Common JUnit 5 Pitfalls and How to Avoid Them
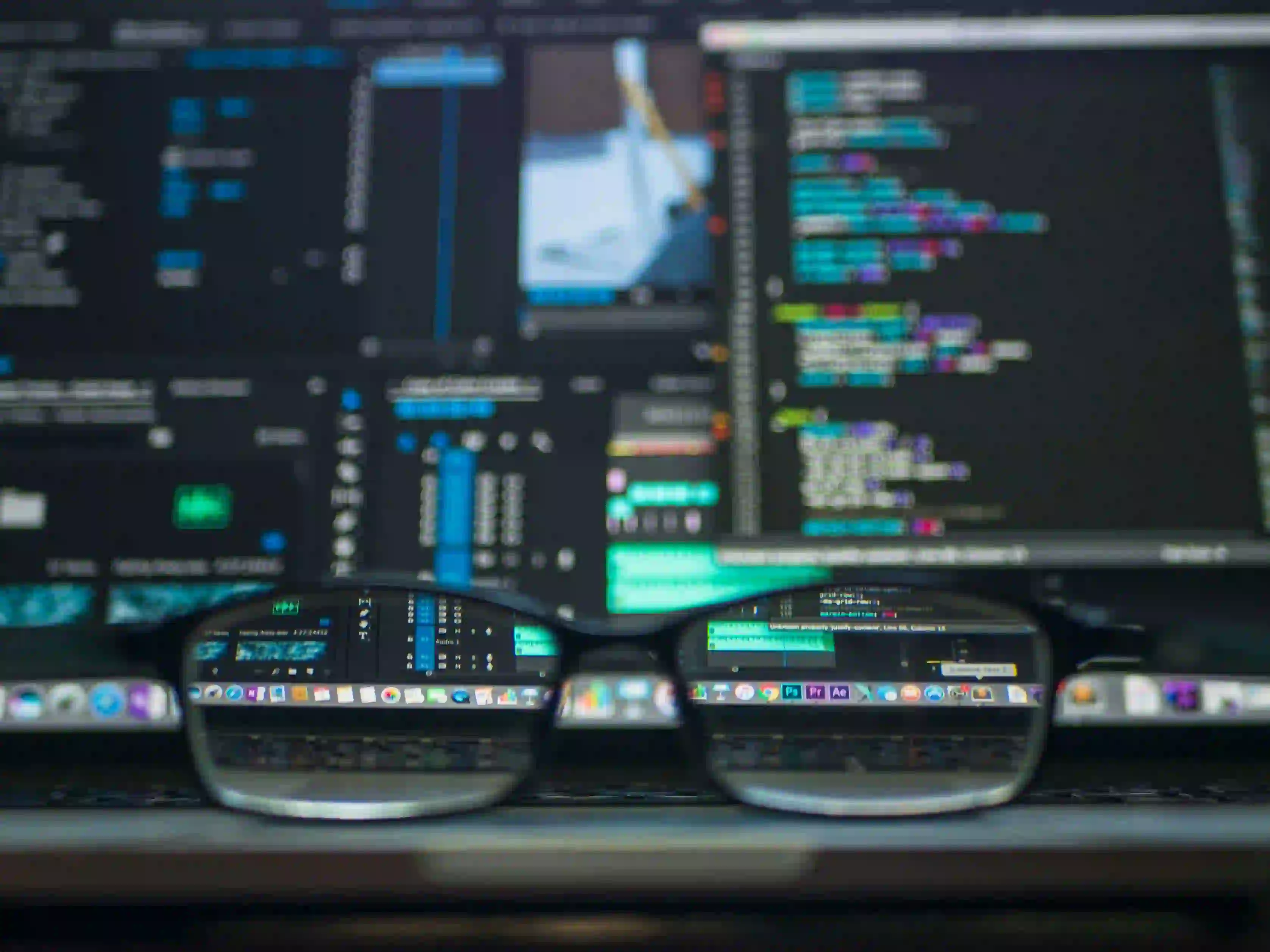
Common JUnit 5 Pitfalls and How to Avoid Them
JUnit 5 is a powerful and flexible testing framework for Java applications. While it builds on the solid foundations of its predecessor (JUnit 4), it introduces many new features and enhancements. This blog post explores some common pitfalls developers may encounter when using JUnit 5 and provides tips on how to avoid these issues.
