Overcoming Kubernetes Complexity for Java Developers
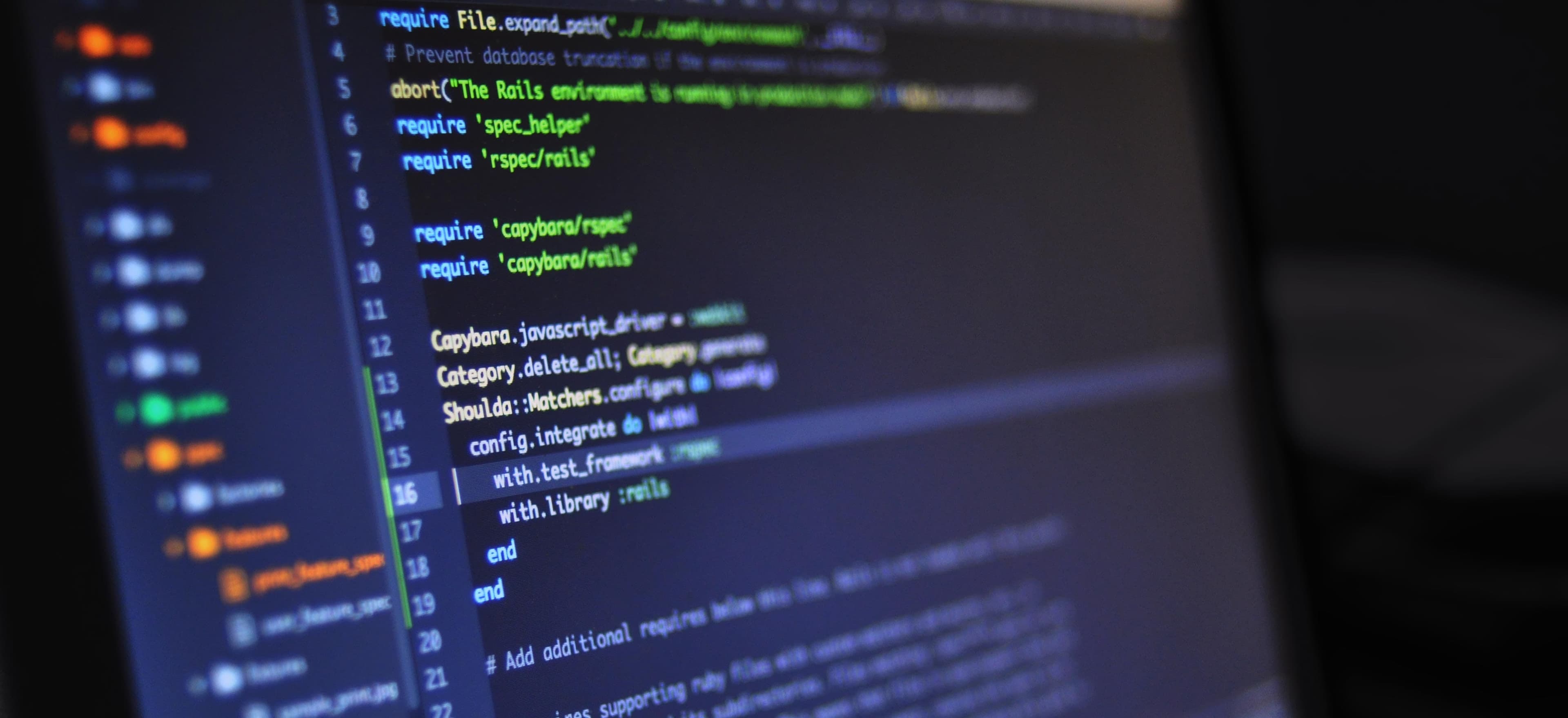
- Published on
Overcoming Kubernetes Complexity for Java Developers
Kubernetes has quickly become the go-to orchestration platform for deploying applications in a cloud-native environment. While this power brings enormous flexibility and scalability, it can also introduce a layer of complexity, especially for Java developers who may not be accustomed to such container orchestration.
In this post, we will explore how Java developers can overcome the complexities of Kubernetes, simplifying the deployment process while maintaining robust application performance.
Understanding Kubernetes Basics
Before diving into the complexities, it is crucial to have a clear understanding of what Kubernetes is and how it interacts with Java applications.
What is Kubernetes?
Kubernetes (often abbreviated as K8s) is an open-source platform that automates deploying, scaling, and operating application containers. It provides a framework for running distributed systems resiliently—aiming to manage containerized applications in a cluster of machines.
Key Concepts of Kubernetes
- Pods: The smallest deployable units that can contain one or more containers. Pods manage the lifecycle of these containers.
- Services: Define how to access your pods, facilitating communication between your applications.
- Deployments: Manage the state of pods, allowing for easy scaling and updates.
- Namespaces: Organize resources in a way that allows segregation by user or team.
Link to Further Reading
For a deeper understanding of Kubernetes architecture, see the official Kubernetes documentation.
Why Use Kubernetes for Java Applications?
