Mastering Dependency Injection: Common Pitfalls and Solutions
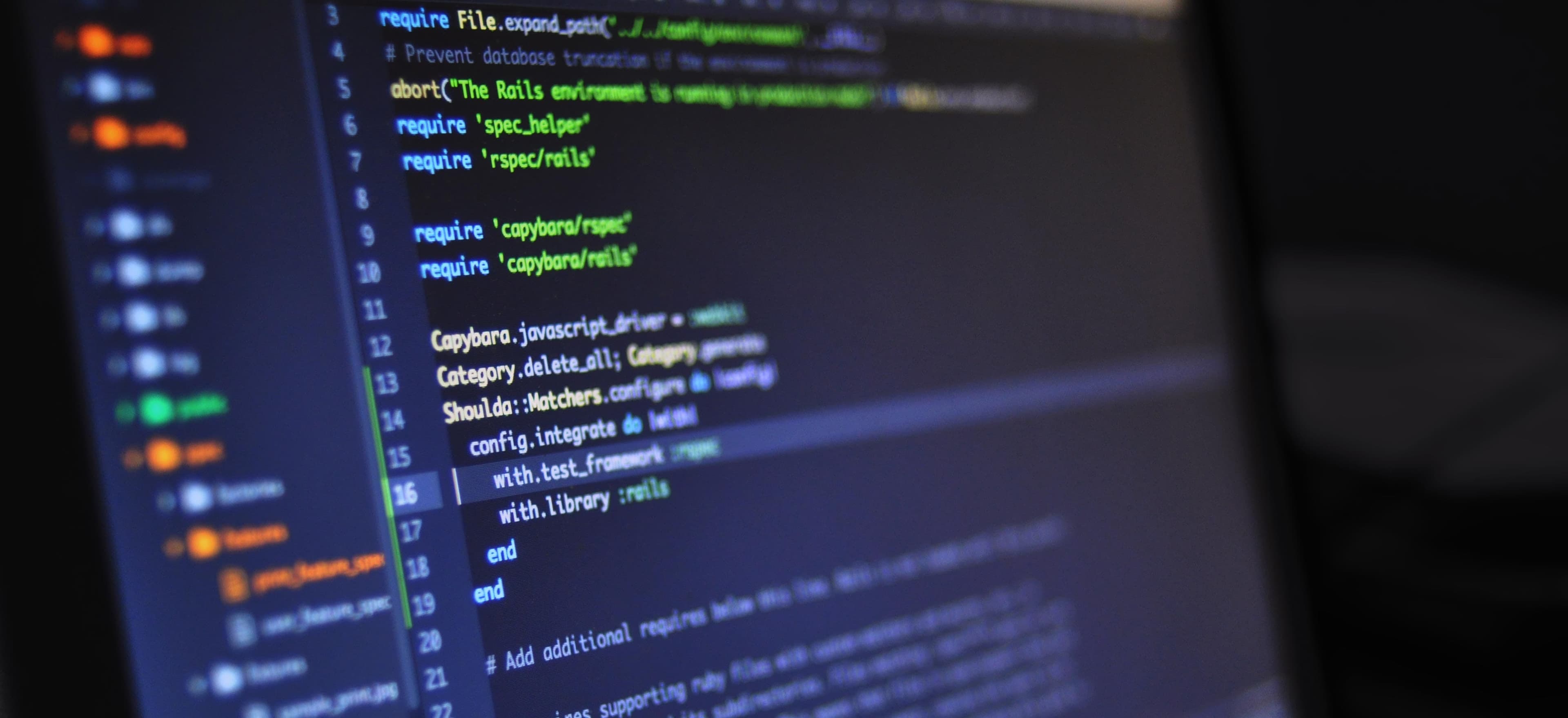
- Published on
Mastering Dependency Injection: Common Pitfalls and Solutions
Dependency Injection (DI) is a powerful design pattern used in software development, particularly with Java. It allows for the creation of loosely coupled, testable, and maintainable applications. However, while DI offers significant benefits, developers often encounter pitfalls. In this blog post, we'll explore common pitfalls associated with Dependency Injection in Java and discuss effective solutions to avoid them.
What is Dependency Injection?
Before we delve deeper into the pitfalls, it's crucial to understand what DI entails. Dependency Injection is a technique whereby one object supplies the dependencies (i.e., other objects) to another object. This is typically done through:
- Constructor Injection
- Setter Injection
- Interface Injection
These methods can enhance your application's flexibility and ease of testing.
