Mastering Inheritance: Common Mistakes in Java OOP
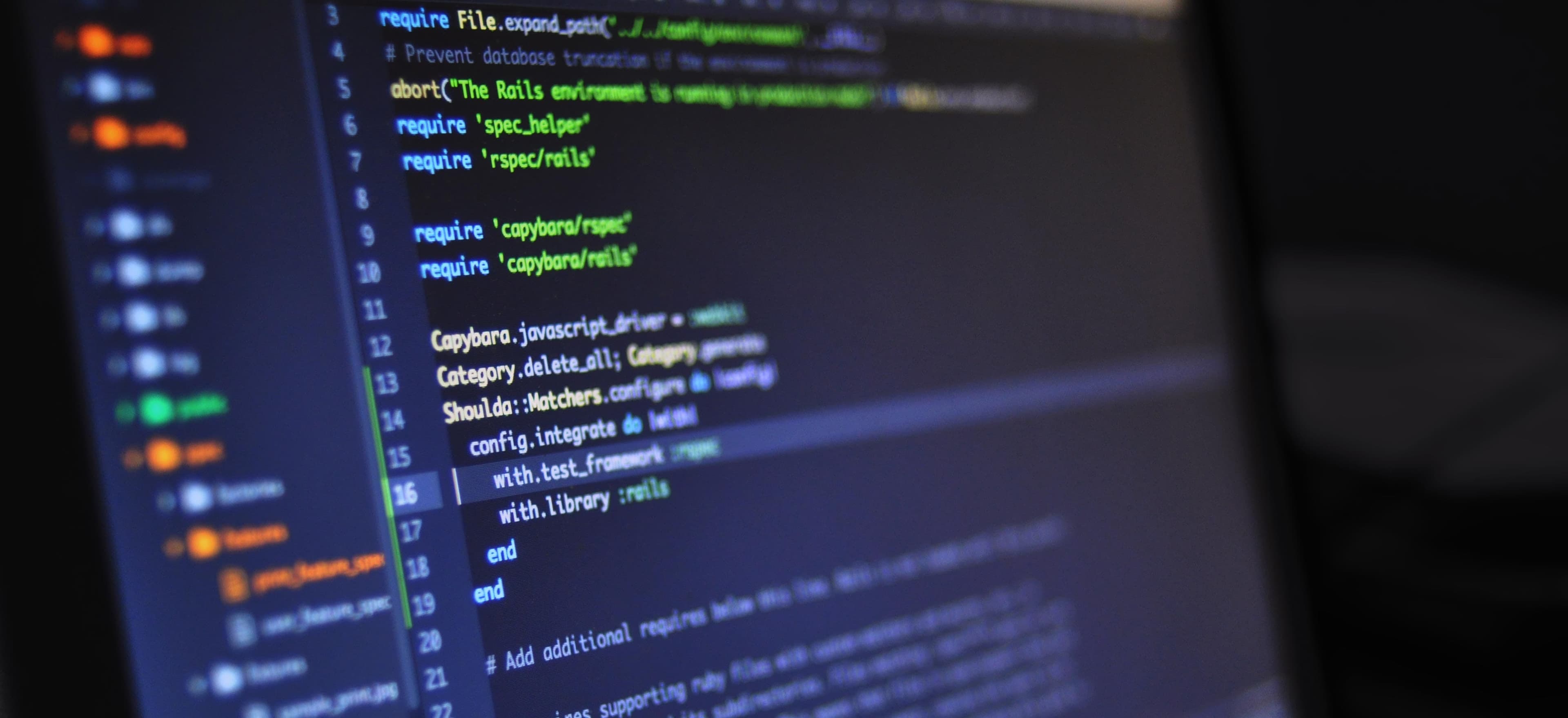
- Published on
Mastering Inheritance: Common Mistakes in Java OOP
Inheritance is one of the cornerstones of Object-Oriented Programming (OOP) in Java. It promotes the reuse of code, allows for the creation of hierarchical classifications, and provides a clear model for structuring applications. However, many novice and even experienced developers can stumble into common pitfalls when working with inheritance. In this blog post, we will explore several of these mistakes, learn how to avoid them, and provide best practices to master inheritance in Java.
Understanding Inheritance in Java
Before diving into common mistakes, let’s briefly recap what inheritance is. In Java, a class can inherit fields and methods from another class. The class that is inherited from is known as the parent class (or superclass), while the class that inherits is called the child class (or subclass).
class Animal {
void eat() {
System.out.println("This animal eats food.");
}
}
class Dog extends Animal {
void bark() {
System.out.println("Woof! Woof!");
}
}
In the above code, Dog
inherits the eat
method from Animal
. The advantage? A Dog
object can utilize both bark
and eat
, showcasing the reusability that inheritance provides.
