Common Pitfalls in Java Serialization You Must Avoid
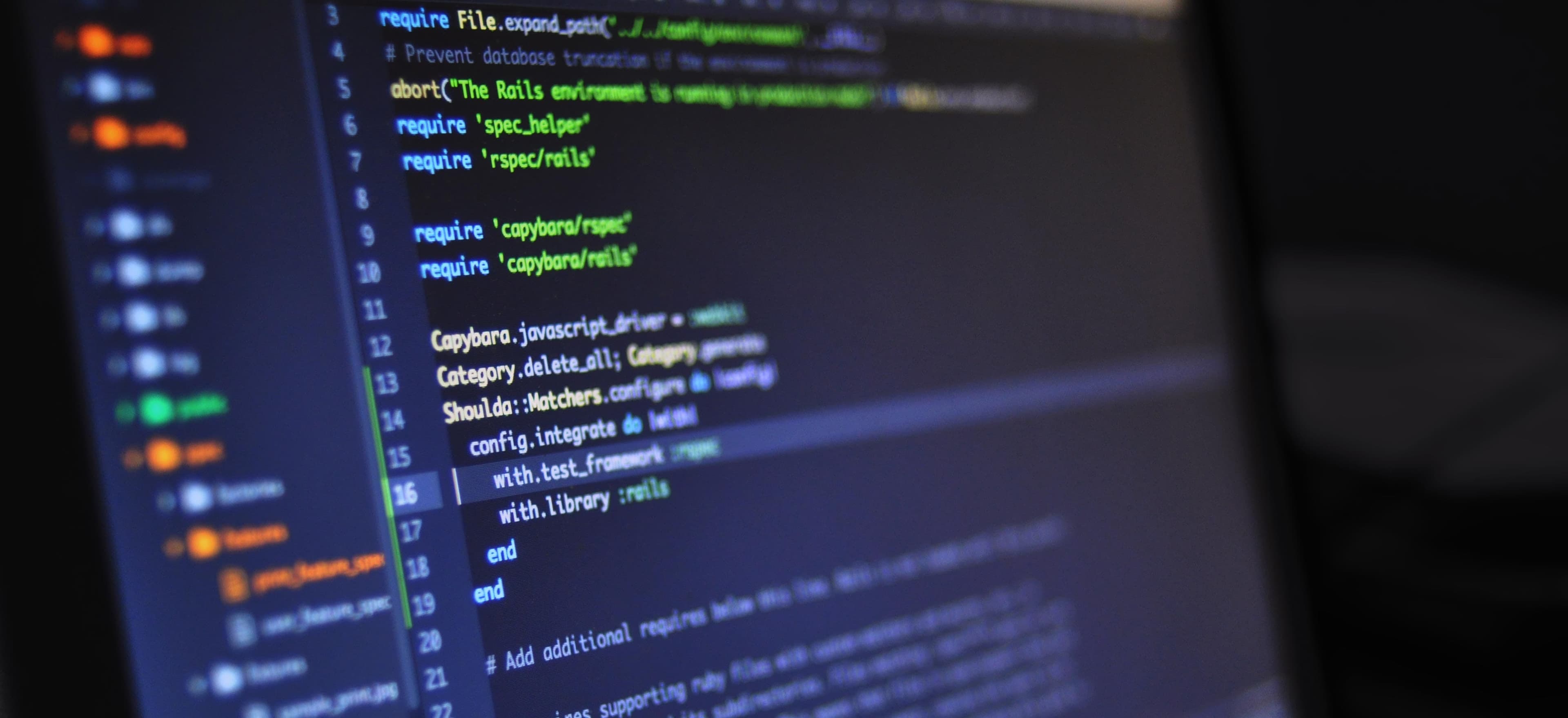
- Published on
Common Pitfalls in Java Serialization You Must Avoid
Java serialization is a powerful mechanism that allows you to convert an object into a byte stream for storage or transmission, enabling easy object persistence and data exchange. However, it comes with its own set of challenges. In this blog post, we’ll explore the common pitfalls in Java serialization that can lead to serialization failures or unwanted behavior. Each pitfall will be discussed in detail, providing you with insights and code snippets to help you avoid them.
What Is Java Serialization?
Java serialization is the process of converting an object into a sequence of bytes, which can then be easily saved to a file or transmitted over a network. The reverse process, deserialization, reconstructs the byte stream back into the original object. Serialization is primarily implemented through the Serializable
interface, which a class must implement for its instances to be serialized.
