Common HQL Mistakes That Will Sabotage Your Queries
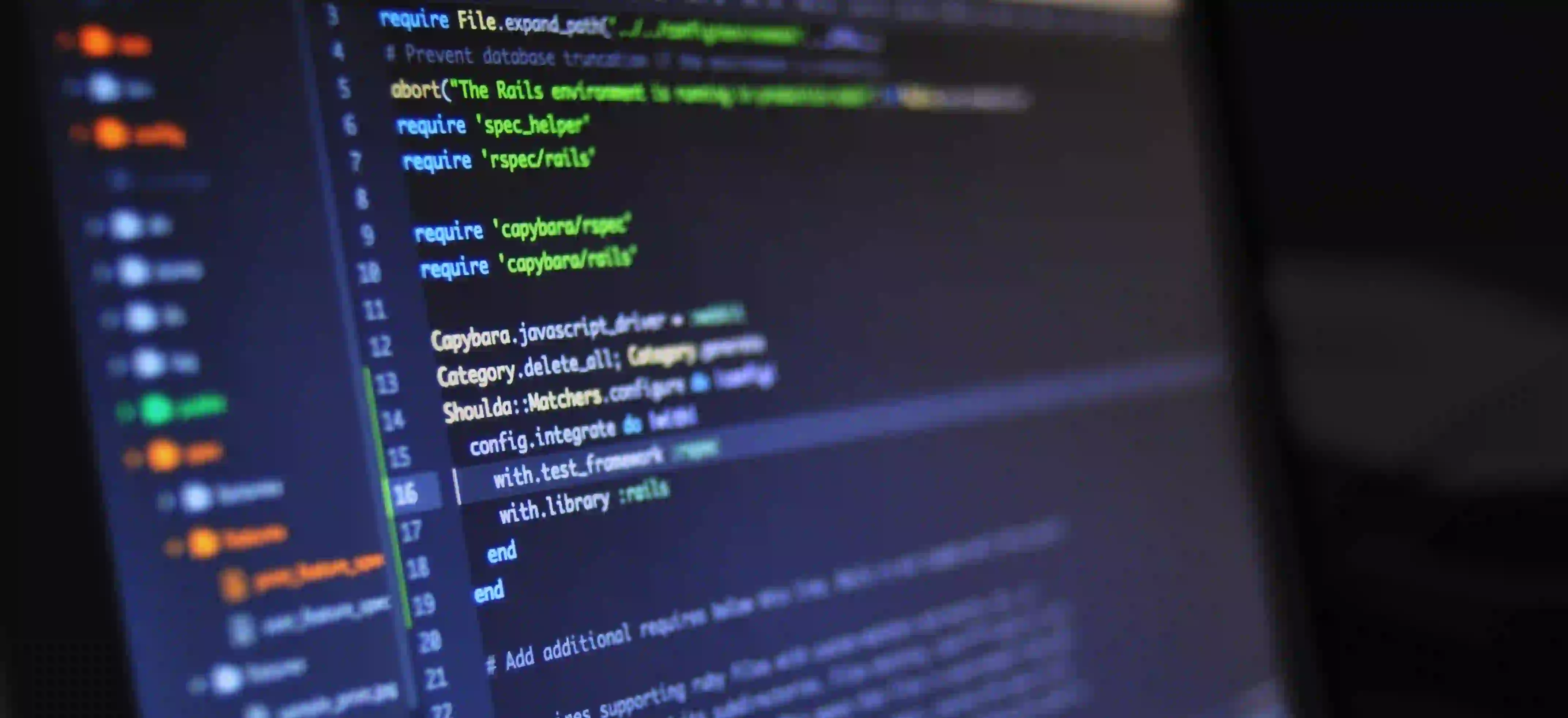
Common HQL Mistakes That Will Sabotage Your Queries
As developers, we often strive to create efficient and effective code. However, it's easy to fall into common pitfalls when using HQL (Hibernate Query Language). HQL allows you to execute database queries in a way that is more object-oriented and closely aligned with Java code. Striving for optimization and clarity in your queries is crucial to the performance and maintainability of your applications.
In this blog post, we'll explore the most common HQL mistakes that can derail your queries. We will also provide guidance on how to avoid them and improve your code quality.
Understanding HQL
Before diving into the mistakes, let's clarify what HQL is. HQL is not SQL (Structured Query Language); rather, it is an object-oriented query language designed for Hibernate. HQL directly operates on the entity classes rather than tables, which makes it more versatile in dealing with complex data relationships in a Java-centric way.
HQL is powerful, but if not used correctly, it can lead to poorly optimized queries and unexpected results. Below, we'll go through the key mistakes often seen in HQL along with strategies to avoid them.
1. Not Using Named Parameters
Mistake
Using positional parameters instead of named parameters can lead to confusion and bugs.
