Common Mistakes When Dockerizing Java Applications
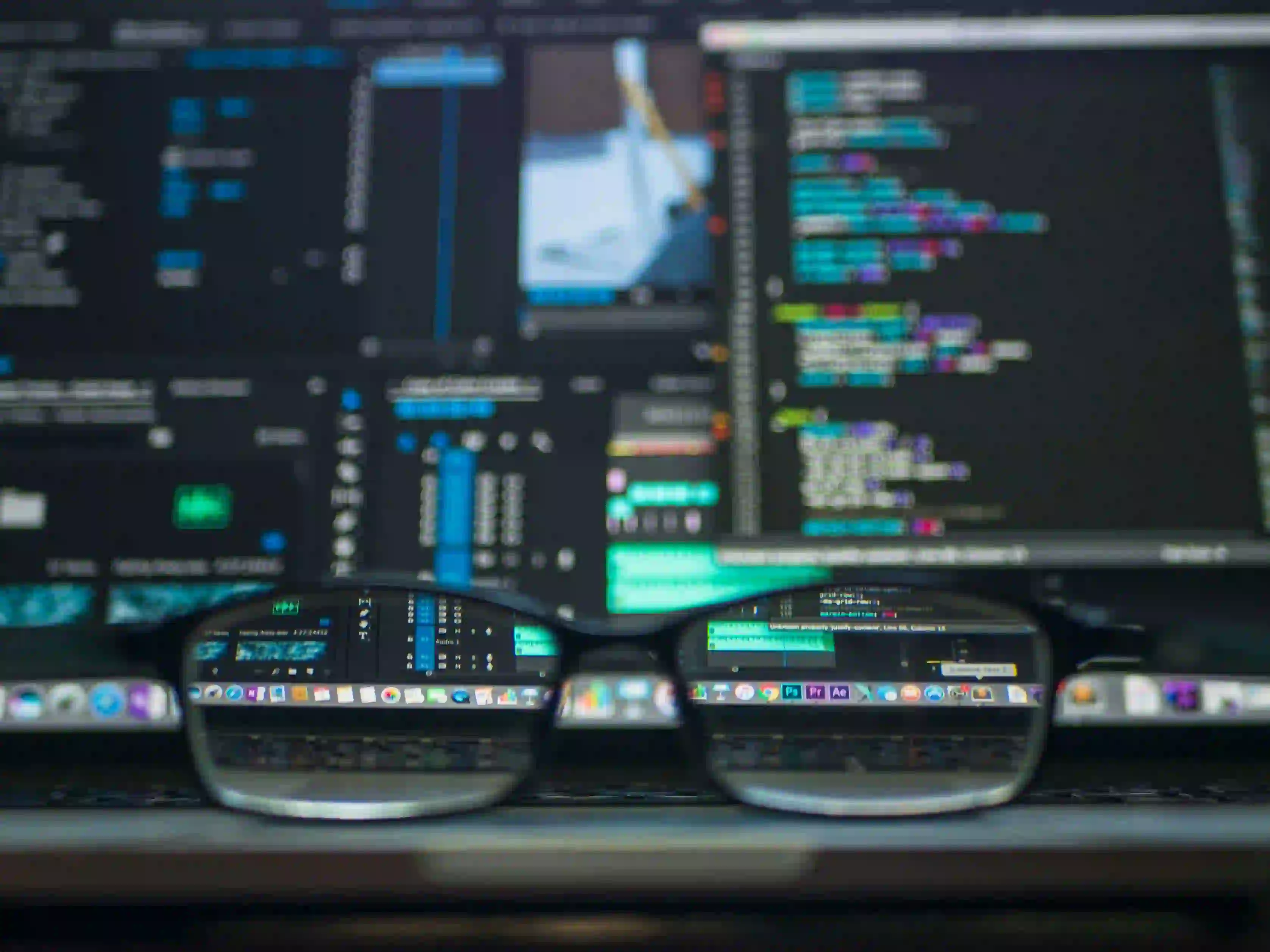
Common Mistakes When Dockerizing Java Applications
Docker has transformed the way developers build, deploy, and manage applications. However, even with this advancement, many developers still encounter pitfalls when they attempt to containerize Java applications. This post aims to highlight these common mistakes and provide insights on how to avoid them.
Understanding Docker Basics
Before diving into the mistakes, let us quickly cover some foundations of Docker. At its core, Docker allows you to pack and distribute applications in containers. This enables consistent environments across development, testing, and production.
Key Concepts of Docker
- Images: Read-only templates used to create containers. They contain everything needed to run an application—code, libraries, and environment variables.
- Containers: The running instances of Docker images.
- Dockerfile: A script containing commands to assemble an image.
Benefits of Docker for Java Applications
- Consistent Environments: Eliminates the "it works on my machine" problem.
