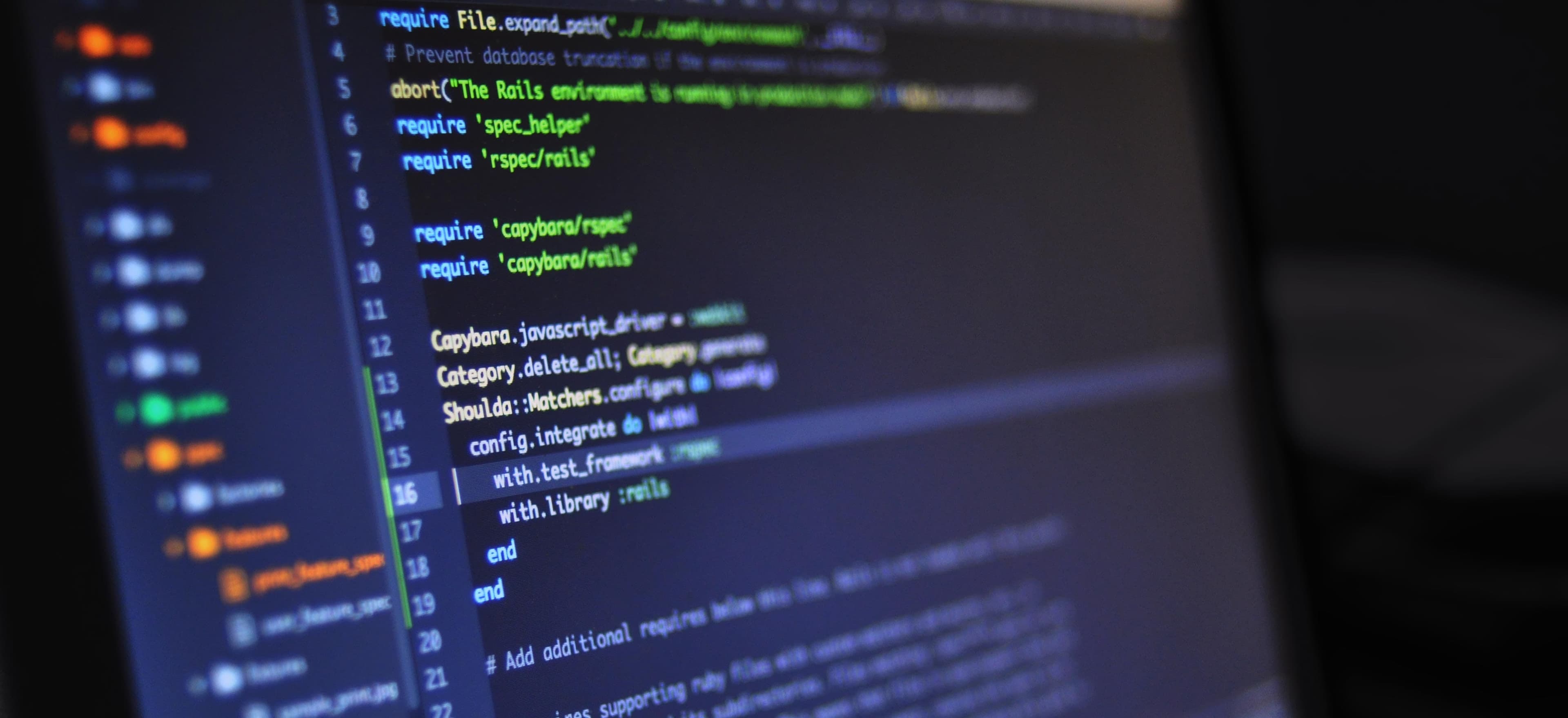
- Published on
Common Pitfalls in Logging Requests to Elasticsearch
Logging is a fundamental practice in software development that helps developers diagnose issues in their applications. When it comes to logging requests to Elasticsearch, the logs can provide insights into performance and request patterns. However, there are common pitfalls that developers encounter when implementing logging in conjunction with Elasticsearch. In this blog post, we will explore these pitfalls, discuss how to avoid them, and present some best practices for effective logging.
What is Elasticsearch?
