Common Pitfalls in Java Memory Model and Thread Safety
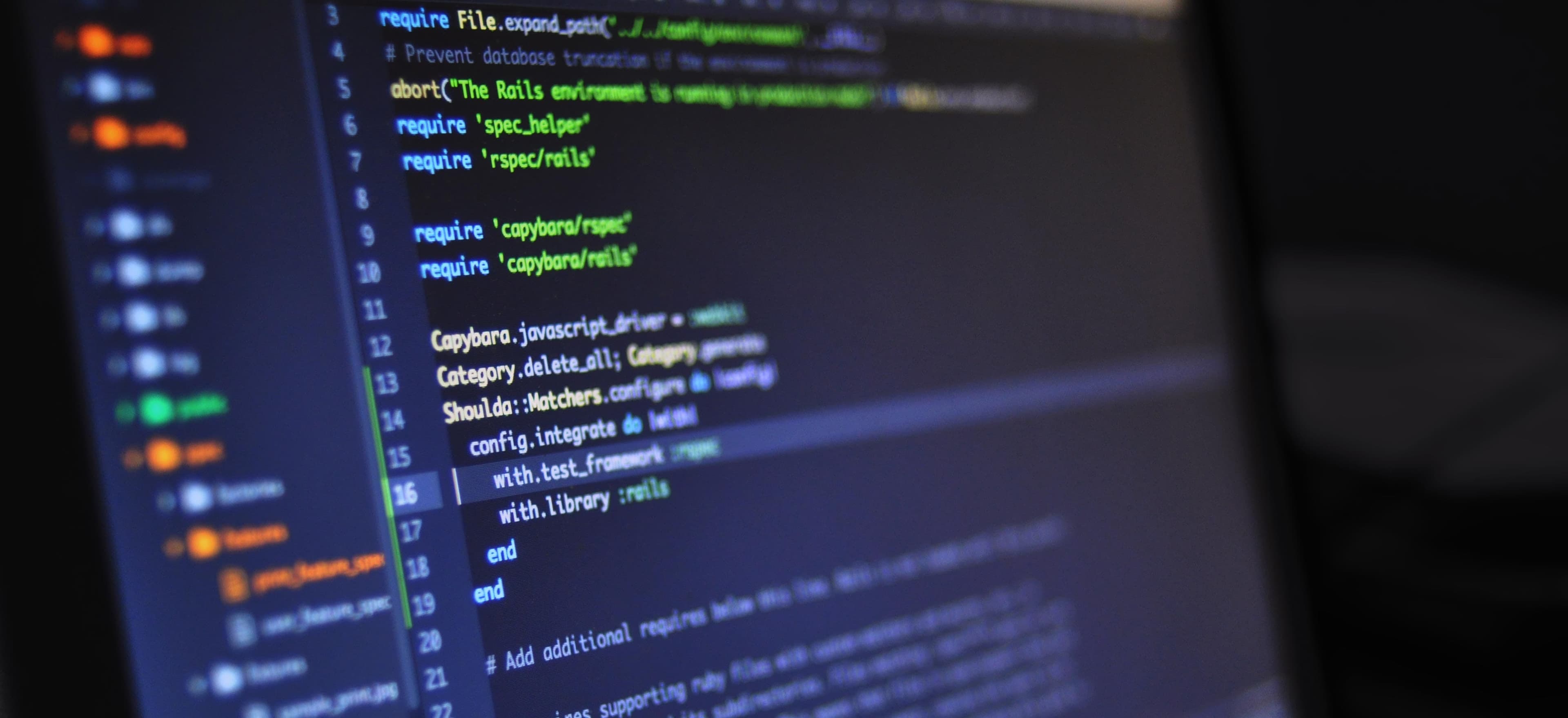
- Published on
Common Pitfalls in Java Memory Model and Thread Safety
The Java programming language has a rich feature set that supports concurrent programming, yet it also comes with its own set of challenges regarding memory management and thread safety. Understanding the Java Memory Model (JMM) is essential for writing efficient and safe concurrent applications. In this blog post, we will explore the common pitfalls developers encounter related to the JMM and thread safety while providing practical code examples and solutions.
Understanding the Java Memory Model
The Java Memory Model defines how threads interact through memory and what behaviors are allowed in concurrent execution. It acts as an abstraction, ensuring that the code behaves consistently across different platforms.
Key Concepts of the Java Memory Model
- Visibility: Guarantees that changes made by one thread are visible to others.
- Atomicity: Ensures that operations are atomic, meaning they're indivisible and will complete fully or not at all.
- Ordering: Refers to the sequence in which actions are performed by threads.