Example Code Snippet
import java.io.*;
class User implements Serializable {
private String username;
private int age;
public User(String username, int age) {
this.username = username;
this.age = age;
}
// Getters and toString method
}
Why: This example demonstrates a simple User
class that implements the Serializable
interface, allowing it to be serialized with ease. Skipping the implementation can lead to a NotSerializableException
.
Common Pitfalls in Java Serialization
1. Forgetting to Implement Serializable
One of the first mistakes developers make is not implementing the Serializable
interface. If a class does not implement this interface, an attempt to serialize an instance of that class will throw a NotSerializableException
.
Avoid This Pitfall
Always ensure that all classes intended for serialization implement the Serializable
interface.
2. Non-Serializable Fields
Another frequent issue arises when a class contains non-serializable fields. If your class has references to non-serializable objects, the serialization process will fail.
Example Code Snippet
class NonSerializableClass {
// Some fields and methods
}
class User implements Serializable {
private NonSerializableClass nonSerializableField;
private String username;
public User(String username) {
this.username = username;
}
}
Why: Here, User
contains a field of a non-serializable type, which will cause serialization to fail. To resolve this, you can mark such fields as transient
.
Transient Fields
Marking a field as transient tells the Java serialization mechanism to ignore it during the process.
class User implements Serializable {
private static final long serialVersionUID = 1L; // Critical for versioning
private transient NonSerializableClass nonSerializableField; // Ignored during serialization
private String username;
// Other codes
}
3. Not Specifying serialVersionUID
Failing to define a serialVersionUID
can lead to compatibility issues between different versions of a class. The serialVersionUID
is a unique version identifier for Serializable classes.
Example Code Snippet
class User implements Serializable {
private static final long serialVersionUID = 1L;
private String username;
// Other attributes and methods
}
Why: By defining serialVersionUID
, you ensure that any changes made to the class over time won’t affect its serialization compatibility. If the identifier is not specified, the JVM generates one at runtime, which can vary based on the class’s structure.
4. Changing Class Fields After Serialization
When you modify the fields of a class after serialization, it can lead to issues during deserialization. The fields must maintain their consistency to ensure proper object reconstruction.
Example Code Snippet
class User implements Serializable {
private static final long serialVersionUID = 1L;
private String username;
private int age;
// User constructor, getters, and setters
}
If you reorder the fields or change their types and then try to deserialize an old serialized object, it might cause an InvalidClassException
.
Best Practice
Always maintain a version control mechanism. If you need to change the fields, update the serialVersionUID
.
5. Using Static Fields
Static fields belong to the class rather than any instance and are not serialized. Thus, using static fields from your class can lead to unexpected behavior upon deserialization.
Example Code Snippet
class User implements Serializable {
private static final long serialVersionUID = 1L;
private String username;
private static int userCount;
// User methods
}
Why: userCount
will not be saved or restored during serialization, leading to inconsistencies. If you rely on such fields, consider moving them outside serialized states to prevent loss of data.
6. Not Handling Exceptions Properly
Handling exceptions during serialization requires special care. Ignoring serialization exceptions can lead to silent data loss or corruption issues.
Example Code Snippet
try (ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream("user.ser"))) {
out.writeObject(user);
} catch (IOException e) {
e.printStackTrace();
}
Why: Always ensure proper exception handling during serialization and deserialization, which maintains data integrity and application stability.
7. Circular References
Circular references between serialized objects can lead to StackOverflowError
upon deserialization. Java's serialization handles circular references, but it still requires careful design to avoid complex situations.
Example Code Snippet
class User implements Serializable {
private static final long serialVersionUID = 1L;
private String username;
private Address address;
// Inner class for Address
}
class Address implements Serializable {
private static final long serialVersionUID = 1L;
private User user; // Circular reference
}
Why: Ensure that the design avoids unnecessary complexity, as it can complicate serialization. Implement careful control to limit circular references.
My Closing Thoughts on the Matter
Java serialization, when handled correctly, provides a robust avenue for data persistence and transmission. However, avoiding the discussed pitfalls is essential for ensuring that your application operates smoothly and efficiently.
By implementing best practices such as defining serialVersionUID
, managing transient fields, and being cautious about class modifications, you can significantly mitigate serialization issues.
For further reading, consider exploring the Java Serialization Documentation for deeper insights.
By consistently applying these strategies, you'll find your Java serialization endeavors much clearer, more manageable, and devoid of undesired surprises. Happy coding!
Checkout our other articles