Common Pitfalls When Using Java RMI: Avoid These Errors!
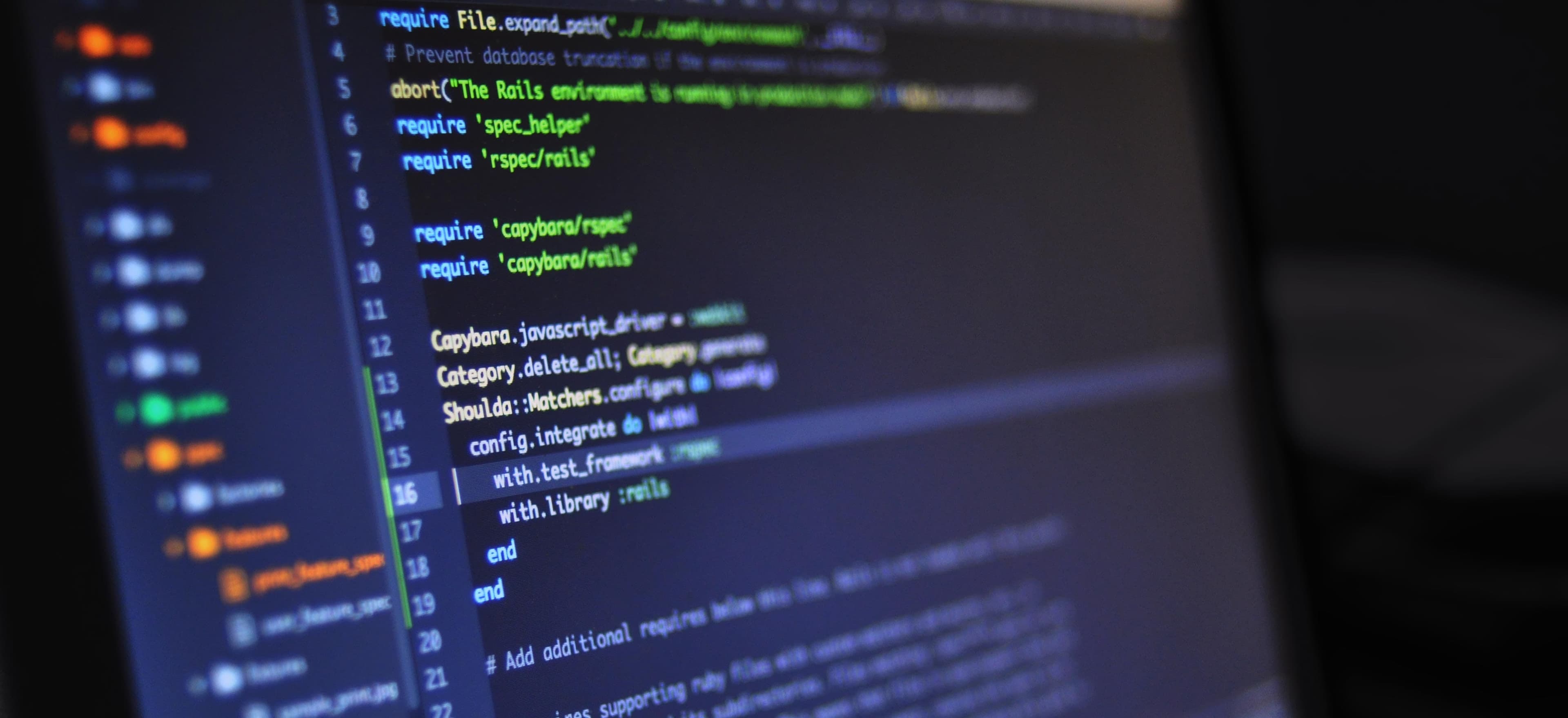
- Published on
Common Pitfalls When Using Java RMI: Avoid These Errors!
Java RMI (Remote Method Invocation) allows Java programs to invoke methods on objects located in different JVMs (Java Virtual Machines). While RMI simplifies distributed application development, it is not without its pitfalls. This blog post explores the common errors developers encounter when using Java RMI, offering insights and best practices to ensure smoother implementations.
Understanding Java RMI
Before we delve into the common pitfalls, let’s quickly review how Java RMI works. RMI allows you to create distributed applications, where the method execution occurs in a remote machine. This technology enables seamless communication between applications over a network.
