Common Maven Errors and How to Fix Them Quickly
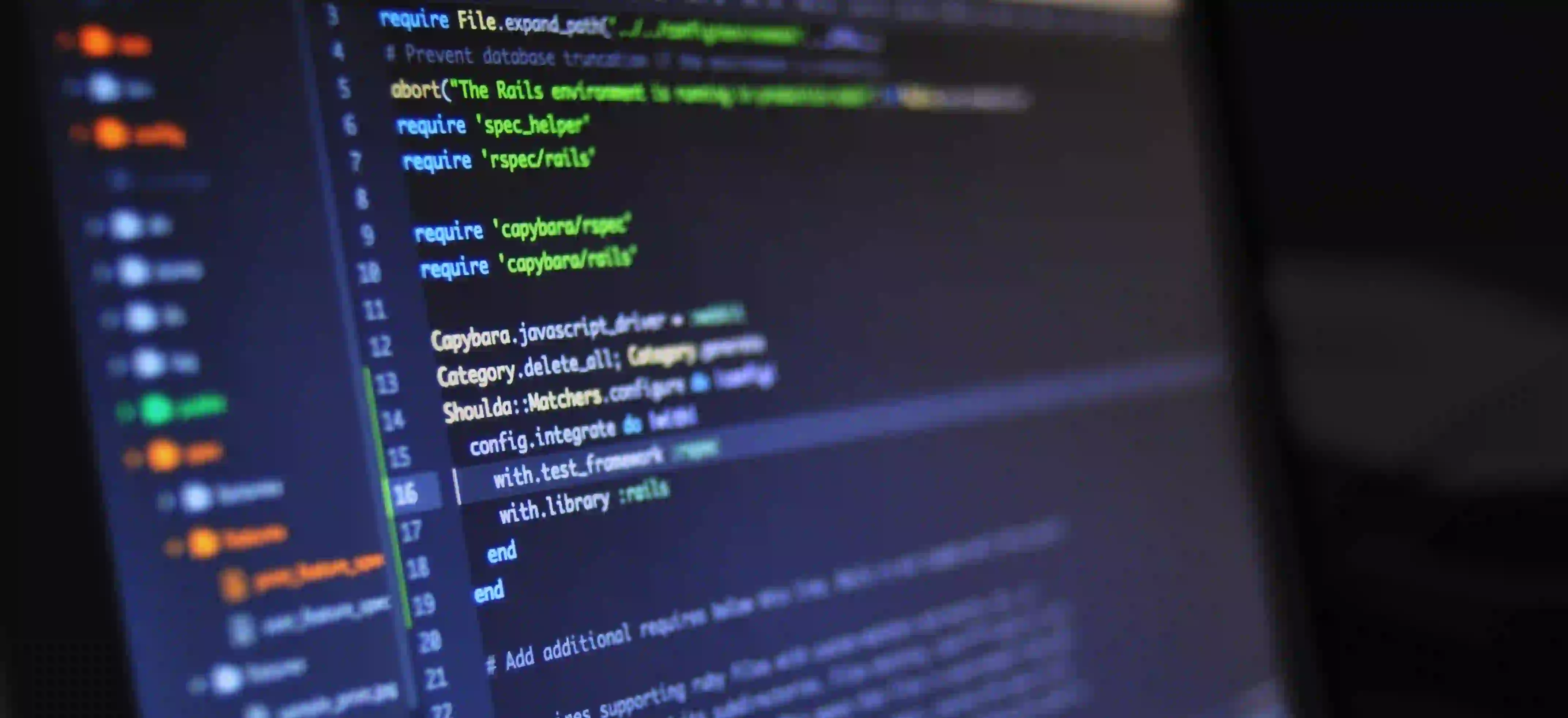
Common Maven Errors and How to Fix Them Quickly
Maven is a powerful build automation tool predominantly used for Java projects. Its widespread adoption is due to its ability to manage project dependencies and build lifecycles effectively. However, like any robust tool, Maven can come with its share of errors that can halt your development workflow. This guide outlines common Maven errors, offering insightful solutions to get you back on track quickly.
