Common Pitfalls in Spring Data JPA and How to Avoid Them
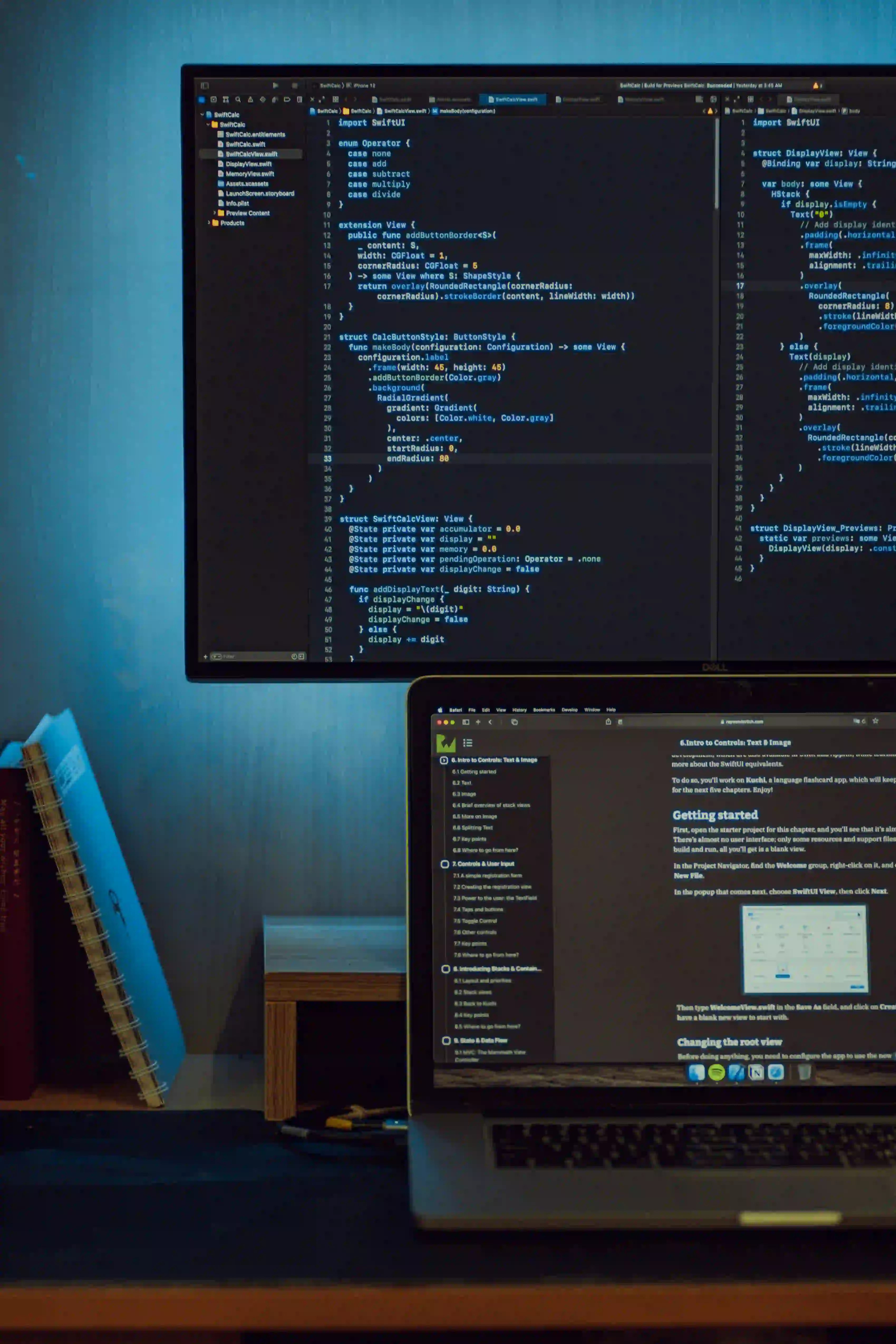
Common Pitfalls in Spring Data JPA and How to Avoid Them
Spring Data JPA is a powerful framework for simplifying the interaction between Java applications and relational databases. However, while it provides many features that streamline database interactions, developers can encounter several pitfalls that may lead to performance issues, data integrity problems, or unintended behavior. In this post, we will explore some common pitfalls in Spring Data JPA and discuss effective strategies to avoid them.
Lack of Transaction Management
The Issue
One of the most critical aspects of working with databases is ensuring that your data remains consistent. In Spring Data JPA, transactions are important for grouping operations and ensuring that either all operations are completed successfully, or none are.
