Common Pitfalls When Using Java 8 Stream API
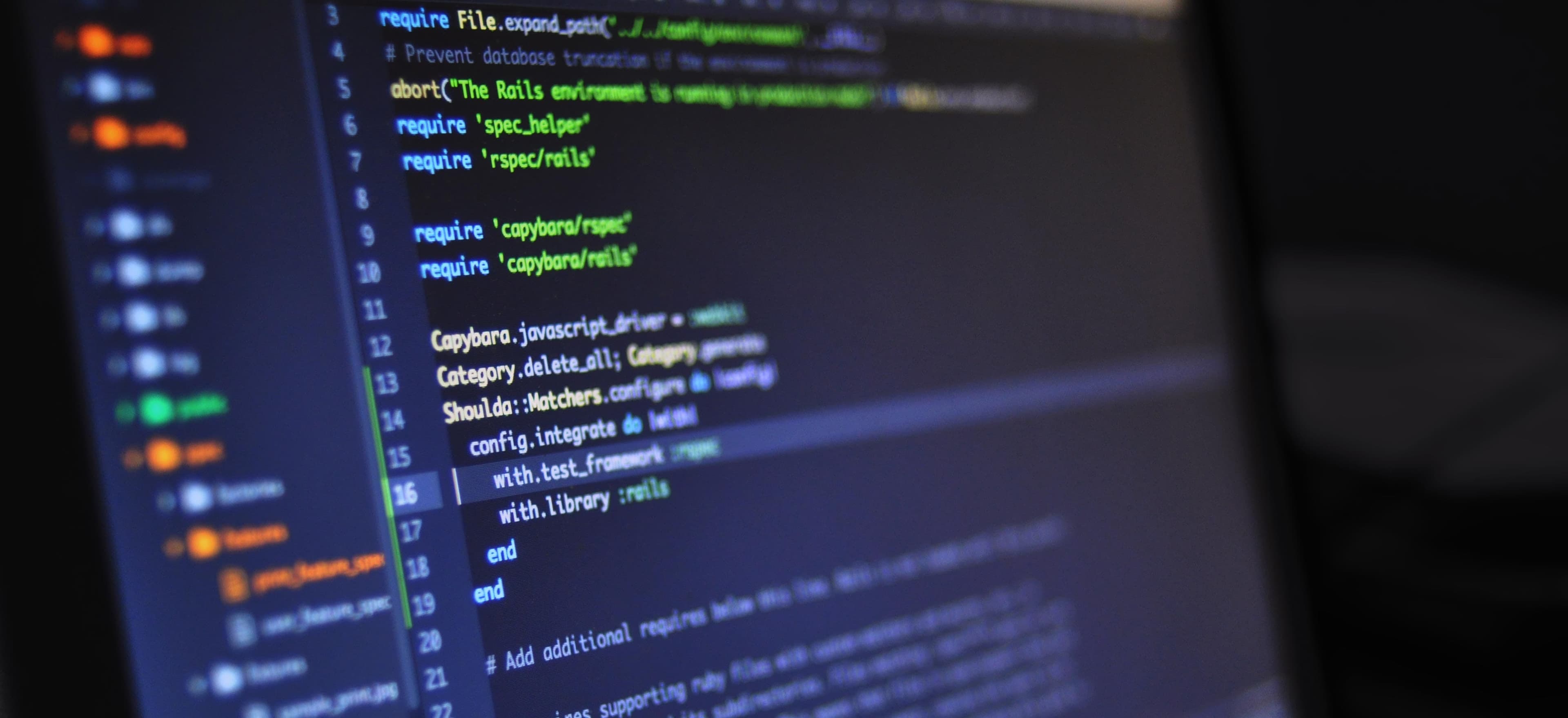
- Published on
Common Pitfalls When Using Java 8 Stream API
Java 8 introduced the Stream API, a powerful feature designed to simplify the process of processing sequences of data. Although it streamlines many operations that were previously cumbersome, using Streams incorrectly can lead to performance issues, code that is hard to read, or unintended behaviors. In this post, we will explore common pitfalls when using the Java 8 Stream API and how to avoid them.
Understanding Streams
Before we dive into the pitfalls, it’s essential to understand what Streams are. Streams can be seen as a sequence of data elements supporting sequential and parallel aggregate operations. They provide built-in methods for filtering, mapping, and reducing data in a functional style.
Let's check out a simple example:
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", "Jane", "Jack", "Jill");
names.stream()
.filter(name -> name.startsWith("J"))
.forEach(System.out::println);
}
}
In this example, we create a Stream from a list of names, filter it to include only names that start with 'J', and then print each name.
Pitfall 1: Not Understanding Intermediate and Terminal Operations
One of the most common mistakes in using Streams is confusing intermediate operations with terminal operations. Intermediate operations (like filter
, map
, sorted
) do not trigger any processing; they are lazy and only define how the data should be processed. Terminal operations (like forEach
, collect
, reduce
) trigger the processing and produce a result.
