Common Pitfalls in Spring Cloud Microservices Implementation
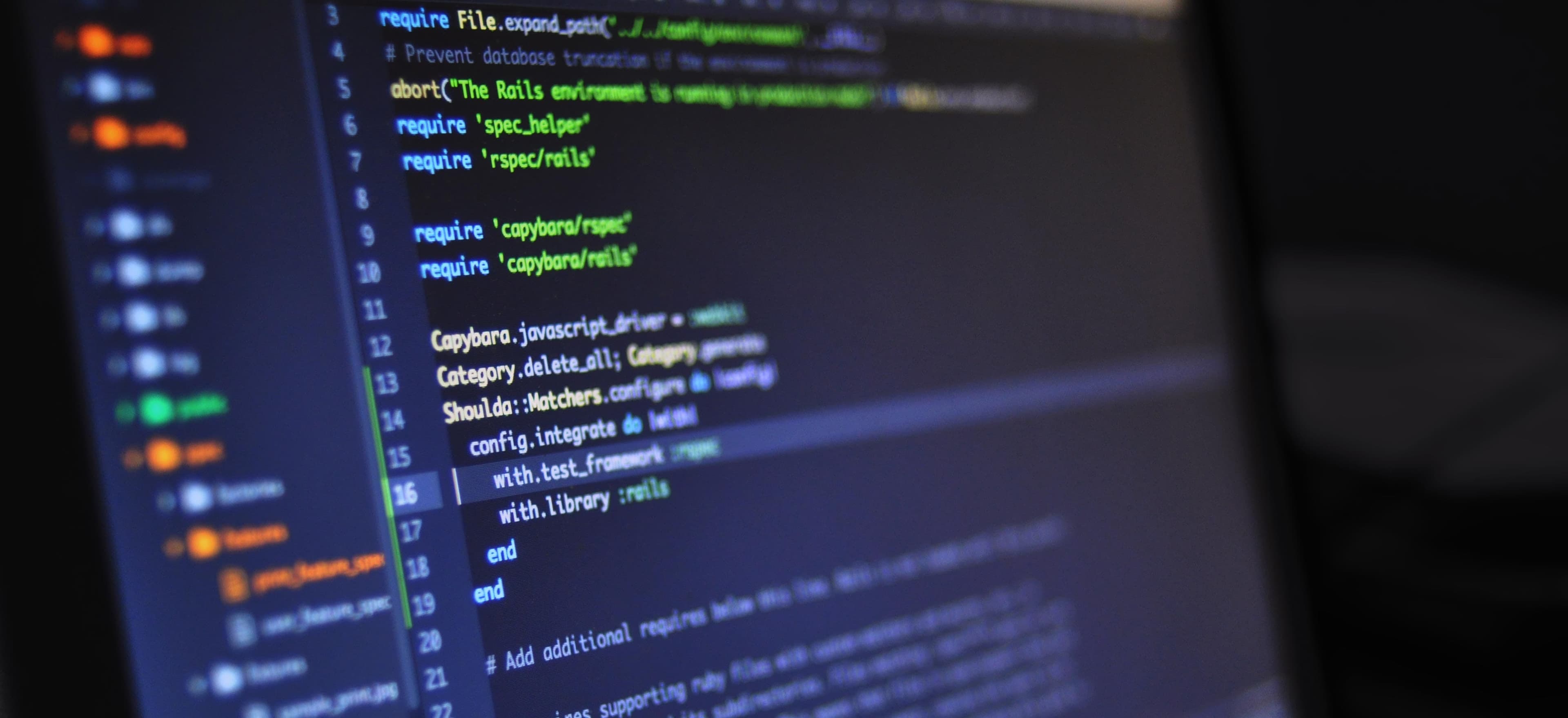
- Published on
Common Pitfalls in Spring Cloud Microservices Implementation
In the world of software development today, microservices architecture has become a dominant pattern, especially with frameworks like Spring Cloud. This approach enables developers to build and scale applications more efficiently by focusing on independently deployable services. Yet, with great flexibility comes great responsibility. Missteps during implementation can lead to inefficiencies and a hefty technical debt. In this post, we will delve into the common pitfalls in Spring Cloud microservices implementation and how to avoid them.
Pitfall #1: Insufficient Service Isolation
One of the primary motivations for adopting microservices is to isolate the services. However, inadequate separation can lead to tightly coupled components, which can negate the benefits of microservice architecture.
Solution:
Ensure that each microservice is responsible for its own data and logic. Implement bounded contexts to define clear boundaries. This practice simplifies deployments and updates while reducing the risk of cascading failures.
@RestController
@RequestMapping("/api/customer")
public class CustomerController {
// Autowired dependencies should be specific to this microservice
private final CustomerService customerService;
public CustomerController(CustomerService customerService) {
this.customerService = customerService;
}
@GetMapping("/{id}")
public ResponseEntity<Customer> getCustomer(@PathVariable Long id) {
return ResponseEntity.ok(customerService.getCustomerById(id));
}
}
In this code snippet, the CustomerController
is focused solely on customer-related tasks, adhering to the single responsibility principle. This isolation allows for easy updates and clearer maintenance paths.