- Version Control: Each image can be versioned and rolled back easily.
- Scalability: Encourages microservices architecture by facilitating the creation of isolated, scalable services.
Now, let’s dive into the common mistakes when dockerizing Java applications.
Common Mistakes When Dockerizing Java Applications
1. Using the Wrong Base Image
One of the most common mistakes is selecting the wrong base image. Developers often use heavy images, unnecessarily bloating their containers.
Example:
FROM openjdk:8
While using OpenJDK is okay, consider using a slimmed-down version.
Better Approach:
FROM openjdk:8-jdk-slim
This smaller base image reduces your container size significantly and improves deployment speed.
Why It Matters
A smaller base image results in faster build time, quicker deployments, and lower bandwidth consumption for pulling images.
2. Copying Unnecessary Files
Many developers copy entire directories into the Docker image, inadvertently including files not required for the application to run.
Wrong Approach:
COPY ./ ./
This command copies everything from the current local directory into the image.
Better Approach:
COPY ./target/myapp.jar /app/myapp.jar
This command specifically copies only the necessary JAR file.
Why It Matters
By copying only the required files, you minimize the image size, making it quicker to build and deploy.
3. Not Using Multi-Stage Builds
When building Java applications, it’s common to end up with a bloated final image. This can be avoided by using multi-stage builds.
Example:
# First stage: build the application
FROM maven:3.6-jdk-11 AS build
WORKDIR /app
COPY pom.xml .
COPY src ./src
RUN mvn clean package
# Second stage: create the runtime image
FROM openjdk:11-jre-slim
COPY --from=build /app/target/myapp.jar /app/myapp.jar
CMD ["java", "-jar", "/app/myapp.jar"]
Why It Matters
Multi-stage builds help you separate the build environment from the runtime environment, resulting in a leaner final image. This method enhances security and performance.
4. Not Managing Dependencies Properly
Java applications often have numerous dependencies. Not managing them can lead to bloated images and increased vulnerabilities.
Example:
Using the default dependency resolution with Maven or Gradle can result in unnecessary components being added to your image.
Better Practice
Consider using tools like Jlink for creating a custom runtime image with only the necessary modules.
5. Hardcoding Configuration Values
Many developers fall into the trap of hardcoding configuration values directly in their application. This makes the application less flexible and harder to manage across different environments.
Example:
String dbUrl = "jdbc:mysql://localhost:3306/mydb"; // hardcoded value
Better Practice
Utilize environment variables to maintain different configurations across multiple environments.
Example:
String dbUrl = System.getenv("DB_URL");
This makes your application more adaptable and maintainable. You can set the DB_URL
environment variable when you run your container.
6. Ignoring Java Best Practices
Even when dockerizing your application, you should not ignore Java best practices. For example, not using asynchronous processing can lead to high latency in response times.
7. Poor Resource Management
Java applications can be resource-intensive. Not properly configuring resource limits in Docker can lead to performance degradation.
Example:
docker run -d my-java-app
Better Practice
Specify resource limits using the --memory
and --cpus
flags.
docker run -d --memory="512m" --cpus="1" my-java-app
Why It Matters
Resource constraints prevent your application from consuming all compute resources, benefiting both your application and your host system.
8. Skipping Health Checks
Failing to add health checks to your Docker configuration can lead to situations where unhealthy containers are running without being detected.
Example:
HEALTHCHECK CMD curl --fail http://localhost:8080/actuator/health || exit 1
This command checks the health endpoint of a Spring Boot application to ensure that it is running as expected.
Why It Matters
By implementing health checks, you can automatically restart unhealthy containers, enhancing the reliability of your service.
9. Not Keeping Images Up to Date
Once a Docker image is built and deployed, many developers neglect to keep the images updated. Outdated images may contain security vulnerabilities and inefficiencies.
Best Practice
Regularly update the base images and libraries within your Dockerfile. Use tools like Docker Hub's automated builds to streamline this process.
10. Failing to Use Docker Volumes Properly
Using Docker volumes improperly can lead to data loss, particularly when working with stateful applications.
Example:
docker run -d my-app
Better Approach
docker run -d -v mydata:/data my-app
Using volumes enables persistent data storage.
Lessons Learned
Dockerizing Java applications can significantly enhance your development workflow by providing consistent environments and scalable architectures. However, avoiding the aforementioned common mistakes is crucial for realizing these benefits fully.
By selecting the right base images, managing dependencies efficiently, and adhering to best practices for configuration and resource management, you can create a well-optimized container for your Java application.
For further reading on effective Docker usage, consider visiting the official Docker documentation or exploring Java's native support for Docker.
As with any technology, practice makes perfect. Continue to refine your containerization strategy, and you’ll see the immense rewards that Docker brings to your Java applications. Happy Dockerizing!