Mastering String Manipulation: Common Pitfalls in Java
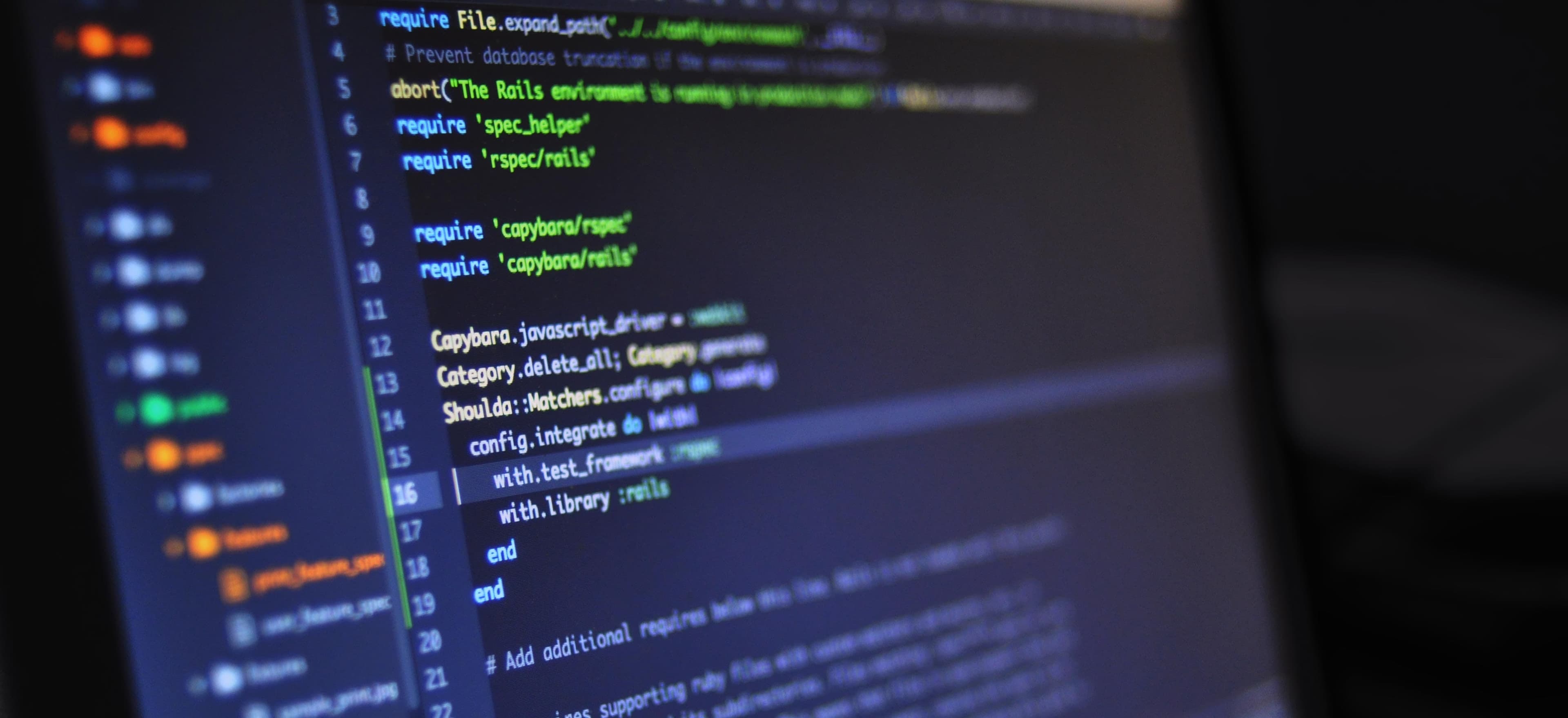
- Published on
Mastering String Manipulation: Common Pitfalls in Java
Java is renowned for its robust functionalities, and string manipulation is one area where developers often need to tread carefully. The language provides various tools and methods to work with text; however, not all methods are created equal, and pitfalls can lead to inefficient code or, worse, bugs. This post will explore common pitfalls in string manipulation in Java, offering practical guidance, tips, and code snippets designed to boost your understanding and coding efficiency.
The String Class: Immutable, But Why?
Before we delve into pitfalls, we should clarify a fundamental aspect of Java: strings are immutable. When a string is created, it cannot be modified. Instead, any operation that appears to change the string actually creates a new one. This characteristic, while it ensures data integrity and security, can cause performance issues if not handled properly.