Pitfall #2: Over-engineering with Too Many Services
While microservices are designed to break applications into smaller units, over-engineering can lead to unnecessary complexity. Many developers fall into the trap of creating too many microservices for trivial functionalities.
Solution:
Adopt a "fit-for-purpose" approach. Start with a few essential services and evaluate the need for additional microservices based on real use cases. Each service should have a clear, distinct purpose.
For instance, consider the following breakdown:
- User service: Handles user authentication and profile management.
- Product service: Manages products and related data.
- Order service: Facilitates order processing.
Creating separate services for highly composite functionalities can lead to excessive inter-service communication, which can affect performance and maintainability.
Pitfall #3: Inadequate Monitoring and Logging
Microservices consist of multiple services, making it crucial to have robust monitoring and logging solutions in place. Without proper observability, diagnosing issues becomes a daunting task.
Solution:
Implement centralized logging systems and monitoring tools. Utilize tools like Spring Cloud Sleuth and Zipkin for distributed tracing. They help trace requests as they move through various services, making it easier to identify bottlenecks or failures.
import org.springframework.cloud.sleuth.Tracer;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class TraceController {
private final Tracer tracer;
public TraceController(Tracer tracer) {
this.tracer = tracer;
}
@GetMapping("/trace")
public String tracingExample() {
// Adding custom tags for tracing
tracer.currentSpan().tag("customTag", "example");
// This method does something that needs to be traced
return "Tracing Example";
}
}
In this example, logging specific custom tags during service calls enhances traceability, allowing you to monitor service health effectively while gaining valuable insights into performance.
Pitfall #4: Ignoring API Gateway
Omitting an API gateway in microservices can lead to multiple challenges, including direct service communication from clients, which can lead to security vulnerabilities and data leakage.
Solution:
Implement an API Gateway pattern to manage requests. This approach allows you to apply cross-cutting concerns like authentication, rate limiting, and caching at the gateway level rather than in each service.
Example using Spring Cloud Gateway:
spring:
cloud:
gateway:
routes:
- id: customer_service
uri: lb://CUSTOMER-SERVICE
predicates:
- Path=/api/customer/**
Here, the Spring Cloud Gateway routes requests to the appropriate customer service, allowing centralized management of all routes. This setup streamlines client-side interactions while enhancing security.
Pitfall #5: Lack of Fault Tolerance
Microservices are inherently distributed systems, and network issues are inevitable. If your services aren't designed to handle failures gracefully, it can lead to a poor user experience.
Solution:
Incorporate fault tolerance patterns such as circuit breakers. Using Netflix Hystrix or Resilience4j provides automatic fallbacks and prevents cascading failures when a service is unresponsive.
import io.github.resilience4j.circuitbreaker.annotation.CircuitBreaker;
import org.springframework.stereotype.Service;
@Service
public class OrderService {
@CircuitBreaker
public Order getOrderDetails(String orderId) {
// Code that might fail
return OrderRepository.findOrder(orderId);
}
}
With the @CircuitBreaker
annotation, the method call will automatically switch to a fallback method if it fails repeatedly. This approach shields other services from failures and provides a seamless experience to users.
Pitfall #6: Not Considering Data Consistency
In a microservices architecture, ensuring data consistency is challenging due to the distributed nature of the system. Using a single database for all services contradicts the very essence of microservices.
Solution:
Adopt eventual consistency patterns. Leverage asynchronous messaging systems like Kafka or RabbitMQ for inter-service communication. This enables microservices to remain decoupled while ensuring data events are propagated correctly.
Example using Spring Kafka:
import org.springframework.kafka.annotation.KafkaListener;
import org.springframework.stereotype.Service;
@Service
public class NotificationService {
@KafkaListener(topics = "order-events", groupId = "notification-group")
public void listenOrderEvents(OrderEvent event) {
// Handle the incoming order event
// For example, send a notification
}
}
By decoupling services through event-based communication, you ensure that even if one service fails, others can still operate normally, thus promoting resilience in the overall system.
Closing Remarks
While Spring Cloud microservices offer immense benefits, avoiding common pitfalls is critical for maintaining efficiency and scalability. From ensuring service isolation to implementing robust monitoring, each aspect of design and implementation must be handled meticulously.
By addressing these challenges proactively, organizations can harness the full potential of microservices, leading to resilient, maintainable, and scalable applications. For further information on building microservices with Spring Cloud, you may find Spring Cloud Official Documentation and Microservices Patterns especially helpful.
With diligence and strategic planning, your journey towards mastering Spring Cloud microservices can yield remarkable results. Whether you are just beginning or reevaluating your existing architecture, avoiding common pitfalls will pave the way for a successful microservices implementation.