Java applications have unique advantages when deployed on Kubernetes:
- Scalability: Kubernetes allows you to scale your Java microservices seamlessly.
- Load Balancing: Efficiently manages network traffic to your Java applications.
- Self-Healing: Automatically replaces failed pods, reducing downtime.
However, the initial complexity can seem daunting. Let's explore how to mitigate that.
Kubernetes Complexity: Common Challenges
- Configuration Management: YAML configurations can become unwieldy.
- Networking: Understanding pod communication across different services.
- Monitoring and Logging: Maintaining observational data from multiple pods and services.
Overcoming Configuration Complexity
The Power of Helm
Helm is a package manager for Kubernetes that simplifies application deployment. Using Helm charts allows you to manage complex Kubernetes configurations more easily.
# Install Helm
brew install helm
Once Helm is set up, you can quickly deploy your Java application by using an existing chart or creating your own. Below is a simple example.
Sample Helm Chart Structure
my-java-app/
├── Chart.yaml
├── templates/
│ ├── deployment.yaml
│ └── service.yaml
└── values.yaml
Why Use Helm?
- Version Control: You can track your application's configurations across versions.
- Easy Upgrades: Installing or rolling back applications becomes straightforward.
- Reusable Templates: Templating saves time and minimizes human error.
Creating Helm Templates
Here’s a simple example of a Helm deployment template for a Java Spring Boot application:
apiVersion: apps/v1
kind: Deployment
metadata:
name: {{ .Release.Name }}
spec:
replicas: {{ .Values.replicaCount }}
selector:
matchLabels:
app: {{ .Release.Name }}
template:
metadata:
labels:
app: {{ .Release.Name }}
spec:
containers:
- name: {{ .Release.Name }}
image: "{{ .Values.image.repository }}:{{ .Values.image.tag }}"
ports:
- containerPort: {{ .Values.service.port }}
Explanation:
- The template uses placeholders for values defined in
values.yaml
. - This promotes reuse and makes updates easier if any configurations change.
Link to Additional Resources
To learn more about Helm's capabilities, visit the Helm Documentation.
Simplifying Networking Challenges
Services and Ingress
For Java applications, especially when microservices are involved, managing service communication is vital. Kubernetes Services expose your pods to other services and the external network.
Example of a Service Manifest
kind: Service
apiVersion: v1
metadata:
name: my-java-service
spec:
selector:
app: my-java-app
ports:
- protocol: TCP
port: 80
targetPort: 8080
Why Define a Service?
- It abstracts the underlying pod IP addresses, providing a stable interface for communication.
- It facilitates load balancing, improving availability.
Using Ingress Controllers
For managing external access to your Java application, an Ingress can be utilized. This manages HTTP and HTTPS traffic and can route requests to your application based on defined rules.
Example Ingress Resource
apiVersion: networking.k8s.io/v1
kind: Ingress
metadata:
name: my-java-ingress
spec:
rules:
- host: my-java-app.example.com
http:
paths:
- path: /
pathType: Prefix
backend:
service:
name: my-java-service
port:
number: 80
Advantages of Using Ingress:
- Simplified routing mechanism for microservices.
- SSL Termination for secure communication.
- Traffic management features such as rate limiting and authentication.
Monitoring and Logging: Essential Aspects
To address monitoring and logging complexities, tools like Prometheus and Grafana are widely used in the Kubernetes ecosystem to acquire observability on Java applications.
Implementing Monitoring
- Prometheus: A powerful open-source monitoring tool.
To install Prometheus, you can use Helm:
helm install prometheus stable/prometheus
- Grafana: A powerful visualization tool that takes metrics from Prometheus and allows for beautiful dashboards.
Example of Adding Prometheus Metrics in Java
Using the Micrometer library, you can expose metrics directly from your Java application:
import io.micrometer.core.instrument.Metrics;
import io.micrometer.core.instrument.Counter;
public class MyApplication {
private static final Counter counter = Metrics.counter("my_custom_counter");
public static void main(String[] args) {
counter.increment();
// Application logic...
}
}
Why Micrometer?
- Integrates seamlessly with Spring Boot applications.
- Provides built-in support for various monitoring systems like Prometheus, InfluxDB, etc.
- Allows you to instrument your application effortlessly.
Bringing It All Together
As a Java developer venturing into Kubernetes, embracing the right tools and practices is crucial for overcoming complexity. Helm streamlines configuration management, Services and Ingress make networking easier, and tools like Prometheus and Grafana bring observability to your applications.
Breaking down Kubernetes complexity doesn't mean compromising on power; instead, it empowers Java developers to take full advantage of modern cloud-native architectures. With practice, collaboration, and the right resources, you can confidently deploy and manage your Java applications in a Kubernetes environment.
Further Reading
- Kubernetes for Java Developers on Baeldung
- Building Microservices with Spring Boot and Kubernetes
With these tools and concepts, you can turn your Kubernetes journey from a daunting challenge into an exciting opportunity for growth and innovation. Happy coding!