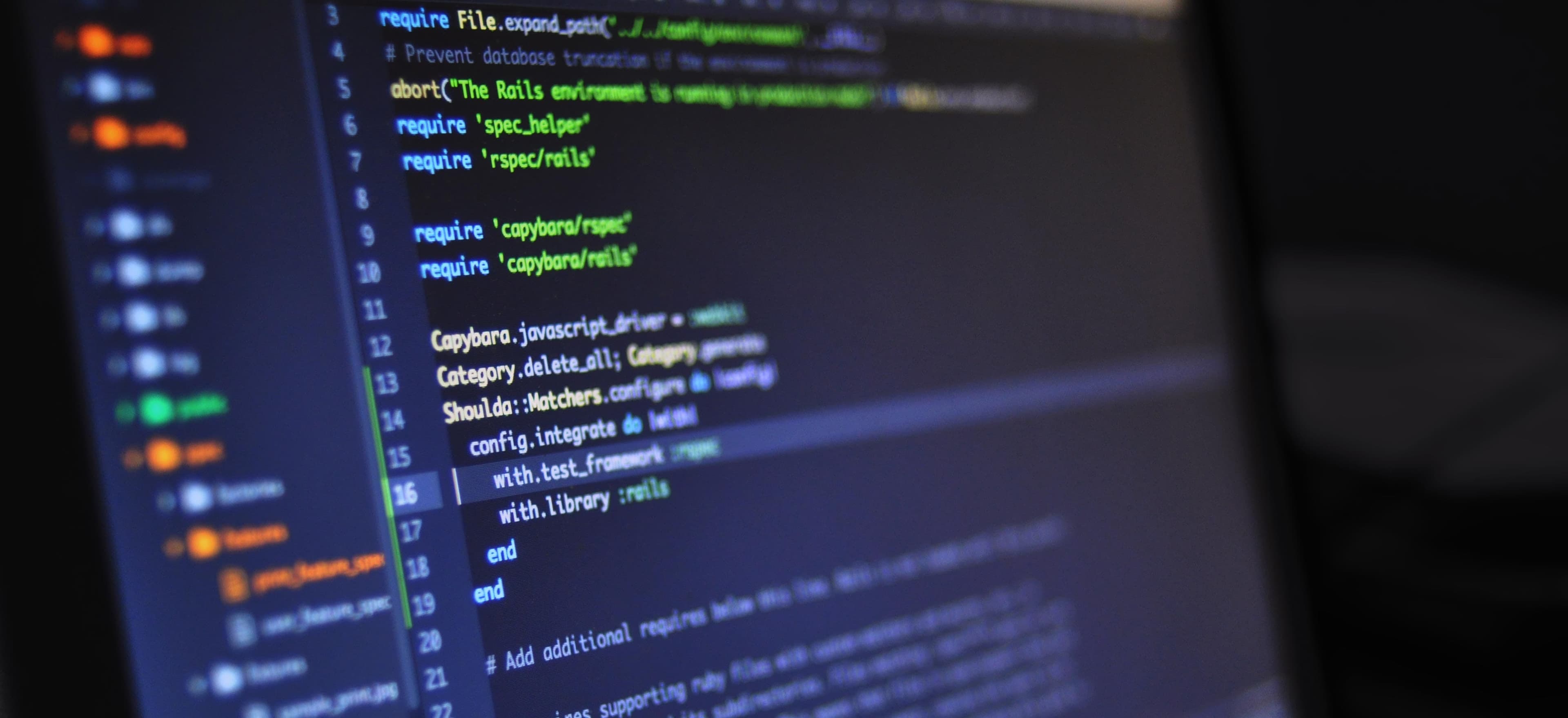
- Published on
Common Spring Security Pitfalls and How to Avoid Them
Spring Security is an integral part of building secure Java applications, but developers often encounter pitfalls that can lead to vulnerabilities and inefficiencies. This post will explore common mistakes and provide practical solutions to safeguard your applications.
Understanding Spring Security
Before we dive into the pitfalls, it's important to recognize that Spring Security is a powerful and customizable authentication and access control framework. It integrates well with Spring applications and offers comprehensive security features.
Key Features of Spring Security
- Authentication and Authorization: Validate users and control access to resources.
- Protection against Common Attacks: Mitigate threats such as CSRF, Session Fixation, and Clickjacking.
- Secure REST APIs: Easily secure your APIs with tokens and roles.
###Common Pitfalls in Spring Security and How to Avoid Them
Let's look at some of the most common pitfalls developers face when implementing Spring Security.