Why is this important? Understanding string immutability helps you choose the right data structures or methods when modifying strings frequently.
Here’s a simple example illustrating immutability:
String original = "Hello";
String modified = original.replace("H", "J");
System.out.println(original); // Outputs: Hello
System.out.println(modified); // Outputs: Jello
In this example, original
remains unchanged. The replace
method returns a new string, demonstrating immutability clearly.
Pitfall 1: String Concatenation Using +
Many developers often use the +
operator for string concatenation, which can lead to performance issues, especially inside loops. Due to immutability, each concatenation creates a new string, leading to increased memory use and longer execution times.
More Efficient Option: StringBuilder
For multiple concatenations, it is advisable to employ StringBuilder
:
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 10; i++) {
sb.append("Number: ").append(i).append("\n");
}
String result = sb.toString(); // Convert StringBuilder to String
System.out.println(result);
Why use StringBuilder
? It's mutable and allows for efficient appending, reducing performance overhead, especially as the size of the final string grows.
Pitfall 2: Using String.equals()
Instead of ==
When checking for string equality, developers sometimes mistakenly use the ==
operator, which checks for reference equality, rather than the String.equals()
method that checks for value equality.
Example of Common Mistake:
String str1 = new String("Hello");
String str2 = new String("Hello");
System.out.println(str1 == str2); // Outputs: false
System.out.println(str1.equals(str2)); // Outputs: true
In this example, str1
and str2
point to different objects, thus ==
returns false.
Ensure proper string equality checks with String.equals()
. For case-insensitive checks, you can use:
System.out.println(str1.equalsIgnoreCase(str2)); // Outputs: true
Pitfall 3: Not Accounting for Null Strings
Another frequent pitfall is failing to account for potential null strings when performing operations. Null pointer exceptions can cause your application to crash.
Defensive Programming: Checking for null
Always check for null before performing any operations:
String str = null;
if (str != null && str.length() > 0) {
System.out.println(str.toUpperCase());
} else {
System.out.println("String is null or empty.");
}
Why this check? It ensures your program doesn’t break due to unexpected null values, enhancing reliability.
Pitfall 4: Using String
for Frequent Modifications
If you find yourself changing strings frequently, consider using alternatives like StringBuilder
or StringBuffer
. While StringBuffer
is synchronized (hence thread-safe), StringBuilder
is the preferred choice due to its lack of synchronization overhead, particularly in single-threaded applications.
Performance Benchmark Example
Below is a simple benchmark showing the performance differences:
public static void main(String[] args) {
long startTime = System.nanoTime();
String str = "";
for (int i = 0; i < 10000; i++) {
str += "Number: " + i + "\n"; // Poor performance
}
long duration = System.nanoTime() - startTime;
System.out.println("Time taken with String concatenation: " + duration + " ns");
startTime = System.nanoTime();
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 10000; i++) {
sb.append("Number: ").append(i).append("\n"); // Better performance
}
duration = System.nanoTime() - startTime;
System.out.println("Time taken with StringBuilder: " + duration + " ns");
}
Expectation
The results will typically show that StringBuilder
outperforms String
concatenation by a significant margin.
Pitfall 5: Regular Expressions Performance
Using string manipulation with regular expressions can lead to unexpected performance issues especially with poorly designed patterns. While regex is powerful, it can also be resource-intensive.
Practice Efficient Regex
Use simpler expressions when possible. Here's an example:
String input = "One, Two, Three";
String[] parts = input.split(",\\s*"); // Efficiently split by comma and trimming spaces
for (String part : parts) {
System.out.println(part);
}
Why not use complex patterns? They may slow down your application, leading to unexpected delays.
Summary: Building a Solid Understanding
Mastering string manipulation in Java involves understanding the potential pitfalls that come with it. Keeping track of immutability, object references, null values, and performance issues will not only safeguard your code against common bugs but also enhance efficiency.
Properly using StringBuilder
, ensuring null checks, and avoiding complex regex patterns are vital practices every developer should adopt.
For further reading on Java Strings, I recommend checking out the Oracle Java Documentation and Effective Java by Joshua Bloch for deeper insights.
By embracing these practices and avoiding common pitfalls, you will enhance your capability to handle string operations, sticking to Java's principles of reliability and performance.
Checkout our other articles