The Solution
Make sure to use @Transactional
annotations properly. This tells Spring to manage transactions for you, wrapping methods in a transaction context.
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
@Transactional
public void saveUser(User user) {
userRepository.save(user);
}
}
By annotating the saveUser
method with @Transactional
, we ensure that if anything goes wrong during the save operation, the transaction will roll back, preserving data integrity.
Ignoring Fetch Types
The Issue
Spring Data JPA offers various fetch types, specifically EAGER
and LAZY
. If you fail to specify these correctly, you may encounter performance bottlenecks. For example, setting fetch type to EAGER
will load all related entities immediately, which can significantly slow down your application.
The Solution
Choose the appropriate fetch strategy based on your use case. Use LAZY
loading where possible, particularly for collections or large entities that you might not need right away.
@Entity
public class Post {
@ManyToOne(fetch = FetchType.LAZY)
private User user;
// Other fields and methods
}
In this example, the user
field will only be loaded when accessed, which can improve performance by not fetching data we do not require immediately.
N+1 Select Problem
The Issue
The N+1 select problem occurs when your application makes additional queries for each related entity, resulting in performance degradation. For example, when you fetch a list of entities, and for each entity, you fetch its related entities one-by-one.
The Solution
Utilize JOIN FETCH
in your queries to load associated entities in one go.
public interface PostRepository extends JpaRepository<Post, Long> {
@Query("SELECT p FROM Post p JOIN FETCH p.user")
List<Post> findAllPostsWithUsers();
}
This JOIN FETCH
query retrieves all posts along with their users in a single query, thus avoiding the N+1 select problem and improving performance.
Incorrect Use of @Query Annotations
The Issue
While the @Query
annotation provides a powerful way to customize queries, incorrect usage can lead to performance issues or exceptions.
The Solution
Be cautious with your custom queries. Always test them thoroughly and watch for inefficiencies or errors.
public interface UserRepository extends JpaRepository<User, Long> {
@Query("SELECT u FROM User u WHERE u.email = ?1")
User findByEmail(String email);
}
The above query is simple and clear. Customize it as needed while ensuring it adheres to your performance and data integrity requirements.
Not Utilizing Paging and Sorting
The Issue
Failing to implement paging or sorting can lead to memory issues, especially when your result sets grow large.
The Solution
Use Spring Data JPA's built-in Pageable
and Sort
features to efficiently manage large datasets.
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
public interface UserRepository extends JpaRepository<User, Long> {
Page<User> findByLastName(String lastName, Pageable pageable);
}
Here, by adding Pageable
as a parameter, you enable pagination. This is essential in production-level applications where data sets can be large.
Misunderstanding Entity Lifecycle
The Issue
Understanding entity lifecycle states (transient, persistent, detached, removed) is crucial. Mismanagement can lead to data loss or incorrect state transitions.
The Solution
Be explicit about the lifecycle state of your entities:
- Create entities using
new
: They are transient. - Attach them via
save()
: They become persistent. - Detach using the
EntityManager
: Be aware of their transitions.
User user = new User();
user.setName("John Doe");
userRepository.save(user); // Now user is persistent
entityManager.detach(user); // Now user is detached
Be mindful of the entity's lifecycle state to maintain data integrity.
Overusing DTOs for Simple Cases
The Issue
Data Transfer Objects (DTOs) are beneficial when you need to transfer data between layers. However, overuse can complicate code and hinder performance in simple cases.
The Solution
Use DTOs strictly when necessary, especially in complex queries. For simple data transfer where the entire entity matches the expected structure, using the entity itself is often sufficient.
public class SimpleUserDto {
private String name;
private String email;
// Getters and setters
}
public interface UserRepository extends JpaRepository<User, Long> {
@Query("SELECT new com.example.SimpleUserDto(u.name, u.email) FROM User u")
List<SimpleUserDto> findAllSimpleUsers();
}
Use DTOs selectively to minimize complexity.
The Last Word
Spring Data JPA is a robust tool designed to simplify data access, but it comes with its pitfalls. By understanding these common issues and implementing the solutions discussed, you can enhance the performance and reliability of your application. Remember, effective transaction management, proper use of fetch strategies, and careful query optimization are fundamental to building efficient data-driven applications.
For more resources and in-depth documentation, consider checking the official Spring Data JPA documentation and the Spring Framework guides. Happy coding!