Streamline Your Java Development: Run without Compilation!
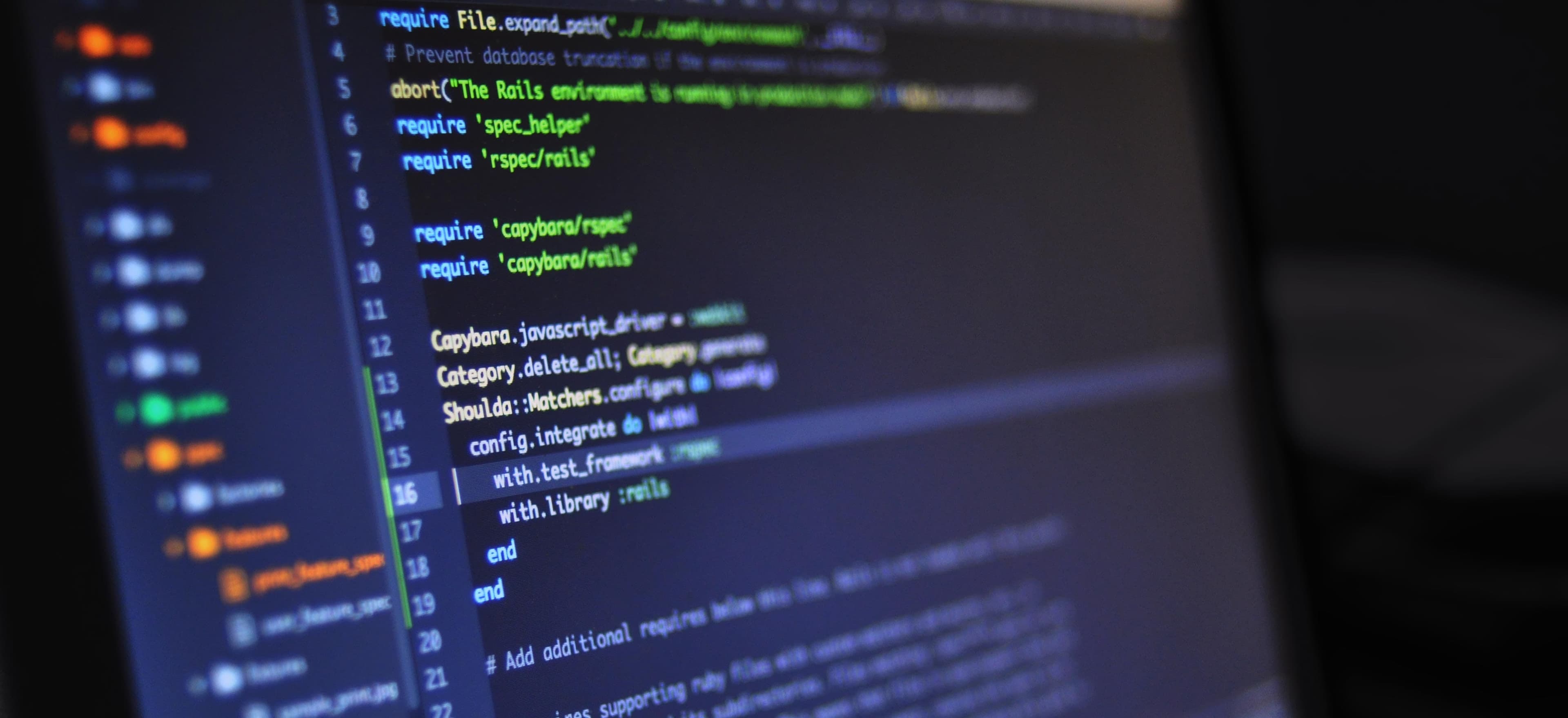
- Published on
Streamline Your Java Development: Run Without Compilation!
Java has long been heralded for its robustness, scalability, and portability. However, one of the significant hurdles for developers is the compilation step that transforms human-readable code into bytecode. This traditional process can sometimes slow down development, especially during the iterative coding phases. What if we told you that you can actually run Java code without the need for explicit compilation? In this blog post, we'll explore how you can streamline your Java development process using different techniques and tools.
