Unraveling Java Reflection: Common Pitfalls and Fixes
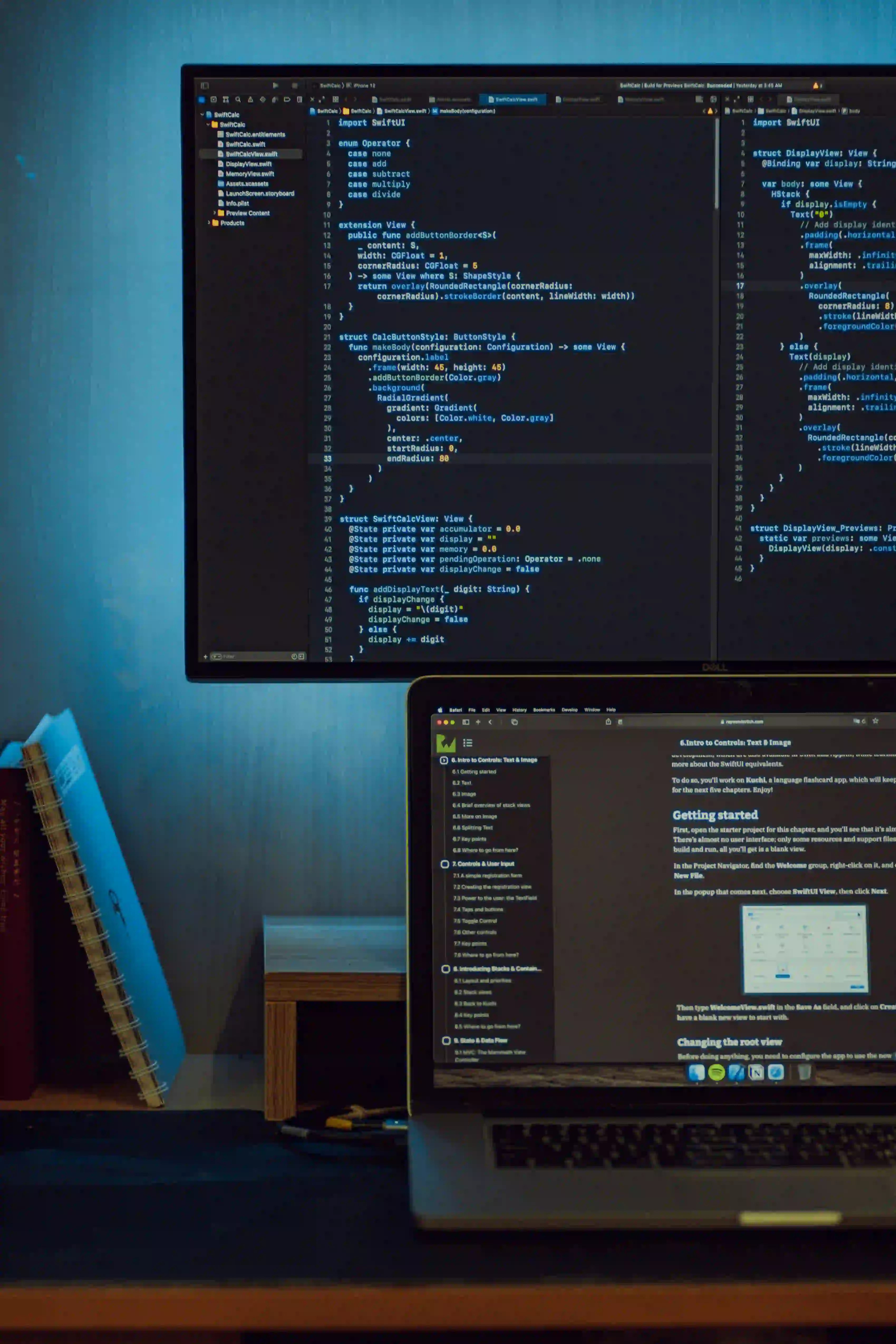
Unraveling Java Reflection: Common Pitfalls and Fixes
Java Reflection is a powerful feature that allows developers to inspect and manipulate classes, methods, fields, and other objects at runtime. While it offers a great deal of flexibility, it also introduces several complexities and potential pitfalls. In this blog post, we'll explore some of the common pitfalls associated with Java Reflection, along with practical fixes and preventive strategies.
