Common Java Performance Bottlenecks and How to Fix Them
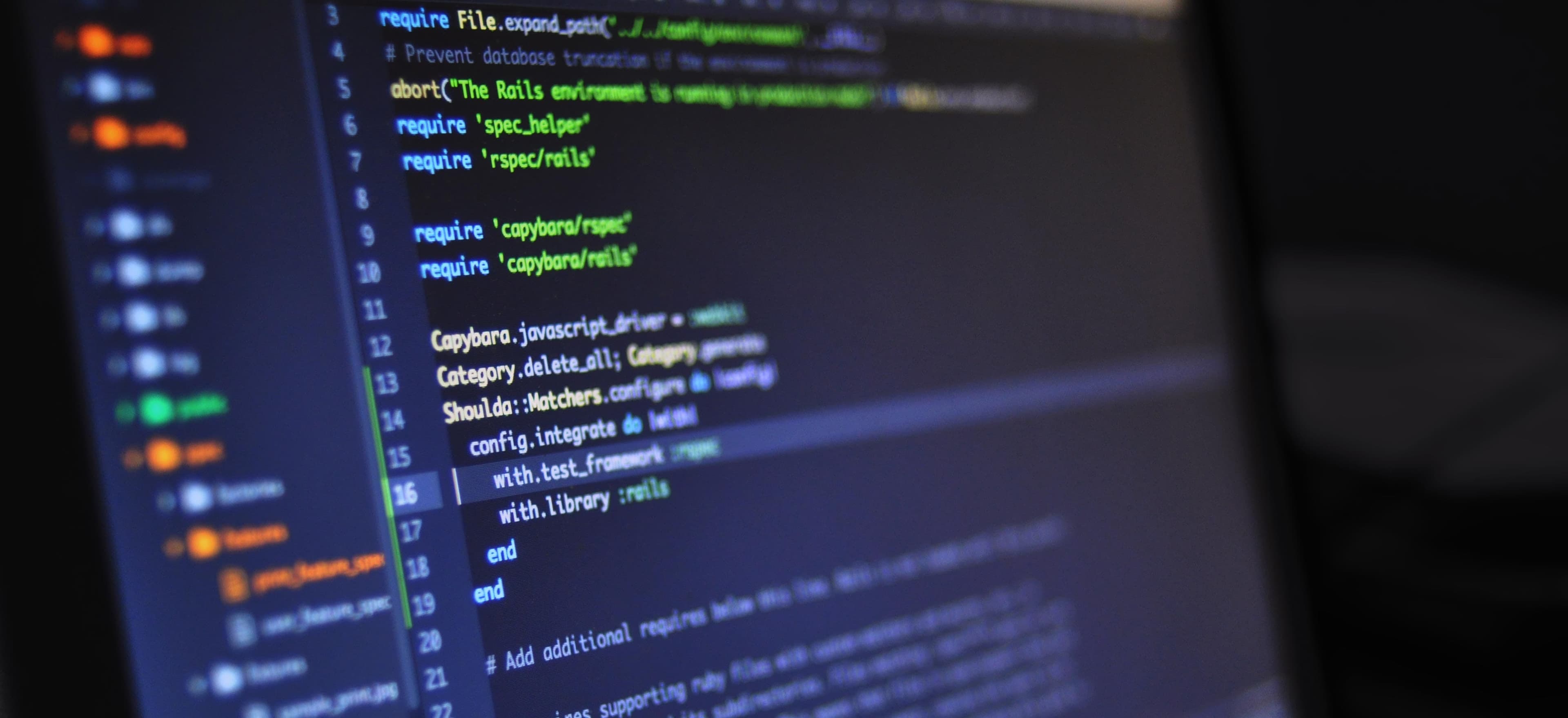
- Published on
Common Java Performance Bottlenecks and How to Fix Them
Java is known for its robustness, portability, and performance. However, even the most skilled Java developers can encounter performance bottlenecks. Understanding and addressing these bottlenecks is crucial for ensuring optimal application performance.
In this blog post, we will explore some of the most common performance bottlenecks in Java applications and discuss how to effectively fix them. Whether you're a beginner or an advanced Java developer, you will find valuable insights to improve the performance of your applications.
Table of Contents
- Understanding Performance Bottlenecks
- Common Java Performance Bottlenecks
- Memory Management Issues
- Inefficient Algorithms
- Poor Database Access Patterns
- Thread Synchronization Problems
- Tips to Improve Java Performance
- Conclusion
Understanding Performance Bottlenecks
A performance bottleneck occurs when a particular component of an application cannot keep up with the workload, leading to a slow-down in overall performance. This can impact response times, enhance latency, or result in resource contention. Identifying bottlenecks is critical in optimizing application behavior and enhancing user experience.
