Top BDD Challenges in Cucumber for Java Developers
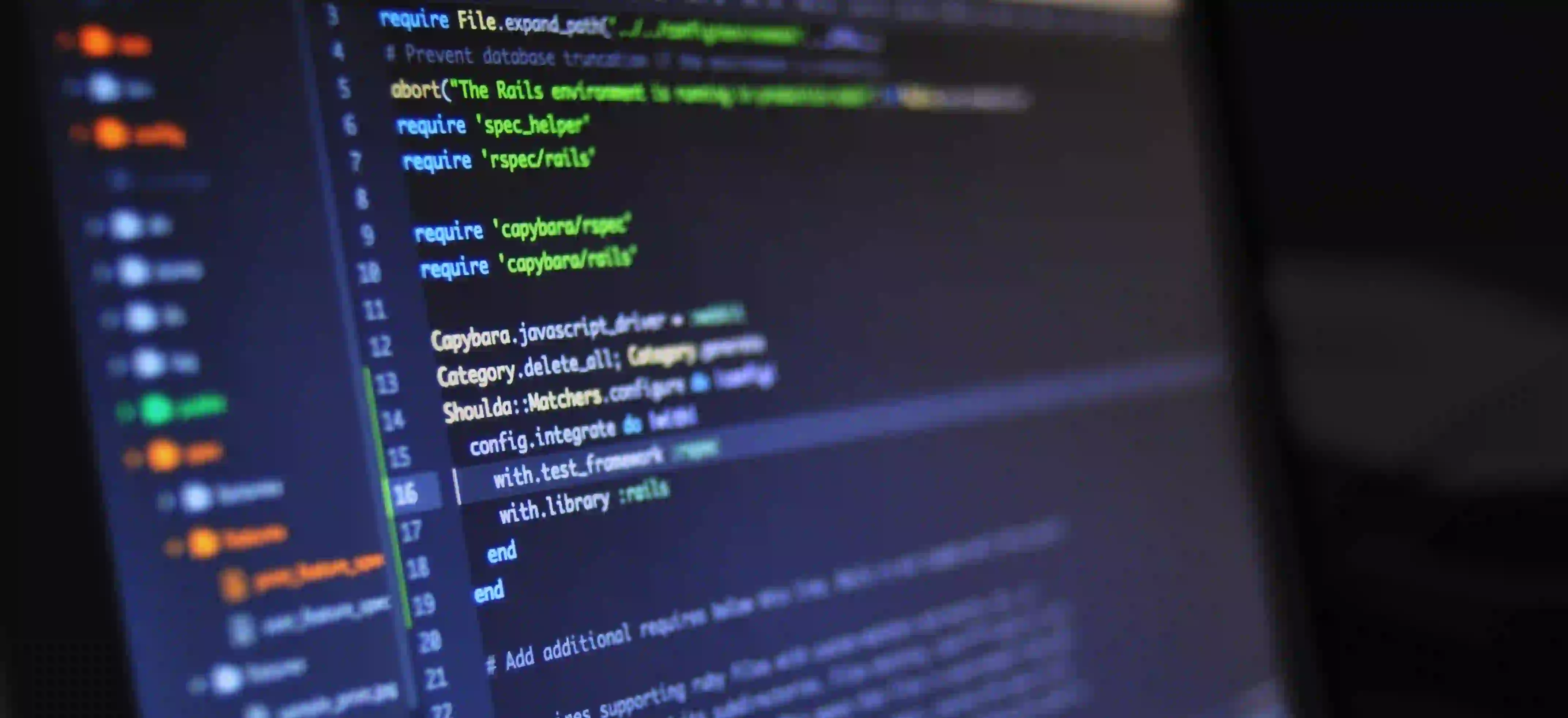
Top BDD Challenges in Cucumber for Java Developers
Behavior-Driven Development (BDD) has become a significant methodology for software development, helping teams to collaborate more effectively. Cucumber, as a popular tool for implementing BDD, allows developers to write specifications in natural language. However, while Cucumber enhances collaboration between developers and other stakeholders, it also comes with its challenges. This blog post will delve into the top BDD challenges that Java developers face when integrating Cucumber into their workflows.
1. Understanding Gherkin Syntax
The Challenge
Gherkin is the language used to write BDD scenarios in Cucumber. For many Java developers, transitioning from traditional programming constructs to writing in Gherkin can be challenging. This syntax is not like standard programming languages, and understanding how to structure scenarios properly is crucial.
Solution
To overcome this challenge, developers should invest time in understanding Gherkin's keywords and syntax, such as Given
, When
, Then
, And
, and But
. Mastering these keywords will help in writing effective scenarios.
Example: A Simple Gherkin Scenario
Feature: Login functionality
Scenario: Successful login with valid credentials
Given the user is on the login page
When the user enters valid credentials
Then the user should be redirected to the dashboard
By adopting a habit of writing scenarios early on, developers will become comfortable with the Gherkin syntax.
