Mastering Java Control Flow: Common Pitfalls and Solutions
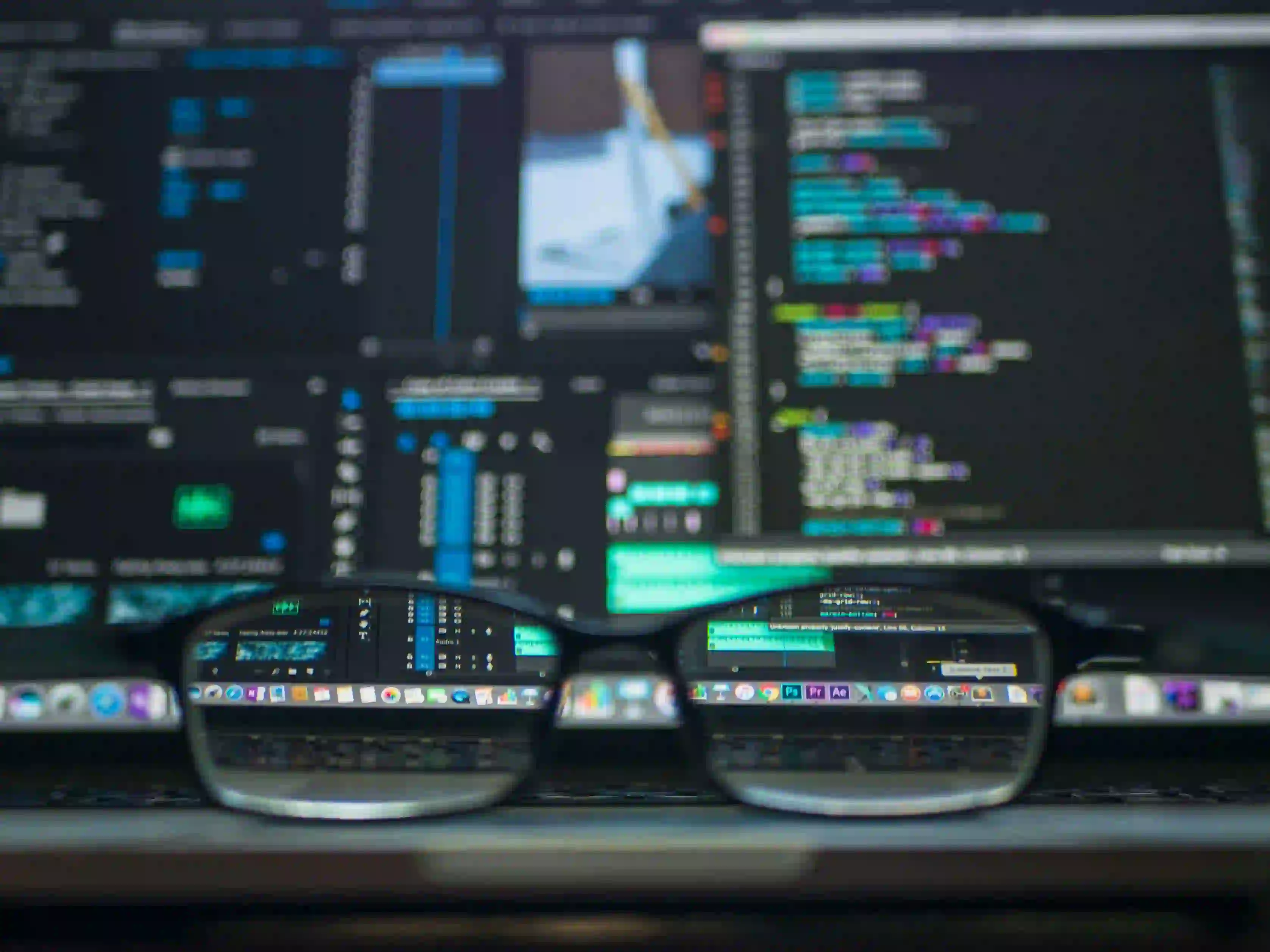
Mastering Java Control Flow: Common Pitfalls and Solutions
Java control flow is foundational in determining how your code operates under different conditions. Whether you're branching with if
statements, iterating with for
loops, or managing operations with switch
cases, the flow of your program can significantly impact functionality and performance. In this blog post, we'll discuss common pitfalls you might encounter with Java control flow and offer practical solutions to help you master this vital programming aspect.