Pitfall #1: Not Using volatile
for Shared Variables
One of the most common pitfalls in Java is failing to declare shared variables as volatile
. When a variable is marked as volatile
, it guarantees visibility across threads. Without volatile
, changes made by one thread may not be visible to other threads, leading to unpredictable results.
public class VolatileExample {
private volatile boolean running = true;
public void run() {
while (running) {
// Do some work
}
}
public void stop() {
running = false;
}
}
Why use volatile
?
In this code snippet, the running
variable is declared as volatile
. This ensures that changes made in one thread (like the stop
method) are immediately visible to the other thread (like the run
method). Without it, the run
method may get stuck in an infinite loop due to thread-local caching of the variable state.
For more detailed insights into volatile
, refer to Oracle’s Java Volatile Documentation.
Pitfall #2: Improper Use of Synchronized Blocks
Using synchronized blocks to control access to shared resources is another common practice. However, misuse can lead to deadlocks or reduced performance.
public class SynchronizedExample {
private final Object lock = new Object();
public void safeMethod() {
synchronized (lock) {
// Critical section
}
}
}
When to use synchronization? The synchronized block in this example ensures that only one thread can access the critical section at a time. However, overusing synchronization can lead to contention and performance bottlenecks. It’s essential only to synchronize the code that needs protection.
Pitfall #3: Incorrectly Implementing Double-Checked Locking
Double-checked locking is a common optimization technique but is often implemented incorrectly. Here is how it should not be done:
public class Singleton {
private static Singleton instance;
public static Singleton getInstance() {
if (instance == null) {
synchronized (Singleton.class) {
if (instance == null) {
instance = new Singleton();
}
}
}
return instance;
}
}
What’s wrong here?
In this example, the double-checked locking pattern does not provide the expected result due to memory visibility issues. The instance
variable might not be fully constructed before another thread reads it.
Correct Approach Using Initialization-on-Demand Holder Idiom
A reliable way to implement the Singleton pattern is to use the Initialization-on-Demand Holder Idiom.
public class Singleton {
private Singleton() {}
private static class Holder {
private static final Singleton INSTANCE = new Singleton();
}
public static Singleton getInstance() {
return Holder.INSTANCE;
}
}
Why this approach is better?
This method is thread-safe and doesn’t require synchronization. The Holder
class is loaded only when getInstance()
is called, ensuring lazy initialization while maintaining performance.
Pitfall #4: Shared Mutable State
Another common pitfall is the misuse of shared mutable state, which can lead to hard-to-track bugs. If multiple threads modify a shared object simultaneously, inconsistencies can arise.
Consider the following example where two threads modify an object:
public class Counter {
private int count = 0;
public void increment() {
count++;
}
public int getCount() {
return count;
}
}
What’s wrong with this?
The increment
method is not synchronized, which means if two threads run this simultaneously, they may read the same count
value and increment it incorrectly.
The Solution: Use Atomic Classes
Using atomic classes from java.util.concurrent.atomic
, like AtomicInteger
, can significantly mitigate these issues.
import java.util.concurrent.atomic.AtomicInteger;
public class AtomicCounter {
private AtomicInteger count = new AtomicInteger(0);
public void increment() {
count.incrementAndGet();
}
public int getCount() {
return count.get();
}
}
Why atomic operations matter?
The AtomicInteger
ensures that the increment operation is performed atomically, eliminating the risk of inconsistent states across threads.
For a deeper dive into atomic variables, check out Oracle’s Official Documentation on Atomic Variables.
Pitfall #5: Relying on Thread Local Variables
Thread-local variables can be a quick way to avoid shared state issues, but relying on them too heavily can result in resource leaks or unexpected behaviors.
public class ThreadLocalExample {
private static ThreadLocal<Integer> threadLocalValue = ThreadLocal.withInitial(() -> 0);
public void incrementThreadLocal() {
threadLocalValue.set(threadLocalValue.get() + 1);
}
public int getThreadLocalValue() {
return threadLocalValue.get();
}
}
What to consider?
While threadLocalValue
provides a separate value for each thread, the resources it creates, like memory, must be cleared when no longer needed. Otherwise, they can lead to memory leaks.
The Closing Argument
The Java Memory Model and thread safety are critical aspects of concurrent programming in Java. Recognizing and avoiding common pitfalls—such as not using volatile
, incorrect synchronization, flawed singleton patterns, shared mutable states, and over-reliance on thread-local variables—can significantly improve your programs' correctness and robustness.
By considering the best practices and strategies presented in this blog post, you will be better equipped to handle the complexities of concurrent programming in Java. Remember that awareness of the Java Memory Model is not just a means to avoid errors; it is a pathway to writing high-performance and reliable applications.
For further reading on concurrency in Java, check out Java Concurrency in Practice by Brian Goetz.
Feel free to share your experiences with thread safety in Java or any questions you might have in the comments below!
Checkout our other articles