Mastering Java Collections: Avoiding Common Pitfalls
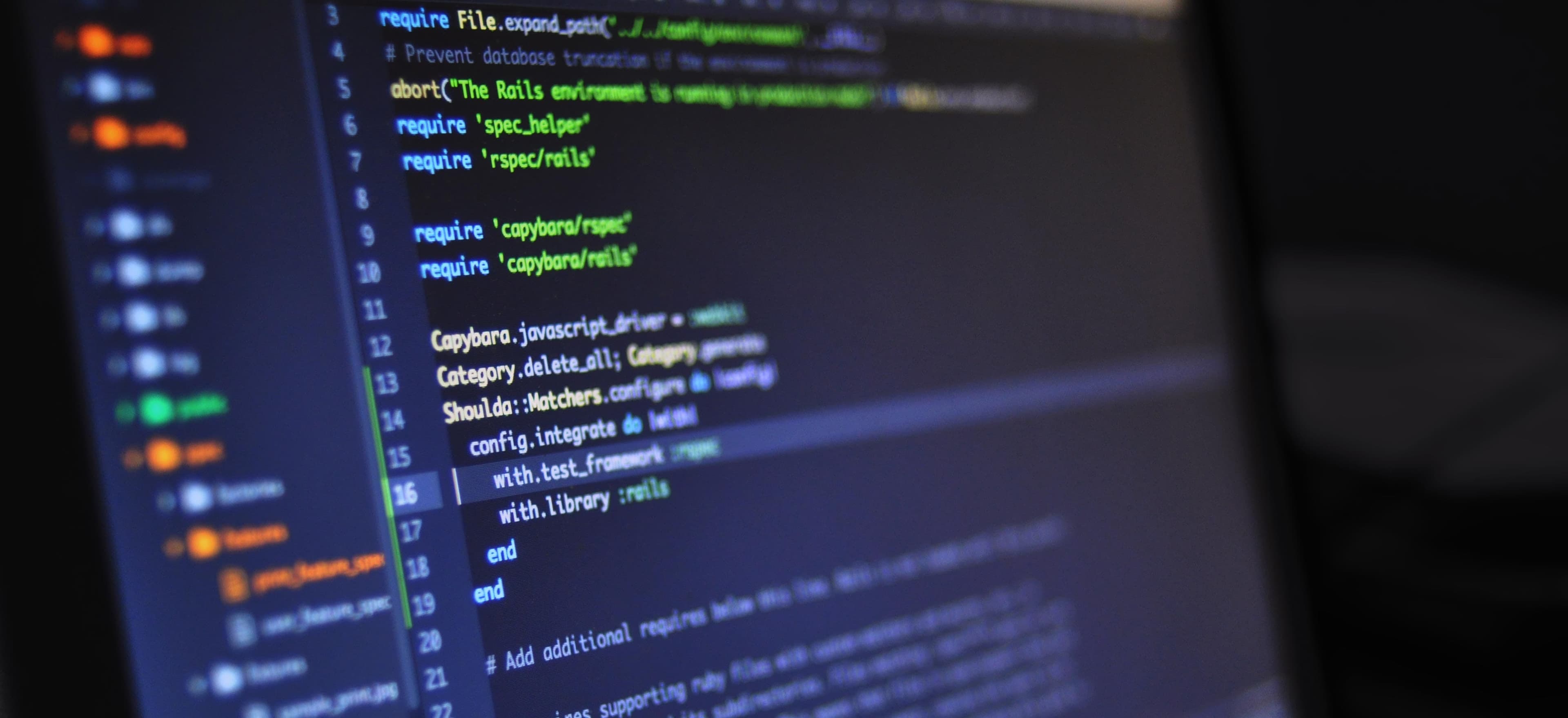
- Published on
Mastering Java Collections: Avoiding Common Pitfalls
Java Collections Framework (JCF) is a powerful and versatile feature of Java that allows programmers to work with groups of objects more efficiently. However, the vastness of JCF can sometimes lead developers astray, especially novices. In this blog post, we'll take a deep dive into common pitfalls associated with Java collections and how to avoid them, thereby helping you master this essential part of the language.
Understanding Java Collections Framework
Before we go into the pitfalls, let's quickly summarize what JCF is. The Java Collections Framework provides data structures like lists, sets, maps, and others, along with algorithms to manipulate these collections. Proper usage of these collections helps optimize performance and maintain code clarity.
Some commonly used interfaces and classes in JCF include:
- List: An ordered collection (also known as a sequence). Implementations:
ArrayList
,LinkedList
. - Set: A collection that doesn't allow duplicate elements. Implementations:
HashSet
,LinkedHashSet
,TreeSet
. - Map: An object that maps keys to values. Implementations:
HashMap
,LinkedHashMap
,TreeMap
.
