Mastering Thread Safety: Avoiding Common Pitfalls in Java
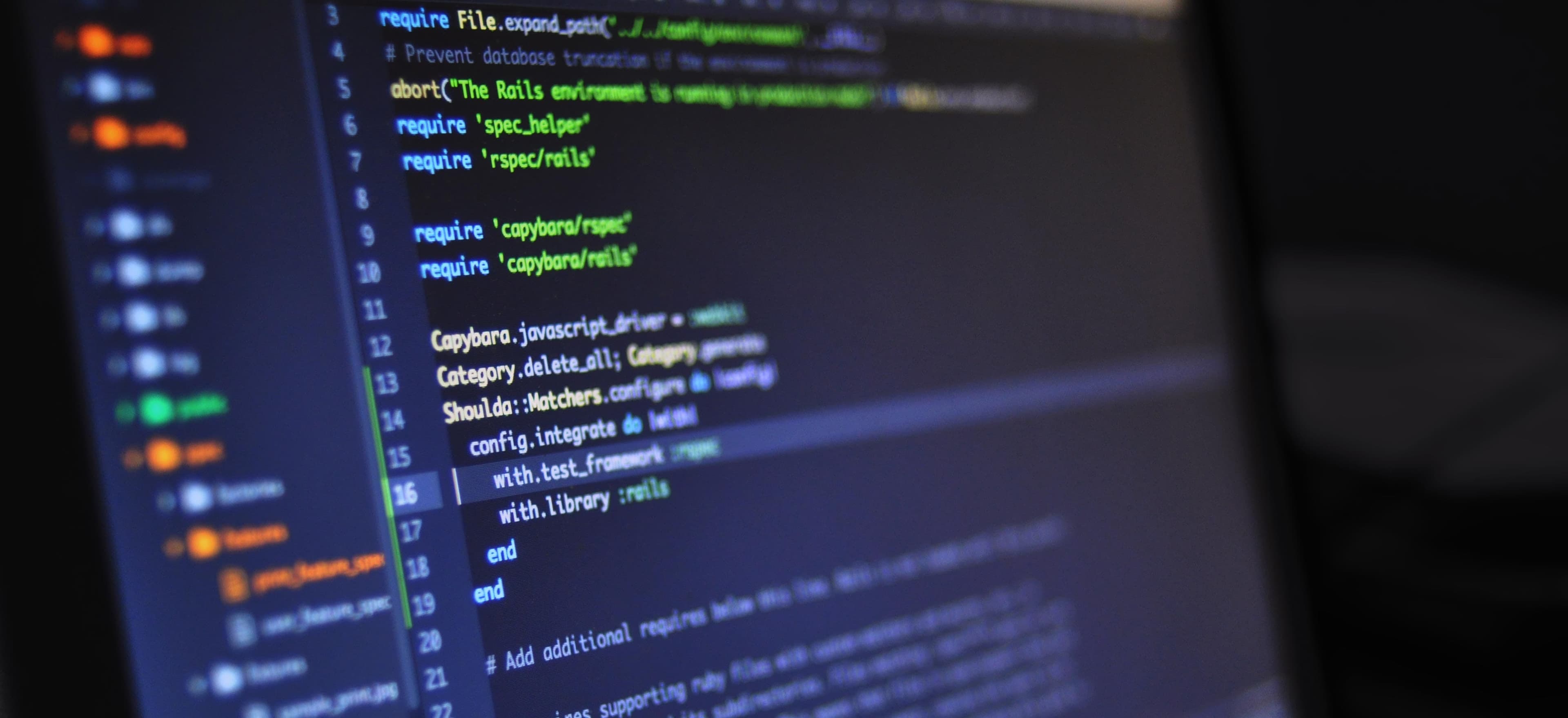
- Published on
Mastering Thread Safety: Avoiding Common Pitfalls in Java
In modern software development, multi-threading is often necessary for achieving optimal performance and responsiveness. However, when multiple threads access shared resources, issues related to thread safety can arise. This blog post aims to provide you with a comprehensive understanding of thread safety in Java, highlighting common pitfalls and how to avoid them.
What is Thread Safety?
Thread safety is the property of a program or code segment to operate correctly during simultaneous execution by multiple threads. When a piece of code is thread-safe, it guarantees that shared data remains consistent and valid, regardless of how many threads are accessing that data at the same time.
Conversely, non-thread-safe code can lead to various runtime issues, such as race conditions, deadlocks, and data corruption. For instance, if two threads modify the same variable simultaneously without synchronization, you cannot predict the final value of that variable.
