Common Pitfalls When Building RESTful APIs with Spring Boot
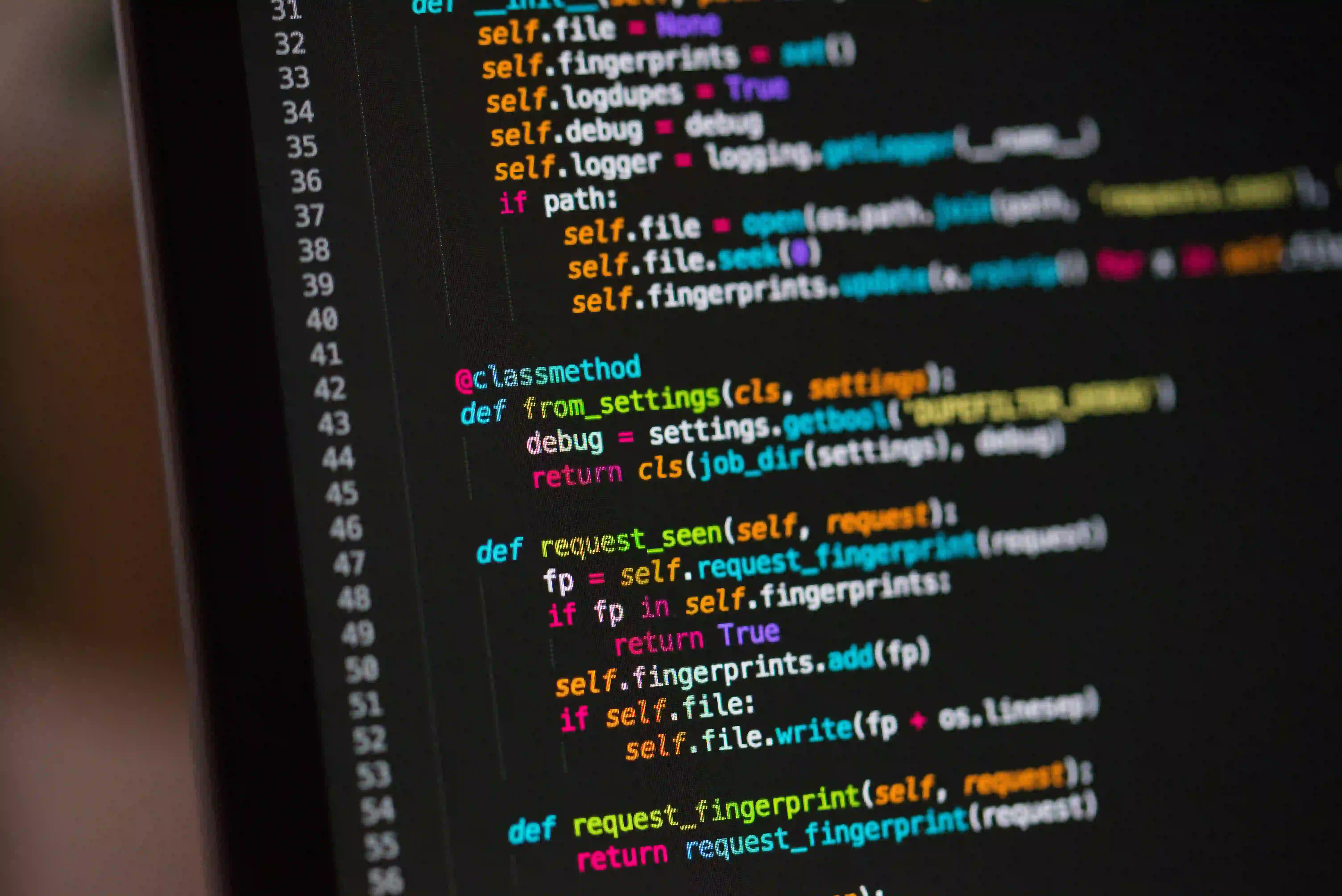
Common Pitfalls When Building RESTful APIs with Spring Boot
Building RESTful APIs using Spring Boot has become a widely adopted approach due to its simplicity and powerful features. However, navigating through the development process is not always straightforward. In this blog post, we'll discuss common pitfalls that developers encounter when building RESTful APIs with Spring Boot, emphasizing best practices to avoid these issues.
Table of Contents
- Understanding RESTful Principles
- Misconfiguring HTTP Methods
- Inadequate Exception Handling
- Not Using Proper Status Codes
- Ignoring Input Validation
- Inefficient Data Handling
- Poorly Designed API Endpoints
- Lack of Documentation
- Conclusion
1. Understanding RESTful Principles
Before diving into the technical aspects, it’s important to have a solid understanding of RESTful principles. REST (Representational State Transfer) is an architectural style that defines a set of constraints for creating web services. The main principle of REST is to use standard HTTP methods (GET, POST, PUT, DELETE) for CRUD operations.
Failing to understand these principles can lead to a confused API design. Make sure to familiarize yourself with the basics of REST. For further reading, check the RESTful API Tutorial.
Code Example: Simple REST Controller
Here's a simple example of a RESTful API controller for managing a book inventory:
@RestController
@RequestMapping("/api/books")
public class BookController {
@Autowired
private BookService bookService;
@GetMapping("/{id}")
public ResponseEntity<Book> getBook(@PathVariable Long id) {
Book book = bookService.findById(id);
return new ResponseEntity<>(book, HttpStatus.OK);
}
}
In this example, we adhere to REST principles by using the @GetMapping
annotation to fetch a book by its ID.