To illustrate, here’s a simple RMI setup:
-
Create a Remote Interface: This interface defines the methods that can be invoked remotely.
import java.rmi.Remote; import java.rmi.RemoteException; public interface HelloService extends Remote { String sayHello() throws RemoteException; }
-
Implement the Remote Interface: This implementation will contain the business logic.
import java.rmi.server.UnicastRemoteObject; public class HelloServiceImpl extends UnicastRemoteObject implements HelloService { protected HelloServiceImpl() throws RemoteException { super(); } @Override public String sayHello() { return "Hello, World!"; } }
-
Create the RMI Registry: This registry helps clients discover remote objects.
import java.rmi.registry.LocateRegistry; import java.rmi.registry.Registry; public class Server { public static void main(String[] args) { try { HelloService service = new HelloServiceImpl(); Registry registry = LocateRegistry.createRegistry(1099); registry.bind("HelloService", service); System.out.println("Service bound and ready."); } catch (Exception e) { e.printStackTrace(); } } }
-
Client Access: Clients can access the remote service.
import java.rmi.registry.LocateRegistry; import java.rmi.registry.Registry; public class Client { public static void main(String[] args) { try { Registry registry = LocateRegistry.getRegistry("localhost", 1099); HelloService service = (HelloService) registry.lookup("HelloService"); System.out.println(service.sayHello()); } catch (Exception e) { e.printStackTrace(); } } }
Now that we have a basic understanding of RMI, let’s explore the potential pitfalls.
1. Not Handling Remote Exceptions Properly
The Pitfall
Since RMI involves network communication, it’s crucial to handle RemoteException
properly. Failing to do so can lead to unexpected behavior or application crashes.
Solution
Always surround your remote invocations with a try-catch block to deal with exceptions gracefully. This not only enhances robustness but also improves user experience.
try {
Registry registry = LocateRegistry.getRegistry("localhost", 1099);
HelloService service = (HelloService) registry.lookup("HelloService");
System.out.println(service.sayHello());
} catch (RemoteException e) {
System.err.println("Remote connection failed: " + e.getMessage());
} catch (NotBoundException e) {
System.err.println("Service not found: " + e.getMessage());
}
2. Ignoring SecurityManager
Settings
The Pitfall
Java RMI requires a security manager to enforce access control. Many developers overlook this requirement, resulting in security exceptions.
Solution
Set a security manager before you bind or lookup any remote services. This ensures that your application adheres to security policies.
if (System.getSecurityManager() == null) {
System.setSecurityManager(new SecurityManager());
}
3. Using Incompatible Serializable Objects
The Pitfall
When passing objects as parameters or return values in RMI methods, developers often assume that all Java objects are serializable. However, objects that do not implement Serializable
will lead to a java.io.NotSerializableException
.
Solution
Always ensure that objects you wish to transfer across the network implement the Serializable
interface. Here’s an example:
import java.io.Serializable;
public class User implements Serializable {
private String name;
private int id;
public User(String name, int id) {
this.name = name;
this.id = id;
}
// Getters and setters
}
4. Neglecting Versioning Issues
The Pitfall
When you modify a serializable class, the serialVersionUID
should be updated. If you fail to do so, older clients may encounter deserialization issues.
Solution
Always declare a serialVersionUID
in your serializable classes. This will help manage versions, ensuring compatibility.
private static final long serialVersionUID = 1L;
5. Poor Exception Logging
The Pitfall
Many developers underestimate the importance of logging. When exceptions occur during remote calls, neglecting adequate logging can hinder troubleshooting efforts.
Solution
Incorporate robust logging practices using libraries such as SLF4J or Log4j. This allows for better monitoring and debugging.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class Client {
private static final Logger logger = LoggerFactory.getLogger(Client.class);
public static void main(String[] args) {
try {
// Remote invocation logic
} catch (Exception e) {
logger.error("An error occurred while calling remote method", e);
}
}
}
6. Misconfigured RMI Registry
The Pitfall
Another common issue arises from misconfiguration of the RMI registry. This can lead to NotBoundException
or connection failures.
Solution
Ensure your RMI registry is configured correctly. Use the right port and ensure it is running when invoking remote methods. For instance, run your RMI registry in a separate terminal before executing your RMI server.
rmiregistry 1099
7. Firewall and Network Issues
The Pitfall
Network settings can sometimes block RMI communication. Firewall settings on the host machines can prevent RMI from functioning properly.
Solution
Check firewall settings and ensure that the necessary ports are open. Further, verify connectivity between client and server using commands like ping
.
Closing Remarks
Java RMI is a powerful tool for building distributed applications, but it comes with its fair share of challenges. By being aware of these common pitfalls, you can navigate around potential errors, making your development process smoother and more efficient.
For further reading about Java RMI, you may check the official documentation. By implementing the best practices discussed in this post, you will not only enhance the performance of your application but also foster a better user experience.
Additional Resources
By avoiding these common pitfalls, you can create robust RMI applications that leverage the power of distributed computing. Happy coding!
Checkout our other articles