Mastering Java: Common Mistakes with Classes and Objects
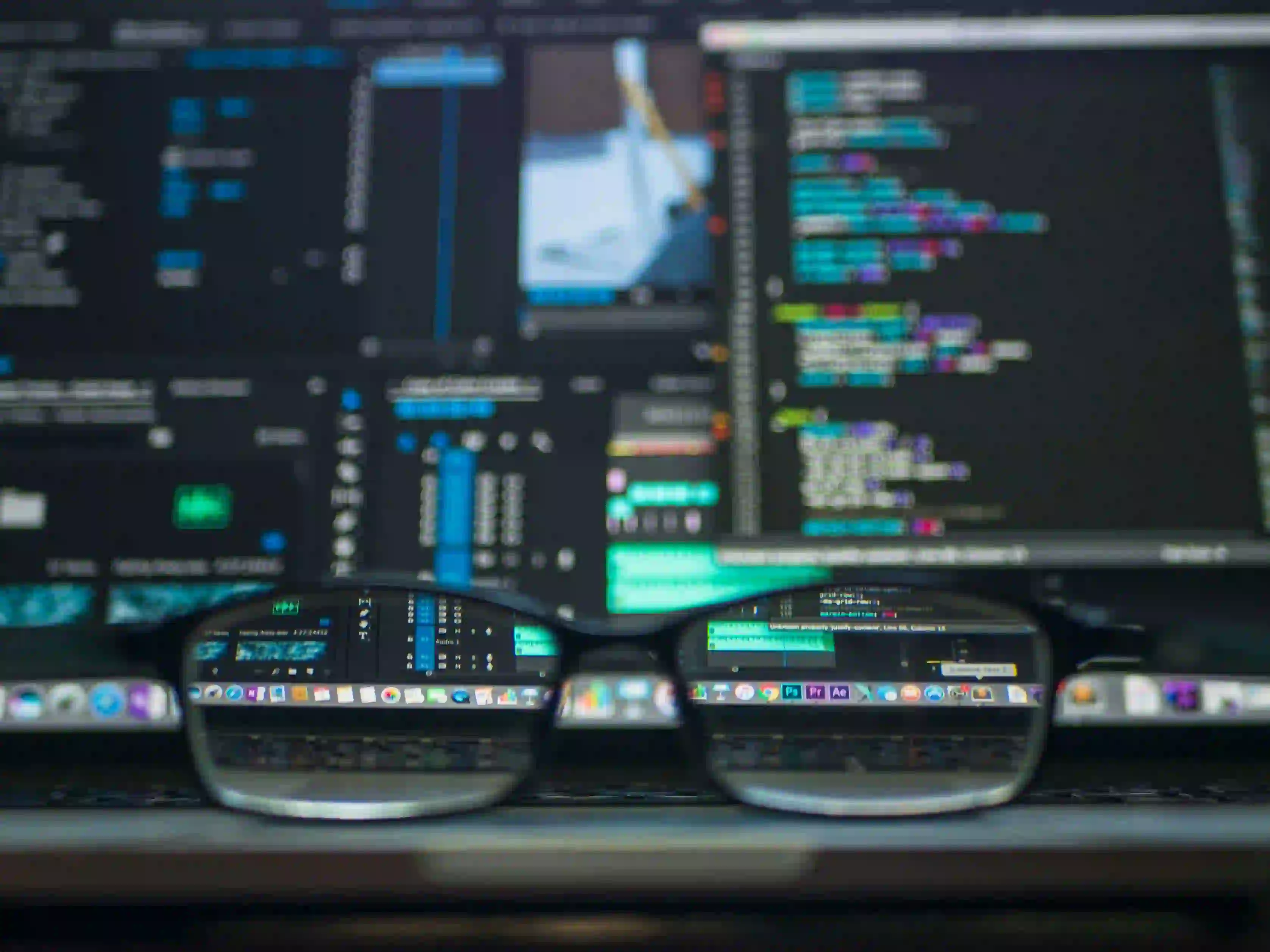
Mastering Java: Common Mistakes with Classes and Objects
Java, a powerful object-oriented programming language, is widely used for various applications, from mobile to enterprise solutions. However, like any tool, it can be misused. In this blog post, we're going to explore some common mistakes developers make with classes and objects in Java. By identifying these issues, you'll be better equipped to write cleaner, more efficient code.
Understanding Classes and Objects in Java
Before we dive into the errors, it's essential to understand the fundamental concepts of classes and objects in Java.
