Understanding Java's Collection Interfaces: Common Pitfalls
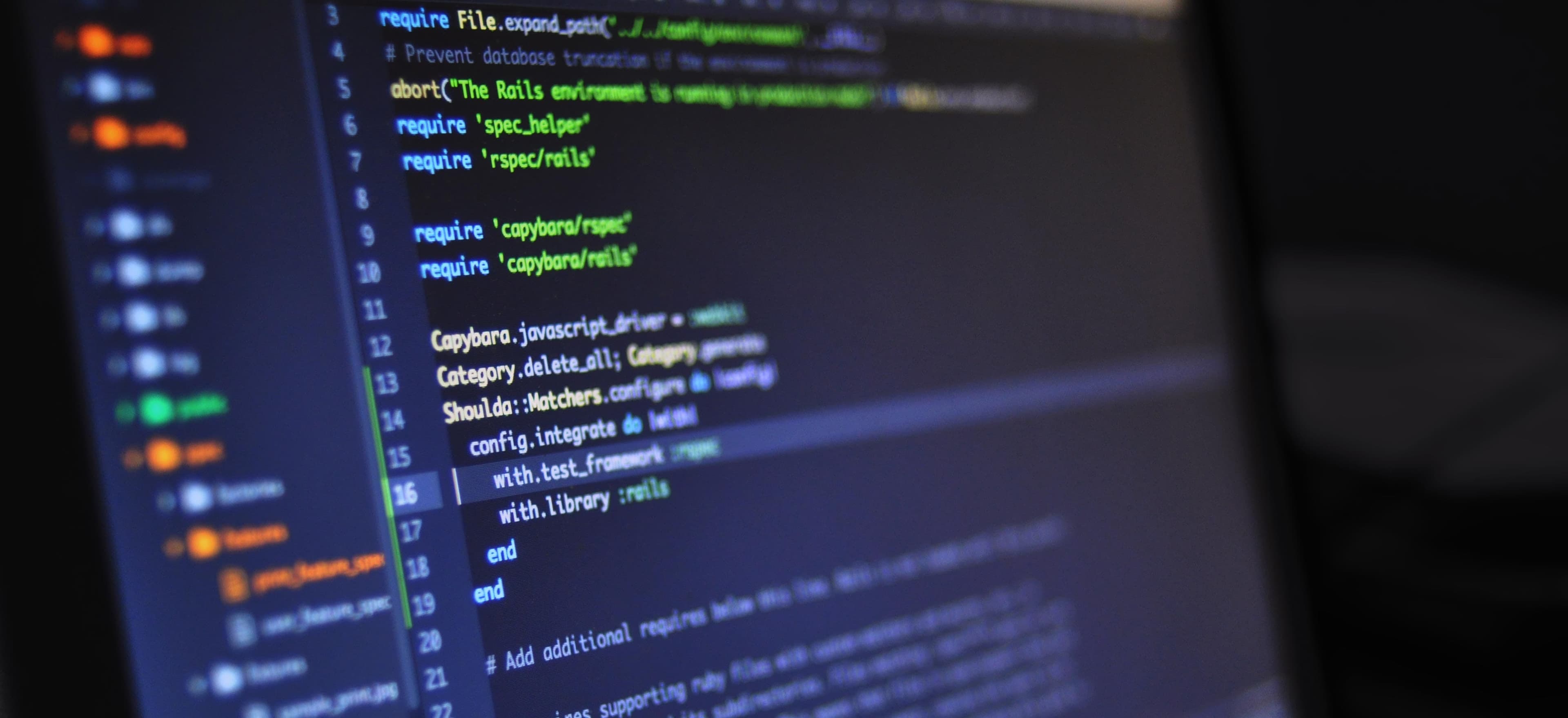
- Published on
Understanding Java's Collection Interfaces: Common Pitfalls
Java's Collection Framework is an essential part of the Java programming language, offering a powerful and flexible way to manage and manipulate groups of objects. However, newcomers often encounter common pitfalls when working with Java's collection interfaces. This blog post aims to demystify these interfaces, elucidate their functionalities, and highlight typical mistakes programmers make. We’ll also include practical examples to solidify your understanding.
What are Collection Interfaces?
