Mastering Java I/O Streams: Common Pitfalls to Avoid
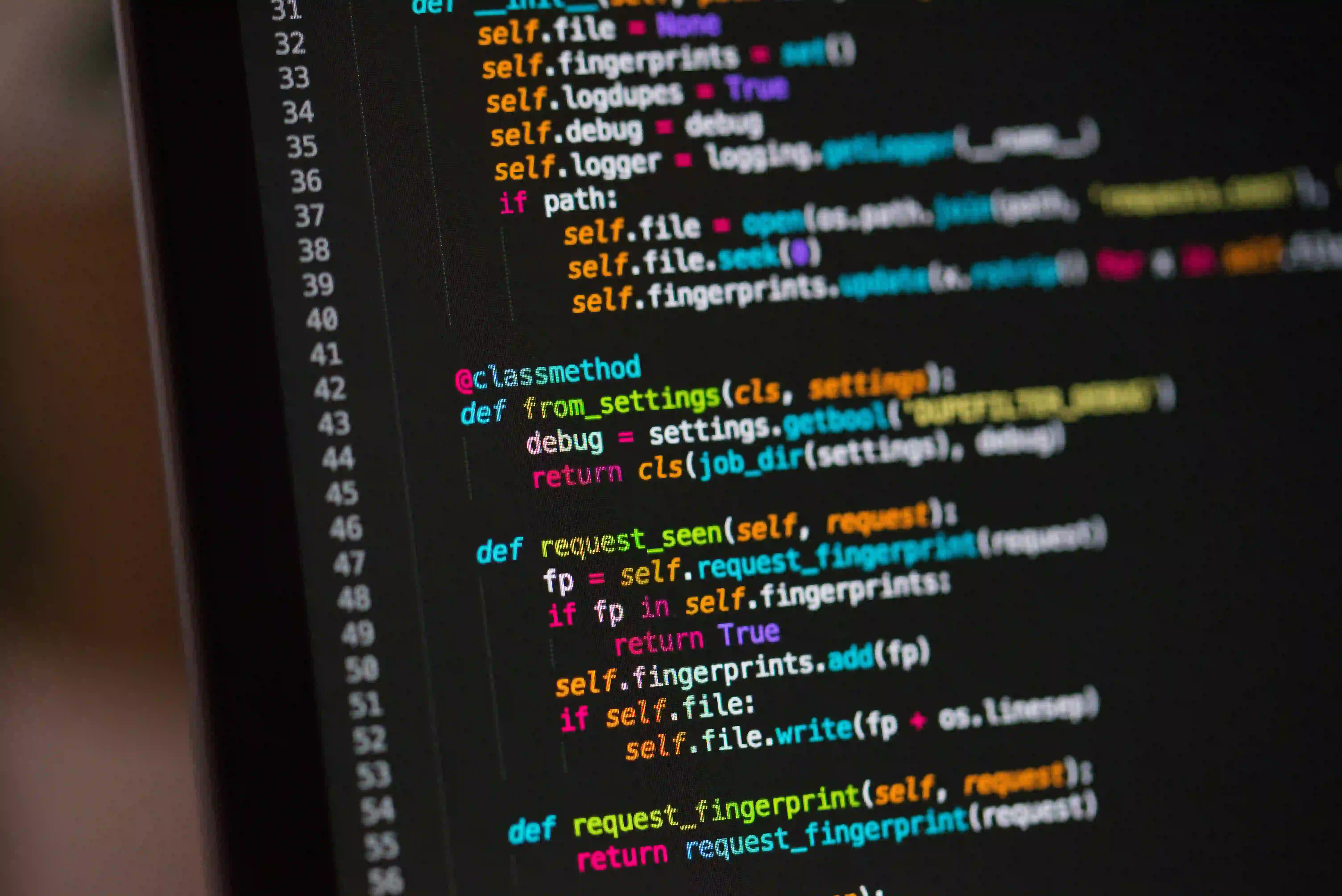
Mastering Java I/O Streams: Common Pitfalls to Avoid
Java's Input/Output (I/O) streams are an essential aspect of the language, allowing you to read from and write to files, sockets, and other data sources. However, many developers, especially newcomers, encounter several pitfalls when working with I/O streams. This blog post aims to illuminate some of these common errors, along with solutions to help you enhance your Java I/O proficiency.
Understanding I/O Streams
Before diving into the pitfalls, let’s briefly discuss what I/O streams are. Java I/O is primarily categorized into two types:
- Byte Streams: Allow for the reading and writing of binary data (e.g., image files, audio files).
- Character Streams: Designed for handling character data, providing support for international character sets.
Java provides several classes for both byte and character streams, located primarily in the java.io
package.
Pitfall #1: Forgetting to Close Streams
One of the most common mistakes when working with I/O streams is neglecting to close them. Not closing streams can lead to resource leaks, which might slow down your application or cause memory issues.
