Unlocking Java 9: Overcoming Common Feature Adoption Challenges
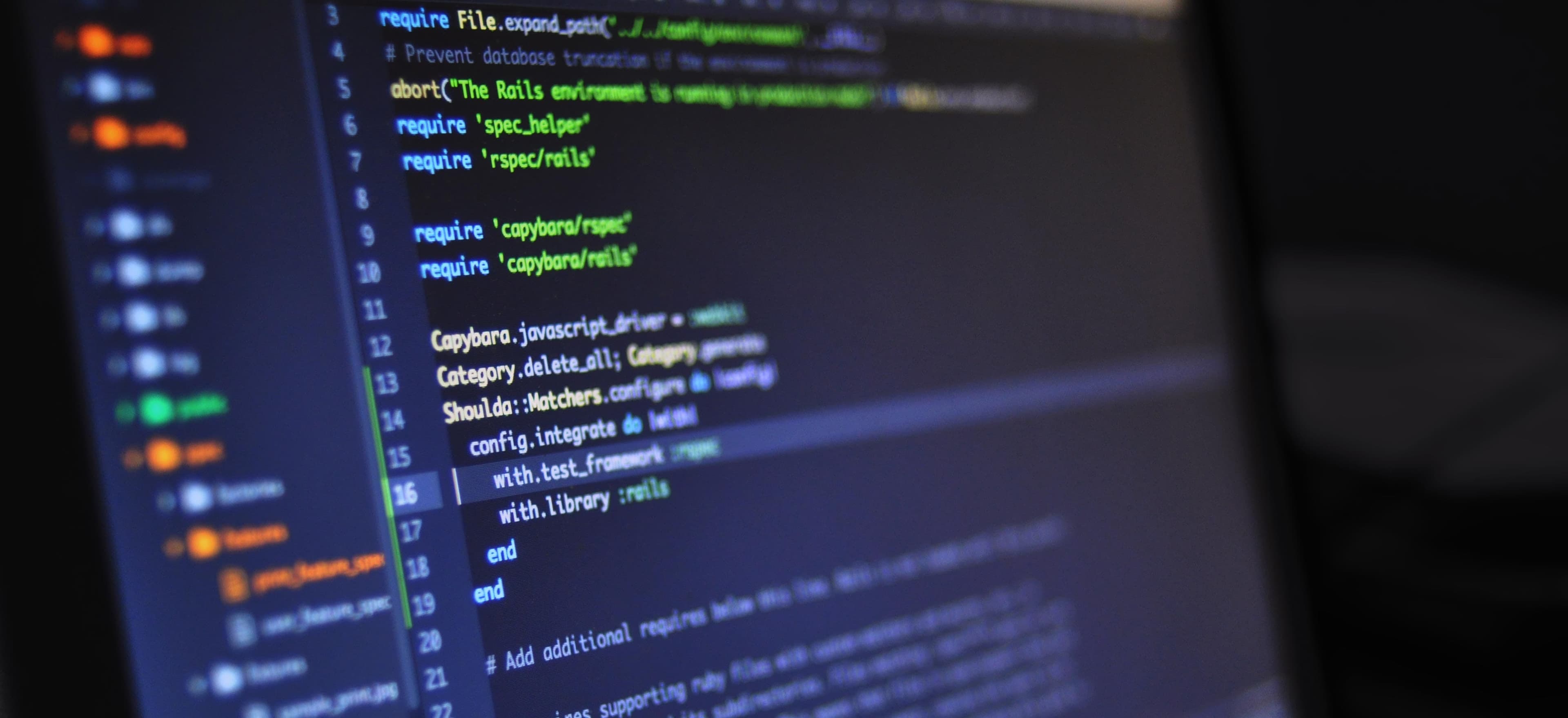
- Published on
Unlocking Java 9: Overcoming Common Feature Adoption Challenges
Java 9 introduced a plethora of new features aimed at enhancing productivity and performance. However, the adoption of these features can be challenging. This blog post will delve into the common hurdles developers face when integrating Java 9 into their workflow and how you can overcome them.
We'll break down Java 9's key features, provide code snippets, and highlight best practices, all while ensuring a better understanding this new version brings to developers.
Java 9 Features Overview
Before diving into the challenges, let’s quickly recap the significant features introduced in Java 9:
- Module System (Project Jigsaw)
- JShell
- Improved Stream API
- Private Methods in Interfaces
- Reactive Streams
These features can significantly enhance your coding experience, but they might pose confusion or roadblocks for those unfamiliar with them.
1. Understanding the Module System
One of the most significant changes in Java 9 is the Module System. This feature allows developers to define modules that encapsulate code, leading to better-organized projects and strong encapsulation.
Common Hurdle: Complexity in Migration
Migrating a legacy system to use modules can seem daunting. Developers often struggle with how to break their code into modules effectively.