Common Java Performance Bottlenecks
1. Memory Management Issues
Java's garbage collection (GC) is a double-edged sword. While it helps manage memory automatically, it can also introduce latency due to frequent pauses during collection.
Solution: Tune Garbage Collection Settings
One way to reduce GC-related bottlenecks is to tune JVM parameters. For example, modifying the heap size and selecting a suitable garbage collector can make a significant difference.
java -Xms512m -Xmx2g -XX:+UseG1GC -XX:MaxGCPauseMillis=100 -jar your-application.jar
-Xms
: Initial heap size-Xmx
: Maximum heap size-XX:+UseG1GC
: Use the G1 Garbage Collector-XX:MaxGCPauseMillis
: Set an upper limit for pause times during garbage collection
Why: Proper tuning can help balance memory allocation and garbage collection cycles, reducing the impact on application performance.
2. Inefficient Algorithms
Sometimes the answer isn't in Java's vast frameworks or libraries but in how the algorithms are implemented. Using inefficient algorithms can lead to excessive CPU consumption and sluggish performance.
Solution: Optimize Algorithms and Data Structures
When dealing with large datasets, consider the complexity of your algorithms. For example, using a HashMap
instead of an ArrayList
for lookups can significantly enhance performance.
Map<String, Integer> frequencyMap = new HashMap<>();
for (String word : words) {
frequencyMap.put(word, frequencyMap.getOrDefault(word, 0) + 1);
}
Why: The HashMap
allows for average-case O(1) time complexity for inserts and lookups, whereas an ArrayList
would require O(N) time. Efficient selection of data structures can drastically reduce your code's execution time.
3. Poor Database Access Patterns
Database calls can often be a source of latency, especially when they are not executed efficiently. Issues may arise from excessive calls, lack of proper indexing, or excessive data fetching.
Solution: Use Connection Pooling and Optimize Queries
Implementing connection pooling reduces the overhead associated with establishing connections. Additionally, optimizing your SQL queries is critical.
Here is an example of using a connection pool:
import org.apache.commons.dbcp2.BasicDataSource;
BasicDataSource dataSource = new BasicDataSource();
dataSource.setUrl("jdbc:mysql://localhost:3306/mydb");
dataSource.setUsername("user");
dataSource.setPassword("password");
dataSource.setMaxTotal(10); // Pool size
Connection connection = dataSource.getConnection();
Why: Connection pooling minimizes connection overhead, allowing your application to handle multiple requests without delay. It can be beneficial to employ prepared statements to reduce query parsing time.
4. Thread Synchronization Problems
Concurrency issues can lead to performance bottlenecks, especially when multiple threads are fighting for the same resources. This may lead to contention, deadlock situations, or inefficient thread management.
Solution: Use Concurrency Utilities
Using Java’s concurrency utilities like java.util.concurrent
can simplify thread management while enhancing performance.
ExecutorService executor = Executors.newFixedThreadPool(10);
for (int i = 0; i < 100; i++) {
executor.submit(() -> {
// Your task here
});
}
executor.shutdown();
Why: Utilizing an ExecutorService
allows for better resource management, leading to multi-threading without overhead. This can lead to more efficient CPU use and reduced latency.
Tips to Improve Java Performance
- Profile Your Application: Use tools like VisualVM or JProfiler to analyze performance.
- Monitor Memory Usage: Keep an eye on memory consumption through tools like JConsole.
- Avoid Premature Optimization: Optimize only the sections of your code that are critical for performance.
- Use the Latest Java Version: Newer versions include performance improvements and better garbage collection strategies.
Bringing It All Together
Performance bottlenecks in Java can frustrate even the most experienced developers. By understanding common issues related to memory management, algorithm selection, database access, and thread synchronization, you can significantly improve the performance of your applications.
Always remember that performance optimization is a continuous process. Regularly profiling your application, monitoring its performance, and iterating on your optimizations will enable you to maintain a high-performance Java application.
For further reading on Java performance tuning, refer to documentation such as the Java Performance Tuning Guide or consult resources like Java Concurrency in Practice for deeper insights into concurrency challenges.
By taking proactive steps related to the tips and solutions provided in this guide, you will be well-equipped to tackle any performance issue in your Java applications efficiently. Happy coding!