Common Pitfalls in Thread Safety
1. Race Conditions
A race condition occurs when two or more threads access shared data at the same time, and at least one of the threads modifies that data. This can lead to unpredictable results.
Example:
public class Counter {
private int count = 0;
public void increment() {
count++;
}
public int getCount() {
return count;
}
}
In the increment method above, if multiple threads call increment()
, you can end up with an inaccurate count
. This happens because the read and write operations are not atomic.
Solution: Synchronized Methods
You can resolve race conditions by using synchronization techniques.
public class SynchronizedCounter {
private int count = 0;
public synchronized void increment() {
count++;
}
public synchronized int getCount() {
return count;
}
}
By marking the increment and getCount methods with the synchronized
keyword, you ensure that only one thread can execute them at a time. However, this approach can lead to reduced performance due to thread contention.
2. Deadlocks
A deadlock is a state in which two or more threads are unable to proceed because each is waiting for the other to release a resource. Therefore, a deadlock leads to a complete halt in execution.
Example:
public class DeadlockExample {
private final Object lock1 = new Object();
private final Object lock2 = new Object();
public void method1() {
synchronized (lock1) {
synchronized (lock2) {
// critical section
}
}
}
public void method2() {
synchronized (lock2) {
synchronized (lock1) {
// critical section
}
}
}
}
In this example, if one thread acquires lock1
and the other acquires lock2
, a deadlock will occur when each thread attempts to acquire the lock held by the other.
Solution: Lock Ordering
To prevent deadlocks, establish a strict order in which locks are acquired. Always acquire lock1
before lock2
and never in the reverse order.
3. Lost Updates
Lost updates usually occur when one thread overwrites the changes made by another thread without any coordination.
Example:
public class LostUpdate {
private int balance;
public void deposit(int amount) {
int newBalance = balance + amount;
// Simulated time delay
balance = newBalance; // This may lose updates
}
public int getBalance() {
return balance;
}
}
If two threads simultaneously invoke deposit
, they might read the same value of balance
, calculate the same newBalance
, and effectively “lose” one of the updates.
Solution: Atomic Variables
To prevent lost updates, you can use Atomic classes provided in java.util.concurrent.atomic
.
import java.util.concurrent.atomic.AtomicInteger;
public class AtomicBalance {
private final AtomicInteger balance = new AtomicInteger(0);
public void deposit(int amount) {
balance.addAndGet(amount);
}
public int getBalance() {
return balance.get();
}
}
Using AtomicInteger
ensures that both reading and writing to the balance
is atomic, thus preventing lost updates.
4. Thread Interference
Thread interference occurs when multiple threads operate on the same shared data without proper synchronization, leading to inconsistent results.
Example:
public class ThreadInterference {
private int count = 0;
public void increment() {
count++;
}
public void decrement() {
count--;
}
}
If two threads run increment and decrement simultaneously, the count
may not return to its expected value zero.
Solution: Synchronized Blocks
Utilize synchronized blocks for specific parts of your code where shared resources are accessed.
public class SynchronizedInterference {
private int count = 0;
public void increment() {
synchronized (this) {
count++;
}
}
public void decrement() {
synchronized (this) {
count--;
}
}
}
5. Using ThreadLocal
ThreadLocal
is a Java class that provides thread-local variables. It enables you to have a variable that is unique to each thread. This way, you can avoid sharing data across threads.
Example:
public class ThreadLocalExample {
private ThreadLocal<Integer> threadLocalCount = ThreadLocal.withInitial(() -> 0);
public void increment() {
threadLocalCount.set(threadLocalCount.get() + 1);
}
public int getCount() {
return threadLocalCount.get();
}
}
In this code, each thread has its own independent count, preventing any thread from interfering with another's state.
Additional Resources
For further reading on thread safety and concurrency in Java, consider exploring these insightful articles:
- Java Concurrency: The Java Tutorials
- Java Thread Safety
Closing the Chapter
Thread safety is a crucial aspect of writing reliable Java applications, especially as your programs grow in complexity. By understanding and avoiding common pitfalls such as race conditions, deadlocks, lost updates, and thread interference, you can write code that is both efficient and safe. Use the discussed techniques, like synchronization, atomic variables, and ThreadLocal
, to guard your applications against concurrency issues.
In a multi-threaded world, mastering thread safety is not just necessary—it's essential for robust software development. Embrace these concepts, and you will build applications that are both performant and reliable.
Checkout our other articles