Table of Contents
- Understanding the Architecture of JUnit 5
- Incorrectly Configured Test Suites
- Ignoring Dependency Management
- Misusing Annotations
- Not Taking Advantage of the Parameterized Tests
- Lack of Proper Assertions
- Overlooking Test Lifecycle Callbacks
- Conclusion
Understanding the Architecture of JUnit 5
Before diving into common pitfalls, it's crucial to understand JUnit 5's architecture. JUnit 5 is comprised of three primary components:
- JUnit Platform: The foundation for launching testing frameworks on the Java Virtual Machine.
- JUnit Jupiter: The programming model and extension model for writing tests in JUnit 5.
- JUnit Vintage: This component provides support for running JUnit 3 and JUnit 4 tests on the JUnit 5 platform.
Understanding this modular structure helps avoid misunderstandings and improper configurations.
Incorrectly Configured Test Suites
Pitfall: Many developers struggle with configuring test suites correctly.
Solution: Use the @Suite
annotation with a clear and concise setup.
Here's how to properly configure a test suite:
import org.junit.platform.suite.api.IncludeEngines;
import org.junit.platform.suite.api.SelectPackages;
import org.junit.platform.suite.api.Suite;
@IncludeEngines("junit-vintage") // Include Vintage engine
@SelectPackages("com.example.tests") // Specify package for tests
@Suite
public class MyTestSuite {
}
Why: Proper configuration ensures that all relevant tests are executed, helping avoid missed or failed tests due to incorrect package selections.
Ignoring Dependency Management
Pitfall: Failing to manage dependencies can lead to compatibility issues.
Solution: Ensure that you are using the correct version of JUnit 5 and related libraries. Here’s a sample pom.xml
dependency setup for Maven:
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter</artifactId>
<version>5.8.1</version>
<scope>test</scope>
</dependency>
Why: Managing dependencies properly helps maintain the application's reliability and performance, avoiding classpath issues that may arise when running tests.
Misusing Annotations
Pitfall: Annotations in JUnit 5 offer powerful functionality, but they can be easily misused.
Solution: Understand the differences among the annotations:
@BeforeEach
vs.@BeforeAll
: Use@BeforeEach
for setup tasks that need to happen before every test, while@BeforeAll
runs once for the entire class.
Example:
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.BeforeAll;
public class MyTests {
@BeforeAll
static void initAll() {
System.out.println("Runs once before all tests");
}
@BeforeEach
void init() {
System.out.println("Runs before each test");
}
}
Why: Misusing these annotations can lead to inefficient setups, causing longer test runs and unclear test results.
Not Taking Advantage of the Parameterized Tests
Pitfall: Many developers don’t utilize parameterized tests due to a lack of understanding.
Solution: Use parameterized tests effectively to run the same test with different inputs using @ParameterizedTest
.
Example:
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.ValueSource;
class ParameterizedTests {
@ParameterizedTest
@ValueSource(ints = { 1, 2, 3 })
void testWithParameter(int number) {
System.out.println(number);
assertTrue(number > 0);
}
}
Why: Parameterized tests can reduce redundancy in tests by allowing you to test multiple cases with different data inputs, thereby increasing coverage and clarity.
Lack of Proper Assertions
Pitfall: Developers sometimes write ineffective assertions that do not adequately test their methods.
Solution: Utilize the assertions provided by JUnit and ensure they are meaningful.
Example:
import static org.junit.jupiter.api.Assertions.*;
class MyAssertions {
@Test
void testAddition() {
int result = add(2, 3);
assertNotNull(result, "The result should not be null");
assertEquals(5, result, "2 + 3 should equal 5");
}
int add(int a, int b) {
return a + b;
}
}
Why: Proper assertions create more reliable tests, clearly reflecting the conditions you are validating and the expected outcomes.
Overlooking Test Lifecycle Callbacks
Pitfall: Failing to use lifecycle callbacks can lead to tests that are difficult to manage.
Solution: Utilize lifecycle methods to set up and tear down your test environment properly.
Example:
import org.junit.jupiter.api.AfterEach;
import org.junit.jupiter.api.BeforeEach;
class LifecycleTests {
@BeforeEach
void setup() {
System.out.println("Setup before each test");
}
@AfterEach
void teardown() {
System.out.println("Teardown after each test");
}
@Test
void sampleTest() {
// Your test code here
}
}
Why: Lifecycle methods provide a structured way to manage test setup and teardown, leading to increased test reliability.
Key Takeaways
JUnit 5 offers a robust framework for Java testing, but pitfalls can arise if care is not taken. By understanding the architecture, correctly configuring test suites, managing dependencies, utilizing annotations properly, leveraging parameterized tests, ensuring effective assertions, and overseeing lifecycle callbacks, developers can create more reliable, maintainable tests.
For additional tips and examples, check out the JUnit 5 User Guide for in-depth explanations and best practices. Happy testing!