Understanding Java Memory Leaks and How to Fix Them
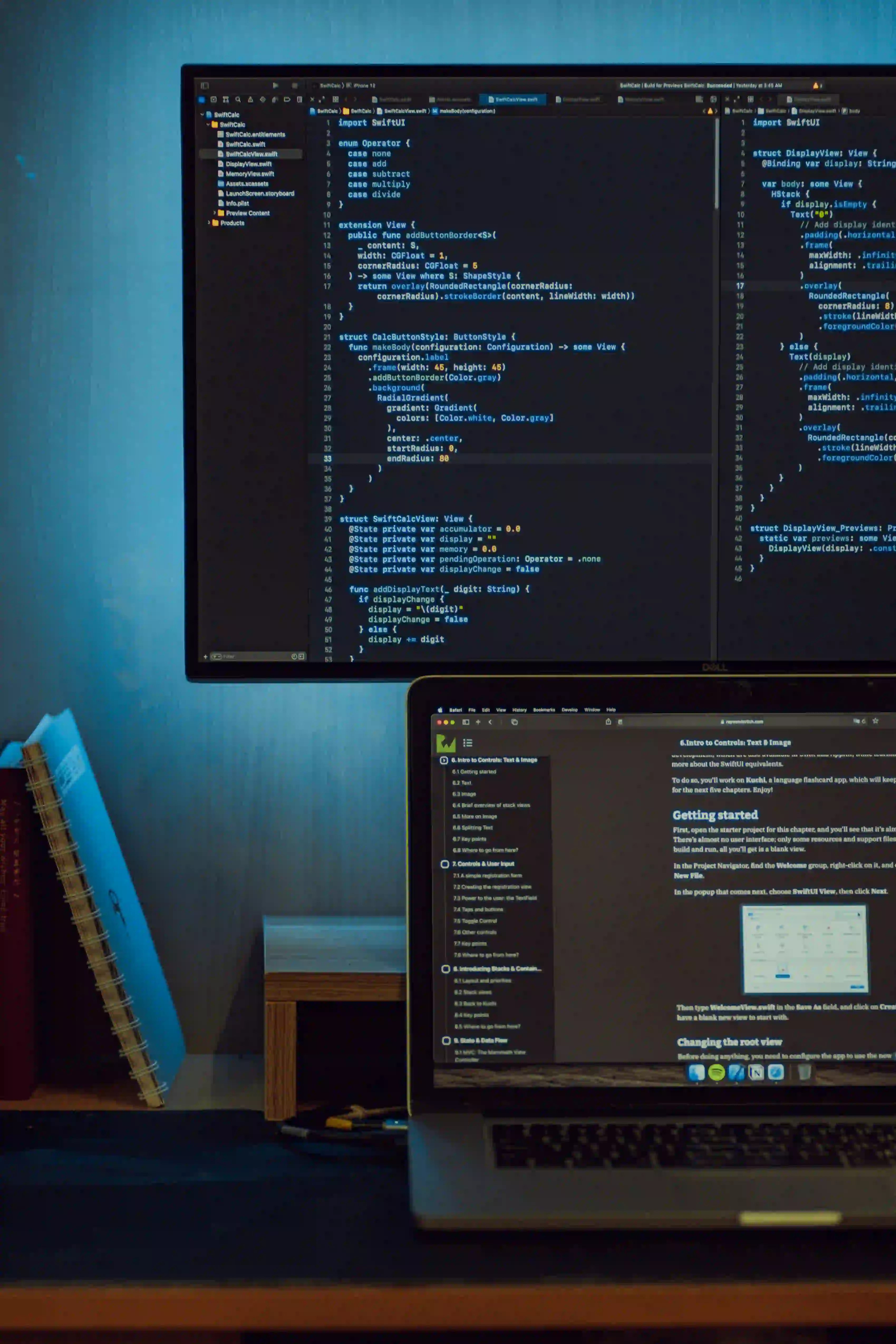
Understanding Java Memory Leaks and How to Fix Them
Java is a powerful programming language that simplifies memory management through garbage collection. However, this does not make it immune to memory leaks. In this blog post, we'll explore what a memory leak is, how to identify it, and practical strategies to fix and prevent memory leaks in Java applications.
Table of Contents
- What is a Memory Leak?
- Why Memory Leaks are Dangerous
- Identifying Memory Leaks
- Common Causes of Memory Leaks in Java
- Strategies to Fix Memory Leaks
- Preventing Future Memory Leaks
- Conclusion
What is a Memory Leak?
In simple terms, a memory leak occurs when a program unintentionally retains references to objects that are no longer needed, preventing the Java garbage collector from reclaiming that memory. As the application runs and allocates memory, the uncollected objects accumulate, which can lead to increased memory usage, slower performance, and eventually an OutOfMemoryError
.
Why Memory Leaks are Dangerous
Memory leaks can cause serious issues in production applications, including:
- Increased Memory Usage: As unused objects pile up, they consume valuable resources.
