Mastering Hibernate: Common Mapping Type Pitfalls
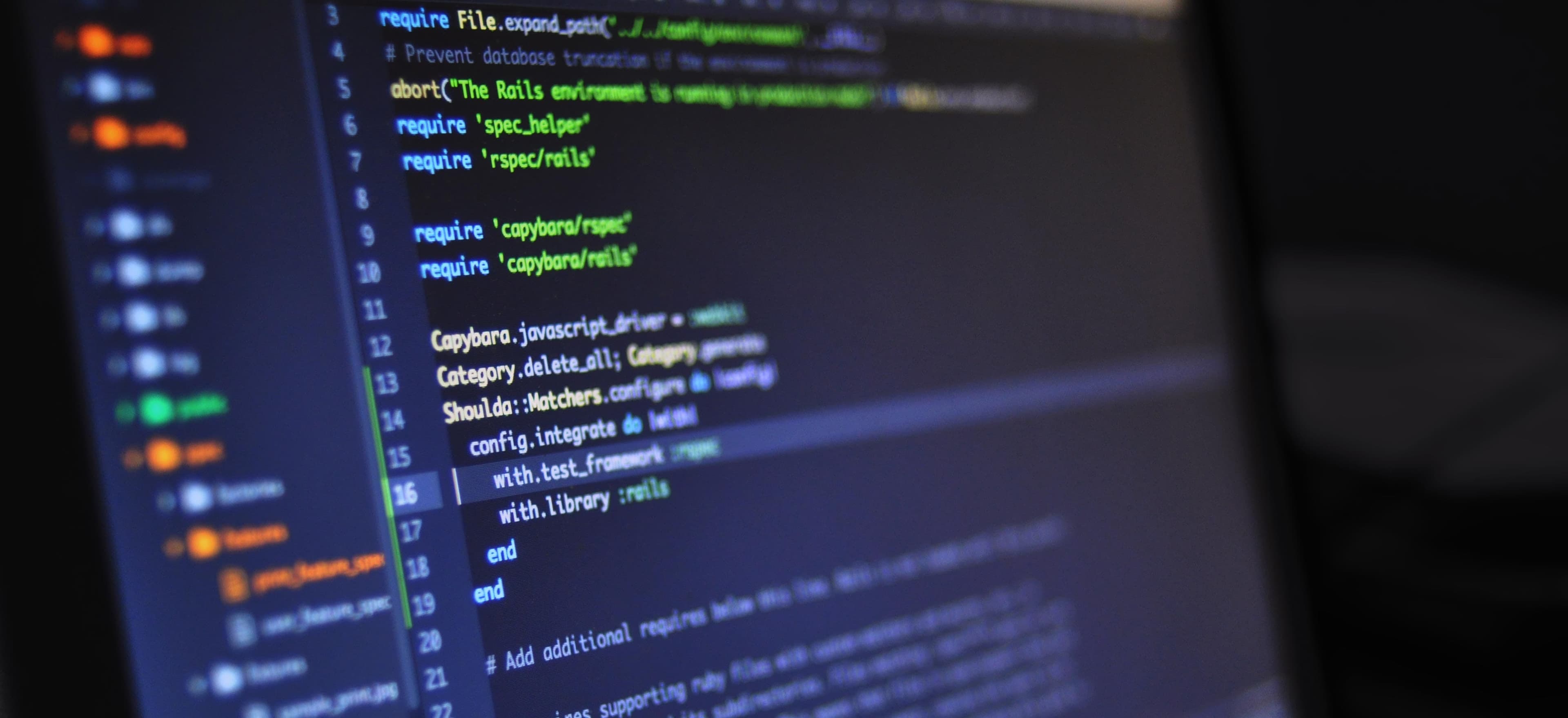
- Published on
Mastering Hibernate: Common Mapping Type Pitfalls
Hibernate is a powerful ORM (Object-Relational Mapping) framework that simplifies database interactions in Java applications. However, developers often encounter mapping type pitfalls when using Hibernate. Understanding these pitfalls and knowing how to avoid them is crucial for productive development. In this blog post, we will explore common mapping type issues, provide best practices, and showcase examples to illustrate how to navigate these challenges effectively.