Example
String hql = "FROM Employee e WHERE e.department.id = ?0";
Query query = session.createQuery(hql);
query.setParameter(0, departmentId);
Why It's a Problem
In this example, the positional parameter can be hard to track, especially as queries get more complex. If you accidentally change the order of parameters, it can break your code without any clear indication.
Solution
Use named parameters for clarity:
String hql = "FROM Employee e WHERE e.department.id = :deptId";
Query query = session.createQuery(hql);
query.setParameter("deptId", departmentId);
Using named parameters makes the code easier to read and maintain.
2. Forgetting to Handle Null Values
Mistake
Not considering null values can lead to unexpected behavior in queries.
Example
String hql = "FROM Employee e WHERE e.name = :name";
Query query = session.createQuery(hql);
query.setParameter("name", name);
Why It's a Problem
If the name
variable is null, your query may not return any results even if there are employees with null names.
Solution
You can handle null values explicitly in your HQL query by adding a conditional check:
String hql = "FROM Employee e WHERE (:name IS NULL OR e.name = :name)";
Query query = session.createQuery(hql);
query.setParameter("name", name);
This ensures that your query will succeed even when the input is null.
3. Neglecting JOIN FETCH
for Eager Loading
Mistake
Failing to use JOIN FETCH
for eagerly loading associated entities can lead to the N+1 select problem, which hampers performance.
Example
String hql = "FROM Department d";
List<Department> departments = session.createQuery(hql).list();
Why It's a Problem
In this case, if each department has a collection of employees that you need to load, Hibernate will issue another query for each department to get the employees, leading to N+1 queries.
Solution
Utilize JOIN FETCH
for eager loading:
String hql = "FROM Department d JOIN FETCH d.employees";
List<Department> departments = session.createQuery(hql).list();
This way, it fetches both departments and their employees in just two queries, significantly boosting performance.
4. Ignoring Pagination
Mistake
Not implementing pagination in your queries can result in loading all results into memory, which might overwhelm your application.
Example
String hql = "FROM Employee";
List<Employee> employees = session.createQuery(hql).list();
Why It's a Problem
Retrieving all employee records can consume a lot of memory, impeding your application's performance.
Solution
Use pagination to limit the results returned:
String hql = "FROM Employee";
Query query = session.createQuery(hql);
query.setFirstResult(0);
query.setMaxResults(10);
List<Employee> employees = query.list();
This way, you only load a manageable number of records into memory, reducing overall resource consumption.
5. Misusing DISTINCT
Mistake
Improper use of the DISTINCT
keyword can lead to unexpected results.
Example
String hql = "SELECT DISTINCT e FROM Employee e JOIN e.projects p WHERE p.name = :projectName";
Query query = session.createQuery(hql);
query.setParameter("projectName", projectName);
Why It's a Problem
Using DISTINCT
can often get omitted when dealing with fetched associations because Hibernate may give misleading distinct results.
Solution
Take caution and structure your queries thoughtfully to avoid logical errors:
String hql = "SELECT e FROM Employee e JOIN e.projects p WHERE p.name = :projectName";
Query query = session.createQuery(hql);
query.setParameter("projectName", projectName);
Understanding how DISTINCT
operates in HQL can improve both accuracy and performance.
6. Not Understanding Implicit vs. Explicit Joins
Mistake
Confusing implicit joins with explicit joins can create inefficiencies and complexity in your code.
Example
String hql = "FROM Employee e WHERE e.department.name = 'Engineering'";
Why It's a Problem
Although this works, it results in implicit joins, which can produce more SQL calls than necessary.
Solution
Using explicit joins when needed enhances clarity and control:
String hql = "SELECT e FROM Employee e JOIN e.department d WHERE d.name = 'Engineering'";
This approach provides better control over the SQL generated and can lead to performance optimizations.
7. Failing to Optimize Queries
Mistake
Not optimizing HQL queries can create bottlenecks in large applications.
Example
String hql = "FROM Employee e WHERE e.salary > :salary";
List<Employee> employees = session.createQuery(hql)
.setParameter("salary", lowSalary)
.list();
Why It's a Problem
Without indexing on the salary
field, this query could be inefficient on a large dataset.
Solution
Always analyze queries and test performance by leveraging SQL indexes and Hibernate’s @Where
or @Filter
annotations.
My Closing Thoughts on the Matter
HQL is a powerful tool when utilized effectively, but it is crucial to be aware of the common mistakes that can hinder your queries. By employing best practices such as using named parameters, properly handling nulls, optimizing joins, and implementing pagination, you can write cleaner, more efficient code.
If you're looking for additional resources on HQL or Hibernate, consider checking out the official Hibernate documentation or various community forums.
By avoiding these common HQL mistakes, you can ensure that your queries run smoothly, perform well, and remain maintainable over the long run. Happy coding!