Pitfall #1: Confusing List Implementations
Many developers tend to use ArrayList
for all list operations, thinking it is always the best choice. However, different scenarios call for different implementations.
Example:
List<String> arrayList = new ArrayList<>();
arrayList.add("Apple");
arrayList.add("Banana");
arrayList.add("Mango");
// Searching an element in ArrayList is O(n)
if (arrayList.contains("Banana")) {
System.out.println("Banana is in the list.");
}
// Use LinkedList when your application requires frequent insertion and deletion
List<String> linkedList = new LinkedList<>();
linkedList.add("Apple");
linkedList.add("Banana");
linkedList.add("Mango");
// Insertion and deletion is O(1)
linkedList.add(1, "Grape");
Why? ArrayList
provides faster random access but slower insertions/deletions, while LinkedList
excels in insertions and deletions but is slower for random access. Choose based on your application's requirements.
Pitfall #2: Ignoring Null Values in Collections
Some developers mistakenly assume that a collection can house null values without issues. While it's true that many collection types do allow nulls, mishandling them can lead to NullPointerExceptions
.
Example:
List<String> list = new ArrayList<>();
list.add(null); // This is allowed
if (list.contains(null)) {
System.out.println("List contains null.");
}
Why? Always validate your data. Use Optional
to tackle potential nulls more gracefully. Here's an example of using Optional
:
Optional<String> optionalString = Optional.ofNullable(list.get(0)); // Safe retrieval
optionalString.ifPresent(System.out::println); // Print if present
Pitfall #3: Modifying Collections During Iteration
Modifying a collection while iterating through it can lead to ConcurrentModificationException
. This is a common pitfall that can be easily overlooked.
Example:
List<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
for (String item : list) {
if (item.equals("Banana")) {
list.remove(item); // This throws ConcurrentModificationException
}
}
Solution? Use Iterator
's remove method instead:
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String item = iterator.next();
if (item.equals("Banana")) {
iterator.remove(); // Safe removal
}
}
Why? The Iterator
is designed to safely modify the collection during iteration.
Pitfall #4: Overusing Synchronized Collections
Java provides synchronized versions of collections, such as Collections.synchronizedList
or Collections.synchronizedMap
. Choosing them without necessity can lead to performance bottlenecks in single-threaded applications.
Example:
List<String> synchronizedList = Collections.synchronizedList(new ArrayList<>());
Why? Only utilize synchronized collections when multiple threads access the same collection. Otherwise, stick with the standard collection types for better performance.
Pitfall #5: Misusing HashMap
HashMap
is a widely used data structure, but its improper usage can lead to performance issues. A common misconception is that it's not necessary to worry about the initial capacity and load factor. This can result in multiple resizing operations.
Example:
Map<String, String> map = new HashMap<>(16, 0.75f); // Initial capacity & load factor
Why? Initialize HashMap
properly to minimize resizing and enhance performance. Proper initial capacity and load factor can boost performance significantly.
Best Practices for Using Java Collections
1. Prefer Interfaces Over Implementations
When declaring your collection types, always utilize the interface (e.g., List
, Set
, Map
) instead of the concrete implementation (e.g., ArrayList
, HashSet
). This boosts flexibility.
List<String> fruits = new ArrayList<>(); // Prefer this
2. Minimize Type Casting
Using generics minimizes type casting and potential ClassCastException
. Always use type parameters when declaring collections.
List<String> strings = new ArrayList<>(); // Type-safe
3. Consider Using Streams
With Java 8, streams provide an elegant way to process collections. They improve readability and maintainability.
List<String> fruits = Arrays.asList("Apple", "Banana", "Cherry");
fruits.stream()
.filter(fruit -> fruit.startsWith("B"))
.forEach(System.out::println);
4. Evaluate Performance
Sometimes, the “best” collection type is context-dependent. Assess your use case—look at factors such as insertion/deletion frequency and access speed.
Closing the Chapter
Mastering the Java Collections Framework involves understanding both its strengths and common pitfalls. By being aware of these pitfalls—including confusing implementations, null handling, concurrent modification issues, overusing synchronization, and improper use of HashMap
—you can enhance your coding efficiency and reliability.
Before implementing a collection in your Java application, always consider your specific use case and choose the right collection type accordingly. The guidelines laid out in this post are just the beginning; practice in real-world projects will further solidify your understanding of Java collections.
For additional reading around Java collections, check out the Oracle documentation on Collections or resources from Baeldung. Happy coding!
Checkout our other articles