Common Pitfalls of Dependency Injection
1. Overusing Dependency Injection
Problem: One common mistake developers make is over-injecting dependencies, resulting in a class that has more dependencies than it needs. This can lead to bloated constructors and classes that are hard to manage.
Solution: Keep your classes focused. Follow the Single Responsibility Principle (SRP). A class should only have one reason to change. If you find that your class is accumulating too many dependencies, consider refactoring it into smaller, more manageable classes.
Example: Consider the following example:
public class UserService {
private final EmailService emailService;
private final SMSNotificationService smsNotificationService;
private final UserRepository userRepository;
public UserService(EmailService emailService, SMSNotificationService smsNotificationService, UserRepository userRepository) {
this.emailService = emailService;
this.smsNotificationService = smsNotificationService;
this.userRepository = userRepository;
}
// Other service methods...
}
Here, UserService
is managing multiple responsibilities. Instead of injecting all those dependencies, separate the concerns:
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
// Method to handle user registration
public void registerUser(User user) {
// Registration logic...
}
}
public class NotificationService {
private final EmailService emailService;
private final SMSNotificationService smsNotificationService;
public NotificationService(EmailService emailService, SMSNotificationService smsNotificationService) {
this.emailService = emailService;
this.smsNotificationService = smsNotificationService;
}
// Method to send notifications
}
2. Circular Dependencies
Problem: Circular dependencies occur when two or more classes depend on each other directly or indirectly. This can lead to runtime errors and make the application difficult to understand.
Solution: One way to resolve circular dependencies is to use interfaces. You can define a contract between the services through an interface. This approach decouples the classes and minimizes the dependency chain.
Example: Instead of directly referencing each other:
public class A {
private final B b;
public A(B b) {
this.b = b;
}
}
public class B {
private final A a;
public B(A a) {
this.a = a;
}
}
Refactor using interfaces:
public interface AInterface {
void doSomething();
}
public interface BInterface {
void doSomethingElse();
}
public class A implements AInterface {
private final BInterface b;
public A(BInterface b) {
this.b = b;
}
public void doSomething() {
// logic...
b.doSomethingElse();
}
}
public class B implements BInterface {
private final AInterface a;
public B(AInterface a) {
this.a = a;
}
public void doSomethingElse() {
// logic...
}
}
3. Ignoring Scope and Lifecycle
Problem: Not considering the lifecycle of the injected instances can lead to resource leaks, unexpected behavior, or performance issues. In frameworks like Spring, the default singleton scope means that the same instance will be shared across the application.
Solution:
Be intentional with the scope of your beans. Use @Scope
annotations in Spring to define the scope (Singleton, Prototype, Request, etc.) according to your specific requirement.
Example: If you need a new instance every time, use:
@Service
@Scope("prototype")
public class PrototypeService {
// Service code...
}
4. Fragile Global State
Problem: Using global state or static classes can make your codebase fragile and lead to challenges in testing and maintenance.
Solution: Avoid using static states and instead rely on dependency injection to manage your dependencies. This ensures each instance is self-contained and easier to manage.
Example: Instead of static access:
public class UtilityClass {
public static void someStaticMethod() {
// Utility logic...
}
}
Consider injecting the utility as a dependency:
public class SomeService {
private final UtilityInterface utility;
public SomeService(UtilityInterface utility) {
this.utility = utility;
}
public void execute() {
utility.someMethod();
}
}
5. Not Utilizing Testing Benefits
Problem: Some developers don't take full advantage of DI for unit testing, often hardcoding dependencies instead, which defeats the purpose of DI.
Solution: Utilize mocking frameworks like Mockito to create mock objects for testing. This allows you to test your classes in isolation.
Example: Here’s how we can use Mockito in a unit test:
@RunWith(MockitoJUnitRunner.class)
public class UserServiceTest {
@InjectMocks
private UserService userService;
@Mock
private UserRepository userRepository;
@Test
public void testRegisterUser() {
User user = new User("test@example.com");
userService.registerUser(user);
verify(userRepository).save(user);
}
}
Lessons Learned
Mastering Dependency Injection in Java is an art that comes with practice and understanding. By being aware of the common pitfalls like overusing DI, circular dependencies, lifecycle management, fragile state, and the lack of testing benefits, you can write cleaner, more maintainable code.
Further Learning:
- Explore Spring Dependency Injection for more advanced DI techniques.
- Check out the Mockito documentation to understand how to effectively mock your dependencies.
By maintaining clarity and structure in your DI setup, you enable your Java applications to remain agile and ready for the fast-paced demands of modern software development. Happy coding!