Mastering Java 8's Date-Time API: Common Pitfalls to Avoid
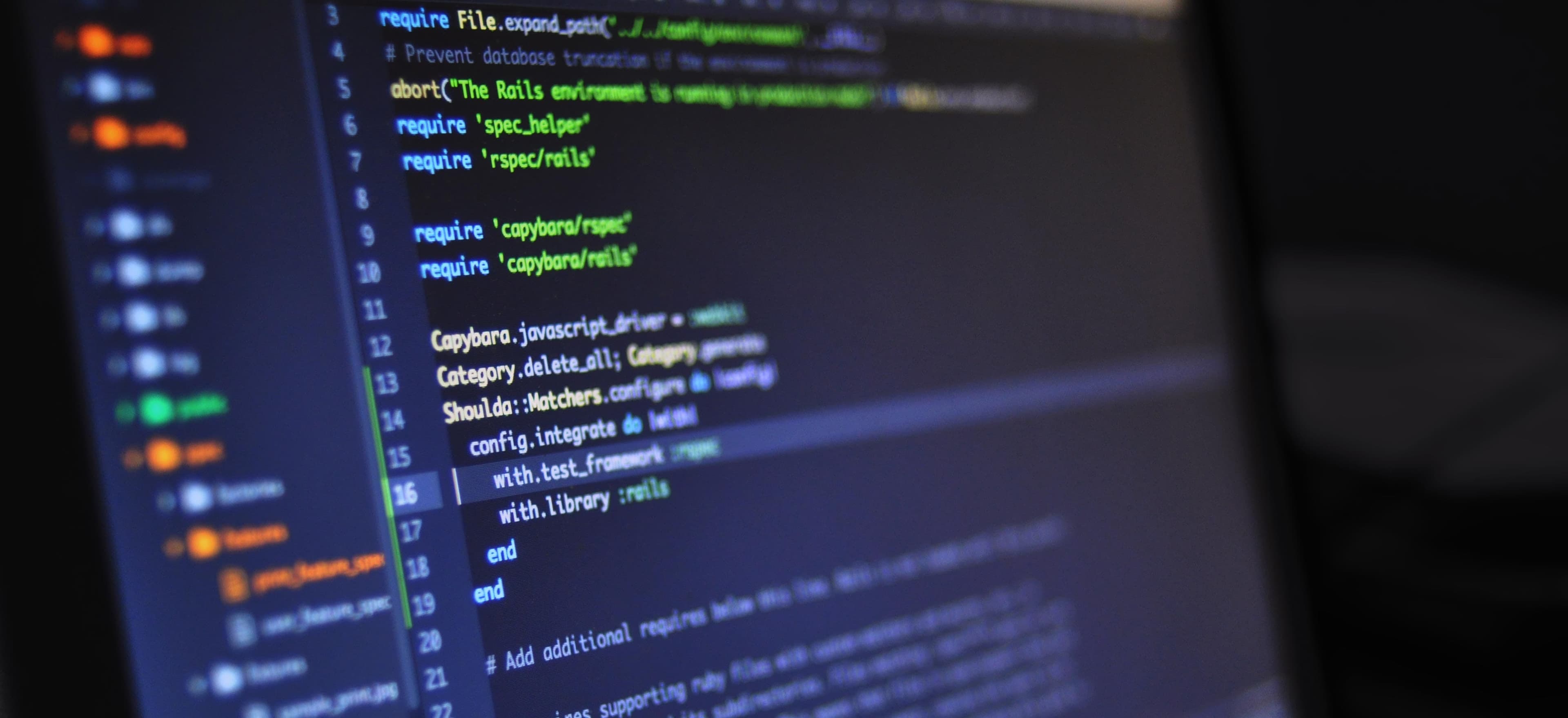
- Published on
Mastering Java 8's Date-Time API: Common Pitfalls to Avoid
Java 8 introduced a powerful and comprehensive Date-Time API that addresses many of the flaws present in the previous Date and Calendar classes. This modern API brings immutability and thread-safety, making it more reliable for developers. However, transitioning to this new API can come with its challenges. In this blog post, we will explore some common pitfalls of Java 8's Date-Time API and how to avoid them, ensuring you harness its full potential.
Why the New API?
Before diving into the pitfalls, let's briefly discuss why Java 8 introduced the Date-Time API.
-
Legacy Issues: The old
java.util.Date
andjava.util.Calendar
classes were mutable, often leading to confusing bugs when the same instances were shared across threads. -
Fluent and Clear Syntax: The new API is designed with a more understandable and fluent interface, making it easier to work with dates and times.
