Mastering Common Pitfalls in Java Arrays Usage
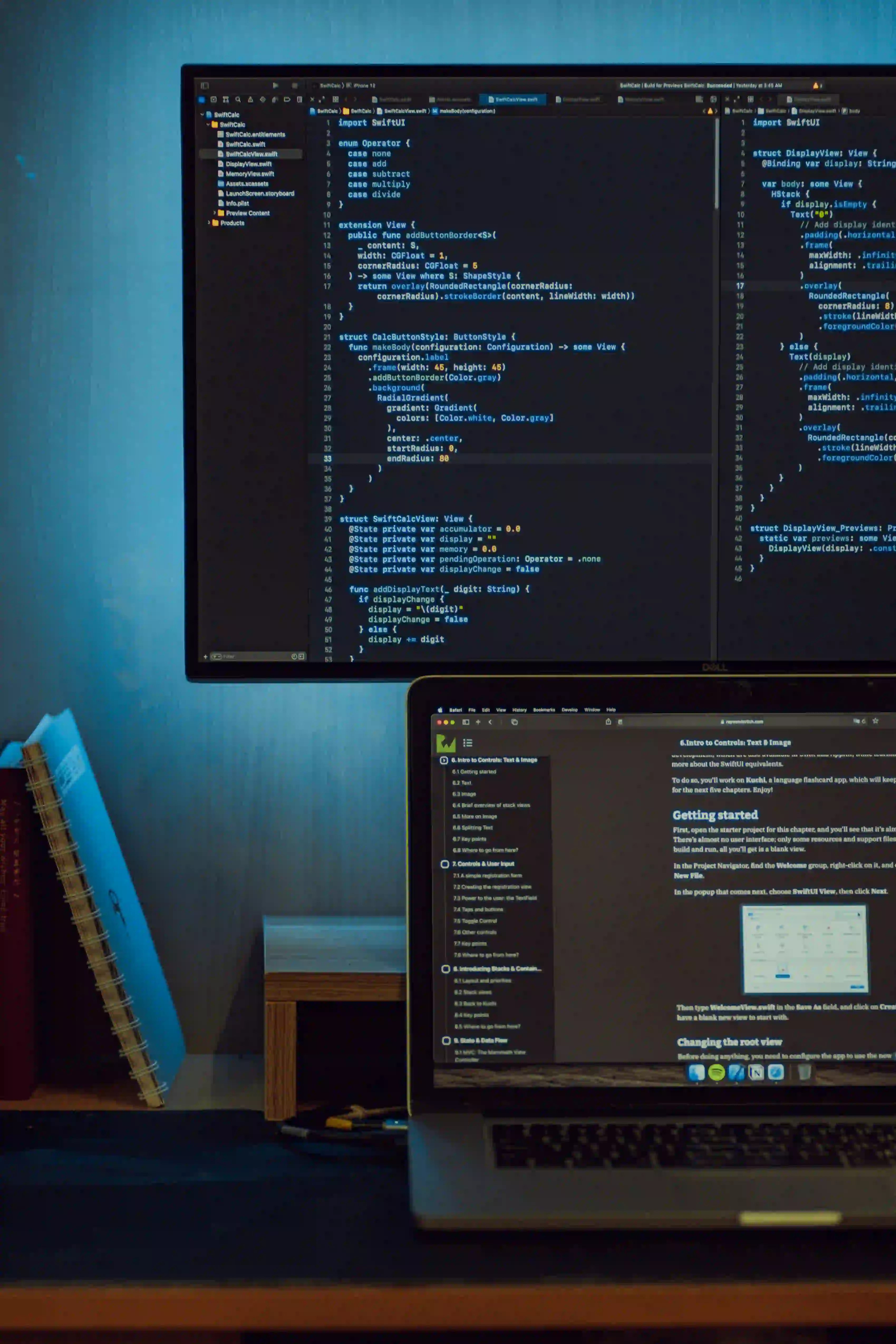
Mastering Common Pitfalls in Java Arrays Usage
Java arrays are powerful data structures that allow developers to store multiple values in a single variable. However, they come with their own set of challenges. Understanding and mastering these common pitfalls can significantly improve the quality of your code and boost performance. In this blog post, we will explore the most prevalent pitfalls while using arrays in Java, provide examples, and discuss how to avoid these mistakes.
