Navigating Deadlocks in Java's Executor Framework
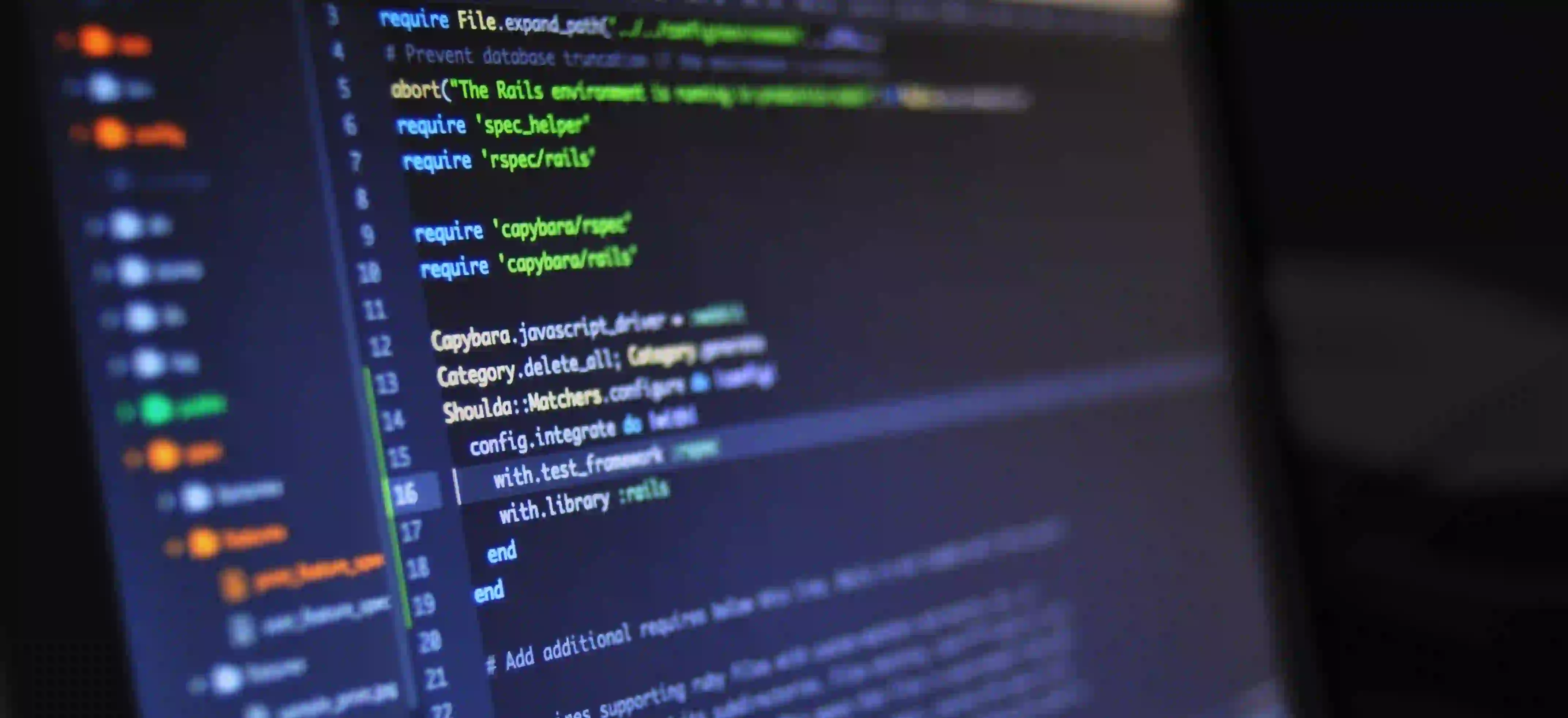
Navigating Deadlocks in Java's Executor Framework
In modern software development, concurrent programming is essential for building efficient applications. Java provides a remarkable toolset for achieving concurrency through its Executor Framework
. However, with concurrency comes the risk of deadlock situations, which can severely impact application performance.
In this blog post, we will delve into what deadlocks are, how they can occur in the Executor Framework
, and practical strategies for avoiding them.
Understanding Deadlocks
A deadlock occurs when two or more threads are blocked forever, waiting for each other to release a resource. In a typical deadlock scenario, Thread A holds Resource 1 and waits for Resource 2, while Thread B holds Resource 2 and waits for Resource 1. This mutual waiting leads to an impasse, resulting in application hang-ups.
Common Symptoms of Deadlocks:
- Thread states are stuck in a waiting state.
- Application responsiveness decreases significantly.
- Unhandled exceptions or timeouts occur when waiting for threads.
The Java Executor Framework: A Brief Overview
The Executor Framework
is part of Java's concurrency utilities and simplifies thread management.
