Mastering Java Exception Handling: Common Pitfalls to Avoid
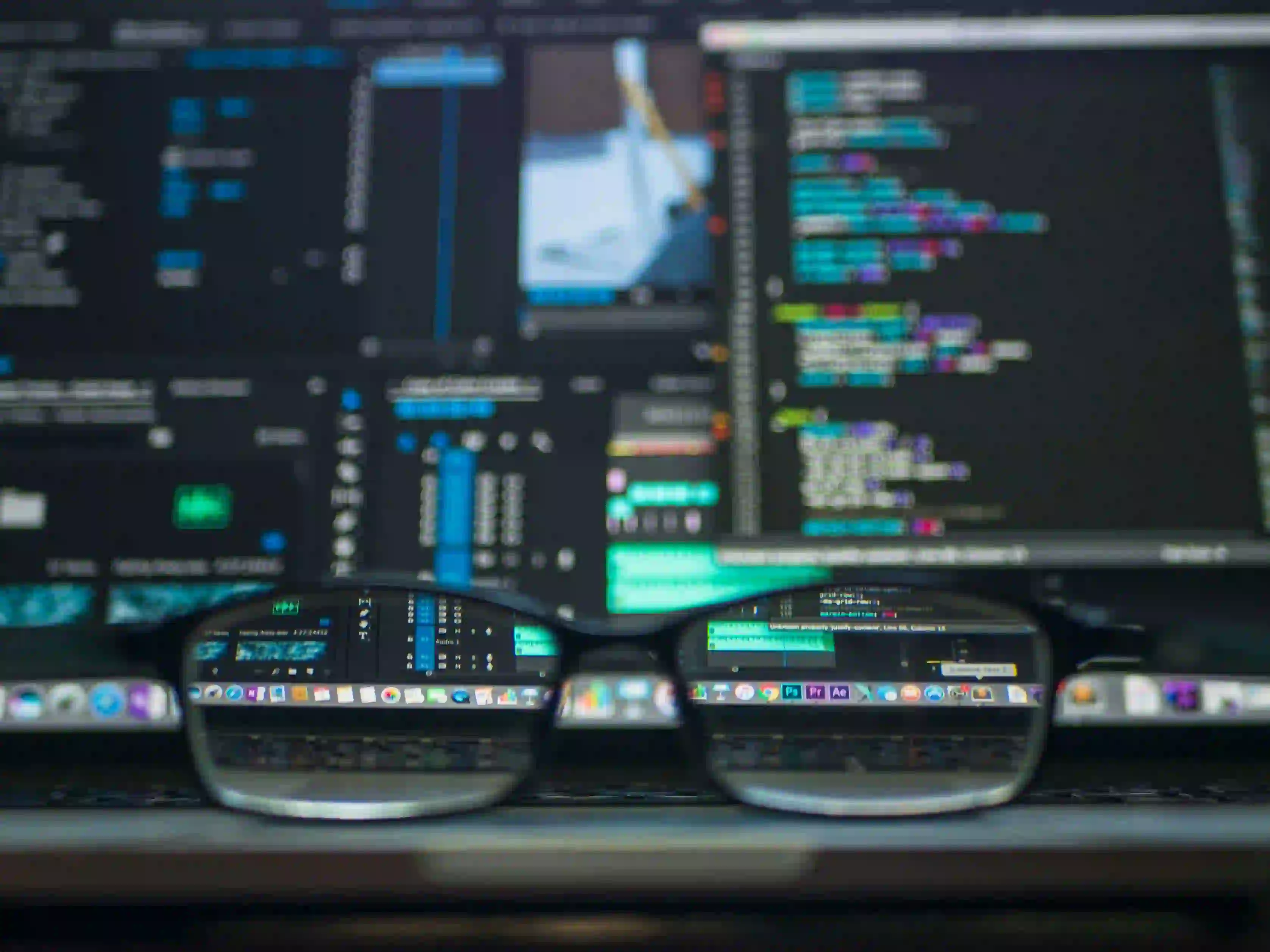
Mastering Java Exception Handling: Common Pitfalls to Avoid
Exception handling is an integral part of robust Java programming. It enables developers to manage errors gracefully and ensures that applications remain resilient in the face of unexpected situations. In this blog post, we will explore common pitfalls in Java exception handling, and I will provide actionable insights to help developers master this crucial skill.
What is Exception Handling?
Exception handling in Java involves using specific language constructs to manage the occurrence of unexpected events that disrupt the normal flow of a program. An exception is an object that describes an unusual condition that has occurred in the code. Java provides a structured mechanism for handling exceptions using try
, catch
, finally
, and throw
keywords.