Java's Collection Framework consists of interfaces, implementations, and algorithms to help manage collections of objects. The core interfaces in the framework are:
- Collection: This is the root interface for all collections.
- List: An ordered collection (also known as a sequence).
- Set: A collection that does not allow duplicate elements.
- Map: An object that maps keys to values.
Each interface serves its own purpose, but they also form a cohesive framework that allows for a diverse range of data structures.
Key Characteristics of Collection Interfaces
- Dynamic Size: Unlike arrays, collections can grow and shrink dynamically.
- Interchangeability: You can often interchange implementations, thanks to polymorphism. For example, you can use a
List
in place of aLinkedList
.
Common Pitfalls and How to Avoid Them
1. Choosing the Wrong Implementation
One common mistake is selecting an inappropriate implementation for your needs. For instance, using ArrayList
when you need frequent insertions and deletions could lead to performance issues since ArrayList
has to shift elements.
Consider this code snippet:
List<String> list = new ArrayList<>();
list.add("First");
list.add("Second");
list.add(1, "Third"); // Inserting at index 1
System.out.println(list); // Output: [First, Third, Second]
While this works, if your application frequently inserts and deletes elements at the middle of the list, a LinkedList
would be more efficient:
List<String> list = new LinkedList<>();
list.add("First");
list.add("Second");
list.add(1, "Third"); // Efficient insertion
System.out.println(list); // Output: [First, Third, Second]
2. Ignoring Type Safety
Java is a statically typed language, yet many developers overlook the benefits of generics. Using raw types can lead to ClassCastException
at runtime.
Example of a pitfall:
List list = new ArrayList();
list.add("String");
list.add(10); // Adding an Integer
String str = (String) list.get(1); // This will throw ClassCastException
Instead, always specify the type:
List<String> list = new ArrayList<>();
list.add("String");
// list.add(10); // Compilation error
String str = list.get(0); // Now it's safe
3. Misunderstanding Set Behavior
Sets in Java do not allow duplicate entries. However, many beginners are unclear about how hash codes work with hash-based sets like HashSet
.
Set<String> set = new HashSet<>();
set.add("Hello");
set.add("Hello"); // Duplicate, will not be added
System.out.println(set); // Output: [Hello]
If you need to store elements that consider equal if they match certain criteria, this can lead to unexpected behavior unless you override hashCode
and equals
.
4. Mixing Collection Types
Mixing different types of collections can lead to confusion. For example, adding a List
to a Set
of String
can create ambiguity:
Set<String> set = new HashSet<>();
List<String> list = new ArrayList<>();
list.add("One");
set.addAll(list); // This is fine
set.add("Two");
System.out.println(set); // Output: [One, Two] (if 'One' was not added more than once)
This might give you a false sense of security as it can lead to unexpected results when checking sizes or iterating through them.
5. Failing to Use Iterators Properly
An often-forgotten best practice is to use iterators when modifying collections during iteration. The following code can cause a ConcurrentModificationException
:
List<String> list = new ArrayList<>(Arrays.asList("A", "B", "C"));
for (String item : list) {
if ("B".equals(item)) {
list.remove(item); // This line will throw ConcurrentModificationException
}
}
The correct approach is to use an Iterator
:
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String item = iterator.next();
if ("B".equals(item)) {
iterator.remove(); // Safe way to remove elements
}
}
6. Overusing Synchronized Collections
While thread safety is vital in multi-threaded applications, using synchronized collections unnecessarily can lead to performance bottlenecks.
List<String> syncList = Collections.synchronizedList(new ArrayList<>());
// Too heavy for a single-threaded context
Consider using concurrent collections such as CopyOnWriteArrayList
when you need to handle multiple threads reading and writing simultaneously.
Closing the Chapter
Understanding Java's Collection interfaces is fundamental for any developer. Being aware of the common pitfalls can save you a lot of time and frustration. Whether you’re building small applications or complex systems, choosing the proper interface and implementation can lead to more efficient, maintainable code.
For further reading on Java's Collection Framework, consider checking the official Java documentation or diving into Java's best practices.
As you continue to work with various collections, keep these tips in mind, and you'll navigate Java's powerful Collection Framework with confidence. Happy coding!
Checkout our other articles