Understanding the Compilation Process
Before we dive into the alternatives, let’s take a moment to understand why Java requires compilation. Java code is written in plain text source files, which are then compiled into bytecode. This bytecode is what the Java Virtual Machine (JVM) executes.
While this compilation step ensures type safety and optimization, it may seem cumbersome, especially when you are making numerous quick changes. Fortunately, there are ways to sidestep this tedious process while still running your code.
Dynamic Execution with JShell
Introduced in Java 9, JShell is an interactive tool that allows developers to execute Java code snippets without the need for a full-blown compilation process.
What is JShell?
JShell is essentially a REPL (Read-Eval-Print Loop) for Java, enabling you to write and run Java code in real-time. With JShell, you can execute code line by line or define entire classes and methods on the fly.
Getting Started with JShell
To get started, launch the JShell in your terminal. You can do this by simply typing jshell
after installing Java 9 or later.
$ jshell
Upon launching JShell, you’ll see a welcome message. You are now ready to execute Java statements interactively.
Example: Running Code in JShell
Here’s a simple example of how to define and call a method within JShell.
jshell> int add(int a, int b) {
...> return a + b;
...> }
Why Use JShell?
JShell allows you to quickly test and debug small pieces of code without the overhead of compiling a complete program. It encourages experimentation and is particularly useful for beginners learning Java or seasoned developers testing snippets.
For more in-depth use cases of JShell, check out Oracle's official documentation.
Using Java with Scripts
If you're familiar with languages like Python or JavaScript, you're probably aware of the ability to execute scripts directly without the compilation step. Java has recently opened doors to similar functionalities.
The java
Command with Script Files
With Java 11 and later, you can run Java source code files directly using the java
command. Simply create a .java
file, and execute it.
Example: Running a Simple Java Program
Let’s say you have a file named HelloWorld.java
:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
To run this code without explicit compilation, use the following command:
$ java HelloWorld.java
Why This Matters
Running Java programs directly helps in reducing the time you spend moving from writing code to seeing results. This is particularly useful when making small, iterative changes that don’t require full-fledged development environments.
Leveraging Build Tools
Ever heard of Gradle or Maven? These build automation tools have streamlined the Java development experience greatly. Both of these tools automate tasks such as compilation, packaging, and running Java applications.
Gradle’s run
Task
Gradle allows you to define a task to run your application. When you have a simple project structure, you can quickly execute your code by defining the run
task in your build.gradle
file.
apply plugin: 'application'
mainClassName = 'HelloWorld'
dependencies {
// Include your dependencies here
}
Following this configuration, you can run your application using:
$ gradle run
Why Build Tools Help
Both Gradle and Maven help manage dependencies, facilitate version control, and automate repetitive tasks. They can significantly reduce the friction of the development process by providing pre-defined tasks for various stages of development.
Using IDEs with Built-in Compilation
Integrated Development Environments (IDEs) such as IntelliJ IDEA and Eclipse come with built-in support for running Java programs efficiently. Typically, when you run a program in these IDEs, they handle the compilation and execution in the background, allowing for a seamless run-compile-experience.
Example: IntelliJ IDEA
In IntelliJ IDEA, simply write your Java program and hit the 'Run' button. The IDE compiles your code automatically and executes it.
Why Choose an IDE?
Using an IDE allows you to take advantage of various productivity features such as code suggestions, debugging tools, and version control integration. You'll spend less time managing tasks and more time focusing on coding.
Summary: Choosing the Right Approach
Java development has evolved considerably, offering numerous methods to reduce or eliminate the need for explicit compilation. Here’s a summary of what you can consider:
- JShell: Ideal for testing and debugging small snippets of code interactively.
- Running Java Scripts: Simplifies the process of testing your code by executing Java files directly.
- Build Tools: Automate mundane tasks and improve dependency management with tools like Gradle and Maven.
- IDEs: Provide a comprehensive set of tools for writing, debugging, and running Java applications effortlessly.
By embracing these tools and practices, you can significantly streamline your Java development process. You'll find yourself writing code more efficiently and focusing more on solving problems rather than dealing with lengthy compilation processes.
Additional Resources
For further reading and a deeper dive into Java's evolving landscape, consider checking out the Java SE Documentation and Gradle User Manual.
In the modern software development world, efficiency is key. So why stick to old paradigms when you can run Java without compilation and enhance your productivity? Happy coding!