Common Pitfalls in Reactive Programming with Spring WebFlux
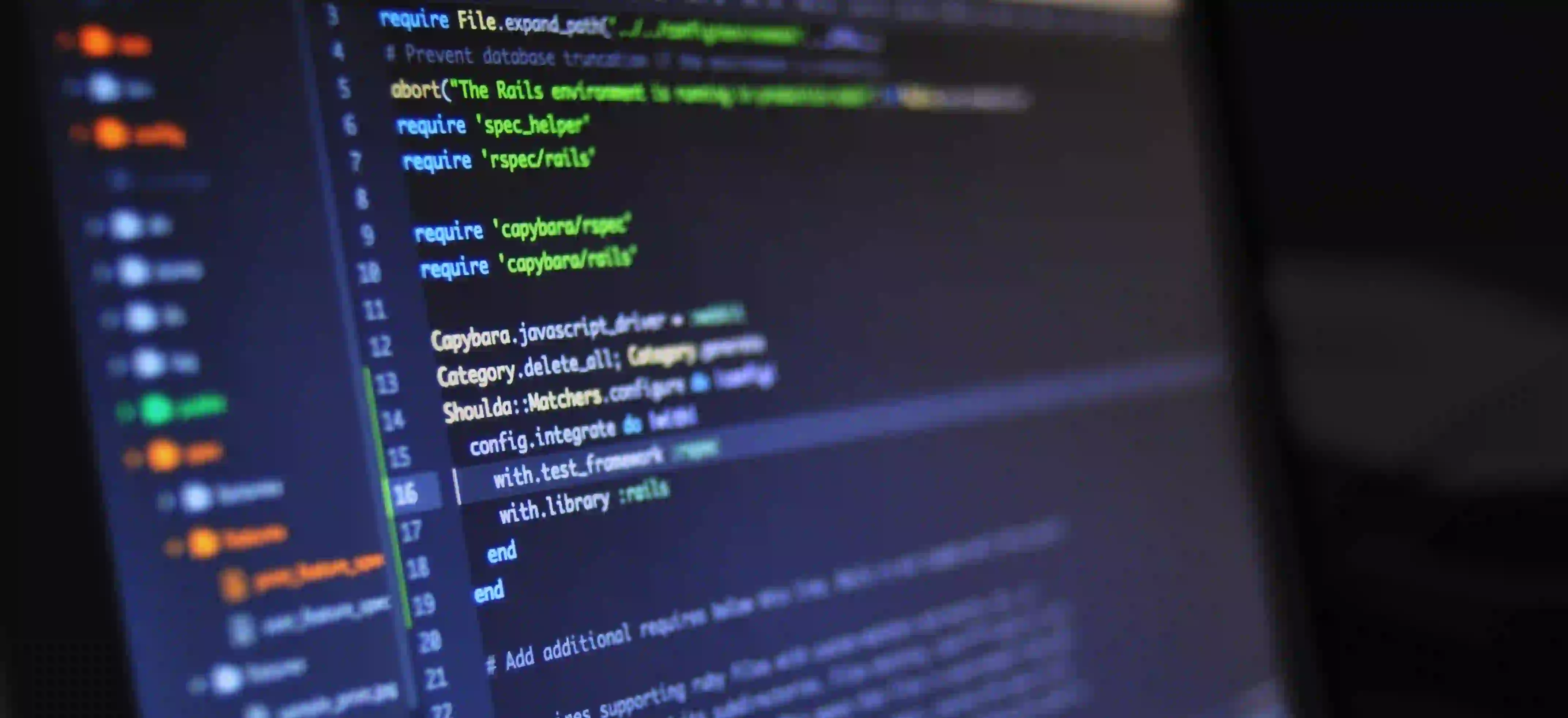
Common Pitfalls in Reactive Programming with Spring WebFlux
Reactive Programming has gained significant traction in recent years, especially with the rise of Spring WebFlux. While it offers tremendous advantages like non-blocking I/O operations and improved scalability, it isn't without its challenges. This blog post aims to delve into some common pitfalls you may encounter while utilizing Reactive Programming in Spring WebFlux, offering insights and code snippets along the way to illustrate these concepts effectively.
What is Spring WebFlux?
Spring WebFlux is a module in the Spring Framework that supports reactive programming by providing an asynchronous, non-blocking framework. It’s built on Project Reactor and works seamlessly with reactive data stores, allowing developers to build robust and scalable applications.
For a deeper dive into what Spring WebFlux entails, refer to the official Spring documentation.
1. Blocking Calls in a Reactive Ecosystem
One of the most common pitfalls in Spring WebFlux is including blocking calls in a reactive stream. Blocking operations can lead to degraded performance, undermining the very essence of a reactive system.
Code Snippet
@GetMapping("/data")
public Mono<Data> getData() {
Data data = blockingDataFetch(); // This blocks the thread, defeating the purpose
return Mono.just(data);
}
Why It's Problematic
In the code above, blockingDataFetch()
executes a blocking operation. This isn't merely inefficient; it can significantly slow down the entire application by consuming valuable threads from the reactive pool.