-
Time Zones Management: Handling time zones is now more convenient and less error-prone with classes like
ZonedDateTime
.
For further reading on Date-Time API's design philosophy, check out the Java Docs.
Common Pitfalls
Pitfall 1: Using LocalDate Instead of ZonedDateTime
One of the most common mistakes is to use LocalDate
or LocalTime
when time zone information is crucial.
LocalDate localDate = LocalDate.now(); // Retrieves the current date
Why This is a Problem: If your application globally interacts with users in different time zones, using LocalDate
strips away critical time zone information.
Solution: Opt for ZonedDateTime
when dealing with date and time that requires the context of a specific zone.
ZonedDateTime zonedDateTime = ZonedDateTime.now(); // Better for time zones
Pitfall 2: Forgetting to Handle DST Changes
Daylight Saving Time (DST) adjustments can lead to unexpected results if not accounted for properly.
ZoneId zoneId = ZoneId.of("America/New_York");
ZonedDateTime winterDate = ZonedDateTime.of(2023, 1, 1, 12, 0, 0, 0, zoneId); // Winter
ZonedDateTime summerDate = ZonedDateTime.of(2023, 7, 1, 12, 0, 0, 0, zoneId); // Summer
Potential Pitfall: Performing calculations across DST transitions can produce incorrect results.
Solution: Always be mindful of DST. You can check if a ZonedDateTime
instance is in DST by utilizing the getOffset()
method.
ZoneOffset offset = winterDate.getOffset(); // Uses standard time offset.
ZoneOffset offsetDST = summerDate.getOffset(); // Updates to DST offset.
Pitfall 3: Using Implicit Conversions
When using different temporal types, avoid implicit conversions that can lead to bugs.
Instant instant = Instant.now();
LocalDateTime dateTime = LocalDateTime.ofInstant(instant, ZoneOffset.UTC); // Safe conversion
Why This is Important: Implicit conversions don't always act as one might expect, especially if they're combined with different types. Explicitly handling conversions is vital.
Best Practice: Use explicit method calls such as ofInstant
with the appropriate ZoneOffset
to ensure safe and predictable results.
Pitfall 4: Ignoring the Immutability
The Java 8 Date-Time API classes are immutable. However, many developers new to Java 8 may still try to modify the instances.
LocalDate originalDate = LocalDate.of(2023, 3, 28);
LocalDate newDate = originalDate.plusDays(5); // Correct use, returns a new instance
Common Mistake: Trying to modify originalDate
directly.
Solution: Always remember that methods like plusDays
, minusDays
, etc., return new instances. Mutating the existing object will not work.
Pitfall 5: Misunderstanding Between LocalDate, LocalDateTime, and ZonedDateTime
Each of these classes serves a purpose, yet new developers might not understand the depth of the differences.
- LocalDate: Represents a date without a time zone.
- LocalDateTime: Represents a date-time without a time zone.
- ZonedDateTime: Represents a full date-time with time zone.
LocalDate date = LocalDate.now();
LocalDateTime dateTime = LocalDateTime.now();
ZonedDateTime zonedDateTime = ZonedDateTime.now();
Why This Matters: Mixing these types can lead to incorrect assumptions about what time-related data you’re working with.
Tip: Use ZonedDateTime
when time zones matter. For calculations or when you don't need a time zone, stick with LocalDateTime
or LocalDate
.
Pitfall 6: Failure to Handle Exceptions Properly
Parsing strings into date-time objects often leads to DateTimeParseException
.
String dateStr = "2023-03-28T10:15:30";
LocalDateTime parsedDate = LocalDateTime.parse(dateStr); // Parsing might throw an exception
Solution: Always handle exceptions when parsing to prevent runtime crashes.
try {
LocalDateTime parsedDate = LocalDateTime.parse(dateStr);
} catch (DateTimeParseException e) {
System.out.println("Unable to parse date: " + e.getMessage());
}
Key Takeaways
By recognizing and avoiding these common pitfalls, you can ensure that your use of Java 8's Date-Time API is both accurate and efficient. Embrace the immutability and thread-safety that this API offers, and you'll be well on your way to mastering date and time operations in Java.
For those looking for further insights and advanced usage scenarios, consider checking out more detailed resources such as Baeldung's Java 8 Date-Time API Guide for additional tutorials and scenarios.
With the right understanding and practices, Java 8’s Date-Time API can significantly simplify date and time management in your applications, providing both clarity and reliability. Happy coding!