Table of Contents
Understanding Maven
Before diving into error resolutions, it's essential to understand how Maven works. Maven utilizes a project object model (POM) file (pom.xml
) to manage project structure, dependencies, build processes, and execution of plugins. It's crucial for you to maintain a clear and organized structure within your POM to minimize potential issues.
Explore Maven's official documentation for a more detailed understanding of its functionalities.
Common Maven Errors
1. Dependency Resolution Errors
Problem: Missing or incorrect dependencies that cannot be resolved.
When you encounter messages like "Could not find artifact" or "Failure to find," it indicates that Maven cannot access the specified dependencies.
Solution:
-
Ensure proper declaration of dependencies in the
pom.xml
. Here’s an example:📄snippet.txt<dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>5.3.8</version> </dependency>
-
Check your internet connection and the dependency repository settings in your
settings.xml
or directly in the POM. -
Utilize the command
mvn clean install
to refresh your dependencies.
2. Plugin Execution Errors
Problem: Errors related to plugin management, such as "Could not find goal" or "Plugin execution not covered by lifecycle."
Plugins in Maven are crucial for various build tasks. If you encounter any execution errors, it's typically due to misconfigured plugins in the POM.
Solution:
-
Verify that the plugins are correctly declared in the
pom.xml
. For example:📄snippet.txt<build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.1</version> <configuration> <source>1.8</source> <target>1.8</target> </configuration> </plugin> </plugins> </build>
-
Ensure the version of the plugin is correct and that it exists in a repository Maven can access.
3. Lifecycle Phase Errors
Problem: Errors like "No goals specified" or "Project build failed" indicate that Maven is struggling to execute the defined lifecycle phases.
Maven has several lifecycle phases, such as validate
, compile
, test
, and package
. Misconfigurations can lead to lifecycle failures.
Solution:
-
Always specify the goals when running Maven from the command line, such as:
🔧snippet.shmvn clean package
-
You can also set the lifecycle phases in the
pom.xml
by ensuring that all required plugins and dependencies are included.
4. Local Repository Issues
Problem: Conflicts or outdated files in your local Maven repository can cause build issues.
If you see errors referencing "Local repository" or "Unable to resolve," it’s often related to your local .m2
repository being in a bad state.
Solution:
-
Clear your local repository by deleting the directory at
~/.m2/repository
. -
Use the command:
🔧snippet.shmvn clean install -U
The
-U
flag forces Maven to update snapshots and releases.
5. Version Conflicts
Problem: When multiple versions of the same library are pulled due to different dependency requirements, it can lead to runtime errors.
Conflicts might result in ClassNotFoundExceptions or NoSuchMethodErrors while running applications.
Solution:
-
Analyze the dependencies using the command:
🔧snippet.shmvn dependency:tree
-
Identify conflicting versions and explicitly declare the required version in your
pom.xml
:📄snippet.txt<dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.13.0</version> </dependency>
Best Practices to Prevent Maven Errors
- Regularly Update Dependencies: Keeping your dependencies up-to-date helps avoid conflicts and deprecated issues.
- Maintain a Clean Local Repository: Periodically clean your local repository to prevent conflicts.
- Organize POM Files: Use proper indentation and comments in your
pom.xml
for ease of debugging. - Use Parent POMs: If multiple projects share dependencies, consider a parent POM for easier management.
- Version Management Tools: Consider tools like Dependabot to manage and automate dependency updates.
Closing the Chapter
Maven is an exceptional tool that can significantly enhance your Java development process. Knowledge of common errors and solutions can save you valuable time and frustration. By adhering to best practices, you can minimize potential issues, enabling a smoother development experience.
For further reading, check out Maven Guides to expand your knowledge and expertise.
Happy coding!