Solution: Use non-blocking alternatives or wrap blocking calls inside a separate scheduler.
@GetMapping("/data")
public Mono<Data> getData() {
return Mono.fromCallable(this::blockingDataFetch)
.subscribeOn(Schedulers.boundedElastic()); // Using a bounded elastic scheduler
}
2. Ignoring Backpressure
Another common oversight is the neglect of backpressure management in your reactive pipeline. Backpressure refers to the ability of a consumer to signal to a producer to slow down.
Code Snippet
Flux.range(1, 100)
.map(this::processData)
.subscribe(data -> {
// Handle processed data
});
Why It's Problematic
In this example, if processData
takes longer than the emission rate of the data from Flux.range()
, it leads to memory consumption issues and potential application crashes.
Solution: Use operators that handle backpressure effectively, such as onBackpressureBuffer
or onBackpressureDrop
.
Flux.range(1, 100)
.onBackpressureBuffer(10) // Buffer up to 10 requests
.map(this::processData)
.subscribe(data -> {
// Handle processed data
});
3. Mixing Reactive and Non-Reactive Programming
Hybrid approaches may seem appealing but can lead to complications. Mixing blocking calls with non-blocking calls can break the flow of your application.
Code Snippet
@GetMapping("/combined")
public Mono<Data> getCombinedData() {
Mono<Data> reactiveData = getReactiveData();
Data blockingData = getBlockingData(); // Mixing reactive and blocking
return reactiveData.map(data -> combine(data, blockingData));
}
Why It's Problematic
This mix creates confusion and hinders the performance of your application. It's vital to preserve the non-blocking nature throughout.
Solution: Keep everything within a reactive context.
@GetMapping("/combined")
public Mono<Data> getCombinedData() {
Mono<Data> reactiveData = getReactiveData();
return reactiveData.flatMap(data -> getBlockingDataMono().map(blockingData -> combine(data, blockingData)));
}
private Mono<Data> getBlockingDataMono() {
return Mono.fromCallable(() -> getBlockingData())
.subscribeOn(Schedulers.boundedElastic());
}
4. Not Leveraging Operators Effectively
Spring WebFlux is rich in operators. Failing to utilize them can lead to inefficient data processing.
Code Snippet
public Flux<Data> fetchAndProcessData() {
return fetchData().filter(this::isValid).map(this::transform);
}
Why It's Problematic
In this example, there’s a lack of operators that can be used for better performance, like flatMap
for concurrent processing.
Solution: Consider using flatMap
for concurrent execution of mapped functions.
public Flux<Data> fetchAndProcessData() {
return fetchData()
.filter(this::isValid)
.flatMap(this::transformAsync); // Ideal for concurrent processing
}
5. Not Handling Errors Appropriately
Error handling can be tricky in a reactive setup. A common problem is neglecting to implement error handling in the reactive chain.
Code Snippet
public Mono<Data> getData() {
return fetchData()
.map(this::processData)
.subscribeOn(Schedulers.parallel());
}
Why It's Problematic
In the above code snippet, if an exception occurs in the fetchData()
or processData()
functions, the error will propagate silently unless explicitly handled.
Solution: Use operators like onErrorResume
to handle exceptions effectively.
public Mono<Data> getData() {
return fetchData()
.map(this::processData)
.onErrorResume(e -> handleError(e))
.subscribeOn(Schedulers.parallel());
}
6. Inefficient Use of Network Calls
One more frequent issue arises from inefficient utilization of network calls. Making multiple asynchronous calls without considering the implications can lead to unnecessary overhead.
Code Snippet
@GetMapping("/user/{id}/data")
public Mono<Data> getUserData(@PathVariable String id) {
Mono<User> userMono = userService.getUser(id);
Mono<Details> detailsMono = detailsService.getDetails(id);
return userMono.flatMap(user -> detailsMono.map(details -> combine(user, details)));
}
Why It's Problematic
If both services hit the network sequentially, it increases response time unnecessarily.
Solution: Use zip
or merge
to make concurrent network calls.
@GetMapping("/user/{id}/data")
public Mono<Data> getUserData(@PathVariable String id) {
Mono<User> userMono = userService.getUser(id);
Mono<Details> detailsMono = detailsService.getDetails(id);
return Mono.zip(userMono, detailsMono)
.map(tuple -> combine(tuple.getT1(), tuple.getT2()));
}
A Final Look
Avoiding these common pitfalls will enhance your experience with Reactive Programming in Spring WebFlux. By blocking calls, managing backpressure, maintaining a completely reactive approach, leveraging powerful operators, handling errors gracefully, and optimizing network calls, you can build efficient and scalable applications.
For more information on reactive programming concepts, consider reading Reactive Programming with Spring and the Project Reactor documentation.
If you're exploring the world of Reactive Programming, remember to embrace its asynchronous nature. Take full advantage of available tools and techniques to create not just functional code but a well-optimized application. Happy coding!