Before diving into the common pitfalls, let's briefly understand what Elasticsearch is. Elasticsearch is a distributed search and analytics engine designed for handling large volumes of data. It is known for its powerful full-text search capabilities, real-time indexing, and near-instantaneous search among vast datasets. By logging requests to Elasticsearch, developers can monitor application performance and troubleshoot issues effectively.
Common Pitfalls in Logging Requests to Elasticsearch
1. Over-Logging
One of the most prominent pitfalls in logging is over-logging.
Why It's a Problem:
Over-logging can lead to a significant amount of data being generated. This makes it hard to identify relevant information, wastes storage space, and can even affect application performance.
Best Practices:
- Use Log Levels Wisely: Java provides various logging levels (e.g., TRACE, DEBUG, INFO, WARN, ERROR). Use them appropriately to balance detail and conciseness.
- Log What Matters: Focus on logging only essential data that helps in diagnosing issues.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyService {
private static final Logger logger = LoggerFactory.getLogger(MyService.class);
public void processRequest(Request request) {
logger.info("Processing request with ID: {}", request.getId());
// Additional processing logic
}
}
2. Ignoring Contextual Information
Context is crucial when logging requests. Ignoring important contextual information can render logs less useful for tracing issues.
Why It's a Problem:
Without context, it can be challenging to understand the circumstances around an error or warning.
Best Practices:
- Include Request Metadata: Log relevant metadata like user ID, IP address, and timestamps.
- Structured Logging: Consider using structured logging formats such as JSON, allowing for easier parsing and filtering of logs.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class RequestLogger {
private static final Logger logger = LoggerFactory.getLogger(RequestLogger.class);
public void logRequest(Request request) {
logger.info("Received request: {}", request.toJson());
}
}
3. Not Using Appropriate Data Types
When logging data, choosing the appropriate data type is essential. Logging complex objects as strings can lead to inefficiencies.
Why It's a Problem:
Converting objects to strings can be resource-intensive and might lead to loss of information or readability.
Best Practices:
- Use Serialization Libraries: Libraries like Jackson or Gson allow you to serialize objects efficiently without losing structure.
- Log Values, Not Objects: Only log the necessary values from objects rather than entire objects.
import com.fasterxml.jackson.databind.ObjectMapper;
public class ObjectLogger {
private static final Logger logger = LoggerFactory.getLogger(ObjectLogger.class);
private static final ObjectMapper objectMapper = new ObjectMapper();
public void logUserData(User user) {
try {
String userJson = objectMapper.writeValueAsString(user);
logger.info("User data: {}", userJson);
} catch (Exception e) {
logger.error("Failed to log user data", e);
}
}
}
4. Neglecting Error Handling in Logs
One of the critical purposes of logging is to assist in diagnosing errors. However, many developers overlook the importance of error handling in the log statements.
Why It's a Problem:
Neglecting to log exceptions or important error messages can lead to incomplete information when troubleshooting issues.
Best Practices:
- Audit Error Logs: Ensure you have adequate logging for all exceptions and errors occurring in your application.
- Log Stack Traces: Whenever possible, include stack traces in your logs to help pinpoint issues.
public void handleError() {
try {
// Some processing that might fail
} catch (Exception e) {
logger.error("An error occurred while processing the request", e);
}
}
5. Failing to Monitor and Rotate Logs
With Elasticsearch, you can ingest vast amounts of data, but failing to monitor and rotate logs can lead to performance bottlenecks.
Why It's a Problem:
As logs grow, they consume storage and can make Elasticsearch less efficient.
Best Practices:
- Implement Log Rotation: Use tools like Logrotate or Elasticsearch's native ILM (Index Lifecycle Management) to handle the log rotation process.
- Monitor Log Size: Set up alerts based on log size consumption to avoid performance degradation.
Closing Remarks
Logging requests to Elasticsearch is an invaluable practice that can vastly improve application maintainability and error resolution. By understanding these common pitfalls and adhering to best practices, developers can enhance their logging strategies. Always remember to log what matters, provide context, and ensure that your logs are efficient and manageable.
For more information about effective logging practices and tools for working with Elasticsearch, consider checking out:
- Elastic Documentation on Logging
- Understanding Log Levels in Java
By implementing better logging practices, you can gain clearer insights into your applications, resulting in quicker resolutions and higher application reliability.