Understanding Control Flow in Java
Control flow refers to the order in which individual statements, instructions, or function calls are executed or evaluated in a program. Java, being a versatile programming language, offers several control flow constructs:
-
Sequential Execution: The default mode where statements are executed in the order they are written.
-
Selection Statements: These include
if
,else if
,else
, andswitch
statements that allow branching based on conditions. -
Repetition Statements: Loops such as
for
,while
, anddo-while
enable code blocks to execute repeatedly based on a condition.
Common Pitfalls in Control Flow
Even the most seasoned developers can encounter pitfalls in control flow. Let's dive into some common issues and how to resolve them.
1. The "Off-by-One" Error
One of the most common mistakes in looping constructs is the "off-by-one" error. This typically occurs when loops iterate one time too many or one time too few.
public class OffByOneExample {
public static void main(String[] args) {
for (int i = 0; i <= 5; i++) { // Off by one mistake
System.out.println("Iteration: " + i);
}
}
}
Why It Matters: In this example, the loop runs six times (from 0 to 5). If your intention was to loop five times, you should change the loop condition to i < 5
.
for (int i = 0; i < 5; i++) {
System.out.println("Iteration: " + i);
}
Solution
Always double-check loop boundaries. Visualize your iterations to ensure you are iterating the expected number of times.
2. Infinite Loops
Infinite loops can often lead to program crashes or hanging applications. They usually occur due to mistaken conditions or incorrect increments.
public class InfiniteLoopExample {
public static void main(String[] args) {
int count = 0;
while (count < 5) {
// Forgetting to increment count
System.out.println("Count: " + count);
}
}
}
Why It Matters: This code will never break out of the loop since the count
variable never changes.
Solution
Always include a way to exit your loops. Ensure that your loop condition can eventually evaluate to false.
3. Misusing the Switch Statement
The switch
statement is a powerful tool for handling multiple conditions. However, developers sometimes forget to include the break
statement, leading to fall-through behavior.
public class SwitchExample {
public static void main(String[] args) {
int number = 2;
switch (number) {
case 1:
System.out.println("One");
case 2:
System.out.println("Two");
case 3:
System.out.println("Three");
default:
System.out.println("Not One, Two, or Three");
}
}
}
Why It Matters: This code will print "Two," "Three," and "Not One, Two, or Three" because there are no break
statements.
Solution
Always use break
statements unless you specifically intend to leverage fall-through for case handling.
public class ProperSwitchExample {
public static void main(String[] args) {
int number = 2;
switch (number) {
case 1:
System.out.println("One");
break;
case 2:
System.out.println("Two");
break;
case 3:
System.out.println("Three");
break;
default:
System.out.println("Not One, Two, or Three");
break;
}
}
}
4. Logical Errors in Conditional Expressions
Sometimes the logic within your conditional statements can lead to mistakes. Assuming the condition evaluates correctly can lead to unexpected behavior.
public class LogicalErrorExample {
public static void main(String[] args) {
int score = 85;
if (score > 90) {
System.out.println("Grade: A");
} else if (score >= 80) {
System.out.println("Grade: B");
} else if (score >= 70) {
System.out.println("Grade: C");
}
}
}
Why It Matters: If you forgot to include an else case for scores below 70, this can leave some users confused about their grades.
Solution
Always consider the complete range of possible values in your conditions. This ensures that all cases are handled appropriately.
public class CompleteLogicExample {
public static void main(String[] args) {
int score = 85;
if (score > 90) {
System.out.println("Grade: A");
} else if (score >= 80) {
System.out.println("Grade: B");
} else if (score >= 70) {
System.out.println("Grade: C");
} else {
System.out.println("Grade: D or lower");
}
}
}
Final Considerations
Java control flow is pivotal for crafting well-functioning applications. By recognizing common pitfalls such as off-by-one errors, infinite loops, improper use of switch statements, or logical mistakes in conditional expressions, you can streamline your coding process and enhance your skills.
To further hone your Java skills, feel free to check out the following resources for additional learning:
- Java Control Flow - Oracle Documentation
- Effective Java - A Must-Read Book for Java Developers
Control flow in Java may appear simple at first glance, but being mindful of potential pitfalls can save you from headaches in the long run. Start implementing these best practices today, and watch your programming skills soar to new heights!