Mastering Java Annotations: Common Missteps to Avoid
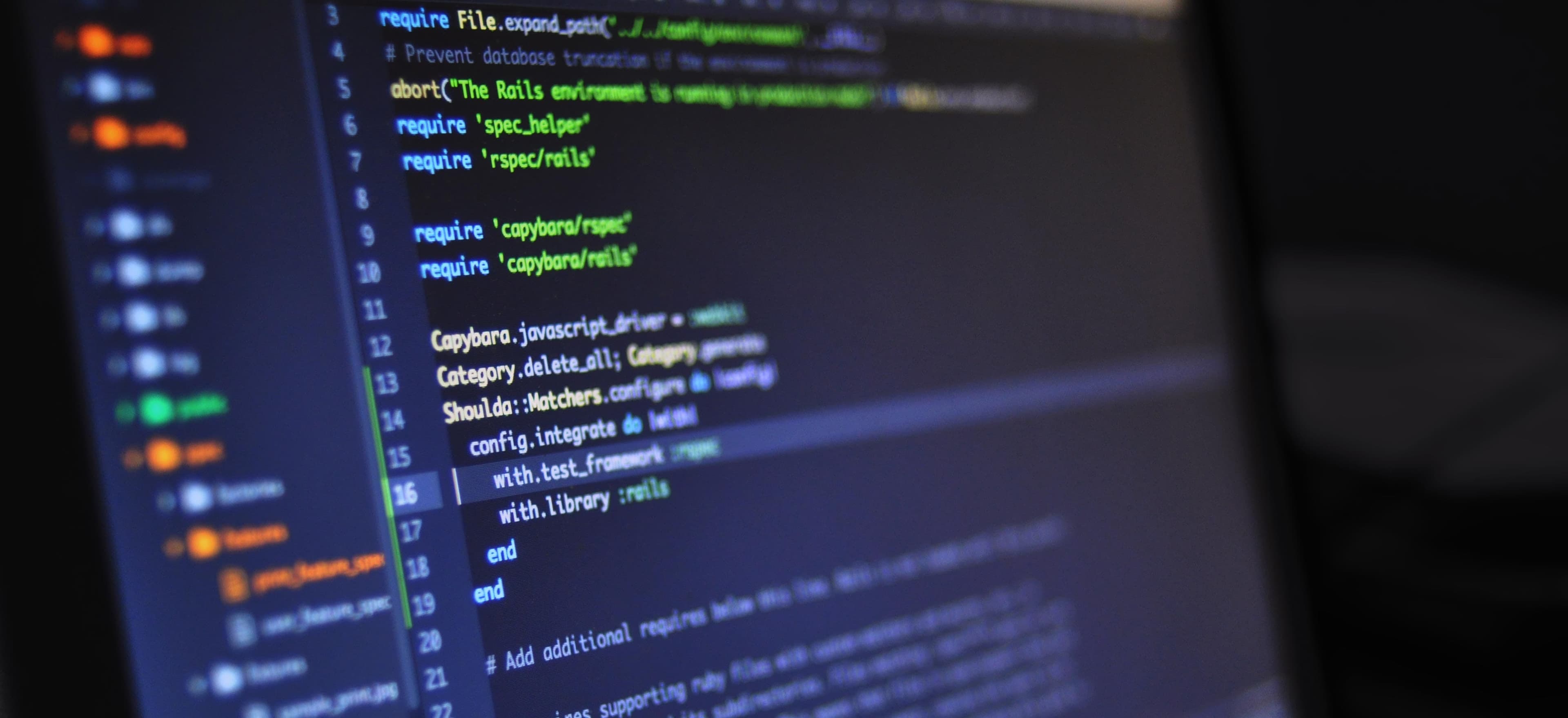
- Published on
Mastering Java Annotations: Common Missteps to Avoid
Java annotations offer a powerful way to add metadata to Java code. They can influence program behavior and provide critical information for both the compiler and runtime environments. However, despite their advantages, developers often encounter pitfalls when using them. This blog post covers common mistakes to avoid while mastering Java annotations, ensuring that you harness their full potential effectively.
Understanding Java Annotations
Java annotations are essentially tags that provide data about a program but have no direct effect on the operation of the code they annotate. They can be classified in several ways:
- Marker Annotations: No elements. Example:
@Override
. - Single Element Annotations: One element. Example:
@SuppressWarnings("unchecked")
. - Full Annotations: Multiple elements in the form of key-value pairs. Example:
@MyAnnotation(name="Example", value=123)
Annotations can serve various purposes—from supplying metadata to improving code readability and providing compile-time checks. However, to utilize Java annotations effectively, one must navigate around common pitfalls.
Common Missteps to Avoid
1. Incorrect Annotation Target
Mistake: Applying an annotation outside its intended context.
