Mastering Functional Interfaces: Avoiding Common Pitfalls
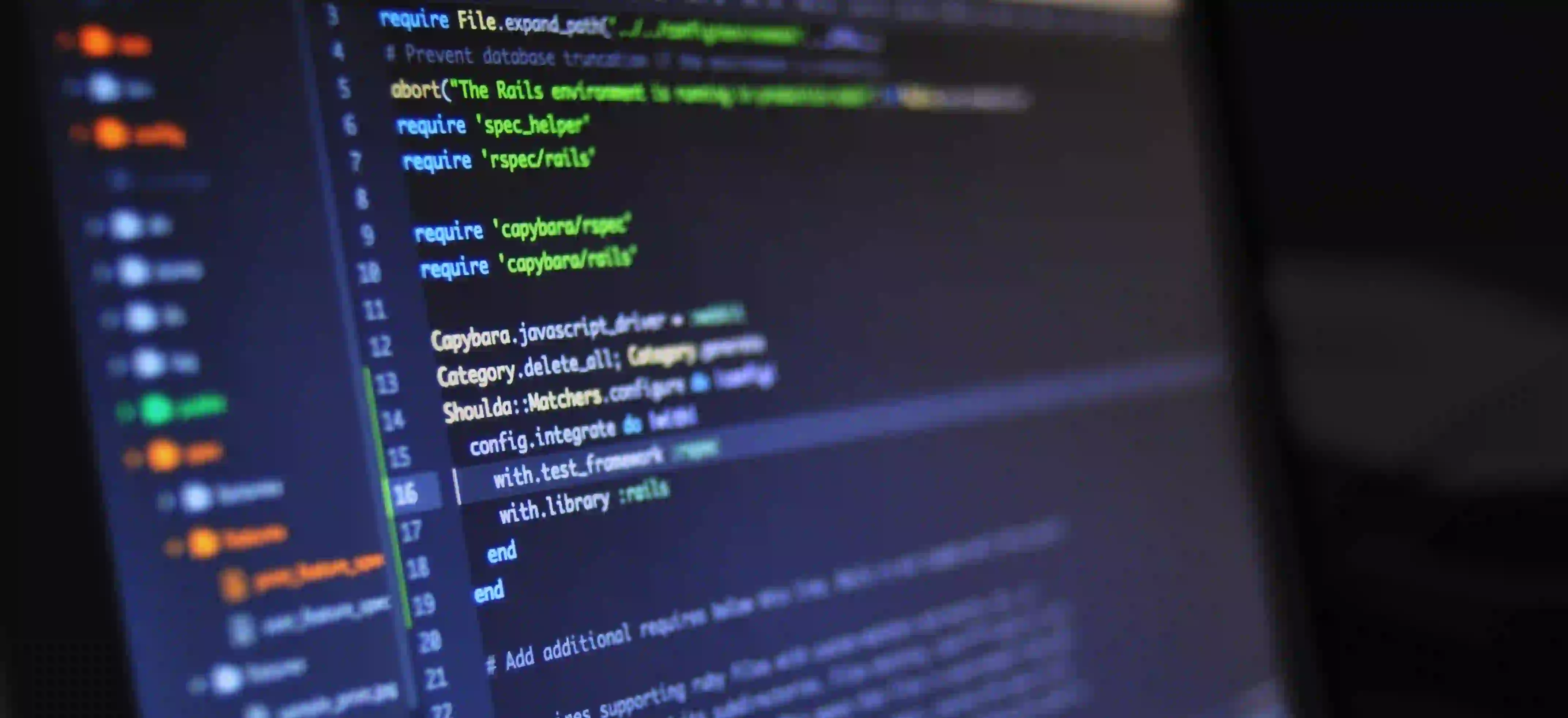
Mastering Functional Interfaces: Avoiding Common Pitfalls
Functional programming has surged in popularity due to its ability to simplify complex tasks and improve code maintainability. In Java, functional interfaces serve as the backbone of functional programming paradigms, primarily introduced in Java 8. This blog post will delve into the fundamentals of functional interfaces, illuminate common pitfalls, and provide practical solutions. Let's embark on this journey to master functional interfaces in Java!
What Are Functional Interfaces?
In Java, a functional interface is an interface that contains exactly one abstract method. They can have multiple default or static methods but only one abstract method, making them suitable for Lambda expressions or method references. This definition aligns with the philosophy of functional programming—concise, reusable code that focuses on functions.