Example of Not Closing a Stream
import java.io.FileReader;
import java.io.IOException;
public class ReadFile {
public static void main(String[] args) {
FileReader fr = null;
try {
fr = new FileReader("sample.txt");
// Reading characters from the file
int data;
while ((data = fr.read()) != -1) {
System.out.print((char) data);
}
} catch (IOException e) {
e.printStackTrace();
}
// The stream is not closed here
}
}
Solution: Use try-with-resources
Java 7 introduced the try-with-resources statement, which automatically closes streams when they are no longer needed. This is the most recommended approach.
import java.io.FileReader;
import java.io.IOException;
public class ReadFile {
public static void main(String[] args) {
try (FileReader fr = new FileReader("sample.txt")) {
int data;
while ((data = fr.read()) != -1) {
System.out.print((char) data);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Using try-with-resources guarantees that fr
is closed, thus preventing resource leaks.
Pitfall #2: Ignoring Buffering
Reading and writing data byte by byte can significantly degrade performance. Many developers fail to implement buffering, leading to inefficient disk I/O operations.
Example of Direct Stream Usage
import java.io.FileReader;
import java.io.IOException;
public class ReadFile {
public static void main(String[] args) {
try (FileReader fr = new FileReader("largefile.txt")) {
int data;
while ((data = fr.read()) != -1) {
System.out.print((char) data);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
This method reads the file one character at a time, which is inefficient.
Solution: Use Buffered Streams
Instead, wrap your stream with a buffer:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class ReadFile {
public static void main(String[] args) {
try (BufferedReader br = new BufferedReader(new FileReader("largefile.txt"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Using BufferedReader
provides a mechanism to read larger chunks of data, improving overall performance.
Pitfall #3: Mismatched Stream Types
Another frequent error is using the wrong stream type. For example, attempting to read binary data with a character stream can lead to corrupted data.
Incorrect Usage Example
import java.io.FileReader;
import java.io.FileNotFoundException;
public class ReadBinaryFile {
public static void main(String[] args) {
try (FileReader fr = new FileReader("binaryfile.bin")) {
int data;
while ((data = fr.read()) != -1) {
System.out.print(data);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Using FileReader
here results in data loss since it’s designed for reading character streams, not binary.
Solution: Use Appropriate Stream Classes
For binary data, always opt for byte streams like FileInputStream
:
import java.io.FileInputStream;
import java.io.IOException;
public class ReadBinaryFile {
public static void main(String[] args) {
try (FileInputStream fis = new FileInputStream("binaryfile.bin")) {
int data;
while ((data = fis.read()) != -1) {
System.out.print(data + " ");
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Pitfall #4: Not Handling Exceptions Properly
I/O operations are prone to various exceptions, such as IOException
. Simply printing the stack trace isn’t always adequate for error handling.
Example of Poor Exception Handling
import java.io.FileReader;
import java.io.IOException;
public class ReadFile {
public static void main(String[] args) {
try (FileReader fr = new FileReader("sample.txt")) {
int data;
while ((data = fr.read()) != -1) {
System.out.print((char) data);
}
} catch (IOException e) {
e.printStackTrace(); // Not a good practice
}
}
}
Solution: Use Custom Error Handling
Instead of just printing the stack trace, manage your exceptions meaningfully:
import java.io.FileReader;
import java.io.IOException;
public class ReadFile {
public static void main(String[] args) {
try (FileReader fr = new FileReader("sample.txt")) {
int data;
while ((data = fr.read()) != -1) {
System.out.print((char) data);
}
} catch (IOException e) {
System.err.println("An error occurred while reading the file: " + e.getMessage());
// Optionally, you can log or throw a custom exception
}
}
}
Pitfall #5: Underestimating the Importance of Encoding
When dealing with text files, it’s crucial to specify the correct character encoding. Ignoring it can lead to data corruption or unexpected characters in the output.
Example of Using Default Encoding
import java.io.FileReader;
import java.io.IOException;
public class ReadFile {
public static void main(String[] args) {
try (FileReader fr = new FileReader("utf8file.txt")) {
int data;
while ((data = fr.read()) != -1) {
System.out.print((char) data);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
This code uses the platform's default encoding, which can lead to inaccuracies.
Solution: Specify Encoding Explicitly
Use InputStreamReader
to specify the encoding:
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStreamReader;
public class ReadFile {
public static void main(String[] args) {
try (BufferedReader br = new BufferedReader(new InputStreamReader(new FileInputStream("utf8file.txt"), "UTF-8"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Explicitly declaring the encoding ensures that your application processes the data correctly.
Key Takeaways
Mastering Java I/O streams involves being aware of these common pitfalls, as well as developing habits to avoid them. Always remember to close your streams, use buffering, match stream types correctly, handle exceptions properly, and specify encoding explicitly.
Adopting best practices in Java I/O will not only improve the efficiency of your applications but will also make your code more robust and maintainable.
For more insights and best practices on Java programming, you can explore resources such as Java Documentation and Baeldung on Java I/O.
Happy coding!