Managing Singleton Complexity in Java: Best Practices
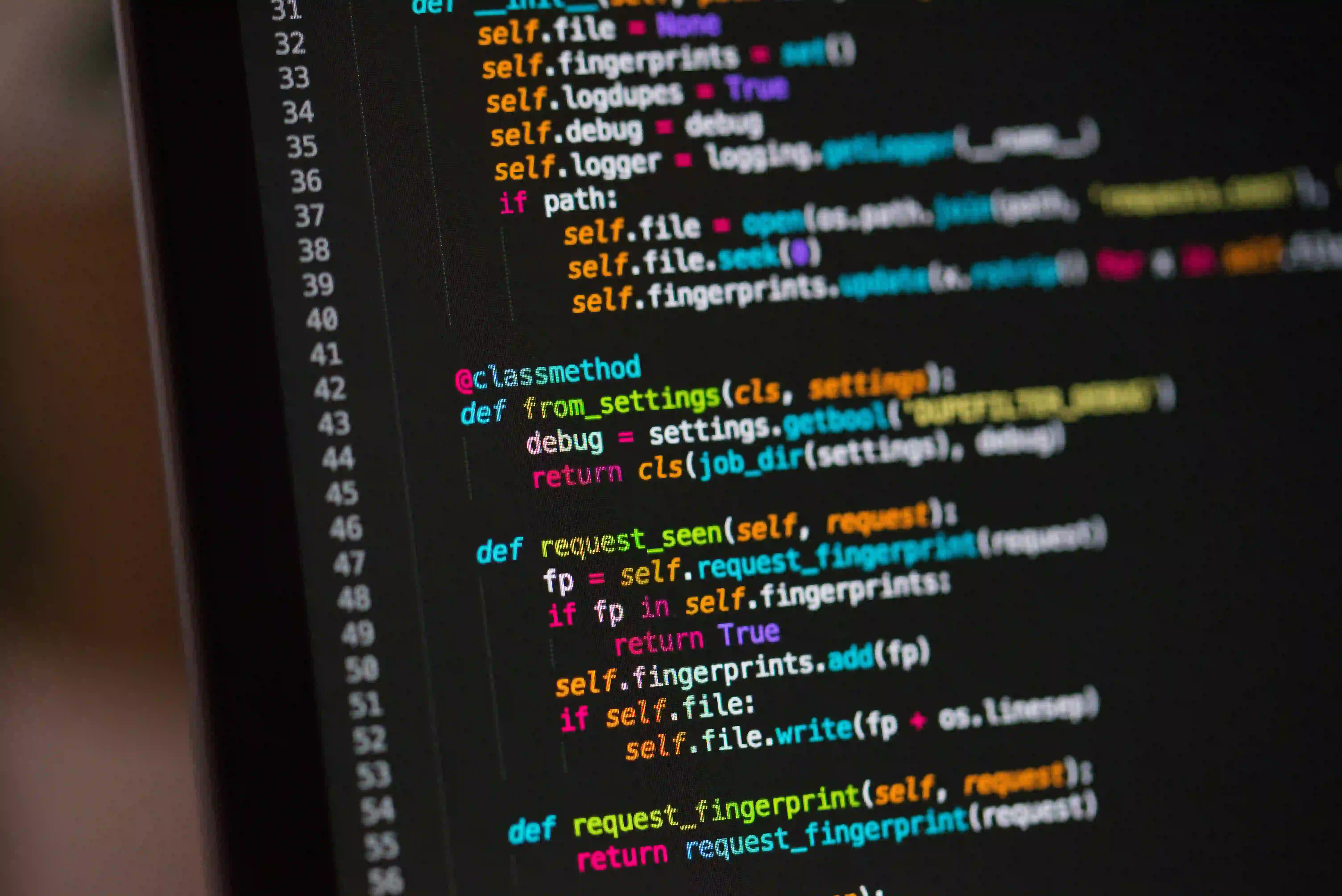
Managing Singleton Complexity in Java: Best Practices
In software design, the Singleton pattern is one of the most well-known creational patterns. It ensures that a class only has one instance and provides a global point of access to that instance. However, implementing Singletons can introduce unnecessary complexities, especially in concurrent applications or when testing. In this blog post, we’ll explore the best practices to manage Singleton complexity in Java, including common pitfalls and effective solutions.
What is a Singleton?
A Singleton is a design pattern that restricts a class from instantiating multiple objects. The Singleton pattern is particularly useful when exactly one object is needed to coordinate actions across a system.
Key Characteristics of Singleton
- Single Instance: Only one instance of the class exists.
- Global Access: The instance is accessible globally.
- Lazy or Eager Initialization: The instance can either be created when first needed (lazy) or at the start of the application (eager).