- Class: A blueprint for creating objects, classes define the properties (attributes) and behaviors (methods) that the objects created from the class can have.
- Object: An instance of a class. It represents a specific implementation of the defined blueprint.
Here's a simple example:
class Car {
String model;
int year;
void drive() {
System.out.println(model + " is driving");
}
}
// Creating an object of the Car class
Car myCar = new Car();
myCar.model = "Toyota";
myCar.year = 2022;
myCar.drive();
In this example, Car
is a class that has attributes model
and year
, and a method drive
. The object myCar
is an instance of the Car
class, demonstrating the basic use of classes and objects.
Common Mistakes with Classes and Objects
1. Not Using Encapsulation
Mistake: Developers often expose class fields directly, violating the principle of encapsulation.
Why It Matters: Encapsulation helps maintain the integrity of an object's state. It prevents external classes from changing the object's internal representation unpredictably.
Correct Approach:
class Car {
private String model;
private int year;
// Getter for model
public String getModel() {
return model;
}
// Setter for model
public void setModel(String model) {
this.model = model;
}
// Other methods...
}
Using getters and setters allows controlled access to the fields, ensuring consistency and validation if needed.
2. Forgetting to Use this
Keyword
Mistake: New developers may forget to use the this
keyword, leading to confusion between class fields and parameters.
Why It Matters: Without the this
keyword, the compiler might incorrectly reference the parameters instead of instance variables, which can lead to unintended behavior.
Correct Approach:
class Car {
private String model;
public Car(String model) {
this.model = model; // Using this to refer to the instance variable
}
}
In this instance, this.model
clearly indicates that we are referencing the instance variable rather than the method parameter.
3. Misunderstanding the final
Keyword
Mistake: Misapplying the final
keyword for classes, methods, or variables can lead to confusion.
Why It Matters: Understanding the implications of final
is crucial to avoid unwanted modifications later in the code.
Correct Usage:
- A final class cannot be subclassed, while a final method cannot be overridden.
- A final variable cannot be reassigned after initialization.
Example:
final class ImmutableCar {
private final String model;
public ImmutableCar(String model) {
this.model = model;
}
// Cannot modify model after set during construction
}
4. Not Overriding toString
, equals
, and hashCode
Mistake: Failing to override toString()
, equals()
, and hashCode()
may lead to difficult debugging experiences and unexpected behavior when using collections like HashMap
.
Why It Matters: Providing meaningful string representations and equality checks is critical when managing collections or logging.
Correct Approach:
class Car {
private String model;
private int year;
@Override
public String toString() {
return "Car{" +
"model='" + model + '\'' +
", year=" + year +
'}';
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (!(obj instanceof Car)) return false;
Car car = (Car) obj;
return year == car.year && model.equals(car.model);
}
@Override
public int hashCode() {
return Objects.hash(model, year);
}
}
By overriding these methods, you'll ensure proper object behavior in collections and logging for debugging.
5. Ignoring Static Context
Mistake: Confusing static and instance contexts may lead to unnecessary memory use and unexpected results.
Why It Matters: Understanding static context helps in managing memory and sharing data among instances.
Correct Approach:
class Car {
private String model;
private static int numberOfCars = 0; // shared across instances
public Car(String model) {
this.model = model;
numberOfCars++;
}
public static int getNumberOfCars() {
return numberOfCars; // Accessing static variable
}
}
Car car1 = new Car("Toyota");
Car car2 = new Car("Honda");
System.out.println(Car.getNumberOfCars()); // Output: 2
6. Overusing Inheritance
Mistake: Developers may excessively rely on inheritance, leading to complex and fragile code.
Why It Matters: Favoring composition over inheritance allows for cleaner, more modular designs.
Correct Approach: Consider using interfaces or composition.
interface Drivable {
void drive();
}
class Car implements Drivable {
private String model;
public void drive() {
System.out.println(model + " is driving.");
}
}
By using interfaces, you allow for more flexible designs that can easily adapt to new components.
My Closing Thoughts on the Matter
Mastering classes and objects in Java requires a firm understanding of fundamental principles and patterns. By avoiding common mistakes like neglecting encapsulation, misusing the final
keyword, or overusing inheritance, you'll become a more effective Java developer.
For more in-depth study, check out the Java Documentation and enhance your understanding of classes and objects. Also, consider reading up on Best Practices in Java for a comprehensive approach to Java programming.
By being aware of these common pitfalls and employing best practices, you'll not only improve your code quality but also contribute to stronger, more maintainable applications. Happy coding!