Why It Matters: Using intermediate operations without a terminal operation can lead to performance issues and confusion in your code.
import java.util.Arrays;
public class MisunderstoodStreams {
public static void main(String[] args) {
Arrays.asList(1, 2, 3, 4)
.stream()
.filter(n -> n % 2 == 0); // No terminal operation
// No output because no processing has been executed
}
}
How to Fix It
Always follow up intermediate operations with a terminal operation to ensure processing happens. Here's the corrected version:
import java.util.Arrays;
public class CorrectedStreams {
public static void main(String[] args) {
Arrays.asList(1, 2, 3, 4)
.stream()
.filter(n -> n % 2 == 0)
.forEach(System.out::println); // Outputs: 2, 4
}
}
Pitfall 2: Excessive Use of Streams
While the Stream API is powerful, overusing it can lead to less readable and harder-to-maintain code. In particular, excessive chaining of operations or using Streams for simple tasks can make your code less straightforward.
Why It Matters: Code clarity is crucial for maintenance. If a feature is quite simple, such as just summing a list of numbers, using a Stream might make the code unnecessarily complex.
import java.util.Arrays;
public class OveruseOfStreams {
public static void main(String[] args) {
int sum = Arrays.asList(1, 2, 3, 4)
.stream()
.reduce(0, Integer::sum); // Unnecessary use of Stream API
System.out.println(sum); // Outputs: 10
}
}
How to Fix It
For simple tasks, consider sticking to traditional loops or methods where appropriate:
import java.util.Arrays;
public class BetterApproach {
public static void main(String[] args) {
int sum = 0;
for(int i : Arrays.asList(1, 2, 3, 4)) {
sum += i; // Simple and clear
}
System.out.println(sum); // Outputs: 10
}
}
Pitfall 3: Mutable State in Streams
Using mutable state within a Stream operation can lead to unpredictable behavior and bugs. Streams are designed to be stateless and sequentially operate over data.
Why It Matters: If you modify a shared variable within a Stream, especially during parallel processing, it can lead to race conditions and incorrect results.
import java.util.Arrays;
import java.util.List;
public class MutableState {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4);
int[] sum = {0}; // Mutable state
numbers.stream()
.forEach(n -> sum[0] += n); // Using mutable state
System.out.println(sum[0]); // Outputs: 10, but risky in a parallel stream
}
}
How to Fix It
Instead, use collector methods or local variables. Here’s how to do that properly:
import java.util.Arrays;
public class StatelessApproach {
public static void main(String[] args) {
int sum = Arrays.asList(1, 2, 3, 4)
.stream()
.mapToInt(Integer::intValue) // Converting to an int stream
.sum(); // Using built-in method
System.out.println(sum); // Outputs: 10
}
}
Pitfall 4: Premature Optimization with Parallel Streams
Using parallel streams can improve performance but can also cause problems if misused. Not all operations benefit from parallelism. If your task is not CPU-intensive, the overhead of managing multiple threads may outweigh the benefits.
Why It Matters: Running small tasks in parallel can actually slow down performance and introduce complexity that may lead to bugs.
import java.util.Arrays;
public class PrematureOptimization {
public static void main(String[] args) {
int sum = Arrays.asList(1, 2, 3, 4)
.parallelStream() // Parallel stream usage
.mapToInt(Integer::intValue)
.sum(); // Might not be efficient
System.out.println(sum); // Outputs: 10
}
}
How to Fix It
Use parallel streams only when the workload justifies the overhead, typically for heavy computations or large datasets. You can profile your code before and after using parallel streams to measure the benefits.
Pitfall 5: Ignoring Exceptions
Streams simplify loop operations, but when it comes to exception handling, many developers forget to manage exceptions. Relying on Stream operations without proper error handling can lead to silent failures.
Why It Matters: Failing to handle exceptions in Streams can sometimes lead to lost data, inconsistencies, and makes debugging difficult.
import java.util.Arrays;
public class IgnoringExceptions {
public static void main(String[] args) {
Arrays.asList("1", "2", "abc") // Includes a string that cannot be parsed
.stream()
.map(Integer::parseInt) // This will throw an exception
.forEach(System.out::println);
}
}
How to Fix It
Utilize try-catch blocks or wrap the parsing in a method that handles possible exceptions gracefully.
import java.util.Arrays;
public class ExceptionHandling {
public static void main(String[] args) {
Arrays.asList("1", "2", "abc")
.stream()
.map(StreamExceptionHandling::safeParse) // Handling exceptions
.forEach(System.out::println);
}
private static Integer safeParse(String str) {
try {
return Integer.parseInt(str);
} catch (NumberFormatException e) {
System.err.println("Invalid number: " + str);
return null; // Or some default value
}
}
}
Key Takeaways
The Stream API in Java 8 brings incredible power and flexibility for data processing. However, it is essential to be wary of common pitfalls, such as misunderstanding operations, overusing streams, involving mutable states, misusing parallelism, and neglecting exception handling.
Being aware of these issues and understanding how to tackle them will help you write cleaner, more efficient, and maintainable code. If you want to learn more about the Stream API, consider consulting the Java Documentation or exploring various Java 8 tutorials.
By keeping these pitfalls in mind, you will be better equipped to leverage the full power of the Stream API effectively. Happy coding!