Basic Example of Singleton in Java
To understand the Singleton pattern better, let’s implement a basic version.
Eager Initialization
public class EagerSingleton {
// Creating instance at the time of class loading
private static final EagerSingleton INSTANCE = new EagerSingleton();
// Private constructor to prevent instantiation
private EagerSingleton() {
}
// Global access point
public static EagerSingleton getInstance() {
return INSTANCE;
}
}
Lazy Initialization
public class LazySingleton {
private static LazySingleton instance;
// Private constructor to prevent instantiation
private LazySingleton() {
}
// Global access point with synchronized method
public static synchronized LazySingleton getInstance() {
if (instance == null) {
instance = new LazySingleton();
}
return instance;
}
}
In these examples, the EagerSingleton initializes the instance at load time, whereas the LazySingleton creates it only when necessary. While lazy initialization is effective in terms of memory consumption, it presents concurrency issues if accessed by multiple threads, which brings us to our next topic.
Handling Concurrency
One of the primary challenges with the Singleton pattern is managing access in concurrent environments. When multiple threads attempt to access the lazy initialization method, it can lead to the creation of multiple instances.
Double-Checked Locking Pattern
We can improve the LazySingleton implementation with double-checked locking.
public class DoubleCheckedLockingSingleton {
private static volatile DoubleCheckedLockingSingleton instance;
private DoubleCheckedLockingSingleton() {
}
public static DoubleCheckedLockingSingleton getInstance() {
if (instance == null) {
synchronized (DoubleCheckedLockingSingleton.class) {
if (instance == null) {
instance = new DoubleCheckedLockingSingleton();
}
}
}
return instance;
}
}
Explanation
- Volatile keyword: Prevents instruction reordering and ensures visibility of changes across threads.
- First Check: The first if-check prevents unnecessary synchronization.
- Synchronized Block: The synchronized block protects the instance creation.
This pattern effectively ensures that the singleton instance is created only when needed and does so in a thread-safe manner.
Safe Lazy Initialization with Holder Classes
An alternative to double-checked locking is using Holder Classes, a clean and thread-safe way to implement Singleton.
public class HolderClassSingleton {
private HolderClassSingleton() {
}
private static class SingletonHelper {
private static final HolderClassSingleton INSTANCE = new HolderClassSingleton();
}
public static HolderClassSingleton getInstance() {
return SingletonHelper.INSTANCE;
}
}
Why Use Holder Classes?
- Thread Safety: The instance is created only when the
getInstance()
method is called for the first time. - Simplicity: No complex synchronization code is needed.
- Performance: No overhead of synchronized methods after the instance is created.
Common Pitfalls in Singleton Implementation
While implementing the Singleton pattern, developers often face several pitfalls. Here are a few to keep in mind:
1. Serialization Issues
Singletons are sensitive to serialization. When a Singleton class implements Serializable
, it may create new instances during deserialization. To prevent this, add the following method:
protected Object readResolve() {
return getInstance();
}
2. Reflection
Reflection allows you to override the private constructor, thereby creating multiple instances. Consider adding the following check in the constructor:
if (instance != null) {
throw new IllegalStateException("Already instantiated.");
}
3. Static Nested Classes
Static nested classes can be a robust way to implement a Singleton. They are only loaded when referenced, making them memory efficient.
public class StaticNestedClassSingleton {
private StaticNestedClassSingleton() {
}
private static class SingletonHolder {
private static final StaticNestedClassSingleton INSTANCE = new StaticNestedClassSingleton();
}
public static StaticNestedClassSingleton getInstance() {
return SingletonHolder.INSTANCE;
}
}
Testing Singleton Classes
Singletons can often be challenging to test. If you've hard-coded dependencies or static calls, consider following these best practices:
- Use dependency injection frameworks (like Spring) that can manage singleton instances effectively.
- Separate concerns and reduce coupling by not tightly coupling singletons with business logic.
Final Considerations
While the Singleton pattern can be a useful tool in a developer's toolkit, its proper implementation is crucial to minimize complexity, ensure thread safety, and maintain consistency. By employing techniques like double-checked locking, Holder classes, and understanding serialization and reflection, developers can create effective Singleton implementations.
Avoid common pitfalls and leverage best practices while keeping your design simple and clear. With these guidelines, managing Singleton complexity in Java should become more straightforward, robust, and suited for your software development needs.
For more insights on design patterns and best practices in Java programming, check out:
- Design Patterns: Elements of Reusable Object-Oriented Software
- Java Concurrency in Practice
Implementing Singleton patterns effectively will not only improve your application's performance but also ensure that your architecture is clean, well-structured, and maintainable.
Happy coding!