Table of Contents
- Understanding Arrays in Java
- Common Pitfalls in Java Arrays
- Off-by-One Errors
- ArrayIndexOutOfBoundsException
- Immutable Size
- Mixed Types
- Not Using Enhanced For-Loop
- Best Practices for Using Arrays
- Conclusion
Understanding Arrays in Java
In Java, an array is a container object that holds a fixed number of values of a single type. The length of an array is decided when it is created, and it cannot be changed later. Here's a simple example demonstrating array declaration, initialization, and usage:
public class ArrayExample {
public static void main(String[] args) {
// Declaring and initializing an array of integers
int[] numbers = {1, 2, 3, 4, 5};
// Accessing an element
System.out.println("First element: " + numbers[0]);
// Iterating over the array
for (int i = 0; i < numbers.length; i++) {
System.out.println("Element at index " + i + ": " + numbers[i]);
}
}
}
In the example, a simple integer array is created and printed. Pay attention to the fact that arrays in Java are zero-indexed, meaning the first element is accessed using index 0.
Common Pitfalls in Java Arrays
Off-by-One Errors
One of the most common errors when working with arrays is the off-by-one error. This occurs when a programmer miscalculates the index of an array.
Example:
public class OffByOneExample {
public static void main(String[] args) {
int[] myArray = {1, 2, 3};
// Incorrectly accessing the fourth element
System.out.println(myArray[3]); // This will throw ArrayIndexOutOfBoundsException
}
}
Why? In this scenario, the code attempts to access the fourth element (index 3) of an array that only has three elements (indexes 0, 1, 2). Always remember to iterate from 0 to array.length - 1.
ArrayIndexOutOfBoundsException
The ArrayIndexOutOfBoundsException
is a common runtime exception that occurs when an attempt to access an element outside the bounds of the array is made.
Example:
public class ScopeExample {
public static void main(String[] args) {
String[] fruits = {"Apple", "Banana", "Cherry"};
// Accessing an index out of bounds
for (int i = 0; i <= fruits.length; i++) {
System.out.println(fruits[i]); // This will cause an exception on the last iteration
}
}
}
Why?
The loop condition should be i < fruits.length
instead of i <= fruits.length
. This prevents the loop from attempting to access an index that does not exist.
Immutable Size
Another pitfall is the immutable size of arrays. Once you declare the size of an array, it cannot be modified. If you need flexibility, you might want to use collection classes like ArrayList
.
Example:
public class SizeExample {
public static void main(String[] args) {
int[] numbers = new int[5];
// Attempting to change the size
numbers = new int[10]; // This is legal, but not the same as resizing the existing array
}
}
Why? In the above code, when you try to create a new array, you lose the reference to the old one. This is a common coding error that can lead to data loss if references are not managed properly.
Mixed Types
Java arrays can only hold elements of a single type. If you need to store multiple types, consider using Object arrays, but that can undermine the type safety of your application.
Example:
public class MixedTypesExample {
public static void main(String[] args) {
Object[] mixedArray = {1, "Hello", 3.14}; // This is valid but not recommended
for (Object obj : mixedArray) {
System.out.println(obj);
}
}
}
Why? While Java allows Object arrays, it is best to adhere to single-type arrays for better performance and maintenance. Using generics can help you define a type-safe collection.
Not Using Enhanced For-Loop
Many beginners may overlook the enhanced for-loop (or "for-each" loop) when iterating through arrays. This can lead to unnecessarily verbose code.
Example:
public class EnhancedForLoopExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
// Using a standard for-loop
for (int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]);
}
// Using enhanced for-loop
for (int number : numbers) {
System.out.println(number); // Cleaner and more readable
}
}
}
Why? The enhanced for-loop provides a more succinct and readable way to iterate through arrays while automatically handling the indexing, which can reduce the potential for errors.
Best Practices for Using Arrays
To avoid the pitfalls discussed above, consider implementing the following best practices:
-
Always check bounds: When accessing any element, ensure you are within the range of the array.
-
Use enhanced for-loops: They simplify the code for iterating over arrays.
-
Consider ArrayLists for dynamic needs: When you require flexibility, switch to
ArrayList
instead of fixed-size arrays. -
Type-Safe Collections: Utilize Java generics to ensure type safety when working with collections.
-
Documentation and Comments: Always document your code, especially in places where index manipulation occurs, to provide clarity.
Final Thoughts
Mastering array usage in Java involves understanding both its capabilities and its limitations. By being aware of common pitfalls such as off-by-one errors, ArrayIndexOutOfBoundsException
, and the immutable size characteristic of arrays, you can write cleaner, more effective Java code. Implement best practices to create robust and maintainable applications. As you refine your understanding of these concepts, you will find yourself becoming a more proficient Java developer.
For more information on Java arrays and their intricacies, consider checking out the Java Documentation for additional resources.