2. Misconfiguring HTTP Methods
Each HTTP method serves a specific purpose, yet many developers confuse them. For example, some might use GET for operations that should be POST. Such misconfigurations can lead to unintended consequences.
Solution
Ensure you are using the appropriate HTTP methods for the right actions: use POST for creating, GET for retrieving, PUT/PATCH for updating, and DELETE for removing resources.
Code Example: Distinction of Methods
@PostMapping
public ResponseEntity<Book> createBook(@RequestBody Book book) {
Book createdBook = bookService.save(book);
return new ResponseEntity<>(createdBook, HttpStatus.CREATED);
}
@PutMapping("/{id}")
public ResponseEntity<Book> updateBook(@PathVariable Long id, @RequestBody Book book) {
Book updatedBook = bookService.update(id, book);
return new ResponseEntity<>(updatedBook, HttpStatus.OK);
}
3. Inadequate Exception Handling
Uncaught exceptions in your REST API can lead to poor user experience and application instability. A common pitfall is failing to implement global exception handling.
Solution
Use @ControllerAdvice
to handle exceptions uniformly. This way, any error thrown in your application will return a structured response.
Code Example: Global Exception Handler
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<ErrorResponse> handleResourceNotFound(ResourceNotFoundException ex) {
ErrorResponse errorResponse = new ErrorResponse(HttpStatus.NOT_FOUND.value(), ex.getMessage());
return new ResponseEntity<>(errorResponse, HttpStatus.NOT_FOUND);
}
}
With this global exception handler, we provide a better experience by returning a consistent error format.
4. Not Using Proper Status Codes
HTTP status codes inform clients about the outcome of their requests. Misuse of status codes can lead to confusion and misinterpretations.
Solution
Always use standard HTTP status codes. For example, return 200 for successful GET requests, 201 for successful resource creation, and 404 for resource not found.
Code Example: Using Status Codes
@GetMapping("/{id}")
public ResponseEntity<Book> getBook(@PathVariable Long id) {
Book book = bookService.findById(id);
if (book == null) {
return new ResponseEntity<>(HttpStatus.NOT_FOUND);
}
return new ResponseEntity<>(book, HttpStatus.OK);
}
5. Ignoring Input Validation
Not validating input data can lead to multiple security vulnerabilities such as SQL injection or corrupted data states.
Solution
Use validation annotations from the javax.validation
package in your model classes and an additional layer of validation in your API controllers.
Code Example: Using Validation Annotations
public class Book {
@NotBlank(message = "Title is mandatory")
private String title;
@NotNull(message = "Author cannot be null")
private String author;
// Getters and Setters
}
@PostMapping
public ResponseEntity<Book> createBook(@Valid @RequestBody Book book) {
Book createdBook = bookService.save(book);
return new ResponseEntity<>(createdBook, HttpStatus.CREATED);
}
6. Inefficient Data Handling
Retrieving unnecessary data can lead to performance bottlenecks. Sending too much data requires more bandwidth and processing power on the client side.
Solution
Implement pagination and filtering where necessary to optimize data retrieval processes.
Code Example: Pagination
@GetMapping
public ResponseEntity<List<Book>> getAllBooks(@RequestParam(defaultValue = "0") int page,
@RequestParam(defaultValue = "10") int size) {
Page<Book> books = bookService.findAll(PageRequest.of(page, size));
return new ResponseEntity<>(books.getContent(), HttpStatus.OK);
}
7. Poorly Designed API Endpoints
Keeping your endpoints well-organized is essential for both maintainability and usability. Cluttered or inconsistent endpoints can confuse users.
Solution
Use nouns for resource names and keep URL structures clean and consistent. Consider RESTful naming conventions.
Code Example: Clear Endpoint Design
@RestController
@RequestMapping("/api/authors")
public class AuthorController {
@GetMapping("/{id}/books")
public ResponseEntity<List<Book>> getBooksByAuthor(@PathVariable Long id) {
List<Book> books = bookService.findByAuthorId(id);
return new ResponseEntity<>(books, HttpStatus.OK);
}
}
8. Lack of Documentation
Documentation is crucial for any API. It helps developers understand how to interact with your service appropriately.
Solution
Use tools like Swagger (OpenAPI) to automatically generate documentation for your API.
Code Example: Integrating Swagger
Add the following dependency to your pom.xml
:
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-boot-starter</artifactId>
<version>3.0.0</version>
</dependency>
Then, create a configuration class to enable Swagger support:
@EnableSwagger2
@Configuration
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2).select()
.apis(RequestHandlerSelectors.any())
.paths(PathSelectors.any())
.build();
}
}
Closing Remarks
Building RESTful APIs in Spring Boot is a rewarding process, but it's easy to fall into pitfalls that can disrupt development and user experience. By understanding REST principles, correctly utilizing HTTP methods and status codes, implementing exception handling, validating input, optimizing data handling, designing clear endpoints, and documenting thoroughly, you can avoid these common mistakes.
Ensuring best practices are in place will not only lead to more robust applications but also a better overall experience for developers and users alike. Happy coding!
For further insights, refer to the Spring Boot Documentation and the official Spring REST guidelines.