Key Components:
- Executor: An interface for object that executes submitted tasks.
- Executors: A factory class for creating different types of
Executor
instances. - ExecutorService: A subinterface of
Executor
that can manage the lifecycle of tasks and provide additional methods for handling futures, shutdowns, etc.
Basic Usage Example
Here’s a simple code snippet that illustrates how to use an ExecutorService
to run tasks concurrently:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ExecutorExample {
public static void main(String[] args) {
ExecutorService executorService = Executors.newFixedThreadPool(2);
executorService.submit(() -> {
System.out.println("Task 1 is running");
});
executorService.submit(() -> {
System.out.println("Task 2 is running");
});
executorService.shutdown();
}
}
In the above example, we create a fixed thread pool and submit two tasks. They will run concurrently, showcasing how easy it is to manage threads with the Executor Framework
.
How Deadlocks Can Occur in Executor Framework
Deadlocks are more likely to happen when tasks submit additional tasks to Executors without proper management. The situation gets trickier in complex applications where multiple threads interact with shared resources.
Situational Example of Deadlock:
Consider two tasks that depend on each other:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class DeadlockExample {
public static void main(String[] args) {
ExecutorService executorService = Executors.newFixedThreadPool(2);
executorService.submit(() -> {
synchronized (Lock1.class) {
System.out.println("Thread 1 acquired Lock1");
try {
// Simulating processing
Thread.sleep(100);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
synchronized (Lock2.class) {
System.out.println("Thread 1 acquired Lock2");
}
}
});
executorService.submit(() -> {
synchronized (Lock2.class) {
System.out.println("Thread 2 acquired Lock2");
try {
// Simulating processing
Thread.sleep(100);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
synchronized (Lock1.class) {
System.out.println("Thread 2 acquired Lock1");
}
}
});
executorService.shutdown();
}
}
Code Explanation
- Thread 1 locks
Lock1
and tries to acquireLock2
. - Thread 2 locks
Lock2
and tries to acquireLock1
. - Both threads end up waiting indefinitely for each other, creating a deadlock.
Identifying Deadlocks
Monitoring tools like the Java VisualVM or Java Mission Control can help identify deadlocks in running applications by visualizing thread states.
Strategies to Avoid Deadlocks
Preventing deadlocks is typically a combination of design considerations, appropriate practices, and careful coding strategies.
1. Lock Ordering
One effective method to avoid deadlocks is to acquire locks in a consistent global order. By defining a lock acquisition hierarchy, it ensures that no matter how threads interact, they will follow the same path, thus avoiding circular wait conditions.
2. Timeouts for Locks
By implementing timeouts, we can ensure that if a thread cannot acquire a lock within a certain period, it will back off and possibly retry, thus preventing a full deadlock from occurring.
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class TimeoutExample {
private static final Lock lock1 = new ReentrantLock();
private static final Lock lock2 = new ReentrantLock();
public static void main(String[] args) {
Thread thread1 = new Thread(() -> acquireLocks(lock1, lock2));
Thread thread2 = new Thread(() -> acquireLocks(lock2, lock1));
thread1.start();
thread2.start();
}
private static void acquireLocks(Lock firstLock, Lock secondLock) {
boolean gotFirstLock = false;
boolean gotSecondLock = false;
try {
while (!gotFirstLock || !gotSecondLock) {
gotFirstLock = firstLock.tryLock();
gotSecondLock = secondLock.tryLock();
if (gotFirstLock && gotSecondLock) {
System.out.println(Thread.currentThread().getName() + " acquired both locks");
} else {
if (gotFirstLock) {
firstLock.unlock();
}
if (gotSecondLock) {
secondLock.unlock();
}
}
// Try again after a short delay
try {
Thread.sleep(50);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
} finally {
if (gotFirstLock) {
firstLock.unlock();
}
if (gotSecondLock) {
secondLock.unlock();
}
}
}
}
3. Use Higher-Level Structures
Using higher-level concurrency utilities like java.util.concurrent.locks
or java.util.concurrent
collections can often lead to better management of resources and fewer opportunities for deadlocks.
4. Review Thread Context
Be mindful of how threads interact and share resources. Doing a thorough review and profiling of the thread context during development can help in catching potential deadlock scenarios before they become issues in production.
Key Takeaways
Navigating deadlocks in Java’s Executor Framework
demands a clear understanding of concurrency processes and practice. While we have covered fundamental strategies to minimize deadlocks, continual learning and monitoring are paramount.
For further reading and deeper insights into Java concurrency mechanisms, you may want to explore the Java Concurrency Tutorial and the Official Java Documentation on java.util.concurrent.
By applying best practices and maintaining vigilance in your design and code, your Java applications can mitigate the risks of deadlocks and enhance their overall efficiency and responsiveness. Happy coding!