Unlocking Java Secrets: Mastering Encapsulation Challenges
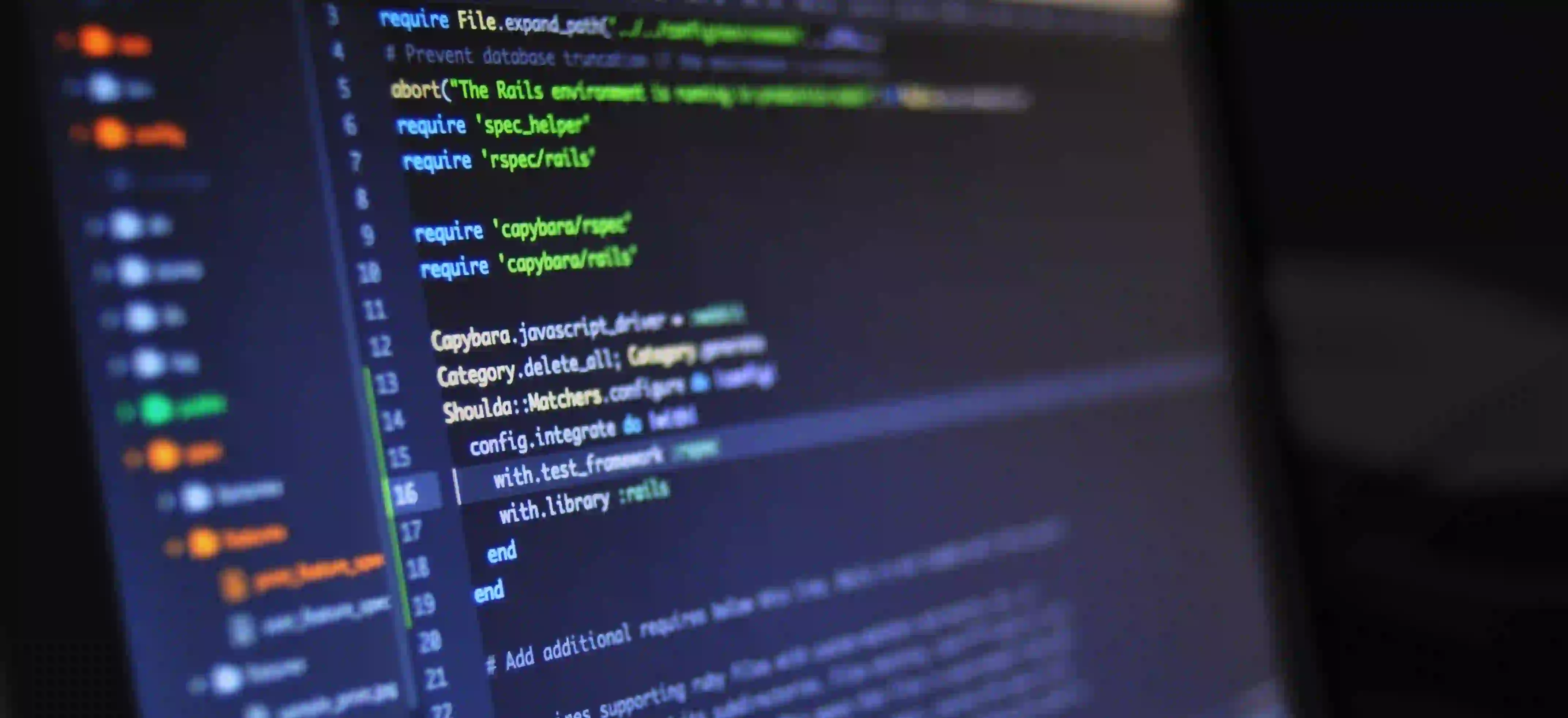
Unlocking Java Secrets: Mastering Encapsulation Challenges
Encapsulation is one of the foundational principles of object-oriented programming (OOP). In Java, it allows developers to wrap data and methods that operate on the data within a single unit, typically a class. While encapsulation is crucial in building robust applications, it can also pose unique challenges. This blog post will delve into the intricacies of encapsulation in Java, explore common pitfalls, and provide practical solutions through illustrative code snippets.