Understanding Hibernate Mapping Types
Hibernate provides a wide range of mapping types for Java objects to SQL database tables. Some commonly used mappings include:
- Basic Types: strings, ints, doubles, and booleans.
- Transient Types: fields that will not be persisted to the database.
- Embeddable Types: complex types that can be embedded into an entity.
- Collections: lists, sets, and maps used to manage collections of entities.
Each mapping type comes with its own set of challenges and nuances. The following sections discuss common pitfalls associated with these types.
1. Basic Type Pitfalls
Pitfall: Implicit Type Conversion
Hibernate automatically performs type conversions between Java types and SQL types. However, implicit conversions can lead to unexpected behavior, especially with string and numeric types.
@Entity
public class Student {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private int age;
// getters and setters
}
Why It Matters: If the database stores 'age' as a string instead of an integer, retrieving the value may throw a ClassCastException
. Always validate your database schema against your entity definitions.
Best Practice
- Ensure that the data types in your database match the corresponding Java types to avoid conversion errors.
2. Transient Type Pitfalls
Pitfall: Missing Transient Annotation
Not marking a field as transient can lead to unintentional persistence of sensitive information.
@Entity
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@Transient
private String password;
// getters and setters
}
Why It Matters: The @Transient
annotation instructs Hibernate to ignore the marked field, preventing it from being stored in the database. Forgetting this annotation can expose sensitive information.
Best Practice
- Always use the
@Transient
annotation for fields that should not be persisted and double-check your entity configurations.
3. Embeddable Type Pitfalls
Pitfall: Ignoring Equality and Hashing Methods
When using embeddable types, developers often forget to override equals()
and hashCode()
methods.
@Embeddable
public class Address {
private String street;
private String city;
// Override equals() and hashCode()
}
Why It Matters: Hibernate uses these methods to determine if instances are the same. Not overriding them can lead to errors when Hibernate tries to manage collections of entities.
Best Practice
- Always implement
equals()
andhashCode()
methods in your embeddable types to ensure reliable entity management.
4. Collection Mapping Pitfalls
Pitfall: Unmanaged Collections
Not initializing collections can lead to NullPointerExceptions
.
@Entity
public class Course {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@OneToMany(mappedBy = "course")
private List<Student> students;
// Ensure initialization
public Course() {
this.students = new ArrayList<>();
}
}
Why It Matters: If the students
list is null, attempting to add or retrieve elements will cause runtime exceptions.
Best Practice
- Always initialize collections in your entity classes to prevent null reference issues.
5. Mapping Relationships Pitfalls
Pitfall: The Cascade Type Confusion
Using the wrong cascade type can lead to unintended deletions or updates in related entities.
@Entity
public class Parent {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@OneToMany(mappedBy = "parent", cascade = CascadeType.ALL)
private List<Child> children;
}
Why It Matters: Setting cascade = CascadeType.ALL
means that any delete operation on Parent
will also delete all associated Child
entities, which may not be the intended behavior.
Best Practice
- Use appropriate cascade types for your relationship mappings to ensure that data management aligns with application requirements. For example, consider using
CascadeType.PERSIST
for operations where you only want to save, not delete or update.
A Final Look
Mastering Hibernate entails understanding its mapping types and recognizing potential pitfalls. By remaining vigilant about common mistakes and implementing best practices, you can ensure more robust and efficient database interactions in your Java applications.
Additional Resources
For further learning about Hibernate and its capabilities, consider exploring the following resources:
- Hibernate Official Documentation
- Java Persistence API (JPA)
- Baeldung’s Hibernate Tutorials
With knowledge of these pitfalls and proactive strategies to avoid them, you'll be well on your way to becoming a Hibernate expert! Happy coding!