2. Creating Meaningful Scenarios
The Challenge
One of the core BDD principles is to create scenarios that are meaningful and provide value to stakeholders. However, Java developers often struggle to connect business requirements to clear, concise scenarios. This disconnect can lead to poorly written tests that do not reflect user needs.
Solution
Collaboration with business analysts and stakeholders is essential. Regular discussions can lead to a clearer understanding of requirements and help craft scenarios that accurately reflect user expectations.
Key Takeaway: Always involve stakeholders when writing scenarios to ensure that they are grounded in real-world user behavior.
3. Maintaining Step Definitions
The Challenge
As projects grow, maintaining step definitions becomes increasingly complex. Over time, duplicate definitions or rapidly changing test requirements can lead to a cluttered codebase that is hard to debug and manage.
Solution
Implementing a clear structure for organizing step definitions can reduce complexity. Java developers should follow best practices, such as grouping related step definitions and reusing existing steps wherever possible.
Example: Step Definition Organization
import io.cucumber.java.en.Given;
import io.cucumber.java.en.When;
import io.cucumber.java.en.Then;
public class LoginSteps {
@Given("the user is on the login page")
public void userOnLoginPage() {
// Navigate to login page
System.out.println("Navigating to Login Page");
}
@When("the user enters valid credentials")
public void userEntersCredentials() {
// Simulate user entering credentials
System.out.println("Entering credentials");
}
@Then("the user should be redirected to the dashboard")
public void userRedirectedToDashboard() {
// Check redirection
System.out.println("User redirected to Dashboard");
}
}
By keeping step definitions organized and well-documented, Java developers can maintain clarity and efficiency in their tests.
4. Handling Test Data
The Challenge
Testing often requires specific data to evaluate functionality accurately. In Cucumber, managing test data effectively can be problematic. Hardcoding data into tests leads to maintenance headaches, while inconsistent data sources can yield unreliable test results.
Solution
Utilizing external data sources such as JSON files, CSV files, or databases allows for more manageable and dynamic test data. This practice not only improves maintainability but also enhances the robustness of the tests.
Example: Using JSON for Test Data
{
"validUser": {
"username": "testUser",
"password": "testPass"
}
}
Java code to load this data:
import com.fasterxml.jackson.databind.ObjectMapper;
public class TestDataLoader {
public static Map<String, String> loadData(String jsonFilename) {
ObjectMapper objectMapper = new ObjectMapper();
try {
return objectMapper.readValue(new File(jsonFilename), new TypeReference<Map<String, String>>() {});
} catch (IOException e) {
e.printStackTrace();
}
return new HashMap<>();
}
}
By separating test data from the logic, developers can streamline changes without impacting test functionality.
5. Ensuring Test Independence
The Challenge
BDD emphasizes comprehensive scenario coverage; however, ensuring that each test is independent can be daunting. Tests that rely on shared states can lead to flaky results, making them unreliable.
Solution
Adopt best practices such as setting up and tearing down your test environment for each scenario. Utilize the @Before and @After hooks in Cucumber to ensure a clean state for each execution.
Example: Using Hooks for Test Independence
import io.cucumber.java.Before;
import io.cucumber.java.After;
public class Hooks {
@Before
public void setup() {
// Setup the test environment
System.out.println("Setting up the test environment");
}
@After
public void tearDown() {
// Clean up after test execution
System.out.println("Tearing down the test environment");
}
}
By ensuring each test runs independently, Java developers can significantly improve the reliability of their BDD tests.
6. Integrating with CI/CD
The Challenge
As projects shift towards continuous integration and continuous deployment (CI/CD), integrating Cucumber tests into the CI/CD pipeline presents challenges. Ensuring that tests run effectively and produce actionable feedback to the development team is crucial.
Solution
Developers should create automated scripts to run Cucumber tests on build servers. These scripts can be integrated into Jenkins, GitLab CI, or similar tools to provide real-time feedback.
Example: Jenkins Pipeline for Cucumber Tests
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean install'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
}
}
This ensures that your Cucumber tests are run automatically with every build, streamlining the feedback process.
Final Considerations
While BDD with Cucumber offers numerous benefits, it is not without its challenges. Java developers must navigate the intricacies of Gherkin syntax, maintain clear and meaningful scenarios, manage step definitions, test data, test independence, and CI/CD integration.
By adopting best practices in step definitions, utilizing external data sources, and collaborating with stakeholders, developers can overcome these challenges and leverage Cucumber to its fullest potential. For further reading on mastering BDD with Cucumber, you can explore the Cucumber Documentation.
In summary, embracing the complexities of BDD while focusing on collaboration can lead to successful software projects that truly meet user needs.