- Reduced Performance: Applications may slow down due to excessive garbage collection cycles.
- Application Crashes: Ultimately, memory leaks can lead to application failures, impacting user experience and potentially leading to data loss.
Identifying Memory Leaks
Detecting memory leaks in Java can be tricky, but several tools and methodologies can help. Some reliable ones include:
- Java VisualVM: A powerful profiling tool that comes bundled with the JDK. It enables monitoring of memory usage and identifying leaking objects.
- Eclipse Memory Analyzer Tool (MAT): A dedicated tool for analyzing memory dumps. It can detect memory leaks and provide a detailed report of memory usage.
- Heap Dumps: You can generate a heap dump using a command like
jmap -dump:live,format=b,file=heapdump.hprof <pid>
. Analyzing these dumps can show which objects are consuming the most memory.
To illustrate heap dump analysis, consider using the following snippet in your code to create a heap dump under low memory conditions:
import java.lang.management.ManagementFactory;
import java.lang.management.MemoryMXBean;
public class HeapDumpExample {
public static void generateHeapDump() {
MemoryMXBean memoryMXBean = ManagementFactory.getMemoryMXBean();
long usedHeapMemory = memoryMXBean.getHeapMemoryUsage().getUsed();
long maxHeapMemory = memoryMXBean.getHeapMemoryUsage().getMax();
if (usedHeapMemory > 0.75 * maxHeapMemory) {
System.out.println("Heap memory usage is high! Generating heap dump...");
// Code to trigger heap dump
}
}
}
This method checks the memory usage and triggers a heap dump when the usage exceeds 75% of maximum heap memory. For more detailed guidance, consider visiting Oracle's Java Garbage Collection documentation.
Common Causes of Memory Leaks in Java
1. Unfinished Threads
When a thread is started and not properly closed, it can hold onto memory resources indefinitely. A common culprit is non-daemon threads that are still running when the application is shutting down.
public class ThreadExample {
private Thread backgroundThread;
public void startThread() {
backgroundThread = new Thread(() -> {
// Thread processing
});
backgroundThread.start();
}
public void stopThread() {
// Logic to stop the thread properly
}
}
Ensure to provide a mechanism for stopping the thread gracefully.
2. Static References
Using static references can retain object references longer than needed. This is a common mistake when caching objects or using singleton patterns.
public class Singleton {
private static final Map<String, Object> cache = new HashMap<>();
public static Object getFromCache(String key) {
return cache.get(key);
}
public static void addToCache(String key, Object value) {
cache.put(key, value);
}
}
While static caches can be useful, ensure to implement a strategy for cache eviction or cleanup to help manage memory effectively.
3. Listener and Callback Issues
Registering listeners or callbacks without deregistering them can lead to strong references that prevent garbage collection.
public class EventSource {
private final List<EventListener> listeners = new ArrayList<>();
public void registerListener(EventListener listener) {
listeners.add(listener);
}
public void deregisterListener(EventListener listener) {
listeners.remove(listener);
}
}
Always ensure that you deregister listeners when they are no longer needed.
Strategies to Fix Memory Leaks
1. Heap Dump Analysis
Once a memory leak is suspected, generate a heap dump and analyze it to track down leaking objects. Look for unusually high counts of specific classes holding references to memory.
2. Use Weak References
In cases where you want to cache objects without preventing garbage collection, use WeakReference
or SoftReference
. This allows the garbage collector to reclaim memory as needed.
import java.lang.ref.WeakReference;
public class Cache {
private WeakReference<Object> cachedObject;
public void cacheObject(Object obj) {
cachedObject = new WeakReference<>(obj);
}
public Object getCachedObject() {
return cachedObject == null ? null : cachedObject.get();
}
}
3. Implement Proper Cleanup Methods
When utilizing resources, always provide methods for cleanup. This is crucial for avoiding leaks from resources like database connections or file handlers.
public class ResourceHandler {
private Connection connection;
public void openConnection() {
connection = /* Code to open connection */;
}
public void closeConnection() {
if (connection != null) {
connection.close();
connection = null;
}
}
}
Preventing Future Memory Leaks
- Code Reviews: Implement regular code reviews to catch potential leaks early.
- Memory Profiling: Regularly profile your application during development to detect and fix leaks.
- Weak References: Use weak references judiciously when caching objects, especially in event-driven applications.
- Framework Best Practices: Follow the recommendations of frameworks that your application uses, as they often have patterns to avoid memory leaks.
Wrapping Up
Memory leaks in Java can significantly degrade application performance and reliability. Understanding their causes and knowing how to fix them is essential for any Java developer. By implementing effective monitoring tools, following best practices, and ensuring proper cleanup, you can safeguard your applications against memory leaks.
For further reading, visit Java Memory Management to gain deeper insights into Java memory management techniques that can help you optimize your code and prevent memory issues.
By maintaining vigilance and employing these strategies, you'll be well-equipped to manage memory more effectively in your Java applications. Happy coding!