Common Mistakes in Inheritance
1. Misuse of Inheritance vs. Composition
One of the most foundational mistakes is using inheritance when composition is more appropriate. The is-a
relationship of inheritance should only be implemented when it makes logical sense. For instance, a Car
is not a type of Engine
, even though it may contain an engine.
class Engine {
void start() {
System.out.println("Engine starting...");
}
}
class Car {
private Engine engine;
Car(Engine engine) {
this.engine = engine;
}
void drive() {
engine.start();
System.out.println("Car is driving.");
}
}
In this case, Car
is composed of an Engine
, illustrating the has-a
relationship, which is often more appropriate than forcing an is-a
relationship through inheritance.
2. Overriding Methods Incorrectly
When overriding a method, the overriding method should match the parent method's signature. Failing to do so can lead to unexpected behavior.
class Parent {
void show() {
System.out.println("Parent show method");
}
}
class Child extends Parent {
@Override
void show(String message) { // Mistake: signature does not match
System.out.println(message);
}
}
The Child
class incorrectly overrides the show
method by changing its signature. To properly override, ensure the method signature remains consistent:
@Override
void show() {
System.out.println("Child show method");
}
3. Ignoring the Use of super
When you override a method in the subclass but need access to the parent class's version of that method, the keyword super
becomes essential.
class Animal {
void move() {
System.out.println("Animal moves");
}
}
class Bird extends Animal {
@Override
void move() {
super.move(); // Calls the parent move method
System.out.println("Bird flies");
}
}
Ignoring super
means you may lose critical behavior defined in the parent class, possibly leading to a breakdown in functionality.
4. Creating Deep Inheritance Trees
While a deep inheritance tree may seem appealing for organization, it can lead to complex architectures that are difficult to understand and maintain. The more levels there are, the harder it becomes to track method calls and properties.
class A {}
class B extends A {}
class C extends B {}
class D extends C {}
// and so on...
Instead, aim for a flatter structure using interfaces or a mix of composition and inheritance to maintain readability and reusability.
5. Using Final Classes Incorrectly
Declaring classes as final
restricts inheritance. While this might be useful for certain classes, it might also hinder the flexibility of your code. Always weigh the pros and cons of marking classes as final.
final class ImmutableClass {
// no subclasses can extend this
}
Using final classes can ensure immutability and thread safety but be cautious of limiting extensibility in scenarios where inheritance may offer benefits.
6. Relying Heavily on Getters and Setters
Using public getters and setters can expose your classes to unwanted modification, resulting in fragile code. Instead, strive to encapsulate the data and provide methods that encapsulate behavior rather than direct access to fields.
class User {
private String name;
public void setName(String name) {
// Invalidate 'name' if it does not meet certain conditions
this.name = name;
}
}
7. Broken Liskov Substitution Principle
One major principle in OOP is the Liskov Substitution Principle (LSP). This principle states that objects of a superclass should be replaceable with objects of a subclass without affecting the correct functioning of the program.
class Rectangle {
void setWidth(int width) { ... }
void setHeight(int height) { ... }
}
class Square extends Rectangle {
// Violates LSP - cannot guarantee behaviors of Rectangle
@Override
void setWidth(int width) {
super.setWidth(width);
super.setHeight(width); // Consequentially changing height
}
}
In this case, using Rectangle
as a superclass creates problems for Square
; therefore, the design could benefit from an interface or proper restructuring.
Best Practices for Inheritance in Java
To avoid the pitfalls discussed, here are some best practices to maintain effective inheritance structures:
-
Prefer Composition Over Inheritance: Use composition to create complex types from simpler types instead of creating long chains of inheritance.
-
Keep Class Hierarchies Shallow: Aim for a simple and understandable class hierarchy while keeping future extensions in mind.
-
Utilize Interfaces and Abstract Classes: They can provide a contract for what methods a class should implement without enforcing a strict inheritance of implementation.
-
Use Proper Access Modifiers: Protect your class members, so only intended elements are exposed, thus maintaining encapsulation.
-
Refactor When Necessary: If your inheritance structure becomes cumbersome, take the time to refactor it. Keeping code clean is vital for long-term maintenance.
-
Document Your Code: Ensure that your classes and their hierarchies are well-documented, which will help other developers (including future you) understand the structure and decisions made.
The Bottom Line
Mastering inheritance in Java OOP is not just about understanding how it works but also knowing the common mistakes and how to avoid them. By adhering to best practices and constantly improving your design approaches, you can create flexible, maintainable, and robust software. Inheritance can simplify your code significantly when used effectively but can also complicate it dramatically if misused.
For further reading on general OOP principles, consider visiting Oracle's official documentation.
By being aware of these nuances and taking a thoughtful approach to OOP principles, you can ensure that your Java applications are built with efficiency and clarity in mind. Happy coding!