Here’s a fundamental example to demonstrate how exception handling works:
public class ExceptionHandlingDemo {
public static void main(String[] args) {
try {
int result = divide(10, 0);
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
System.out.println("Exception caught: " + e.getMessage());
} finally {
System.out.println("Execution complete.");
}
}
public static int divide(int a, int b) {
return a / b; // Possible ArithmeticException if b is 0
}
}
In this example, the divide
method will throw an ArithmeticException
if we try to divide by zero. The catch
block catches this exception and prevents the program from crashing.
Why Exception Handling is Essential
- Prevent Program Crashes: Without proper exception management, user applications may unexpectedly terminate.
- Graceful Degradation: It allows applications to continue functioning, potentially offering default behavior or recovery options.
- Debugging Assist: Helps identify the origin of errors, making debugging more manageable.
For an in-depth understanding of exceptions in Java, check out the official Java Exception Handling documentation.
Common Pitfalls in Java Exception Handling
While exception handling is a straightforward concept, several common pitfalls can lead to ineffective or suboptimal code. Let’s feast our eyes on these pitfalls and investigate how to avoid them.
1. Overuse of Exception Handling
Pitfall: Using exceptions for normal control flow is a common mistake among novice developers. Wrapping normal conditional checks in a try-catch
block can degrade performance.
Solution: Use exceptions only for exceptional situations, not for regular control flow.
public class OveruseExample {
public static void main(String[] args) {
try {
int num = getNumber(); // Assume this method can throw an exception
System.out.println("User number: " + num);
} catch (Exception e) {
// This is not a good design if you're expecting it to fail normally
System.out.println("An error occurred: " + e.getMessage());
}
}
private static int getNumber() {
// Simulate a normal flow; do not use exceptions for validation
return -1; // return -1 where it could signify an error state, not an exception!
}
}
2. Catching General Exceptions
Pitfall: Catching Exception
or Throwable
can obscure the actual issue, making debugging difficult.
Solution: Be specific about the exceptions you catch to gain clarity.
try {
// Some risky code
} catch (IOException e) {
// Handle IOException specifically
} catch (SQLException e) {
// Handle SQLException specifically
} catch (Exception e) {
// Generic catch should be the last resort
}
3. Ignoring Exception Details
Pitfall: Failing to log or otherwise utilize exception stack traces can lead to missed opportunities for debugging.
Solution: Always log the stack trace and meaningful messages when catching exceptions.
import java.util.logging.*;
public class LoggingExample {
private static final Logger logger = Logger.getLogger(LoggingExample.class.getName());
public static void main(String[] args) {
try {
riskyOperation();
} catch (Exception e) {
logger.log(Level.SEVERE, "An error occurred", e); // Log the exception details
}
}
private static void riskyOperation() throws Exception {
// Simulate risky operation
throw new Exception("Something went wrong!");
}
}
4. Not Using Finally Block
Pitfall: Forgetting to release resources (like database connections or file handlers) in case of exceptions.
Solution: Use a finally
block or try-with-resources statement for resource management.
import java.io.*;
public class ResourceManagement {
public static void main(String[] args) {
try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
System.out.println("Failed to read the file: " + e.getMessage());
}
// The reader will automatically close, even if an exception occurs
}
}
5. Not Propagating Exceptions
Pitfall: Catching an exception without re-throwing or properly handling it can lead to silent failures.
Solution: Rethrow exceptions or wrap them in RuntimeException
if necessary.
public void readFile(String fileName) {
try {
// code that may throw an IOException
} catch (IOException e) {
// log and rethrow as a runtime exception
throw new RuntimeException("Failed to read file: " + fileName, e);
}
}
6. Swallowing Exceptions
Pitfall: Catching an exception with an empty catch block leads to hiding errors, making debugging impossible.
Solution: Always handle exceptions in a meaningful way.
try {
// Some code that could throw an exception
} catch (Exception e) {
// Don't do this: simply ignore the exception
// Log the exception or throw a custom exception
}
7. Failure to Validate Input
Pitfall: Not validating input can lead to unexpected exceptions.
Solution: Always validate user input and manage exceptions when they do arise.
public void setAge(int age) {
if (age < 0) {
throw new IllegalArgumentException("Age cannot be negative");
}
this.age = age;
}
The Closing Argument: Empowering Your Java Exceptions Handling
Mastering Java exception handling is not just about writing code that works. It’s about creating a resilient application that can handle failures gracefully and provide a smooth user experience. By avoiding the common pitfalls discussed above, developers can write clearer, more efficient code and enhance the maintainability and robustness of their applications.
Always remember: Exception handling is not just a safety net; it's an integral piece of your application’s architecture.
For further reading on best practices and more advanced concepts in exception handling, feel free to visit the following resources:
- Effective Java by Joshua Bloch - A must-read book that covers many best practices, including exception handling.
- Java Brains on Exception Handling - A video tutorial that can help deepen your understanding of exception management strategies.
By applying these principles, you will not only avoid common pitfalls but will also enhance your journey to become a well-rounded Java developer. Happy coding!