Table of Contents
What is Java Reflection?
Java Reflection is part of the java.lang.reflect
package, allowing you to examine classes, interfaces, fields, and methods at runtime, without knowing the names of the classes or methods beforehand. This is beneficial for scenarios such as:
- Object serialization
- Dependency injection frameworks
- ORM frameworks like Hibernate
To get started with reflection, consider the following example:
Class<?> clazz = Class.forName("java.lang.String");
Method[] methods = clazz.getDeclaredMethods();
for (Method method : methods) {
System.out.println(method.getName());
}
In this code, we dynamically load the String
class and print all its methods. This is just a glimpse of the capabilities Java Reflection offers.
Common Pitfalls in Java Reflection
Despite its advantages, Java Reflection can lead to several issues if not used judiciously.
Performance Issues
One of the most notable drawbacks of using reflection is performance overhead. Reflective operations are inherently slower than direct access. This can impact the application, especially if reflection is used in critical performance paths.
Fix: Cache reflective resources. For example, cache Method or Constructor instances after retrieving them once:
Map<String, Method> methodCache = new HashMap<>();
Method method = methodCache.computeIfAbsent("myMethod", key -> {
try {
return MyClass.class.getMethod(key);
} catch (NoSuchMethodException e) {
throw new RuntimeException(e);
}
});
Accessibility Concerns
Reflection can access private members of classes, which can expose your application to unintended side effects. Frequently, the use of reflection leads to manipulating private fields or methods.
Fix: Be cautious and limit access. Consider using public methods or constructors whenever possible. Here's how to access a private field safely:
Field field = MyClass.class.getDeclaredField("privateField");
field.setAccessible(true);
Object value = field.get(myObject);
While setAccessible(true)
allows access, it should be used sparingly.
Type Safety
Reflection bypasses compile-time type checking, leading to potential ClassCastException
at runtime.
Fix: Use generics wherever possible. If you know the exact types you are dealing with, explicitly define them. Here is a safer approach:
Field field = MyClass.class.getDeclaredField("myField");
field.setAccessible(true);
if (field.getType().equals(String.class)) {
String value = (String) field.get(myObject);
}
This check ensures you are only casting to a String when it is appropriate.
Security Manager Restrictions
Java programs operated under a security manager can experience potential restrictions when using reflection. Certain operations might throw a SecurityException
if the security manager does not permit them.
Fix: If you're working in an environment with a security manager, check the permissions required for reflective operations:
if (System.getSecurityManager() != null) {
// Check permissions here
}
Always design your applications knowing they may run under restrictive environments.
Code Maintenance and Readability
Code that relies heavily on reflection can become complex and challenging to maintain. This can make it difficult for developers unfamiliar with the codebase to understand its functionality.
Fix: Use reflection judiciously. If specific tasks can be achieved without it, prefer standard Java object-oriented programming practices. Document any complex reflective code with ample comments to improve readability.
Here’s an example of complex reflection code that could be avoided:
Method method = MyClass.class.getDeclaredMethod("doSomething", String.class);
method.invoke(myObject, "test");
Instead of reflecting on methods, consider using interfaces or design patterns such as Strategy or Factory patterns for more maintainable solutions.
Best Practices and Fixes
-
Limit Reflection Use: Use reflection in moderation. It is a tool best suited for specific scenarios such as testing and frameworks.
-
Prefer Direct Access: If possible, access fields and methods directly rather than through reflection. Always prefer compile-time checks over runtime checks when available.
-
Keep It Local: Encapsulate reflective code as much as possible. This makes it easier to isolate and test.
-
Consider Alternatives: Investigate whether libraries like Spring Framework or Project Lombok can fulfill your needs without resorting to reflection.
-
Performance Measurements: If performance is critical, always measure the impact of reflection in your specific use cases.
Bringing It All Together
Java Reflection is an essential tool for advanced Java developers. Understanding its potential pitfalls is crucial for building robust, maintainable applications. Whether addressing performance issues, maintaining security, or ensuring code quality, reflective programming requires a thoughtful approach. Always balance flexibility with the principles of clean coding and maintainability.
Have you navigated pitfalls while using Java Reflection? Share your experiences in the comments below!
By embracing the power of Java Reflection judiciously, developers can effectively harness its capabilities while safeguarding the integrity and performance of their applications. For further reading, explore the official Java Reflection Documentation.