1. Inadequate Configuration
The Issue
One of the most frequent mistakes is failing to properly configure Spring Security. A misconfigured security context can lead to public access to sensitive areas or data leaks.
The Solution
Ensure that you give careful attention to your configuration files. Here’s a basic example:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public/**").permitAll() // Public endpoint
.anyRequest().authenticated() // All other requests need authentication
.and()
.formLogin() // Enables form login
.permitAll()
.and()
.logout()
.permitAll();
}
}
Why: This configuration permits public access to specific endpoints while protecting all others. A comprehensive approach ensures sensitive data and pages are secured.
2. Using Basic Authentication in Insecure Contexts
The Issue
Basic authentication transmits user credentials in an easily decodable format. If implemented over an insecure connection (HTTP), credentials can be intercepted by attackers.
The Solution
Always use HTTPS when implementing basic authentication. Here's an annotated approach:
@Bean
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password(passwordEncoder().encode("password")).roles("USER");
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
Why: Using BCryptPasswordEncoder ensures passwords are stored securely. The combination of HTTPS and proper password encoding greatly enhances security.
3. Not Managing User Sessions Properly
The Issue
Web applications often neglect session management, leading to potential session fixation attacks or excessive resource consumption via sessions.
The Solution
Implement session management rules within your Spring Security configuration. Here's how:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.sessionManagement()
.maximumSessions(1) // Limit number of concurrent sessions
.maxSessionsPreventsLogin(true); // Prevents login when limit is reached
}
Why: This configuration restricts concurrent logins, reducing the risk of session hijacking. Proper session management is key to maintaining secure user access.
4. Ignoring Cross-Site Request Forgery (CSRF) Protection
The Issue
Many developers assume that CSRF protection is optional, but neglecting it can open your application to significant vulnerabilities.
The Solution
Ensure CSRF protection is enabled by default. Here's how you can reinforce it:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf()
.csrfTokenRepository(CookieCsrfTokenRepository.withHttpOnlyFalse()); // Enable CSRF protection
}
Why: This implementation adds an additional layer of security by ensuring that requests are validated, protecting against CSRF attacks.
5. Over-reliance on Annotations for Security
The Issue
While annotations such as @PreAuthorize
offer a convenient way to control access, overusing them can lead to a fragmented security strategy.
The Solution
Combine method-level security with centralized configuration. Below is an example of using annotations effectively:
@RestController
@RequestMapping("/api")
public class ApiController {
@PreAuthorize("hasRole('ADMIN')")
@GetMapping("/admin")
public String adminAccess() {
return "Admin Access Granted!";
}
}
Why: This keeps business logic clean while ensuring security policies are clear. Use annotations where they make sense but maintain centralized configurations for broader security measures.
6. Weak Password Policies
The Issue
Simply allowing any password can compromise user security, especially if users choose weak passwords.
The Solution
Implement a robust password policy that requires complexity and regular changes. Here’s an example:
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder(10); // Configuring strength
}
Why: A BCrypt strength of 10 suggests a good balance of security and speed. Encourage users to select complex passwords to fortify their accounts.
7. Forgetting About Secure API Design
The Issue
Many developers focus only on securing endpoints and neglect the secure design of their APIs, leading to various vulnerabilities, including improper error handling and exposure of sensitive information.
The Solution
Adopt a defensive programming approach. Here's a basic framework for error handling:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleException(Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR)
.body("An error occurred: " + e.getMessage());
}
}
Why: This pattern captures unhandled exceptions and provides a user-friendly response, preventing sensitive data from being disclosed.
8. Not Regularly Updating Dependencies
The Issue
Keeping your dependencies up-to-date is critical for security. Neglecting this can lead to vulnerabilities that have been patched in newer versions.
The Solution
Regularly check for updates and apply them. Use a dependency management tool like Maven or Gradle. Here’s a command for Maven:
mvn versions:display-dependency-updates
Why: Regular updates ensure that you benefit from security fixes and enhancements, which play a crucial role in overall application security.
Lessons Learned
Spring Security is a robust framework, but it's vital to understand and avoid common pitfalls. By implementing best practices like proper configuration, session management, and error handling, you can significantly enhance the security of your applications.
As you build secure applications, always remember to stay informed about security trends and regularly review your security implementations. For further reading on Spring Security, refer to the Spring Security Documentation.
By diligently avoiding these pitfalls and consistently applying security best practices, you can create secure Java applications that protect user data and enhance trust in your services. Happy coding!
Checkout our other articles