Avoiding Common Mistakes in Spring JPA Repository with In-Memory Database
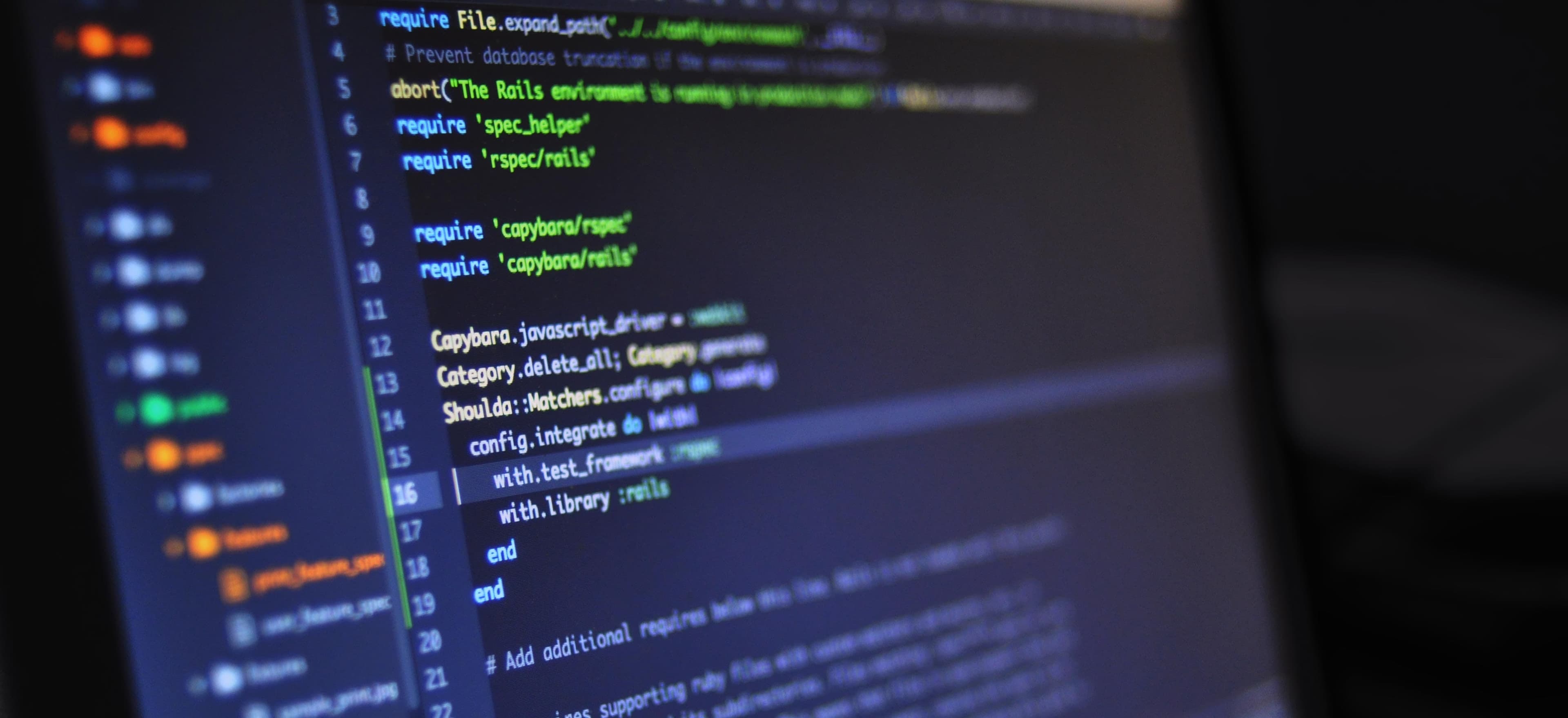
- Published on
Avoiding Common Mistakes in Spring JPA Repository with In-Memory Database
Spring Data JPA has revolutionized the way Java developers interact with relational databases. By providing a simple abstraction for working with databases, it enables us to focus more on building our application rather than writing boilerplate database code. However, when using an in-memory database for testing, there are common pitfalls worth avoiding. This blog post aims to highlight these common mistakes and how you can sidestep them effectively.
