Top 5 Common Pitfalls in Eclipse MicroProfile You Should Avoid
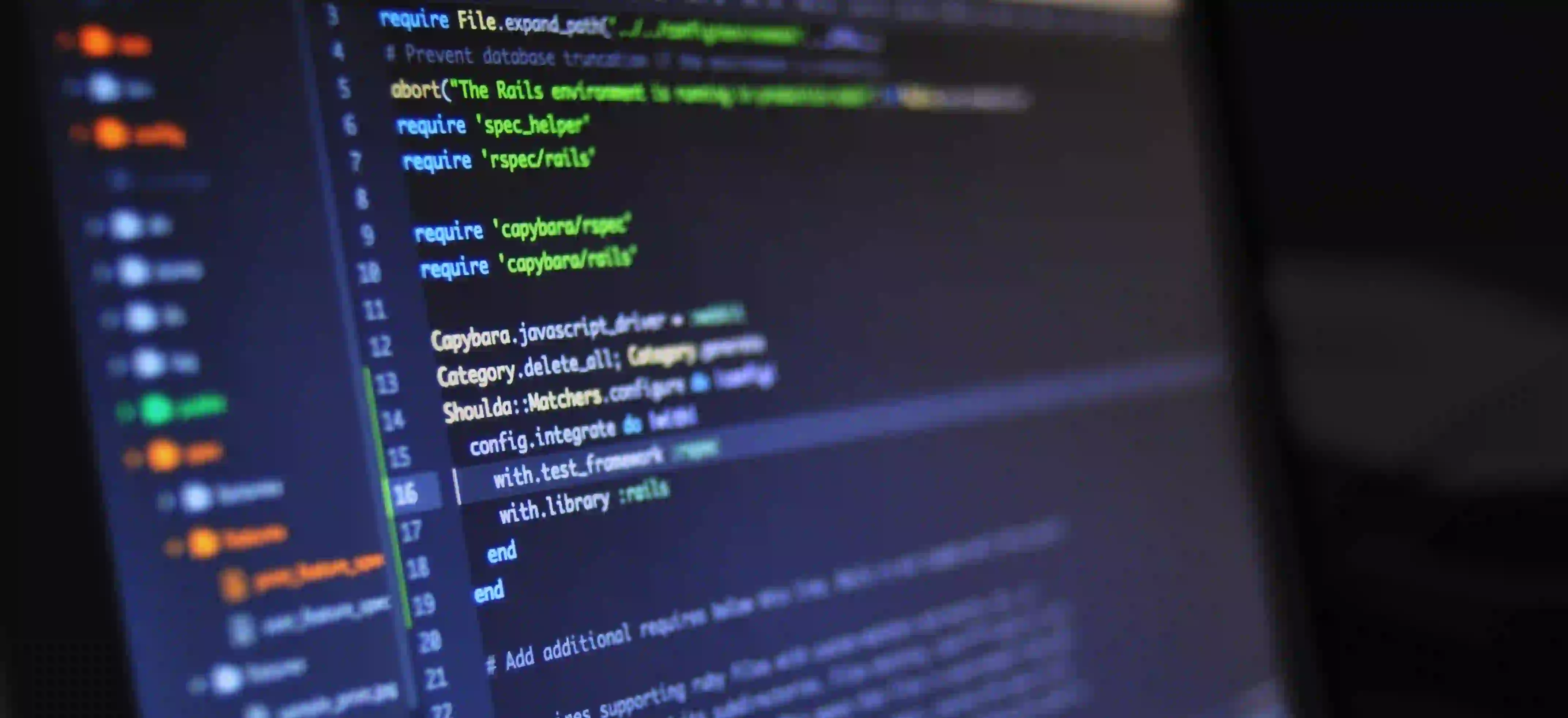
Top 5 Common Pitfalls in Eclipse MicroProfile You Should Avoid
Eclipse MicroProfile is a key player in the microservices landscape, designed to optimize enterprise Java for microservices architecture. While it offers numerous advantages, including reduced boilerplate code and enhanced productivity, developers often fall into common pitfalls during their implementations. This blog post will outline the top five pitfalls you should avoid to keep your MicroProfile projects on track.