Solution: Gradual Module System Adoption
Start small. Identify independent components in your application that can be converted into modules without immediately altering your entire project.
Here is a simple module definition:
module com.example.myapp {
exports com.example.myapp.services;
}
Commentary:
By exporting the services
package, you allow other modules to utilize the public classes defined within it. This encapsulation promotes modularity and maintainability.
Helpful Resources
For detailed guidance on modularity, check out Oracle's Java Module System Documentation.
2. Leveraging JShell for Quick Prototyping
JShell is the interactive Java REPL (Read-Eval-Print Loop) that allows developers to write and run snippets of Java code quickly.
Common Hurdle: Shifting Mindset
Many developers are accustomed to a traditional compile-run cycle, making it hard to embrace JShell's interactive capabilities.
Solution: Incorporating JShell into Daily Workflow
Try using JShell for testing algorithms or trying out Java APIs.
Here's an example of how JShell can be used:
// Start JShell and execute these lines
String greeting = "Hello, Java 9!";
System.out.println(greeting);
Commentary:
This approach allows for experimentation without the overhead of setting up an entire project, fostering a more agile development mindset.
Helpful Resources
Learn more about using JShell by visiting the JShell documentation.
3. Enhanced Stream API
Java 9 has added a few new methods to the Stream API, making data processing easier and more flexible.
Common Hurdle: Understanding New Methods
The new methods, such as takeWhile
, dropWhile
, and ofNullable
, can be confusing at first.
Solution: Practice with Practical Examples
Utilize these methods in appropriate scenarios to find their value.
Here is a code example using takeWhile
:
import java.util.List;
import java.util.stream.Collectors;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = List.of(1, 2, 3, 4, 5, 6);
List<Integer> evenNumbers = numbers.stream()
.takeWhile(n -> n % 2 == 0)
.collect(Collectors.toList());
System.out.println(evenNumbers);
}
}
Commentary:
In this snippet, takeWhile
will process the stream until the condition fails. This improves readability and directly communicates the programmer's intent.
Helpful Resources
For a closer look at the Stream API enhancements, refer to Java SE 9 Documentation on Streams.
4. Private Methods in Interfaces
Java 9 allows for private methods in interfaces, which can lead to cleaner and more maintainable code.
Common Hurdle: Adjusting to Interface Changes
Some developers may fear that private methods will complicate the simple interface structure they’re used to.
Solution: Embrace the Changes
Recognize that private methods can reduce code duplication within the interface, leading to cleaner code.
Example:
public interface MyInterface {
default void greet() {
System.out.println(getGreeting());
}
private String getGreeting() {
return "Hello from MyInterface!";
}
}
Commentary:
A private method in an interface can be utilized by default methods, allowing for reusability without exposing the utility functionality to the implementing classes.
Helpful Resources
To understand this feature better, check out the Java SE 9 Documentation on Private Methods.
5. Working with Reactive Streams
Java 9 introduces the java.util.concurrent.Flow
API, which implements the Reactive Streams specification, enabling asynchronous programming.
Common Hurdle: Conceptual Overhead of Reactivity
The reactive paradigm can be abstract and complex for many developers transitioning from traditional imperative programming.
Solution: Start with Small Implementations
Begin with simple use cases to understand the reactive approach gradually.
Here's a short example:
import java.util.concurrent.Flow;
public class SimplePublisher implements Flow.Publisher<String> {
private final String message;
SimplePublisher(String message) {
this.message = message;
}
@Override
public void subscribe(Flow.Subscriber<? super String> subscriber) {
subscriber.onSubscribe(new SimpleSubscription(subscriber, message));
}
}
class SimpleSubscription implements Flow.Subscription {
private final Flow.Subscriber<? super String> subscriber;
private final String message;
SimpleSubscription(Flow.Subscriber<? super String> subscriber, String message) {
this.subscriber = subscriber;
this.message = message;
}
public void request(long n) {
for (int i = 0; i < n; i++) {
subscriber.onNext(message);
}
subscriber.onComplete();
}
public void cancel() {}
}
Commentary:
In this example, we create a simple publisher that sends messages to subscribers. Understanding how to implement these reactive components can initially feel overwhelming, but practice makes it easier.
Helpful Resources
Explore more about the Flow API in the official Java SE documentation.
Closing Remarks
Adopting new features in Java 9 can pose several challenges. However, by proactively addressing common hurdles—be they related to module migration, embracing JShell, understanding the new Stream API methods, redefining interface structures, or learning reactive programming—you can integrate these advancements smoothly into your development practices.
The benefits Java 9 provides are worthwhile, realigning your approach to Java programming with modern needs. Engage with these features, utilizing hands-on examples to build familiarity and confidence. As you do, you’ll find that the transition to Java 9 can be not just manageable, but transformative.
For more in-depth knowledge, consider exploring the official Java 9 release notes and stay updated with new resources as Java continues to evolve. Happy coding!