Java annotations are bound to specific targets, such as classes, methods, fields, and parameters. Attempting to apply an annotation against its designated target can lead to runtime errors or compiler complaints.
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD) // This annotation can only be used on methods
@interface MyMethodAnnotation {
String value();
}
// Incorrect application of the annotation
@MyMethodAnnotation(value = "This will throw an error")
class MyClass {
@MyMethodAnnotation(value = "Correct usage") // Correct application
public void myMethod() {
}
}
Tip: Clearly understand the @Target
of your annotations. Always refer to the Javadocs for reference.
Java Documentation provides detailed information regarding annotation usage based on their target.
2. Misunderstanding Retention Policy
Mistake: Ignoring the retention policy of annotations.
The retention policy determines whether annotations are discarded by the compiler or retained during runtime:
SOURCE
: Annotations are only available in the source code.CLASS
: Annotations are available in the class file but not during runtime.RUNTIME
: Annotations are available at runtime.
Many developers mistakenly expect annotations to be available at runtime without specifying RetentionPolicy.RUNTIME
.
@Retention(RetentionPolicy.RUNTIME)
@interface RuntimeAnnotation {}
@Retention(RetentionPolicy.CLASS)
@interface ClassAnnotation {}
In this example, RuntimeAnnotation
can be retrieved via reflection, while ClassAnnotation
cannot during runtime.
Tip: Use @Retention(RetentionPolicy.RUNTIME)
if you intend to access the annotations during the execution of the program.
3. Overusing Annotations
Mistake: Excessive use of annotations can lead to code bloat.
While annotations can improve readability, overusing them may create ambiguity in code, making it hard to understand. Annotations are useful for clarifying behavior, but like the adage says, too much of a good thing can be detrimental.
@Deprecated
@MyMethodAnnotation(value = "Don't use this!")
@AnotherAnnotation
public void oldMethod() {
// method implementation
}
Tip: Use only those annotations necessary for your implementation. Always aim for clarity. Discuss with your team before adding any additional annotations.
4. Ignoring Annotations in Inheritance
Mistake: Failing to consider how annotations behave in subclasses.
Annotations on the superclass may not inherently propagate to subclass methods. If you annotate a method in a superclass, be cautious—the annotation may not apply to overridden methods in subclasses.
class SuperClass {
@MyMethodAnnotation(value = "SuperClass method")
public void display() {}
}
class SubClass extends SuperClass {
@Override
public void display() {
// Annotation is technically not inherited
}
}
Tip: If you want an annotation to apply to subclasses, explicitly reapply the same annotation.
5. Failing to Use Built-in Annotations
Mistake: Reinventing the wheel by creating custom annotations.
Java comes equipped with a wealth of built-in annotations—like @Override
, @Deprecated
, and @SuppressWarnings
. Developers can sometimes be tempted to create their annotations for scenarios that are perfectly handled by built-in counterparts.
// Unnecessary custom annotation
@interface CustomDeprecated {
String message();
}
// Instead of
@CustomDeprecated(message="Use newMethod instead.")
public void oldMethod() {}
// Use:
@Deprecated
public void oldMethod() {}
Tip: Leverage existing annotations to keep your code cleaner and easier to maintain.
6. Not Leveraging Spring Annotations
Mistake: Overlooking the use of Spring's powerful annotations.
For those developing with the Spring framework, neglecting to utilize its rich set of annotations can lead to boilerplate code that becomes unwieldy. Without annotations, you may revert to XML configuration, which can be cumbersome and less readable.
@Service
public class MyService {
@Autowired // Automatically injects the repository
private MyRepository repository;
}
Tip: Familiarize yourself with Spring’s annotations such as @Service
, @Controller
, and @Component
to reduce configuration hassle.
For more insights, refer to the Spring Framework Documentation.
7. Neglecting Error Handling
Mistake: Failing to validate annotations.
When using annotations that accept parameters, be careful to validate these inputs. Non-validated parameters can lead to unexpected runtime errors.
@Retention(RetentionPolicy.RUNTIME)
@interface ValidatedAnnotation {
int value() default 1;
}
@ValidatedAnnotation(value = -5) // Should validate that the value is >= 1
public void myValidatedMethod() {}
Tip: Implement logic to check the validity of annotation parameters during your application startup or through custom annotations.
My Closing Thoughts on the Matter
Java annotations are a powerful tool for improving code quality and ensuring better maintainability. By avoiding the common pitfalls outlined in this article, you can harness their potential effectively. Understanding the correct context for applying them, knowing how to deal with retention policies, and leveraging existing features can vastly improve your Java development process.
Mastering Java annotations is a journey, but with these insights, you can avoid missteps and make the most of this feature. Don’t forget to dig deeper into Java's documentation and best practices to keep advancing your skills.
Feel free to leave comments or questions below! Happy coding!