Understanding Spring Data JPA and In-Memory Databases
Before delving into the mistakes, it's crucial to comprehend what Spring Data JPA and in-memory databases like H2 offer. Spring Data JPA simplifies database interactions and supports the development of Java applications by providing repository interfaces. An in-memory database, such as H2, can be instantiated and used in your tests, allowing for rapid data operations without the overhead of configuring a full database system.
In-memory databases are advantageous because:
- They offer fast read/write operations.
- No additional installation is required; they run in the Java Virtual Machine (JVM) memory.
However, the use of in-memory databases comes with its own challenges. Let's dive into those pitfalls.
Common Mistakes in Spring JPA Repository
1. Not Keeping Synchronous Entity State
One common mistake is not understanding how the entity state works in a Spring context. When you use an in-memory database, the entities may not be synchronized as expected.
Solution: You should always use @Transactional
in your service layers. This will ensure that the session is properly flushed and that your entities' states remain consistent throughout the transaction.
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
@Transactional
public User createUser(User user) {
// The user entity is saved in a transactional context
return userRepository.save(user);
}
}
2. Ignoring Spring's Repository Configuration
Often, developers overlook the necessity of annotating repository interfaces with @Repository
. While Spring can still pick them up through component scanning, an explicit declaration provides clarity and helps manage exceptions effectively.
Solution: Always annotate your repositories clearly.
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
}
3. Testing with Incorrect Data Flush
Another common mistake is assuming data is always immediately available after saving. With in-memory databases, data may only be flushed as part of the transaction.
Solution: Ensure you understand the flush behavior in your tests. You can explicitly call flush()
on the repository.
@Autowired
private UserRepository userRepository;
@Test
public void testUserCreation() {
User user = new User("John Doe");
userRepository.save(user);
userRepository.flush(); // Ensures change is persisted immediately
Optional<User> retrievedUser = userRepository.findById(user.getId());
assertTrue(retrievedUser.isPresent());
}
4. Not Using Proper Keys
Using in-memory databases often leads to managing primary keys incorrectly. If you do not specify a generation strategy, it could lead to issues where your application fails to find records due to non-unique identifiers.
Solution: Always define your ID generation strategy appropriately.
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY) // Use identity for auto-incrementing IDs
private Long id;
private String name;
// Constructors, getters, and setters
}
5. Failing to Setup Schema Properly
Utilizing the H2 database, developers sometimes embark on testing without ensuring that the table schemas align with the application entities. If schema definitions are inconsistent, it can lead to DataIntegrityViolationException
.
Solution: You should always verify that the schema matches the expected entity state.
Add H2 to your application.properties
:
spring.h2.console.enabled=true
spring.datasource.url=jdbc:h2:mem:testdb
spring.jpa.hibernate.ddl-auto=create-drop // Automatically drops and creates every run
6. Using Lazy Loading Incorrectly
Lazy loading is a fantastic feature to optimize performance, but it can lead to issues in testing due to the unpredictable transaction boundaries in a testing environment.
Solution: Be cautious about how you use lazy loading in tests. If you're accessing lazy-loaded fields outside of the transactional context, you'll encounter LazyInitializationException
.
@Service
public class OrderService {
@Autowired
private OrderRepository orderRepository;
@Transactional(readOnly = true)
public List<Order> getAllOrders() {
return orderRepository.findAll(); // Should be in a transactional context
}
}
7. Overlooking the Performance Implications
When using an in-memory database for testing, developers do not always account for the performance differences from a production environment. A query that performs well in-memory may perform poorly against a production database.
Solution: Conduct performance testing in an environment that closely resembles production.
Final Considerations
By avoiding these common mistakes, you can effectively harness the power of Spring Data JPA with an in-memory database like H2, yielding a more efficient and maintainable Java application. Always remember to thoroughly test your repository configurations, adequately manage entity states, and understand the implications of lazy loading.
For further reading and to deepen your understanding of Spring Data JPA, check out these resources:
Embrace the tools available to you, and happy coding!