Understanding Encapsulation
Before we dive into practical challenges, let’s clarify what encapsulation actually means. In simple terms, encapsulation restricts direct access to some of an object's components and can prevent the accidental modification of data. By implementing access modifiers, we can control the visibility of class members.
Access Modifiers in Java
Java provides four primary access modifiers:
- public: Members are accessible from any other class.
- private: Members are accessible only within the same class.
- protected: Members are accessible within the same package and by subclasses.
- default (no modifier): Members are accessible within the same package only.
Example Code: Access Modifiers
Here’s a simple demonstration of how these access modifiers work:
public class Vehicle {
private String brand; // Only accessible within this class
protected int speed; // Accessible in subclasses and same package
public int year; // Accessible everywhere
public Vehicle(String brand, int speed, int year) {
this.brand = brand;
this.speed = speed;
this.year = year;
}
public String getBrand() {
return brand; // Getter for private member
}
public void setSpeed(int speed) {
this.speed = speed; // Setter for protected member
}
}
In the example above:
- The
brand
variable is private, making it inaccessible outside theVehicle
class. - The
speed
variable is protected, allowing access in subclasses. - The
year
variable is public, accessible from any class.
The Benefits of Encapsulation
Encapsulation provides various advantages:
- Control: It offers control over data by restricting unauthorized access.
- Flexibility: Internal implementation can change without affecting external code.
- Maintenance: Easier maintenance leads to fewer bugs in the application.
Challenges with Encapsulation
While encapsulation is beneficial, it poses several challenges for developers, particularly in larger applications. Below, we outline a few common issues and provide solutions.
1. Overusing Getters and Setters
One of the most common pitfalls is the indiscriminate use of getters and setters. While these methods can provide controlled access, frequent use may lead to exposing internal structure unnecessarily.
Code Example: Overusing Getters and Setters
public class Employee {
private String name;
private double salary;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
}
Why this is a problem? With the above structure, one can change an employee's salary to an invalid value without any checks.
Solution: Implement validation within setters to maintain encapsulation integrity.
public void setSalary(double salary) {
if (salary < 0) {
throw new IllegalArgumentException("Salary cannot be negative");
}
this.salary = salary;
}
2. Exposure to Immutable Objects
While encapsulation encourages controlled access, it can sometimes lead to the creation of immutable objects, which may not always be ideal. For instance, what if you want to allow modification but still maintain certain control over the internal state?
Code Example: Mutable Class with Controlled Access
public class Circle {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
if (radius > 0) {
this.radius = radius;
} else {
throw new IllegalArgumentException("Radius must be positive");
}
}
}
By controlling the radius's mutability through the setter, encapsulation maintains the integrity of our object.
3. Chaotic Complexity in Object Interactions
As applications scale up, keeping track of various object interactions can become challenging. This complexity can lead to tightly coupled classes, which can be difficult to maintain and test.
Solution: Aggregate Objects into Larger Structures
One effective approach is to aggregate related object data into larger data structures or services. For example, consider a Team
class that encapsulates Employee
objects.
import java.util.ArrayList;
import java.util.List;
public class Team {
private List<Employee> employees;
public Team() {
this.employees = new ArrayList<>();
}
public void addEmployee(Employee employee) {
employees.add(employee);
}
public void printTeamDetails() {
for (Employee emp : employees) {
System.out.println("Name: " + emp.getName() + ", Salary: " + emp.getSalary());
}
}
}
This allows encapsulation at the Team
level, controlling how employees are managed without exposing individual employee manipulation directly.
The Closing Argument
Encapsulation is a powerful principle of Java that, when understood and applied correctly, can lead to well-structured and maintainable code. However, developers must be cautious of common pitfalls like the overuse of getters and setters, exposure to immutable objects, and maintaining control over complex object interactions.
By implementing best practices — such as strategic validation, thoughtful design of object relationships, and judicious use of access modifiers — you can unlock the full potential of encapsulation in Java.
To further your understanding of object-oriented programming in Java, consider exploring the Oracle Java Tutorials, where you can find more in-depth discussions on encapsulation, inheritance, and polymorphism.
Happy coding!