Understanding Eclipse MicroProfile
Before we delve into the pitfalls, let's get a brief overview of what Eclipse MicroProfile is. It’s a set of specifications that enhance the Java EE platform to keep pace with the evolving needs of microservices. This suite includes important APIs such as:
- MicroProfile Config
- MicroProfile Fault Tolerance
- MicroProfile Metrics
- MicroProfile OpenAPI
These tools help developers build efficient, resilient, and maintainable microservices.
1. Neglecting Configuration Management
Why It Matters
Configuration management is critical in any microservices architecture. In MicroProfile, configuration details can be managed using resources like microprofile-config.properties
. Failing to appropriately utilize this feature can lead to hardcoded values, making the application inflexible and difficult to maintain.
Best Practices
When utilizing configuration management, remember to externalize your configuration settings. This ensures that your application can be easily updated without requiring code changes.
# src/main/resources/microprofile-config.properties
app.mode=production
db.url=jdbc:mysql://localhost:3306/mydb
Comment
Using properties files allows environments to dictate configurations, providing flexibility and promoting best practices like 12-factor apps.
Further Reading
Consider exploring the official MicroProfile Config documentation for deeper insights.
2. Underestimating Fault Tolerance
Why It Matters
Microservices systems are distributed, meaning that failures are a reality. MicroProfile offers the Fault Tolerance specification, preventing your application from crashing due to unforeseen errors. Ignoring this feature can compromise the stability of your application.
Best Practices
Implementing retries, circuit breakers, and timeouts can significantly enhance reliability. Here’s a simple example using the @Retry
annotation:
import org.eclipse.microprofile.faulttolerance.Retry;
@Retry(maxRetries = 3, delay = 1000)
public String fetchDataFromService() {
// Logic to fetch data from a remote service
}
Comment
Using retries allows your system to gracefully handle transient errors—and specifying a delay can mitigate cascading failures.
Further Reading
Check out the Fault Tolerance documentation for advanced techniques.
3. Ignoring Metrics and Monitoring
Why It Matters
Metrics provide visibility into the performance and health of your microservices. Not leveraging the MicroProfile Metrics can lead to blind spots in your application’s performance.
Best Practices
Always include metrics in your services. Use annotations to track methods and expose metrics through a dedicated endpoint.
import org.eclipse.microprofile.metrics.MetricRegistry;
import org.eclipse.microprofile.metrics.annotation.Metric;
@Metric(name = "requestCount")
private Meter requestCount;
public void processRequest() {
requestCount.mark();
// Processing logic
}
Comment
Using metrics helps in identifying bottlenecks and provides immediate feedback regarding system behavior. Incorporating Grafana or Prometheus can further enhance your monitoring setup.
Further Reading
Explore the MicroProfile Metrics documentation for additional insights.
4. Overlooking Proper Dependency Injection
Why It Matters
Dependency injection (DI) facilitates the management of component dependencies. Mismanaging DI can lead to tightly coupled codes, making your microservices less adaptable and harder to test.
Best Practices
Follow the principles of Inversion of Control (IoC). Use annotations like @Inject
properly to ensure components are loosely coupled:
import javax.inject.Inject;
public class MyService {
@Inject
private MyRepository repository;
public List<Data> getData() {
return repository.fetchData();
}
}
Comment
By ensuring that your application components can operate independently, you gain flexibility and the power to swap implementations easily.
Further Reading
For more details, check out the CDI documentation.
5. Mismanagement of API Versioning
Why It Matters
In a microservices architecture, API versioning becomes essential. Neglecting to implement proper version control can lead to unexpected breakages anytime updates are made.
Best Practices
Never use hardcoded API paths. Instead, add versioning into your endpoint URLs to ensure backward compatibility.
@Path("/api/v1/data")
public class DataResource {
@GET
public Response getData() {
// Retrieve data
}
}
Comment
Versioning allows you to support multiple clients smoothly, enabling gradual migrations to newer API versions without disrupting service.
Further Reading
Refer to the API Versioning strategies for different implementation methodologies.
Key Takeaways
While working with Eclipse MicroProfile brings significant advantages to microservice development, steering clear of these common pitfalls will help maintain a robust, scalable, and efficient architecture. By focusing on configuration management, fault tolerance, metrics, proper dependency injection, and API versioning, you set your projects up for success.
Should you wish to deepen your understanding of MicroProfile, the official MicroProfile website is an excellent resource for both beginners and experienced developers alike. Engage with the community, explore documentation, and most importantly, keep coding!
Happy coding!