Example of a Functional Interface
Here's an example of a simple functional interface:
@FunctionalInterface
public interface Greeting {
void sayHello(String name);
}
In this interface, sayHello
is the single abstract method. You can implement this interface using a Lambda expression, as shown below:
public class Main {
public static void main(String[] args) {
Greeting greeting = (name) -> System.out.println("Hello, " + name + "!");
greeting.sayHello("World");
}
}
Why Use Functional Interfaces?
Functional interfaces promote clear and concise code. They enable the implementation of higher-order functions—functions that can accept other functions as parameters or return them as results—thus streamlining processes such as sorting, filtering, and mapping data collections.
Common Pitfalls When Working with Functional Interfaces
Pitfall 1: Not Using the @FunctionalInterface Annotation
While it's not mandatory, it’s a best practice to use the @FunctionalInterface
annotation. This annotation signals the intention of the interface to be functional. Failing to do so might lead to confusion, and worse, it could lead to runtime errors if you accidentally add additional abstract methods.
Example Without @FunctionalInterface
public interface Action {
void perform();
void execute(); // This will cause a compilation error
}
Using the annotation clearly communicates intent.
Pitfall 2: Confusing Functional Interfaces with Regular Interfaces
A common misconception is to treat functional interfaces as regular interfaces just because they can have default methods. Remember, functional interfaces are designed to facilitate functional programming, and overcomplicating them can defeat their purpose.
Example of Overcomplication
@FunctionalInterface
public interface Calculator {
int calculate(int a, int b);
default double squareRoot(int a) {
return Math.sqrt(a);
}
default double cubeRoot(int a) {
return Math.cbrt(a);
}
}
While it is allowed, avoid extending the functional interface with too many default methods. Instead, create separate utility classes.
Pitfall 3: Ignoring Type Inference
Java's type inference allows you to omit the type declaration in Lambda expressions. However, overlooking the target type can lead to unexpected errors.
Correct Usage
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println(name)); // Correct usage
Pitfall 4: Not Leveraging Built-in Functional Interfaces
Java provides several built-in functional interfaces, such as Predicate
, Consumer
, Function
, and Supplier
. However, many developers fall into the trap of reinventing the wheel by creating their own functional interfaces.
Example of Use Cases
Instead of defining your own IntFilter
:
@FunctionalInterface
public interface IntFilter {
boolean filter(int value);
}
Use a Predicate<Integer>
from the Java standard library.
Predicate<Integer> isEven = x -> x % 2 == 0;
You'll find this approach saves time and improves code readability.
Practical Usage: Cases Where Functional Interfaces Shine
1. Stream Processing
Functional interfaces shine in the Java Stream API. They simplify transformations, filtering, and collecting data.
Example of Using Streams
import java.util.List;
import java.util.stream.Collectors;
public class Example {
public static void main(String[] args) {
List<String> names = List.of("Alice", "Bob", "Charlie", "David");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("C"))
.collect(Collectors.toList());
System.out.println(filteredNames); // Outputs: [Charlie]
}
}
In this example, the filter
method utilizes a Predicate
functional interface to process the data seamlessly.
2. Event Handling in GUI Applications
Functional interfaces can effectively handle events in GUI applications as well, enabling clean and simplified code.
import javax.swing.*;
import java.awt.event.ActionListener;
public class ButtonExample {
public static void main(String[] args) {
JButton button = new JButton("Click Me");
button.addActionListener(e -> System.out.println("Button clicked!"));
JFrame frame = new JFrame("Functional Interface Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(button);
frame.setSize(200, 200);
frame.setVisible(true);
}
}
Here, the ActionListener
is implemented using a Lambda expression to provide the functionality behind the button click event.
Bringing It All Together
Mastering functional interfaces is pivotal to optimizing your Java programming skillset. Recognizing and avoiding common pitfalls is crucial for writing concise and efficient code. While working with functional interfaces, remember to treat them as special constructs designed for functional programming.
Always use the @FunctionalInterface
annotation, leverage built-in functional interfaces, and grasp the power of type inference. Your journey into functional programming within Java starts here.
For more in-depth understanding, you can explore Java's Functional Programming Features or dive into the Java Stream API documentation for practical implementations.
Embrace the simplicity and power of functional interfaces and elevate your Java development skills!